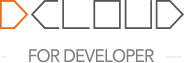
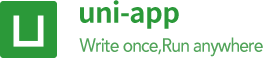
English
uniCloud is divided into two parts: the client and the cloud. Some interfaces have the same name and similar parameters. Here are the interfaces/properties that can be used in the client sdk to avoid confusion.
List of Client APIs
API | Description |
---|---|
uniCloud.importObject() | Get the cloud object reference to call the cloud object interface Details |
uniCloud.callFunction() | The client calls cloud functions Details |
uniCloud.database() | The client accesses the cloud database and obtains the object reference of the cloud database Details |
uniCloud.uploadFile() | Client uploads files directly to cloud storage Details |
uniCloud.getTempFileURL() | The client gets the temporary path of the cloud storage file Details |
uniCloud.chooseAndUploadFile() | The client selects a file and uploads it Details |
uniCloud.getCurrentUserInfo() | Get current user information Details |
uniCloud.init() | Initialize additional service space when using multiple service spaces at the same time Details |
uniCloud.addInterceptor() | Add Interceptor Details |
uniCloud.removeInterceptor() | Remove the interceptor Details |
uniCloud.interceptObject() | Intercept cloud object requests Details |
uniCloud.onResponse() | Listen to the server (cloud function, cloud object, clientDB) response Details |
uniCloud.offResponse() | Remove the response from the monitoring server (cloud function, cloud object, clientDB) Details |
uniCloud.onNeedLogin() | Listen for login required events Details |
uniCloud.offNeedLogin() | Remove the need to log in event Details |
uniCloud.onRefreshToken() | Listen to the token update event Details |
uniCloud.offRefreshToken() | Remove monitoring token update event Details |
uniCloud.initSecureNetworkByWeixin() | Shake hands with the cloud function before sending the secure network request of the WeChat MiniAppdetails |
uniCloud.getFileInfo() | When Alibaba Cloud migrates the service space to the commercial version, use the beta cloud storage link to obtain the commercial version cloud storage link Details |
HBuilderX 3.1.0+
Parse the client token to obtain user information. It is often used to judge the current logged-in user status and user permissions on the front end, such as displaying and hiding certain buttons according to different permissions.
Notice
uni_id_token
in storageUsage: uniCloud.getCurrentUserInfo()
This method is a synchronous method.
Response parameters
Field | Type | Description |
---|---|---|
uid | Number | current user uid |
role | Array | List of user roles. admin user returns ["admin"] |
permission | Array | A list of user permissions. Note that the admin role this array is empty |
tokenExpired | Number | token expiration time |
The following results are returned when the user information cannot be obtained
{
uid: null,
role: [],
permission: [],
tokenExpired: 0
}
Example
console.log(uniCloud.getCurrentUserInfo().role.indexOf('admin')>-1); // 如果是admin用户的话,打印结果为true
console.log(uniCloud.getCurrentUserInfo().role.indexOf('admin')>-1); // If it is an admin user, the print result is true
Added in HBuilderX 3.1.20
Interface form: uniCloud.addInterceptor(String apiName, Object interceptor)
Platform Compatibility
Alibaba Cloud | Tencent Cloud |
---|---|
√ | √ |
Introduction to parameters
Fields | Type | Required | Description |
---|---|---|---|
apiName | string | Yes | Api name to be intercepted, optional values: callFunction, database, uploadFile |
interceptor | object | Yes | the interceptor to add |
Interceptor parameter description
Parameter Name | Type | Required | Default Value | Description | Platform Difference Description |
---|---|---|---|---|---|
invoke | Function | No | Invoke before interception | ||
success | Function | No | Success callback interception | ||
fail | Function | No | Fail callback interception | ||
