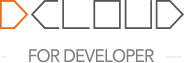
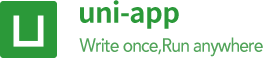
English
Ordinary cloud function
CallFunction method cloud function, also known as ordinary cloud function.
The front-end code of uni-app no longer executes uni.request
for networking, but calls cloud functions through uniCloud.callFunction
.
The callFunction method avoids the server providing the domain name, does not expose the fixed IP, and reduces the risk of being attacked.
uniCloud.callFunction
can be executed in the uni-app frontend or in the uniCloud cloud function. That is, both the front end and the cloud can call another cloud function.
The parameters and return values of the callFunction
method are as follows:
uniCloud.callFunction
expects a json object as parameter with 2 fields
Fields | Type | Required | Description |
---|---|---|---|
name | String | yes | cloud function name |
data | Object | No | Parameters that the client needs to pass |
return json
Field | Type | Description |
---|---|---|
result | Object | The return result of the code return in the cloud function |
requestId | String | The serial number of the cloud function request, which is used for troubleshooting, which can be found in the cloud function log in the uniCloud web console |
header | Object | Server header information |
errCode | Number or String | Server Error Code |
success | bool | Whether the execution is successful |
Notice:
header
when running cloud functions locally, it needs to run cloud functions on the cloud to returnThe return format is explained in detail below see details
Front-end sample code
If the cloud service space has a cloud function named "hellocf", the front end can call this cloud function as follows
// promise method
uniCloud.callFunction({
name: 'hellocf',
data: { a: 1 }
})
.then(res => {});
//callback method
uniCloud.callFunction({
name: 'hellocf',
data: { a: 1 },
success(){},
fail(){},
complete(){}
});
When the client callFunction calls the cloud function, the cloud function receives the client data through the input parameters, obtains the client information through the header information context, and returns the result to the client after business logic processing.
Suppose the client code calls the cloud function hellocf
and passes the data of {a:1,b:2}
,
// The client calls the cloud function and passes the parameters
uniCloud.callFunction({
name: 'hellocf',
data: {a:1,b:2}
})
.then(res => {});
Then the code on the cloud function side is as follows, summing the two incoming parameters and returning it to the client:
// hellocf cloud function index.js entry file code
'use strict';
exports.main = async (event, context) => {
//event is the parameter uploaded by the client
let c = event.a + event.b
return {
sum: c
} // 通过return返回结果给客户端
}
The cloud function has two incoming parameters, one is the event
object and the other is the context
object.
event
refers to the event that triggers the cloud function. When the client calls the cloud function, event
is the parameter passed in when the client calls the cloud function.context
object contains the context of the request, including the client's ip, ua, appId, and other information, as well as the cloud function's environment, calling source, and other information.The event object can be understood as the json object in the upstream parameters of the client. After using uni-id
and logging in successfully, an additional uniIdToken
attribute is automatically added.
The uni-id token can be obtained through event.uniIdToken
, as follows:
'use strict';
exports.main = async (event, context) => {
let token = event.uniIdToken // 客户端uni-id token
}
Therefore, developers should pay attention not to include the uniIdToken attribute in their upstream parameter objects to avoid conflicts with the same name.
The volume limit of the input parameters
The parameter content of the cloud function upstream cannot be too large.
context
object contains the context of the request, including the client's ip, ua, appId and other information, as well as the cloud function's environment, calling source, and other information.The list of properties of the context object is as follows:
属性名称 | 类型 | 说明 |
---|---|---|
SPACEINFO | object | 服务空间信息 |
|- spaceId | string | 服务空间id |
|- provider | string | 服务空间供应商:alipay|aliyun|tencent |
|- useOldSpaceId | boolean | 当前获取的服务空间id是否为迁移前的服务空间id,新增于HBuilderX 3.6.13 |
SOURCE | string | 云函数调用来源 详见 |
FUNCTION_NAME | string | 获取云函数名称 |
FUNCTION_TYPE | string | 获取云函数类型,对于云函数来说,这里一定会返回cloudfunction ,新增于HBuilderX 3.5.1。 |
CLIENTIP | string | 客户端IP。如果调用来源是其他服务器,会返回调用方的ip |
CLIENTUA | string | 客户端userAgent。注意非本地运行环境下客户端getSystemInfoSync也会获取ua参数并上传给云函数,但是云函数会从http请求头里面获取ua而不是clientInfo里面的ua |
uniIdToken | string | 客户端uni-id token字符串,新增于HBuilderX 3.5.1。 |
requestId | string | 当前请求id,新增于HBuilderX 3.5.5。 |
In addition to the above properties, if the uni-app client accesses the cloud function through callfunction, the context will also append a batch of client information.
uni.getSystemInfo
. For example, appId and osName are named in camel case. These properties are many and may change with the front-end API update. For details, see uni.getSystemInfoIn order to maintain backward compatibility, the new version does not remove the client information of the uppercase attributes of the old version, but the document is marked as obsolete. For users of newer versions of HBuilderX, please use the camelCase property returned by uni.getSystemInfo
.
