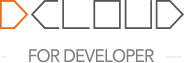
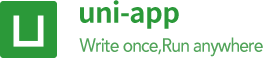
English
The <unicloud-db>
component is a database query component that repackages the js library of clientDB
.
The front-end directly obtains the data in the cloud database of uniCloud through components, and binds it to the interface for rendering.
In traditional development, developers need to define data on the front end, obtain interface data through request networking, and then assign values to data. At the same time, the backend also needs to write an interface to query the database and feedback data.
With the <unicloud-db>
component, the above works in just 1 line! Write the component, set the properties of the component, specify which tables, fields, and query conditions to check in the properties, and you're done!
Type the udb
code block in HBuilderX to get the following code, and then specify the table "table1" to be queried through the collection attribute, specify the field "field1" to be queried through the field attribute, and specify the data whose query id is 1 in the where attribute. The query result data can be directly rendered on the interface.
<unicloud-db v-slot:default="{data, loading, error, options}" collection="table1" field="field1" :getone="true" where="id=='1'">
<view>
{{ data}}
</view>
</unicloud-db>
The <unicloud-db>
component is especially suitable for display pages such as lists and details. Development efficiency can be greatly improved.
The query syntax of the <unicloud-db>
component is jql
, which is a more concise query syntax than sql statement and nosql syntax and more in line with the habits of js developers. You can easily master it without learning the front end of sql or nosql. See details in jql
The <unicloud-db>
component supports more than just queries. It also comes with add, remove, update methods, see the method section below
Platform differences and release notes
App | H5 | 微信小程序 | 支付宝小程序 | 百度小程序 | 抖音小程序、飞书小程序 | QQ小程序 | 快应用 | 360小程序 | 快手小程序 | 京东小程序 |
---|---|---|---|---|---|---|---|---|---|---|
√ | √ | √ | √ | √ | √ | √ | x | √ | √ | √ |
Requires HBuilderX 3.0+
<unicloud-db>
is upgraded from the original <uni-clientdb> plugin
. Since HBuilderX 3.0, <unicloud-db>
is built into the framework, regardless of the version of the Mini Program base library.
If you need to use the clientDB component in versions below HBuilderX3.0, you need to download the <uni-clientdb> plugin
from the plugin market separately. The download address is: https://ext.dcloud.net.cn/plugin?id=3256. However, it is still recommended to upgrade HBuilderX 3.0+.
property | type | description |
---|---|---|
v-slot:default | Query status (failed, online) and result (data) | |
ref | string | vue component reference tag |
spaceInfo | Object | Service space information, added in HBuilderX 3.2.11 . Same as uniCloud.init parameter, reference: uniCloud.init |
collection | string | Table name. Supports inputting multiple table names, which are separated by , . Since HBuilderX 3.2.6 , it also supports inputting an array composed of tempCollection |
field | string | Specifies the field to query, multiple fields are separated by , . If you don't write this property, it means to query all fields. Support renaming the returned field with oldname as newname |
where | string | Query conditions, filter records. see below |
orderby | string | Sort field and reverse order setting |
foreign-key | String | Manually specify the relationship to use, HBuilderX 3.1.10+ Details |
page-data | String | Pagination strategy selection. The value of add means that the data of the next page is appended to the previous data, which is often used to scroll to the end to load the next page; when the value is replace , the current data data is replaced, which is often used for PC-style interaction, and there are page numbers at the bottom of the list. button, default is add |
page-current | Number | Current page |
page-size | Number | Number of data per page |
getcount | Boolean | Whether to query the total number of data items, the default is false , when paging mode is required, specify true |
getone | Boolean | Specifies whether the query result only returns the first data of the array, the default is false. In the case of false, an array is returned. Even if there is only one result, it needs to be obtained by [0]. When the value is true, the result data is returned directly, with one less array, generally used for non-list pages, such as details page |
action | string | Before or after the database query is executed in the cloud, an action function is triggered to perform preprocessing or postprocessing. [Details](/uniCloud/uni-clientDB?id=%e4%ba%91%e7% ab%af%e9%83%a8%e5%88%86). Scenario: Data that the front end does not have permission to operate, such as the number of readings + 1 |
manual | Boolean | **Obsolete, use loadtime instead ** Whether to manually load data, the default is false, the data will be automatically loaded online when the page is onready. If set to true, you need to specify the timing to trigger networking through the method this.$refs.udb.loadData() , where udb refers to the ref value of the component. Generally, onLoad cannot get this.$refs.udb because the timing is too early, but it can be obtained in onReady |
gettree | Boolean | Whether to query tree structure data, HBuilderX 3.0.5+ Details |