complete | Function | No | Complete callback interception |
Example
uniCloud.addInterceptor('callFunction', {
invoke(param) {
// param is the parameter for intercepting Api Example {name: 'functionName', data: {'functionParam1': 1, 'functionParam2': 2}}
// Return an error here to terminate the api execution
},
success(res) {
// res is the return value of callFunction, the return value can be modified here
},
fail(err) {
// err is the error thrown by callFunction
},
complete(res){
// The res in complete is the res or err above
}
})
Added in HBuilderX 3.1.20
Interface form: uniCloud.removeInterceptor(String apiName, Object interceptor)
Introduction to parameters
Fields | Type | Required | Description |
---|---|---|---|
apiName | string | Yes | Api name to be intercepted, optional values: callFunction, database, uploadFile |
interceptor | object | Yes | The interceptor to be removed, optional, if this parameter is not passed, remove all interceptors of this API |
Interceptor parameter description
Parameter Name | Type | Required | Default Value | Description | Platform Difference Description |
---|---|---|---|---|---|
invoke | Function | No | Invoke before interception | ||
success | Function | No | Success callback interception | ||
fail | Function | No | Fail callback interception | ||
complete | Function | No | Complete callback interception |
Notice:
// Wrong usage, cannot remove invoke interceptor
uniCloud.addInterceptor('callFunction', {
invoke(param) {
console.log('callFunction invoked, with param:',param)
}
})
uniCloud.removeInterceptor('callFunction', {
invoke(param) {
console.log('callFunction invoked, with param:',param)
}
})
// correct usage
function invokeInterceptor(param) {
console.log('callFunction invoked, with param:',param)
}
uniCloud.addInterceptor('callFunction', {
invoke: invokeInterceptor
})
uniCloud.removeInterceptor('callFunction', {
invoke: invokeInterceptor
})
Added in HBuilderX 3.5.5
Interface form: uniCloud.interceptObject(Object interceptor)
Interceptor parameter description
Parameter Name | Type | Required | Default Value | Description | Platform Difference Description |
---|---|---|---|---|---|
invoke | Function | No | Invoke before interception | ||
success | Function | No | Success callback interception | ||
fail | Function | No | Fail callback interception | ||
complete | Function | No | Complete callback interception |
The invoke interceptor will receive parameters of the following form
{
objectName: "", // 云对象名称
methodName: "", // 云对象的方法名称
params: [] // 参数列表
}
The success interceptor will receive parameters of the following form
{
objectName: "", // 云对象名称
methodName: "", // 云对象的方法名称
params: [], // 参数列表
result: {} // 云对象响应结果
}
The fail interceptor will receive parameters of the following form
{
objectName: "", // 云对象名称
methodName: "", // 云对象的方法名称
params: [], // 参数列表
error: new Error() // 错误对象
}
The complete interceptor will receive the same parameters as the success or fail interceptor, depending on whether the cloud function is successfully executed or not.
Added in HBuilderX 3.4.13
Used to monitor cloud functions, cloud objects, and clientDB's request responses
Code example:
uniCloud.onResponse(function(event) {
// event format see below
})
Response format
interface OnResponseEvent {
type: 'cloudobject' | 'cloudfunction' | 'clientdb',
content: {} // content同云对象方法、云函数、clientDB请求的返回结果或错误对象
content: {} // content is the same as cloud object method, cloud function, return result of clientDB request or error object
}
Take calling cloud object method as an example
uniCloud.onResponse(function(e){
console.log(e)
})
const todo = uniCloud.importObject('todo')
const res = await to.add('todo title', 'todo content')
The e format printed in the above code is as follows
// successful response
e = {
type: 'cloudobject',
content: { // content内容和上方代码块中的res一致
