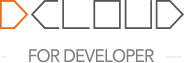
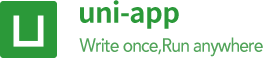
English
uniCloud, you can choose the serverless of Alibaba Cloud and Tencent Cloud. Similar to uni-app, which shields the end-to-end differences of various mini-programs, uniCloud also shields the differences between WeChat mini-program cloud development and Alipay mini-program cloud development.
That is to say, when uniCloud chooses Tencent Cloud, it is connected with WeChat applet cloud development, which is a set of cloud services, which are officially provided by Tencent Cloud.
Although the hardware is the same, including the API, there are still some software-level differences between uniCloud and WeChat cloud development.
uniCloud removes some functions developed by WeChat Cloud and adds more functions. And the difference in ecological support is also relatively large.
WeChat Cloud Development | uniCloud | |
---|---|---|
Cross-end | Only WeChat applet (although other tools can be used to develop H5 and QQ applet in disguise, but the end is not complete and it is difficult to maintain consistency) | full end. App, web, and various small program quick applications are fully supported |
Cross Cloud | Only Tencent Cloud is supported. Cloud functions written for it cannot run on other cloud platforms | Alibaba Cloud and Tencent Cloud are both supported for easy switching. Open to other clouds too |
Pricing | The price of using uniCloud Tencent Cloud Edition is the same as that of WeChat Cloud. DCloud just gets rebates from Tencent Cloud, not a price increase. If you choose uniCloud Aliyun version, it is cheaper than WeChat cloud development see details | |
Front-end operation database | Using WeChat account permissions instead of application account permissions, cannot program, and cannot effectively control data permission security. The client-side js sdk is bulky and affects performance | Perfect clientDB module, using the application's own user permission system, flexible programming, safe and reliable control permissions |
Database schema | Not supported | Perfect database schema design, with its own permission verification and data legitimacy verification |
Database query syntax | MongoDB syntax. The learning threshold is high and the writing method is complicated, especially the join table query is difficult to use | In addition to the MongoDB syntax, JQL syntax is supported, which greatly reduces the learning threshold, reduces the amount of code for database operations, and quickly completes complex queries |
Development tools | WeChat applet tool, poor coding experience | General programming tool HBuilderX, efficient operation and perfect, open plug-in system |
Frontend database watch | Supported. Permissions are implemented according to the WeChat account system | There is a more powerful free websocket service View details |
opendb | 无 | 开放的数据库规范,众多价值,详情 |
账户服务 | 仅微信登录,但鉴权更简单 | uni-id支持应用自己的账户体系,手机号或email,内置短信验证码和app端一键登录,支持微信、支付宝等三方登录,支持权限、角色、社交裂变等众多功能 |
admin system | Does not come with | With open source uniCloud admin system, self-adaptive to large and small screens, comes with user, role, permission functions, and more Multiple plugins ready to use |
Payment | Only WeChat Pay, but the authentication is simpler | uniPay, cross-end unified payment |
cms | Support. But the front-end part lacks cross-end | support. Front-end cross-end, management end open source |
Plugin Ecology | Tencent Cloud has developed some plugins | Rich plugin ecology, including plugins developed by Tencent Cloud for uniCloud, many ready-made project modules, [Details](https://ext.dcloud.net.cn/?cat1=7&orderBy =UpdatedDate) |
The above differences, in conclusion, uniCloud is more open, ecologically richer, and more efficient in development.
The reasons for the high development efficiency include important functions and tools such as clientDB, JQL, and HBuilderX that have a great impact on efficiency, as well as a large number of ready-made wheels. For details, see: 2021, let's increase the development efficiency by 10 times!
If you have already developed WeChat Mini Programs and want to migrate to uniCloud, the following provides technical migration guidelines for cloud functions and databases.
uniCloud可以使用uni-id更简单的接入微信小程序登录。参考uni-id
Different from directly obtaining openid in WeChat cloud development, the login interface provided by uni-id will add user records in the uni-id-users table of the database.