startwith | String | The first level condition of gettree, this initial condition can be omitted, if startWith is not passed, the query starts from the top level by default, HBuilderX 3.0.5+ |
limitlevel | Number | The maximum level of the tree returned by the gettree query. Nodes beyond the set level will not be returned. Default level 10, maximum 15, minimum 1, HBuilderX 3.0.5+ |
groupby | String | Group data, HBuilderX 3.1.0+ |
group-field | String | Group statistics on data |
distinct | Boolean | Whether to deduplicate the duplicate records in the data query result, the default value is false, HBuilderX 3.1.0+ |
loadtime | String | 加载数据时机,默认auto,可选值 auto|onready|manual,详情 HBuilderX3.1.10+ |
ssr-key | String | 详情 HBuilderX 3.4.11+ |
@load | EventHandle | Callback on success. After the network returns the result, if you want to modify the data first and then render the interface, modify the data in this method |
@error | EventHandle | Failure callback |
TODO: The in subquery function is not supported yet. will be added later
Note: page-current/page-size
changes do not reset data (page-data="replace"
) and (loadtime="manual"
) except for collection/action/field/getcount/orderby/ where
clears the existing data after the change
Example
For example, cloud database has a user table with fields id and name. To query data with id=1, the writing method is as follows:
Note that the following example uses getone to return a piece of data in the form of an object. If getone is not used, the data will be in the form of an array, that is, one more layer
<template>
<view>
<unicloud-db v-slot:default="{data, loading, error, options}" collection="user" field="name" :getone="true" where="id=='1'">
<view>
{{ data.name}}
</view>
</unicloud-db>
</view>
</template>
Note: Unless you use the admin account to log in, you need to add Schema permission configuration to the table to be queried in the uniCloud console. At least configure read permission, otherwise you will not have permission to query on the front end, see DB Schema
<unicloud-db v-slot:default="{data, pagination, loading, hasMore, error, options}"></unicloud-db>
property | type | description |
---|---|---|
data | Array|Object | Query result, the default value is Array , when getone is specified as true , the value is the first data in the array, the type is Object , one layer is reduced |
pagination | Object | Pagination Properties |
loading | Boolean | Status in query. According to this state, the waiting content can be displayed in the template through v-if, such as <view v-if="loading">Loading...</view> |
hasMore | Boolean | Is there more data. According to this status, there is no more data displayed through v-if in the template, such as <uni-load-more v-if="!hasMore" status="noMore"></uni-load-more> , <uni-load-more> Detailshttps://ext.dcloud.net.cn/plugin?id=29 |
error | Object | Query error. According to this state, the waiting content can be displayed in the template through v-if, such as <view v-if="error">loading error</view> |
options | Object | In the applet, the slot cannot access the external data, it needs to be passed through this parameter, and the transfer function is not supported |
Hint: If no paging mode is specified, data
is a collection of multiple queries
Status example:
<unicloud-db v-slot:default="{data, loading, error, options}" collection="user">
<view v-if="error">{{error.message}}</view>
<view v-else-if="loading">正在加载...</view>
<view v-else>
{{data}}
</view>
</unicloud-db>
collection has the following forms
Single collection string
<unicloud-db v-slot:default="{data, loading, error, options}" collection="user">
<view v-if="error">{{error.message}}</view>
<view v-else-if="loading">正在加载...</view>
<view v-else>
{{data}}
</view>
</unicloud-db>
Multiple collection string concatenation
For join table query, note that the main table and the sub-table need to be associated with foreignKey in the schema (the sub-table supports multiple). The following example uses book as the main table, associates the author table for query, and sets the author_id field in the schema of the book table to point to the author table
<unicloud-db v-slot:default="{data, loading, error, options}" collection="book,author">
<view v-if="error">{{error.message}}</view>
<view v-else-if="loading">正在加载...</view>
<view v-else>
{{data}}
</view>
</unicloud-db>
An array of multiple temporary tables
It is also used for join table query, but different from the way of directly splicing multiple strings, the main table can be processed first and then associated. Compared with the direct use of multiple table name string splicing, the performance is significantly improved in the case of a large amount of data in the main table
<template>
<unicloud-db v-slot:default="{data, loading, error, options}" :collection="colList">
<view v-if="error">{{error.message}}</view>
<view v-else-if="loading">正在加载...</view>
<view v-else>
{{data}}
</view>
</unicloud-db>
</template>
<script>
const db = uniCloud.database()
export default {
data() {
return {
colList: [
db.collection('book').where('name == "水浒传"').getTemp(),
db.collection('author').getTemp()
]
}
},
onReady() {},
methods: {}
}
</script>
where specifies the conditions to be queried. For example, only query records whose value of a field meets certain conditions.
