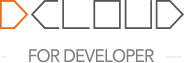
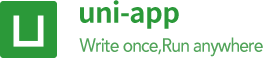
English
This document is
uni-pay 1.x
version document. Good for old projects. For new projects, please refer to uni-pay 2.x version documentation.
unipay
provides a simple, easy-to-use and unified payment capability package for uniCloud
developers. So that developers do not need to study the back-end development of Alipay, WeChat and other payment platforms, do not need to write different codes for them, use it immediately, and shield the differences.
The front-end of uni-app
has encapsulated the full-end payment api uni.requestPayment
, and now the server side has also encapsulated unipay for uniCloud
, from now on, developers can complete the front and back payment services extremely quickly.
At present, the payment capabilities of the App terminal (WeChat payment and Alipay payment), WeChat applet, and Alipay applet have been packaged.
unipay
is an open source sdk and can be used with confidence. This plug-in also includes example projects, which can be run after configuring the relevant configuration applied for by WeChat and Alipay.
为了更好的体验支付流程可以在插件市场导入unipay
的示例项目快速体验,插件市场 unipay。gitee仓库 unipay公共模块。
There are also templates repackaged based on uniPay in the plug-in market. Front-end payment and management-side order management have been written and used. See: [BaseCloud - Unified Order Payment Service Module](https://ext.dcloud.net. cn/plugin?id=2668)
Notice
appId
, mchId
need to pay attention to caseTroubleshooting Guidelines
Developers can choose whether to import from the plugin market or install from npm. The import method is slightly different. Please see the example below
// plugin market import
const unipay = require('uni-pay')
// npm install
const unipay = require('@dcloudio/unipay')
Notice
Perform the initialization operation and return the unipay instance
Added in
uni-pay 1.1.0
Entry Instructions
parameter name | type | required | default value | description |
---|---|---|---|---|
appId | String | Yes | - | The appId of the current application on the corresponding payment platform |
mchId | String | Yes | - | Merchant ID |
v3Key | String | Yes | - | API v3 key |
appCertPath | String | Yes | - | Merchant API certificate file path (choose one of file path and string) |
appCertContent | String | Yes | - | Merchant API certificate string (choose one of file path and string) |
appPrivateKeyPath | String | Yes | - | Merchant API private key file path (choose one of file path and string) |
appPrivateKeyContent | String | Yes | - | Merchant API private key string (choose one of file path and string) |
timeout | Number | No | 5000 | Request timeout, unit: millisecond |
const path = require('path'); // 引入内置的path模块
const unipayIns = unipay.initWeixinV3({
appId: 'your appId',
mchId: 'your mchId',
v3Key: 'you parterner key',
appCertPath: path.resolve(__dirname, 'your appCertPath'),
// appCertContent: "",
appPrivateKeyPath: path.resolve(__dirname, 'your appPrivateKeyPath'),
// appPrivateKeyContent: "",
})
illustrate
Certificate string and private key string format requirements:
-----BEGIN CERTIFICATE-----
and -----END CERTIFICATE-----
, and the content of the certificate cannot be wrapped-----BEGIN PRIVATE KEY-----
and -----END PRIVATE KEY-----
, and the content of the private key cannot be wrappedexample:
// certificate content
-----BEGIN CERTIFICATE-----
your certificate content...
-----END CERTIFICATE-----
// certificate string
appCertContent = 'your certificate content...'
