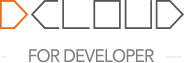
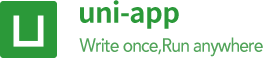
English
Added in HBuilderX version 3.3.1
For database operations that are not suitable for exposure on the front end, traditional MongoDB syntax can only be used in cloud functions in the past. This update makes it possible for cloud functions to also use JQL to operate on the database.
Compared with the traditional MongoDB syntax, JQL brings the following values to cloud functions:
This article mainly explains how to use JQL in cloud functions. For detailed JQL syntax, see the document: JQL database operations
The engine code of JQL operation is not small. Considering that some cloud functions do not operate the database or use JQL, the related functions are stripped in the uni-cloud-jql
extension library.
Starting from HBuilderX 3.4, when creating a new cloud function/cloud object, the uni-cloud-jql
extension library is loaded by default. For old cloud functions, you can also right-click the cloud function -> manage public modules or extended library dependencies -> select uni-cloud-jql
Developers before HBuilderX 3.4 need to manually add the extension library in the package.json of the cloud function. (If there is no package.json in the cloud function directory, it can be generated by executing npm init -y
in the cloud function directory)
The following is an example of package.json for a cloud function with the jql extension library enabled. Note that there should be no comments. The comments in the following file content are for illustration only. If you copy this file, remember to remove the comments
{
"name": "jql-test",
"version": "1.0.0",
"description": "",
"main": "index.js",
"extensions": {
"uni-cloud-jql": {} // 配置为此云函数开启jql扩展库,值为空对象留作后续追加参数,暂无内容
},
"author": ""
}
For those who do not need to use the JQL extension library in cloud functions/cloud objects, right-click the cloud function and cancel the uni-cloud-jql
extension library. This reduces cloud function volume and improves performance.
uniCloud.databaseForJQL
method, pass in the client information, you can get a JQL database operation object.
// simple usage example
'use strict';
exports.main = async (event, context) => {
const dbJQL = uniCloud.databaseForJQL({ // 获取JQL database引用,此处需要传入云函数的event和context,必传
event,
context
})
const bookQueryRes = await dbJQL.collection('book').where("name=='三国演义'").get() // 直接执行数据库操作
return {
bookQueryRes
}
};
Added in HBuilderX 3.4.10
The event
and context
cannot be obtained in the cloud object. In order to facilitate the use of jql extensions in the cloud object, since HBuilderX 3.4.10, the uniCloud.databaseForJQL
method receives the cloud object clientInfo
as a parameter
Example
module.exports = {
addTodo (title, content) {
const dbJQL = uniCloud.databaseForJQL({ // 获取JQL database引用,此处需要传入云对象的clientInfo
clientInfo: this.getClientInfo()
})
}
}
In the above example, the jql extension uses the client's identity, to be precise, the user corresponding to the client's uniIdToken as the user performing database operations.
To specify another user identity to execute in the cloud function/cloud object, use the setUser
method
The parameter of the setUser method is an object, which can be passed in the uid, role, and permission of the uni-id, and the combination will take effect.
example:
'use strict';
exports.main = async (event, context) => {
const dbJQL = uniCloud.databaseForJQL({ // 获取JQL database引用,此处需要传入云函数的event和context
event,
context
})
dbJQL.setUser({ // 指定后续执行操作的用户信息,此虚拟用户将同时拥有传入的uid、role、permission
uid: 'user-id', // 用户id
role: ['admin'], // 指定当前执行用户的角色为admin。如果只希望指定为admin身份,可以删除uid和permission字段
permission: [] // 用户权限
})
const bookQueryRes = await dbJQL.collection('book').where("name=='三国演义'").get() // 直接执行数据库操作
return {
bookQueryRes
}
};
Although both use JQL, there is still a little difference between the cloud and the client
uni-id-common
公共模块、redis扩展库schema
, action
, validateFunction
into the module, if you have a lot of the above files, it will greatly increase the size of the cloud function, so the cloud function with this extension is enabled cold start It will take a little longer, it is recommended not to enable this extension for too many cloud functions