The where attribute of the component is consistent with the JS API of clientDB, and has more content, so see the relevant jql
document in the js API for details: Details
However, there is a difference between the component and the js API, that is, if you use the variables in js in the properties of the component, you need to pay extra attention.
For example, to query the uni-id-users table, the value of the field username is specified by the js variable. There are several ways as follows:
Method 1. Use template strings, wrap variables with ${}
<template>
<view>
<unicloud-db collection="uni-id-users" :where="`username=='${tempstr}'`"></unicloud-db>
</view>
</template>
<script>
export default {
data() {
return {
tempstr: '123'
}
}
}
</script>
Method 2. Do not write in properties, but concatenate strings in js
<template>
<view>
<unicloud-db ref="udb" collection="uni-id-users" :where="sWhere" loadtime="manual"></unicloud-db>
</view>
</template>
<script>
export default {
data() {
return {
tempstr: '123',
sWhere: ''
}
}
onLoad() {
this.sWhere = "id=='" + this.tempstr + "'"
// The component is configured with loadtime = "manual", where data needs to be loaded manually
this.$nextTick(() => {
this.$refs.udb.loadData()
})
}
}
</script>
Example of multi-condition query:
Method 1. Use template strings and wrap variables with ${}
<template>
<view>
<unicloud-db ref="udb" collection="uni-id-users" where="`id==${this.tempstr} && create_time > 1613960340000`"></unicloud-db>
</view>
</template>
<script>
export default {
data() {
return {
tempstr: '123'
}
}
}
</script>
Method 2. Use js to splice strings
<template>
<view>
<unicloud-db ref="udb" collection="uni-id-users" :where="sWhere" loadtime="manual"></unicloud-db>
</view>
</template>
<script>
export default {
data() {
return {
tempstr: '123',
sWhere: ''
}
}
onLoad() {
// id = this.tempstr and create_time > 1613960340000
this.sWhere = "id=='" + this.tempstr + "' && create_time > 1613960340000"
// this.sWhere = "id=='" + this.tempstr + "' || name != null"
// Loadtime = "manual" is configured on the component, here the data needs to be loaded manually
this.$nextTick(() => {
this.$refs.udb.loadData()
})
}
}
</script>
The above example uses the == comparator, and for fuzzy searches, regular expressions are used. The plug-in market provides a complete cloud-integrated search template, and search pages can be used directly without self-development. See details
Example of using regular fuzzy query:
<template>
<view class="content">
<input @input="onKeyInput" placeholder="请输入搜索值" />
<unicloud-db v-slot:default="{data, loading, error, options}" collection="goods" :where="where">
<view v-if="error">{{error.message}}</view>
<view v-else>
</view>
</unicloud-db>
</view>
</template>
<script>
export default {
data() {
return {
searchVal: ''
}
},
computed: {
where() {
return `${new RegExp(this.searchVal, 'i')}.test(name)` // 使用计算属性得到完整where
}
},
methods: {
onKeyInput(e) {
// In actual development, there should be anti-shake or throttling operations here, no demonstration here
this.searchVal = e.target.value
}
}
}
</script>
Again, the where condition has more content, and the usage of components and api is the same. The complete where condition document is in the api document, see also: JQL document
The format is field name
space asc
(ascending order)/desc
(descending order), multiple fields are separated by ,
, the priority is the field order
Single field sorting, sample code
<unicloud-db orderby="createTime desc"></unicloud-db>
Multi-field sorting, sample code
<unicloud-db orderby="createTime1 asc,createTime2 desc"></unicloud-db>
value | type | description |
---|---|---|
auto | String | Load data when the page is ready or after the attribute changes, the default is auto |
onready | String | The data will not be loaded automatically when the page is ready, and it will be loaded after the attribute changes. It is suitable for receiving the parameters of the previous page as the where condition in onready. |
manual | String | Manual mode, data is not loaded automatically. If paging is involved, you need to manually modify the current page first, and load data when calling |
The load event is triggered after query execution and before rendering, and is generally used for secondary processing of query data. For example, when the library query result cannot be directly rendered, the data can be preprocessed in the load event.