Introduction to parameters
parameter name | type | required | default value | description |
---|---|---|---|---|
appId | String | Yes | - | The appId of the current application on the corresponding payment platform |
mchId | String | Yes | - | Merchant ID |
subAppId | String | No | - | Sub-merchant appId |
subMchId | String | No | - | Sub-merchant ID |
key | String | yes | - | payment merchant key (API key) |
pfx | String|Buffer | Required to use the refund function | - | WeChat payment merchant API certificate, mainly used for refund |
timeout | Number | No | 5000 | Request timeout, unit: millisecond |
signType | String | No | MD5 | Signature type |
sandbox | Boolean | No | false | Whether to enable the sandbox environment |
const unipayIns = unipay.initWeixin({
appId: 'your appId',
mchId: 'your mchId',
key: 'you parterner key',
pfx: fs.readFileSync('/path/to/your/pfxfile'), // 建议以p12文件绝对路径进行读取,使用微信退款时需要
})
// Take the certificate placed in the cert directory of the same level as the cloud function index.js as an example, the index.js can be written as follows
const fs = require('fs');
const path = require('path');
const unipayIns = unipay.initWeixin({
appId: 'your appId',
mchId: 'your mchId',
key: 'you parterner key',
pfx: fs.readFileSync(path.resolve(__dirname, 'cert/xxx.p12'))
})
Introduction to parameters
Parameter Name | Type | Required | Default Value | Description |
---|---|---|---|---|
appId | String | Yes | - | The appId of the current application on the corresponding payment platform |
mchId | String | Yes | - | Merchant ID |
privateKey | String | yes | - | application private key string |
alipayPublicKey | String | No | - | Alipay public key, used for signature verification |
keyType | String | No | PKCS8 | Application Private Key String Type |
timeout | Number | No | 5000 | Request timeout, unit: milliseconds |
signType | String | No | RSA2 | Signature Type |
sandbox | Boolean | No | false | Whether to enable the sandbox environment |
alipayRootCertPath | String | No | - | 1.0.6+ , Alipay root certificate file path |
appCertPath | String | No | - | 1.0.6+ , path to the application public key certificate file |
alipayPublicCertPath | String | No | - | 1.0.6+ , Alipay public key certificate file path |
const unipayIns = unipay.initAlipay({
appId: 'your appId',
mchId: 'your mchId',
privateKey: 'your privateKey',
// If you don't use a certificate (normal public key mode), you need alipayPublicKey
alipayPublicKey: 'you alipayPublicKey', // 使用支付时需传递此值做返回结果验签
// If you use a certificate, you need to pass alipayRootCertPath, appCertPath, alipayPublicCertPath
alipayRootCertPath: path.join(__dirname,'../fixtures/alipayRootCert.crt'),
appCertPath: path.join(__dirname,'../fixtures/appCertPublicKey.crt'),
alipayPublicCertPath: path.join(__dirname,'../fixtures/alipayCertPublicKey_RSA2.crt'),
})
common problem
error:0D0680A8:asn1 encoding routines:ASN1_CHECK_TLEN:wrong tag
when paying with Alipay, please confirm your private key format. If it is not PKCS8, you need to pass in the keyType parameter during initialization, and the value is the corresponding private key. key formatIntroduction to parameters
Parameter Name | Type | Required | Default Value | Description |
---|---|---|---|---|
sandbox | Boolean | no | false | whether to enable sandbox environment |
password | String | No | - | App-specific shared secret, which is the unique code used to receive a receipt for this app's auto-renewing subscription. If you are transferring this app to another developer or do not want to disclose the master shared key, it is recommended to use the app-specific shared key. This parameter is not required for non-auto-renewal scenarios |
timeout | Number | No | 5000 | Request timeout, unit: milliseconds |
const unipayIns = unipay.initAppleIapPayment({
sandbox: true,
password: 'your password',
})
unipayIns.getOrderInfo
, this interface only supports WeChat applet, Alipay applet, and App platform
Introduction to parameters