If you want to control the permissions of cloud storage, you can use custom login, uniCloud Tencent Cloud Custom Login
uniCloud provides uniPay to realize payment function, and supports WeChat and Alipay unipay document
The code of the old WeChat cloud development + native applet is as follows:
// The code of the cloud function part
const cloud = require('wx-server-sdk')
cloud.init({
env: cloud.DYNAMIC_CURRENT_ENV
})
exports.main = async (event, context) => {
const res = await cloud.cloudPay.unifiedOrder({
"body" : "商品描述",
"outTradeNo" : "商户订单号",
"spbillCreateIp" : "127.0.0.1",
"totalFee" : 1,
// Service space ID and cloud function name where the cloud function used to receive payment asynchronous notifications is located
"envId": "test-f0b102",
"functionName": "pay_cb"
})
return res
}
// code of the applet part
wx.cloud.callFunction({
name: '函数名',
data: {
// ...
},
success: res => {
const payment = res.result.payment
wx.requestPayment({
...payment,
success (res) {
console.log('pay success', res)
},
fail (res) {
console.error('pay fail', err)
}
})
},
fail: console.error,
})
Migrating to the uniCloud + uni-app system, the code is changed to this:
// The code of the cloud function part
const unipayIns = unipay.initWeixin({
appId: 'your appId',
mchId: 'your mchId',
key: 'you parterner key',
// pfx: fs.readFileSync('/path/to/your/pfxfile'), // p12 file path, it is required when using WeChat refund, it should be noted that absolute path must be used
})
exports.main = async (event, context) => {
const res = await unipayIns.getOrderInfo({
openid: 'user openid',
body: '商品描述',
outTradeNo: '商户订单号',
totalFee: 1, // 金额,单位分
notifyUrl: 'https://xxx.xx' // 支付结果通知地址
})
return res
}
// code for the client part
uniCloud.callFunction({
name: '云函数名',
data: {
// ...
},
success(res) {
uni.requestPayment({
provider: 'wxpay',
...res.result.orderInfo
success (res) {
console.log('pay success', res)
},
fail (res) {
console.error('pay fail', err)
}
})
}
})
Notice
Temporary CDN is mainly used to solve scenarios that need to transfer large files to cloud functions. When using uniCloud, it can be achieved by uploading to cloud storage first and then passing the fileID to cloud functions.
WeChat cloud development writing method:
wx.cloud.callFunction({
name: 'test',
data: {
filePathDemo: wx.cloud.CDN({
type: 'filePath',
filePath: 'xxxxxxxx',
})
},
})
uniCloud writing:
uniCloud.uploadFile({
filePath: filePath,
cloudPath: 'a.jpg'
}).then(res => {
const fileID = res.fileID
uniCloud.callFunction({
name: 'test',
data: {
filePathDemo: fileID
}
})
})
Scenarios using the WeChat open interface can be replaced with mp-cloud-openapi. The usage is basically the same as WeChat cloud development
WeChat cloud development writing method:
const cloud = require('wx-server-sdk')
cloud.init()
exports.main = async (event, context) => {
try {
const result = await cloud.openapi.wxacode.createQRCode({
path: 'page/index/index',
width: 430
})
return result
} catch (err) {
return err
}
}
uniCloud writing:
// mp-cloud-openapi, address: https://ext.dcloud.net.cn/plugin?id=1810
const openapi = require('mp-cloud-openapi')
const openapiWeixin = openapi.initWeixin({
appId: 'appId',
secret: 'secret'
})
exports.main = async (event, context) => {
try {
const result = await openapiWeixin.wxacode.createQRCode({
path: 'page/index/index',
width: 430
})
return result
} catch (err) {
return err
}
}
The console developed by WeChat Mini Program Cloud can export json data. This format is the same as that of uniCloud. The export file can be imported directly from the web console of uniCloud.
Wechat applet cloud development add, update, set operation parameters have one more layer of data than uniCloud The most important things to pay attention to when migrating from WeChat applet cloud development
WeChat cloud development writing method:
const res = await db.collection('todos').doc('todo-id').add({
data: {
description: "learn cloud database",
done: false
}
})
uniCloud writing:
const res = await db.collection('todos').doc('todo-id').add({
description: "learn cloud database",
done: false
})
UniCloud also supports client-side operation of the database (hereinafter referred to as clientDB), but it is slightly different from WeChat cloud development. There are two main points
In addition clientDB supports the following extension capabilities