...
<unicloud-db @load="handleLoad" />
...
handleLoad(data, ended, pagination) {
// `data` current query result
// `ended` has more data
// `pagination` pagination information HBuilderX 3.1.5+ support
}
The time in the database is generally a timestamp and cannot be rendered directly. Although the time can be formatted in the load event, an easier way is to use the <uni-dateformat>
component, no need to write js processing.
The error event is triggered when a query reports an error, such as failure to connect to the network.
...
<unicloud-db @error="handleError" />
...
handleError(e) {
// {message}
}
Valid when H5 ssr is released, it is used to ensure that server-side rendering and front-end loading data correspond
Default: Unique value generated from (page path + line number in unicloud-db
component code)
Note: When two or more unicloud-db
components appear on the page at the same time, this property needs to be configured, and the value must be unique throughout the application
When the manual attribute of the <unicloud-db>
component is set to true, the data will not be queried online when the page is initialized. In this case, you need to use this method to manually load the data when needed.
this.$refs.udb.loadData() //udb为unicloud-db组件的ref属性值
Generally, onLoad cannot get this.$refs.udb because the timing is too early, but it can be obtained in onReady.
For example, in a common scenario, the page pagea obtains the parameter id in the url, and then loads the data
Request address: /pages/pagea?id=123
pagea.vue source:
<template>
<view>
<unicloud-db ref="udb" collection="table1" :where="where" v-slot:default="{data,pagination,loading,error,options}" :options="options" manual>
{{data}}
</unicloud-db>
</view>
</template>
<script>
export default {
data() {
return {
_id:'',
where: ''
}
},
onLoad(e) {
const id = e.id
if (id) {
this._id = id
this.where = "_id == '" + this._id + "'"
}
else {
uni.showModal({
content:"页面参数错误",
showCancel:false
})
}
},
onReady() {
if (this._id) {
this.$refs.udb.loadData()
}
}
}
</script>
Pull to refresh example
this.$refs.udb.loadData({clear: true}, callback)
,
Optional parameter clear: true
, whether to clear data and paging information, true
means clear, default false
callback
is a callback function that is fired after loading data (even if loading fails)
<script>
export default {
data() {
return {
}
},
// Page life cycle, triggered after pull-down refresh
onPullDownRefresh() {
this.$refs.udb.loadData({
clear: true
}, () => {
// stop pull down to refresh
uni.stopPullDownRefresh()
})
}
}
</script>
In the scenario of loading the next page of the list, use the ref method to access the component method to load more data. Each time the load is successful, the current page will be +1
this.$refs.udb.loadMore() //udb为unicloud-db组件的ref属性值
Clear loaded data without resetting current pagination information
this.$refs.udb.clear() //udb为unicloud-db组件的ref属性值
Reset the current pagination information without clearing the loaded data
this.$refs.udb.reset() //udb为unicloud-db组件的ref属性值
Clear and reload current page data
this.$refs.udb.refresh() //udb为unicloud-db组件的ref属性值
grammar
this.$refs.udb.remove(id, options)
udb is the ref attribute value of the unicloud-db component
Required parameter id
property | type | default value | description |
---|---|---|---|
id | string|Array | _id |
optional parameters options
property | type | default value | description |
---|---|---|---|
action | string | Before or after the cloud executes the database query, an action function is triggered to perform preprocessing or postprocessing, details. Scenario: Data that the front end does not have permission to operate, such as the number of readings + 1 | |
confirmTitle | string | Prompt | Delete Confirmation Box Title |
confirmContent | string | Whether to delete the data | Delete confirmation box prompt |
needConfirm | boolean | true | Controls whether there is a popup box, HBuilderX 3.1.5+ |
needLoading | boolean | true | Whether to display Loading, HBuilderX 3.1.5+ |
loadingTitle | string | '' | Display loading title, HBuilderX 3.1.5+ |
success | function | Delete the callback after success | |
fail | function | Remove callback after failure | |
complete | function | Callback after completion |
On the list page, if you want to delete an item, you have to do a lot of things:
In order to reduce repeated development, the unicloud-db component
provides a remove method, which binds the index when the list is rendered, and directly calls the remove method to complete the above 5 steps with one line of code.
