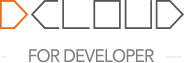
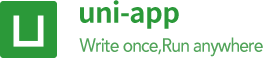
English
Documentation related to JQL syntax has been moved to: JQL syntax
Since
HBuilderX 2.9.5
, it is supported to directly useuniCloud.database()
method to obtain database references on the client side, that is, to operate the database directly on the front end, this function is calledclientDB
If users before
HBuilderX 2.9.5
have usedclientDB
, please delete the front-end library and cloud functions ofclientDB
after the upgrade. The new version has built-inclientDB
in the front-end and cloud.
Big vernacular: traditional database operations can only be implemented on the server side, because he has security problems in the front-end use. The cloud database of uniCloud has a table structure (DB Schema). Through simple js expressions, it configures: whether accounts with various role permissions can read and write certain standardized data, etc., which solves the security of front-end operations. problem; so the cloud database of uniCloud can be called directly on the front end.
Advantages of using clientDB
: **No need to write server code! **
Half of the workload of 1 application development is directly saved.
Of course, using clientDB
requires reversing the traditional concept of back-end development, no longer writing server-side code, and operating the database directly on the front-end. But for data security, DB Schema
needs to be configured on the database.
In DB Schema
, configure the permissions of data operations and field value domain validation rules to prevent inappropriate data reading and writing at the front end. See: DB Schema
If it is necessary to execute association logic on the cloud before or after database operations (for example, after obtaining article details, the number of article readings +1), clientDB
provides database trigger (from HBuilderX 3.6. 11 starts).
In the lower version that does not support database triggers, use action cloud function
Notice
clientDB
依赖uni-id(1.1.10+版本
)提供用户身份和权限校验,存在uni-id-common时clientDB会优先依赖uni-id-common,如果你不了解uni-id,请参考:uni-id文档,uni-id-common文档clientDB
依赖的uni-id需要在uni-id的config.json内添加uni-id相关配置,通过uni-id的init方法传递的参数不会对clientDB生效,参考:uni-id 配置,uni-id新版配置(uni-id-co + uni-id-common)clientDB
,需要获取不同角色用户拥有的权限(在权限规则内使用auth.permission),请先查阅:uni-id 角色权限,uni-id新版角色权限(uni-id-co + uni-id-common)Demo: online address book project, rendering cloud data to view
27 lines of code need to be written, as shown in the figure:
37 lines of code need to be written, as shown in the figure:
Traditional cloud-separated development methods, total: 64 lines of code.
Only: 5 lines of code as shown:
**Summary: Based on the uniCloud cloud collaborative development method, there is no need to write js code or server-side code. Write 6 lines of code directly in the view template to complete the effect that traditional development methods require 64 lines of code to complete. And it's not just a matter of code size. The experience of the entire development process has improved the development efficiency by more than 10 times. **
clientDB调用流程参考:JQL图解
The front end of clientDB
can be used in two ways. You can use the js API to operate cloud database, or you can use the <unicloud-db>
component.
js API can perform all database operations. The <unicloud-db>
component is a repackage of the js API, which further simplifies the amount of code for common database operations such as queries.
<unicloud-db>
component has been built in and can be used directly. Documentation see also: <unicloud-db>
componentThe following article focuses on the js API of clientDB
. As for the usage of components, see also documentation.
The client part of clientDB
is mainly responsible for providing API, allowing the front-end to write database operation instructions, and processing some fields that are not convenient for the client to represent, such as user ID (see the syntax extension section below for details)
clientDB
supports traditional nosql query syntax and adds jql
query syntax. jql
is an easier to use query syntax.
Traditional nosql query syntax example
This sample code directly queries the list
table of ApsaraDB for a front-end page, and specifies the query condition that the name
field value is hello-uni-app
, and the res in then is the returned query result.
// get db reference
const db = uniCloud.database() //代码块为cdb
db.collection('list')
.where({
name: "hello-uni-app" //传统MongoDB写法,不是jql写法。实际开发中推荐使用jql写法
}).get()
.then((res)=>{
// res is the database query result
}).catch((err)=>{
console.log(err.code); // 打印错误码
console.log(err.message); // 打印错误内容
})
jql query syntax example
// get db reference
const db = uniCloud.database() //代码块为cdb
db.collection('list')
.where('name=="hello-uni-app"')
.get()
.then((res)=>{
// res is the database query result
}).catch((err)=>{
console.log(err.code); // 打印错误码
console.log(err.message); // 打印错误内容
})
Instructions for use
The syntax of the front-end operation database is the same as that of cloud functions, but has the following limitations (the same is true when using jql syntax):
db.command.inc
, etc. cannot be used when updating the database{'a.b.c': 1}
, it needs to be written in the form of {a:{b:{c:1}}}
clientDB has two ways to obtain database reference uniCloud.database()
and uniCloud.databaseForJQL()
(newly added in HBuilderX 3.6.7). It is recommended to use the databaseForJQL
interface in the version that supports the databaseForJQL
interface, which is consistent with the return structure of the cloud jql extension library, which is convenient for code reuse
The database interface and databaseForJQL have the following differences
In the above example the structure of res is as follows
{
result: {
data: [{
xx: xx
}]
}
}
If you use the databaseForJQL interface, the res structure is as follows
{
data: [{
xx: xx
}]
}
databaseForJQL
is used as the interface name. For more information about the interceptor, please refer to: uniCloud InterceptorTransparently transmit the uni-id automatically refreshed token to the client
HBuilderX 3.2.11 and above, clientDB will automatically save the refreshed token and expiration time in storage.
usage
const db = uniCloud.database()
function refreshToken({
token,
tokenExpired
}) {
uni.setStorageSync('uni_id_token', token)
uni.setStorageSync('uni_id_token_expired', tokenExpired)
}
// Bind refresh token event
db.on('refreshToken', refreshToken)
// Unbind refresh token event
db.off('refreshToken', refreshToken)
Global clientDB error event, supported since HBuilderX 3.0.0.
usage
const db = uniCloud.database()
function onDBError({
code, // 错误码详见https://uniapp.dcloud.net.cn/uniCloud/jql.html#returnvalue
message
}) {
// handle errors
}
// Bind clientDB error event
db.on('error', onDBError)
// Unbind clientDB error events
db.off('error', onDBError)
clientDB uses JQL to write query statements on the client side. For the JQL syntax, please refer to: JQL syntax