content: { // content content is the same as res in the above code block
errCode: 0
}
}
// fail response
e = {
type: 'cloudobject',
content: {
errCode: 'invalid-todo-title',
errMsg: 'xxx'
}
}
You can judge whether it is a failure or a successful response by judging whether there is a true errCode in the content
uniCloud.onResponse(function(e){
if(e.content.errCode) {
console.log('请求出错')
console.log('Request error')
}
})
Added in HBuilderX 3.4.13
Used to remove the listener added by onResponse
Notice
// Wrong usage, can't remove listener
uniCloud.onResponse(function(e) {
console.log(e)
})
uniCloud.offResponse(function(e) {
console.log(e)
})
// correct usage
function logResponse(e) {
console.log(e)
}
uniCloud.onResponse(logResponse)
uniCloud.offResponse(logResponse)
Added in HBuilderX 3.5.0
用于监听客户端需要登录事件,此接口需要搭配uniIdRouter使用,参考:uniIdRouter
Code example:
uniCloud.onNeedLogin(function(event) {
// event format see below
})
Response format
interface OnNeedLoginEvent {
errCode: number | string,
errMsg: string,
uniIdRedirectUrl: string // 触发onNeedLogin页面前的页面地址(包含路径和参数的完整页面地址)
uniIdRedirectUrl: string // The page address before the onNeedLogin page is triggered (the full page address including the path and parameters)
}
Notice
onNeedLogin
Added in HBuilderX 3.5.0
Notice
// Wrong usage, can't remove listener
uniCloud.onNeedLogin(function(e) {
console.log(e)
})
uniCloud.offNeedLogin(function(e) {
console.log(e)
})
// correct usage
function log(e) {
console.log(e)
}
uniCloud.onNeedLogin(log)
uniCloud.offNeedLogin(log)
Added in HBuilderX 3.5.0
Used to monitor client token refresh events, including automatic token update when cloud object returns newToken and clientDB automatic token update, note that the token returned by uni-id-co login will also trigger this event
Code example:
uniCloud.onRefreshToken(function(event) {
// event format see below
})
Response format
interface OnRefreshTokenEvent {
token: string,
tokenExpired: number
}
Notice
onNeedLogin
Added in HBuilderX 3.5.0
Notice
// 错误用法,无法移除监听
uniCloud.onRefreshToken(function(e) {
console.log(e)
})
uniCloud.offRefreshToken(function(e) {
console.log(e)
})
// correct usage
function log(e) {
console.log(e)
}
uniCloud.onRefreshToken(log)
uniCloud.offRefreshToken(log)
Added in 3.6.8
For secure network related documents, please refer to: secure network
parameter
参数 | 类型 | 必填 | 默认值 | 说明 |
---|---|---|---|---|
callLoginByWeixin | boolean | 否 | false | 是否在安全网络初始化同时执行一次uni-id-co的微信登录,配置为false时不进行微信登录仅调用uni-id-co的secureNetworkHandshakeByWeixin方法进行握手 |
openid | string | 否 | - | 新增于HBuilderX 3.7.7,传入此参数后此方法内部不再调用uni-id-co的任何方法,此时需要由开发者自行实现一些凭据的存储逻辑,详情参考:不使用uni-id-pages时如何使用微信小程序安全网络 |
example
// App.vue
<script>
export default {
onLaunch: async function() {
// #ifdef MP-WEIXIN
const res = await uniCloud.initSecureNetworkByWeixin({
callLoginByWeixin: true
})
console.log('initSecureNetworkByWeixin', res);
// #endif
console.log('App Launch')
}
}
</script>
<style>
</style>
Notice
Usage: uniCloud.config.provider
Accessing this property will return tencent
and aliyun
representing Tencent Cloud and Aliyun respectively
When the client requests the cloud (including requesting cloud functions, cloud objects, clientDB, cloud storage, etc.), there may be scenarios where errors may be thrown. In this case, an uniCloud standard error object (referred to as uniCloudError hereinafter) will be thrown, and uniCloudError contains the following attributes
property | type | required | description |
---|---|---|---|
errCode | string | yes | error code |
errMsg | string | yes | error message |
requestId | string | No | RequestId, for troubleshooting |
detail | object | No | This property only exists when the cloud object actively returns the response body specification corresponding to the error |
In addition, there are code properties and message properties on the uniCloudError object, neither of which is recommended.