参数名 | 类型 | 必填 | 默认值 | 说明 | 支持平台 |
---|---|---|---|---|---|
openid | String | 支付宝小程序、微信小程序必填,App端支付不需要 | - | 通过对应 uni-id 接口进行获取,服务商模式应使用子商户获取的openid | 支付宝小程序、微信小程序 |
subject | String | 支付宝支付必填,微信支付时忽略此项 | - | 订单标题 | 支付宝支付 |
body | String | 微信支付必填 | - | 商品描述 | 微信支付 |
outTradeNo | String | 必填 | - | 商户订单号,有长度限制(微信支付为32字符以内,支付宝为64字符以内)、只能包含字母、数字、下划线;需保证在商户端不重复 | |
totalFee | Number | 必填 | - | 订单金额,单位:分 | 支付宝小程序、微信小程序 |
notifyUrl | String | 必填 | - | 支付结果通知地址,需要注意支付宝支付时退款也会通知到此地址,务必处理好自己的业务逻辑 | |
spbillCreateIp | String | 必填 | - | 客户端IP,云函数内可以通过context.CLIENTIP 获取 | - |
tradeType | String | 是 | - | 交易类型;见下方 tradeType 的说明 | |
sceneInfo | Object | 微信tradeType为MWEB时必填 | - | 见下方sceneInfo的说明 | - |
注意
此接口支持直接传微信和支付宝官方文档上的参数,如微信的 support_fapiao
参数,转成驼峰 supportFapiao
即可
let orderInfo = await unipayIns.getOrderInfo({
...前面参数省略
notifyUrl: 'https://xxx.xx', // 支付结果通知地址
supportFapiao: true
})
tradeType description
tradeType supports the following options
Added in uni-pay 1.0.24
)sceneInfo description
When WeChat payment tradeType is MWEB, you need to pass sceneInfo in the following format
{
"h5Info": {
"type": "Wap",
"wapUrl": "https://pay.qq.com", // 开发者网站的网址
"wapName": "腾讯充值" // 开发者网站名称
}
}
Return value description
Parameter Name | Type | Description | Supported Platforms |
---|---|---|---|
orderInfo | Object | String | The parameters required by the client for payment can be directly returned to the client. The following will introduce how to use it with the client |
Use example
When the tradeType is NATIVE, directly convert orderInfo.codeUrl to QR code and use the corresponding client to scan the code to pay.
When the tradeType is MWEB, you can directly jump to orderInfo.mwebUrl for payment
// cloud function - getOrderInfo
exports.main = async function (event,context) {
let orderInfo = await unipayIns.getOrderInfo({
openid: 'user openid',
subject: '订单标题', // 微信支付时不可填写此项
body: '商品描述',
outTradeNo: '商户订单号',
totalFee: 1, // 金额,单位分
notifyUrl: 'https://xxx.xx' // 支付结果通知地址
})
return {
orderInfo
}
}
// client
uniCloud.callFunction({
name: 'getOrderInfo',
success(res) {
uni.requestPayment({
// #ifdef APP-PLUS
provider: selectedProvider, // App端此参数必填,可以通过uni.getProvider获取
// #endif
// #ifdef MP-WEIXIN
...res.result.orderInfo,
// #endif
// #ifdef APP-PLUS || MP-ALIPAY
orderInfo: res.result.orderInfo,
// #endif
...res.result.orderInfo
success(){},
fail(){}
})
}
})
// QR code payment
uniCloud.callFunction({
name: 'getOrderInfo',
success(res) {
// Display this content as a QR code
console.log(res.result.orderInfo.codeurl)
}
})
unipayIns.orderQuery
, query order information according to the order number of the merchant or the order number of the platform. It is mainly used to verify the payment result when the payment notification is not received.
Introduction to parameters
Parameter Name | Type | Required | Default Value | Description |
---|---|---|---|---|
outTradeNo | String | Choose between transactionId and transactionId | - | Merchant order number |
transactionId | String | Choose one from outTradeNo | - | Platform order number |
Return value description
parameter name | type | description | supported platform |
---|---|---|---|
appId | String | Application ID assigned by the platform | WeChat payment |
mchId | String | Merchant ID, (WeChat payment document is called merchant ID: mch_id, Alipay payment is called seller id: seller_id) | WeChat payment |
outTradeNo | String | Merchant order number | - |
transactionId | String | Platform order number | - |
tradeState | String | order status, see the description of order status below | |
totalFee | Number | Price amount, unit: cent | - |
settlementTotalFee | Number | Amount of the order payable, unit: cents | Alipay payment |
cashFee | Number | Cash payment amount, unit: cent | - |
Order Status
WeChat Pay: SUCCESS—Successful payment REFUND—Transfer to Refund NOTPAY—Not paid CLOSED—closed REVOKED—Revoked (card payment) USERPAYING--User is paying PAYERROR--payment failed (other reasons, such as bank return failure).