First, bind the id to the delete button when the list is generated:
<unicloud-db ref="udb" :collection="collectionName" v-slot:default="{data,pagination,loading,error}">
<uni-table :loading="loading" :emptyText="error.message || '没有更多数据'" border stripe >
<uni-tr>
<uni-th>用户名</uni-th>
<uni-th>操作</uni-th>
</uni-tr>
<uni-tr v-for="(item,index) in data" :key="index">
<uni-th>{{item.username}}</uni-th>
<uni-td>
<view>
<button @click="confirmDelete(item._id)" type="warn">删除</button>
</view>
</uni-td>
</uni-tr>
</uni-table>
</unicloud-db>
Then there is only one line of code in the confirmDelete method:
confirmDelete(id) {
this.$refs.udb.remove(id)
}
The parameter of the remove method of the clientDB
component only supports deletion of the _id passed in the database, and does not support deletion of other where conditions.
The _id passed in by the parameter supports single or multiple, that is, it can be deleted in batches. The format of multiple ids is:
this.$refs.udb.remove(["5f921826cf447a000151b16d", "5f9dee1ff10d2400016f01a4"])
In the DB Schema
interface of the uniCloud web console, you can self-generate the admin management plug-in of the data table, in which there are examples of batch deletion of multiple rows of data.
The full instance, the second is an optional parameter.
var ids = ["5f921826cf447a000151b16d", "5f9dee1ff10d2400016f01a4"]
this.$refs.udb.remove(ids, {
action: '', // 删除前后的动作
confirmTitle: '提示', // 确认框标题
confirmContent: '是否删除该数据', // 确认框内容
success: (res) => { // 删除成功后的回调
const { code, message } = res
},
fail: (err) => { // 删除失败后的回调
const { message } = err
},
complete: () => { // 完成后的回调
}
})
grammar
this.$refs.udb.add(value, options)
udb is the ref attribute value of the unicloud-db component
Required parameter value
property | type | default value | description |
---|---|---|---|
value | Object | Add data |
optional parameters options
property | type | default value | description |
---|---|---|---|
action | string | Before or after the cloud executes the database query, an action function is triggered to perform preprocessing or postprocessing, details. HBuilder 3.1.0+ | |
showToast | boolean | true | Whether to show the prompt box after successful update |
toastTitle | string | Add success | Add a toast prompt after success |
needLoading | boolean | true | Whether to display Loading, HBuilderX 3.1.5+ |
loadingTitle | string | '' | Display loading title, HBuilderX 3.1.5+ |
success | function | Add a callback after success | |
fail | function | Add callback after failure | |
complete | function | Callback after completion |
<unicloud-db ref="udb" :collection="collectionName" v-slot:default="{data,pagination,loading,error}">
</unicloud-db>
this.$refs.udb.add(value)
full example
this.$refs.udb.add(value, {
toastTitle: '新增成功', // toast提示语
success: (res) => { // 新增成功后的回调
const { code, message } = res
},
fail: (err) => { // 新增失败后的回调
const { message } = err
},
complete: () => { // 完成后的回调
}
})
grammar
this.$refs.udb.update(id, value, options)
udb is the ref attribute value of the unicloud-db component
Required parameter id
property | type | default value | description |
---|---|---|---|
id | string | The unique identifier of the data |
Required parameter value
property | type | default value | description |
---|---|---|---|
value | Object | New data to be modified |
optional parameters options
属性 | 类型 | 默认值 | 描述 |
---|---|---|---|
action | string | 云端执行数据库查询的前或后,触发某个action函数操作,进行预处理或后处理,详情。HBuilder 3.1.0+ | |
showToast | boolean | true | 是否显示更新成功后的提示框 |
toastTitle | string | 修改成功 | 修改成功后的toast提示 |
needConfirm | boolean | true | 控制是否有更新确认弹出框,HBuilderX 3.1.5+ |
confirmTitle | string | true | 更新确认弹出框标题,HBuilderX 3.1.5+ |
confirmContent | string | true | 更新确认弹出框内容,HBuilderX 3.1.5+ |
needLoading | boolean | true | 是否显示Loading,HBuilderX 3.1.5+ |
loadingTitle | string | '' | 显示loading的标题,HBuilderX 3.1.5+ |
success | function | 更新成功后的回调 | |
fail | function | 更新失败后的回调 | |
complete | function | 完成后的回调 |
Using the update method of the unicloud-db component, in addition to updating the data in the cloud database, the data data in the unicloud-db component of the current page will also be updated at the same time, and the content of the page rendering will also be automatically updated automatically. At the same time, the update method also encapsulates the toast prompt for successful modification.