HBuilderX 3.4.9起,context 的属性还可以打印出channel
和scene
,即App的渠道包标记和小程序场景值。但这个功能属于未完成功能,开发者暂不使用这2个属性,后续会升级完善。目前如开发者需要这2个属性,请自行在客户端使用uni.getLaunchOptionsSync上传。
Example:
'use strict';
exports.main = async (event, context) => {
//...
//The context of the client call can be obtained in the context
let clientIP = context.CLIENTIP // 客户端ip信息
let spaceInfo = context.SPACEINFO // 当前环境信息 {spaceId:'xxx',provider:'tencent'}
let source = context.SOURCE // 云函数调用来源
// The following properties can only be obtained by using uni-app to call with callFunction, that is, context.SOURCE=="client", if the caller is not a uni-app client, there is no corresponding data
let appid = context.appId // manifest.json中配置的appid
let deviceId = context.deviceId // 客户端标识,新增于HBuilderX 3.1.0,同uni-app客户端getSystemInfo接口获取的deviceId
//... //Other business code
}
context.SOURCE, returns the source of the cloud function call, its value range is:
value | explain |
---|---|
client | Client callFunction method |
http | Cloud function url call |
timing | Timed trigger call |
function | Called by other cloud function callFunction |
server | Called by the uniCloud management terminal, uploaded and run in HBuilderX |
'use strict';
exports.main = async (event, context) => {
let source = context.SOURCE // 当前云函数被何种方式调用
// client client callFunction method call
// http cloud function url call
// timing timing trigger call
// server is called by the management side, uploaded and run in HBuilderX
// function is called by other cloud function callFunction
}
Precautions
context.PLATFORM
has two cases: app
and app-plus
.
app
app-plus
, after uni-app 3.4.9, the value is changed to app
Except CLIENTIP, other client information can only be obtained by calling the uni-app client with callFunction. If the cloud function is urlized and called by uni-app through request, there is no client information.
In the scenario where the cloud function is URLized, the client platform information cannot be obtained. Before calling the interface interface that depends on the client platform (recommended at the cloud function entry), the client platform information can be manually passed in by modifying context.PLATFORM for other plugins (such as: uni-id) use
example:
exports.main = async (event, context) => {
context.PLATFORM = 'app'
}
Common cloud functions return data in json format to the client. The return result is wrapped under result.
The front-end initiates callFunction to the cloud to receive parameters and respond, and then feed back to the front-end, and the front-end receives it. The complete process code is as follows:
// The client initiates a call to the cloud function hellocf and passes in the data
uniCloud.callFunction({
name: 'hellocf',
data: {a:1,b:2}
}).then((res) => {
console.log(res.result) // 结果是 {sum: 3}
}).catch((err) => {
console.error(err)
})
// The code of the cloud function hellocf receives the data passed by the client, and adds a and b to the client
'use strict';
exports.main = async (event, context) => {
//event is the parameter uploaded by the client
console.log('event : ', event)
//return data to client
return {sum : event.a + event.b}
};
Then the res structure obtained by the client is as follows
{
"errCode": 0,
"errMsg": "",
"header": {
"access-control-expose-headers": "Date,x-fc-request-id,x-fc-error-type,x-fc-code-checksum,x-fc-invocation-duration,x-fc-max-memory-usage,x-fc-log-result,x-fc-invocation-code-version"
"content-disposition": "attachment"
"content-length": "38"
"content-type": "application/json"
"date": "Sat, 25 Jun 2022 19:28:34 GMT"
"x-fc-code-checksum": "92066386860027743"
"x-fc-instance-id": "c-62b761c4-5a85e238b3ce404c817d"
"x-fc-invocation-duration": "23"
"x-fc-invocation-service-version": "LATEST"
"x-fc-max-memory-usage": "66.61"
"x-fc-request-id": "80854b93-b0c7-43ab-ab16-9ee9f77ff41e"
"x-serverless-request-id": "ac1403831656185314624173902"
"x-serverless-runtime-version": "1.2.2"
}
"requestId": "ac1403831656185314624173902"
"result": {sum: 3}
"success": true
}
Among them, result
is the data returned by the developer's cloud function code, and the rest are returned by the cloud platform.
Note: When HBuilderX runs the cloud function locally, if there is no system error, only result
will be returned, and other cloud functions will be returned only if the cloud function needs to be run in the cloud.
uniCloud response body specification
In order to facilitate unified interception of errors, it is recommended that developers use uniCloud response body specification
When a business error is reported, errCode and errMsg are returned in result. as follows:
'use strict';
exports.main = async (event, context) => {
//event is the parameter uploaded by the client
console.log('event : ', event)
if (!event.a) {
return {errCode : 1,errMsg : "参数a不能为空"}
}
if (!event.b) {
return {errCode : 2,errMsg : "参数b不能为空"}
}
const c = event.a + event.b
if (isNaN(c)) {
return {errCode : 3,errMsg : "参数a和b无法求和"}
}
//return data to client
return {sum:c,errCode : 1,errMsg : "0"}
};
For details, see: Using cookies in url-based scenarios