pay by AliPay: USERPAYING (transaction created, waiting for buyer to pay) CLOSED (unpaid transactions are closed over time, or a full refund after payment is completed) SUCCESS (transaction payment successful) FINISHED (transaction closed, non-refundable)
Use example
exports.main = async function (event) {
let res = await unipayIns.orderQuery({
outTradeNo: 'outTradeNo',
})
return res
}
unipayIns.closeOrder
is used for the user to make no payment within a certain period of time after the transaction is created. This API can be called to directly close the unpaid transaction to avoid repeated payment.
Notice
Introduction to parameters
Parameter Name | Type | Required | Default Value | Description |
---|---|---|---|---|
outTradeNo | String | Required when using WeChat, choose either transactionId or transactionId when using Alipay | - | Merchant order number |
transactionId | String | When using Alipay and outTradeNo choose one | - | Platform order number |
Return value description
parameter name | type | description | supported platform |
---|---|---|---|
appId | String | Application ID assigned by the platform | WeChat Pay V2 |
mchId | String | Merchant ID | WeChat Pay V2 |
outTradeNo | String | Merchant order number | Alipay payment |
transactionId | String | Platform order number | Alipay payment |
Use example
exports.main = async function (event) {
let res = await unipayIns.closeOrder({
outTradeNo: 'outTradeNo',
})
return res
}
unipayIns.cancelOrder
, this interface is only supported by Alipay, if the payment transaction fails or the payment system times out, call this interface to cancel the transaction. If the user fails to pay for this order, the Alipay system will close the order; if the user pays successfully, the Alipay system will return the order funds to the user. Note: Cancellation can only be called when the payment system times out or the payment result is unknown. If you need to implement the same function for other normal payment orders, please call the Refund Request API. After submitting the payment transaction, call [Query Order API], and call [Cancel Order API] if there is no clear payment result.
Introduction to parameters
Parameter Name | Type | Required | Default Value | Description |
---|---|---|---|---|
outTradeNo | String | Choose one from transactionId | - | Merchant order number |
transactionId | String | Choose one from outTradeNo | - | Platform order number |
Return value description
Parameter Name | Type | Description | Supported Platforms |
---|---|---|---|
outTradeNo | String | Merchant order number | Alipay payment |
transactionId | String | Platform order number | Alipay payment |
Use example
exports.main = async function (event) {
let res = await unipayIns.cancelOrder({
outTradeNo: 'outTradeNo',
})
return res
}
unipayIns.refund
, when the transaction needs to be refunded due to the buyer or the seller within a certain period of time, the seller can return the payment to the buyer through the refund interface.