<unicloud-db ref="udb" :collection="collectionName" v-slot:default="{data,pagination,loading,error}" :getone="true">
</unicloud-db>
The first parameter id
is the unique identifier of the data, and the second parameter value
is the new data that needs to be modified
this.$refs.udb.update(id, value)
The full instance, the third is an optional parameter
this.$refs.udb.update(id, value, {
toastTitle: '修改成功', // toast提示语
success: (res) => { // 更新成功后的回调
const { code, message } = res
},
fail: (err) => { // 更新失败后的回调
const { message } = err
},
complete: () => { // 完成后的回调
}
})
Notice:
replace
, and each page has a fixed number, then after the remove method of the clientDB
component deletes the data, it will re-request the current page data.add
, and the data on the next page is scrolled, then after the remove method of the clientDB
component deletes the data, the data will not be re-requested, but moved from the existing data. except deleted items. (Component version 1.1.0+ support)In js, the method to get the data of the <unicloud-db>
component is as follows:
console.log(this.$refs.udb.dataList);
If the value of dataList is modified, the interface rendered by the component will also change synchronously.
However, this cannot be used to print and view data in the browser console. For this reason, the unidev.clientDB.data
method has been added to optimize the debugging experience.
H5 platform, in development mode, enter unidev.clientDB.data
in the browser console, you can view the internal data of the component, and multiple components can view unidev.clientDB.data[0]
through the index
There are two ways to write a join table query. For tables with a slightly larger amount of data, it is recommended to use an array of multiple temporary tables as the collection. You can filter in the getTemp of the main table first to reduce the performance consumption when joining tables.
For more information about joint table query, please refer to: JQL joint table query
Multiple collection string concatenation
For join table query, note that the main table and the sub-table need to be associated with foreignKey in the schema (the sub-table supports multiple). The following example uses book as the main table, associates the author table for query, and sets the author_id field in the schema of the book table to point to the author table
<unicloud-db v-slot:default="{data, loading, error, options}" collection="book,author">
<view v-if="error">{{error.message}}</view>
<view v-else-if="loading">正在加载...</view>
<view v-else>
{{data}}
</view>
</unicloud-db>
An array of multiple temporary tables
It is also used for join table query, but different from the way of directly splicing multiple strings, the main table can be processed first and then associated. Compared with the direct use of multiple table name string splicing, the performance is significantly improved in the case of a large amount of data in the main table
<template>
<unicloud-db v-slot:default="{data, loading, error, options}" :collection="colList">
<view v-if="error">{{error.message}}</view>
<view v-else-if="loading">正在加载...</view>
<view v-else>
{{data}}
</view>
</unicloud-db>
</template>
<script>
const db = uniCloud.database()
export default {
data() {
return {
colList: [
db.collection('book').where('name == "水浒传"').getTemp(),
db.collection('author').getTemp()
]
}
},
onReady() {},
methods: {}
}
</script>
The unicloud-db component simplifies the writing method of list paging. It only needs to simply configure how much data each page needs (the default is 20). Whether it is a database paging query or a front-end list paging display, it is automatically encapsulated.
There are 2 modes of list paging, one is common on mobile phones to pull to the bottom to load the next page, and the other is common on PC to list the page number at the bottom, click the page number to jump to the page.
The following sample code does not use the uList component. It is recommended to use the uList component during actual development to avoid performance problems with long lists.
