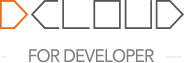
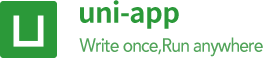
English
HBuilderX 3.5.0+ support
Cloud function common module
is a way for different cloud functions to share code. If you don't know what is cloud function common module
, please read the document public module
uni-id-common
is a common module for token management in the uni-id
system.
Old version uni-id public module is a large and comprehensive public module, which is not suitable for being referenced by many cloud functions.
The new version of uni-id-common
only includes token verification, generation and refresh functions. The implementation of user registration, login, forgotten password, etc. are all moved to the uni-id-co
cloud object (the cloud object is built in uni-id-pages).
This not only reduces the size of the common module, but also simplifies the learning cost.
Starting from HBuilderX 3.5, the uni-id-common
dependency is automatically loaded when creating a new uniCloud project. That is, uni-id-common
is built into every project by default.
Generally, developers do not need to understand the API of the uni-id-common
public module, and can directly use the uni-id-pages cloud integrated page template.
If you want to understand the internal implementation of the uni-id-common
common module, you can read this chapter.
Note: Unlike the old version of uni-id common module, uni-id-common must call this interface to create an instance before calling interfaces such as checkToken
用法:uniID.createInstance(Object CreateInstanceOptions);
CreateInstanceOptions内可以传入云函数context,也可以传入clientInfo参数,作用和context类似。方便在云对象内获取clientInfo后直接传入,什么是云对象?。
// Cloud function code, pass in context
const uniID = require('uni-id-common')
exports.main = async function(event,context) {
context.APPID = '__UNI__xxxxxxx' // 替换为当前客户端的APPID,通过客户端callFunction请求的场景可以使用context.APPID获取
context.PLATFORM = 'web' // 替换为当前客户端的平台类型,通过客户端callFunction请求的场景可以使用context.PLATFORM获取
context.LOCALE = 'zh-Hans' // 替换为当前客户端的语言代码,通过客户端callFunction请求的场景可以使用context.LOCALE获取
const uniIDIns = uniID.createInstance({ // 创建uni-id实例
context: context,
// config: {} // Complete uni-id configuration information, no need to pass this parameter when using config.json for configuration
})
payload = await uniIDIns.checkToken(event.uniIdToken) // 后续使用uniIDIns调用相关接口
if (payload.code) {
return payload
}
}
// Cloud object code passes in clientInfo
const uniID = require('uni-id-common')
module.exports = {
_before() {
const clientInfo = this.getClientInfo()
this.uniID = uniID.createInstance({ // 创建uni-id实例,其上方法同uniID
clientInfo
})
},
refreshToken() {
// ...
// this.uniID.refreshToken()
}
}
Why do you need to create a uni-id instance yourself
By default, some interfaces of uni-id-common
will automatically obtain the client's PLATFORM (platform, such as app, h5, mp-weixin) and other information from the global context.
In the scenario of cloud functions single instance, multiple concurrency, it may not be obtained correctly (the global object will be overwritten by subsequent requests, which may cause the previous request to use the PLATFORM of the subsequent request) information). Therefore, it is recommended to pass in the context for the uni-id after enabling the single-instance multi-concurrency of cloud functions.
In addition, when the cloud function is urlized, the client information cannot be obtained, and it is also necessary to pass the client information into the uni-id in this way.
An API that verifies the uniIdToken that comes with the request initiated by the client (uniCloud.callFunction), and obtains the user's uid, token, token expiration time, role, and permissions.
This is a very high frequency and important API that is usually used in exchange for the user ID of the current cloud function.
think
If you have no experience in server-side development, you may think: Why do you need to exchange the user ID with a token, instead of letting the client pass the user ID directly? This involves security issues. There is a saying: "The parameters passed by the front end cannot be trusted." For example, if you go to the bank to withdraw money, the counter will ask to show your ID card to prove who you are, instead of telling the bank counter directly who you are. Otherwise this is a huge security hole. To sum up: All server-side operations involving account information related content need to be obtained by using tokens instead of parameters passed by the front-end.
Usage: uniIDIns.checkToken(String token, Object checkTokenOptions)
Parameter Description
Fields | Type | Required | Description |
---|---|---|---|
token | String | Yes | The token brought by the client callFunction |
options | object | no | options for checkToken method |
|- autoRefresh | boolean | No | Whether it is necessary to automatically determine the refresh token, the default is true |
illustrate
Response parameters
Field | Type | Description |
---|---|---|
errCode | Number|String | Error code, 0 means success |
message | String | Details |
uid | String | User ID, it will be returned after successful verification |
token | String | When the user token is about to expire, the newly generated token will only have this behavior when the value of tokenExpiresThreshold is configured in config |
role | Array | - |
permission | Array | A list of user permissions. |
uni-id uses jwt to generate a token. The token generated by jwt consists of three parts, and the stored information is plaintext information. uni-id only verifies whether the client token is legal based on the tokenSecret.
Role permissions will be cached in the token, which can reduce or eliminate the number of checkToken checks (effectively saving costs and reducing response time)
Notice:
uni_id_token
when the token is stored in the storage, which will be compatible with the camel-case uniIdToken
for a period of timeAdded in uni-id 3.3.14
用法:uniIDIns.refreshToken(Object RefreshTokenOptions);
Parameter Description
Fields | Type | Required | Description |
---|---|---|---|
token | String | Yes | user token |
Example
const {
token,
tokenExpired
} = await uniIDIns.refreshToken({
token: 'xxx'
})
Notice
用法:uniIDIns.createToken(Object CreateTokenOptions)
Parameter Description
Fields | Type | Required | Description |
---|---|---|---|
uid | String | Yes | UserId |
role | Array | no | Specifies the role cached in the token |
permission | Array | No | Specifies the permissions cached in the role |
Response parameters
Fields | Type | Required | Description |
---|---|---|---|
token | String | Yes | Generated token |
tokenExpired | Number | Yes | Timestamp corresponding to token expiration time |
illustrate