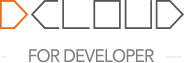
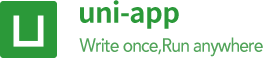
English
The difference in date processing between Tencent Cloud and Alibaba Cloud is mainly reflected in the storage of date strings in ISOString format (for example: 2022-07-01T02:50:11.142Z
). Tencent Cloud will not perform special processing when entering the database, and will store this string in the form of a string, and Alibaba Cloud will convert this format string to date object storage. The following code is easier to understand this problem
// cloud function code
'use strict';
const db = uniCloud.database()
function getType(val) {
return Object.prototype.toString.call(val).slice(8, -1).toLowerCase()
}
exports.main = async (event, context) => {
const addRes = await db.collection('date-test').add({
dateStr: '2022-07-01T02:50:11.142Z',
dateObj: new Date()
})
const getRes = await db.collection('date-test').doc(addRes.id).get()
const {
dateStr,
dateObj
} = getRes.data[0]
console.log(getType(dateStr)) // 腾讯云:string,阿里云:date
console.log(getType(dateObj)) // 腾讯云:date,阿里云:date
return {}
};
Both are date objects, and the formats displayed in the web console Tencent Cloud and Alibaba Cloud are not consistent. Take the dateObj field in the above code as an example
Tencent Cloud will be displayed in the following format:
{
"dateObj": {
"$date":1656424298294
}
}
Alibaba Cloud will display it in the following format:
{
"dateObj": "2022-07-01T03:17:39.226Z" // 阿里云在web控制台展示为字符串,但实际底层存储的是日期对象
}
When clientDB stores date strings and date objects, it behaves the same as the cloud vendor used. But when I take out the date object I get a string in ISOString format
// client code
const db = uniCloud.database()
async function test() {
const getRes = await db.collection('date-test').get()
const dateObj = getRes.result.data[0].dateObj // "2022-07-01T03:17:39.226Z"
}
test()
Alibaba Cloud and Tencent Cloud nodejs8 will freeze the rest of the logic after the cloud function returns and will no longer be executed. The performance of Tencent Cloud nodejs12 is just the opposite. After the cloud function returns, it will wait for the rest of the logic to be executed before the cloud function instance is idle. For details, see: keepRunningAfterReturn