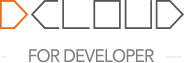
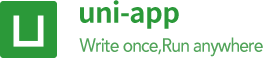
English
Cloud functions support public modules. The shared parts of multiple cloud functions can be extracted into common modules and then referenced by multiple cloud functions.
Version requirements: HBuilderX 2.6.6+
Take the following directory structure as an example to introduce how to use it.
cloudfunctions
├─common // 云函数公用模块目录
| └─hello-common // 云函数公用模块
| ├─package.json
| └─index.js // 公用模块代码,可以不使用index.js,修改 package.json 内的 main 字段可以指定此文件名
└─use-common // 使用公用模块的云函数
├─package.json // 在 use-common 目录执行 npm init -y 生成
└─index.js // 云函数入口文件
Create and import common modules
common
directory under the cloudfunctions
directorycommon
directory to create a common module directory (in this case, hello-common
, see the example below), the entry index.js
file and package.json
will be created automatically, Do not modify The name field of this package.jsonhello-common
右键上传公用模块管理公共模块依赖
,添加依赖的公共模块Public modules depend on other public modules in the same way
Precautions
hello-common
) under the common
directory and select Update cloud functions that depend on this module
npm install
to become invalid. If this happens, delete node_modules
and package-lock.json
and re npm install
Using common modules
Still taking the above directory as an example, exports
in the public module and require
in the cloud function can be used. The sample code is as follows:
// common/hello-common/index.js
function getVersion() {
return '0.0.1'
}
module.exports = {
getVersion,
secret: 'your secret'
}
// use-common/index.js
'use strict';
const {
secret,
getVersion
} = require('hello-common')
exports.main = async (event, context) => {
let version = getVersion()
return {
secret,
version
}
}
If you only need to export a function, you can also use the following writing
// common/hello-common/index.js
module.exports = function(e){
return e
}
// use-common/index.js
'use strict';
const echo = require('hello-common')
exports.main = async (event, context) => {
let eventEcho = echo(event)
return {
eventEcho
}
}