<template>
<view class="content">
<unicloud-db ref="udb" v-slot:default="{data, pagination, loading, error, options}"
:options="options"
collection="table1"
orderby="createTime desc"
field="name,subType,createTime"
:getone="false"
:action="action"
:where="where"
@load="onqueryload" @error="onqueryerror">
<view v-if="error" class="error">{{error.message}}</view>
<view v-else class="list">
<view v-for="(item, index) in data" :key="index" class="list-item">
{{item.name}}
<!-- Use date formatting component, see plugin for details https://ext.dcloud.net.cn/search?q=date-format -->
<uni-dateformat :date="item.createTime" />
</view>
</view>
<view v-if="loading" class="loading">加载中...</view>
</unicloud-db>
</view>
</template>
<script>
export default {
data() {
return {
options: {}, // 插槽不能访问外面的数据,通过此参数传递, 不支持传递函数
action: '',
where: {} // 类型为对象或字符串
}
},
onPullDownRefresh() { //下拉刷新
this.$refs.udb.loadData({
clear: true //可选参数,是否清空数据
}, () => {
uni.stopPullDownRefresh()
})
},
onReachBottom() { //滚动到底翻页
this.$refs.udb.loadMore()
},
methods: {
onqueryload(data, ended) {
// Data can be preprocessed here before rendering the interface
},
onqueryerror(e) {
// failed to load data
}
}
}
</script>
<style>
.content {
display: flex;
flex-direction: column;
background-color: #f0f0f0;
}
.list-item {
background-color: #fff;
margin-bottom: 1px;
padding: 30px 15px;
}
.loading {
padding: 20px;
text-align: center;
}
.error {
color: #DD524D;
}
</style>
<template>
<view class="content">
<unicloud-db ref="udb" v-slot:default="{data, pagination, loading, error, options}"
:options="options"
collection="table1"
orderby="createTime desc"
field="name,subType,createTime"
:getcount="true"
@load="onqueryload" @error="onqueryerror">
<view>{{pagination}}</view>
<view v-if="error" class="error">{{error.errMsg}}</view>
<view v-else class="list">
<view v-for="(item, index) in data" :key="index" class="list-item">
{{item.name}}
<!-- Use date formatting component, see plugin for details https://ext.dcloud.net.cn/search?q=date-format -->
<uni-dateformat :date="item.createTime" />
</view>
</view>
<view class="loading" v-if="loading">加载中...</view>
<!-- Pagination component -->
<uni-pagination show-icon :page-size="pagination.size" :total="pagination.count" @change="onpagination" />
</unicloud-db>
</view>
</template>
<script>
export default {
data() {
return {
options: {}, // 插槽不能访问外面的数据,通过此参数传递, 不支持传递函数
}
},
onPullDownRefresh() { //下拉刷新(一般此场景下不使用下拉刷新)
this.$refs.udb.loadData({
clear: true
}, () => {
uni.stopPullDownRefresh()
})
},
methods: {
onqueryload(data, ended) {
// Data can be preprocessed here before rendering the interface
},
onqueryerror(e) {
// failed to load data
},
onpagination(e) {
this.$refs.udb.loadData({
current: e.current
})
}
}
}
</script>
<style>
.content {
display: flex;
flex-direction: column;
background-color: #f0f0f0;
}
.list-item {
background-color: #fff;
margin-bottom: 1px;
padding: 30px 15px;
}
.loading {
padding: 20px;
text-align: center;
}
.error {
color: #DD524D;
}
</style>
Use paging controls, which are common on the PC side. In uniCloud Admin, there are complete paginated display data and sample code for adding and deleting data.
The <unicloud-db>
component supports nesting.
When accessing parent component data in child component, options need to pass data
The following example demonstrates the nesting of 2 components and how to access the parent component data in the child component
<unicloud-db v-slot:default="{data, loading, error, options}" :options="options" collection="table1"
orderby="createTime desc" field="name,createTime">
<view v-if="error" class="error">{{error.errMsg}}</view>
<view v-else class="list">
<!-- Data returned by table1 -->
<view v-for="(item, index) in options" :key="index" class="list-item">
{{ item.name }}
</view>
</view>
<!-- nested -->
<!-- :options="data", the data returned by the parent component is passed to the component through options, and the child component is accessed through options -->
<unicloud-db ref="dataQuery1" v-slot:default="{loading, data, error, options}" :options="data" collection="table2"
orderby="createTime desc" field="name,createTime" @load="onqueryload" @error="onqueryerror">
<!-- The data returned by the parent component table1 -->
<view v-for="(item, index) in options" :key="index" class="list-item">
{{ item.name }}
</view>
<!-- Data returned by subcomponent table2 -->
<view v-for="(item, index) in data" :key="index" class="list-item">
{{ item.name }}
</view>
</unicloud-db>
</unicloud-db>
For a complete project example, see the example project in the plugin market: https://ext.dcloud.net.cn/plugin?id=2574
Debugging Tips
unidev.clientDB.data
in the browser console, you can view the internal data of the component, and multiple components can view unidev.clientDB.data[0]
through the index