WeChat payment precautions
Introduction to parameters
Parameter name | Type | Mandatory | Default value | Description | Supported platforms |
---|---|---|---|---|---|
outTradeNo | String | or transactionId | - | Merchant order number | - |
transactionId | String | and outTradeNo | - | Platform order number | - |
outRefundNo | String | Mandatory for WeChat Pay, optional for Alipay | - | Merchant Refund No. | - |
totalFee | Number | Required for WeChat payment | - | Total order amount | - |
refundFee | Number | Mandatory | - | Total Refund Amount | WeChat Pay |
refundFeeType | String | Required for WeChat Pay V3 | - | Currency Type | WeChat Pay V3 |
refundDesc | String | Optional | - | Reason for refund | - |
notifyUrl | String | Optional for WeChat Pay, not supported by Alipay | - | Refund notification url, Alipay will notify you of the notification address when obtaining payment parameters | WeChat Pay |
Return value description
Parameter Name | Type | Description | Supported Platforms |
---|---|---|---|
outTradeNo | String | Merchant order number | - |
transactionId | String | Platform order number | - |
outRefundNo | String | Merchant Refund Number | WeChat Pay |
refundId | String | Platform refund order number | - |
refundFee | Number | Total refund amount | - |
cashRefundFee | Number | Cash refund amount | - |
Use example
exports.main = async function (event) {
let res = await unipayIns.refund({
outTradeNo: '商户订单号',
outRefundNo: '商户退款单号', // 支付宝可不填此项
totalFee: 1, // 订单总金额,支付宝可不填此项
refundFee: 1, // 退款总金额
})
return res
}
unipayIns.refundQuery
, after submitting a refund application, query the refund status by calling this API.
Introduction to parameters
Parameter name | Type | Mandatory | Default value | Description | Supported platforms |
---|---|---|---|---|---|
outTradeNo | String | WeChat Pay V2 to choose one of four, Alipay and transactionId to choose one of two | - | Merchant order number | - |
transactionId | String | WeChat Pay V2 to choose one of four, Alipay and outTradeNo to choose one of two | - | Platform order number | - |
outRefundNo | String | Required for WeChat Pay V3, one of four for WeChat Pay V2, required for Alipay | - | Merchant Refund No. | - |
refundId | String | WeChat payment V2 choose one of four | - | platform refund number | WeChat payment |
offset | Number | Optional for WeChat Pay V2 | - | Offset, which can be used when the number of partial refunds exceeds 10, indicating that the returned query results start to fetch records from this offset | - |
Notice
outRefundNo
is the incoming merchant refund order number when using Alipay to request a refund interface. If not passed in the refund request, the value is the merchant's order number when the transaction was created, i.e. outTradeNo
Return value description
parameter name | type | description | supported platform |
---|---|---|---|
outTradeNo | String | Merchant order number | - |
transactionId | String | Platform order number | - |
totalFee | Number | Order Amount | - |
refundId | String | Platform refund number, only returned by Alipay and WeChat Pay V3 | - |
refundFee | Number | Total Refund Amount | - |
refundDesc | String | Reason for refund | - |
refundList | Array<refundItem> | Refund information in installments, only returned by WeChat Pay V2 | WeChat Pay V2 |
refundRoyaltys | Array<refundRoyaltysItem> | Details of refund account, only returned by Alipay | Alipay payment |
refundItem description
Parameter name | Type | Description | Supported platforms |
---|---|---|---|
outRefundNo | String | Merchant refund order number | - |
refundId | String | Platform refund order number | - |
refundChannel | String | Refund channel, ORIGINAL—refund on the same route, BALANCE—return to the balance, OTHER_BALANCE—return to another account with an abnormal balance from the original account, OTHER_BANKCARD—return to another bank card in an abnormal way from the original bank card | |
refundFee | Number | Refund amount requested | - |
settlementRefundFee | Number | Refund amount, Refund amount = Refund amount applied for - Non-recharge voucher refund amount, Refund amount <= Refund amount applied | |
refundStatus | String | Refund status, SUCCESS—refund is successful, REFUNDCLOSE—refund is closed, PROCESSING—refund processing, CHANGE—refund is abnormal, the bank found that the user’s card is invalid or frozen, leading to the same way If the refund of the bank card fails, you can go to the merchant platform (pay.weixin.qq.com) - transaction center to process the refund manually. | |
couponRefundFee | Number | Total coupon refund amount | - |
couponRefundCount | Number | Number of refund coupons used | - |
refundAccount | String | Source of refund funds | - |
refundRecvAccout | String | Refund credit account | - |
refundSuccessTime | String | Refund success time | - |
couponList | Array<couponItem> | Refund information by tranches | - |
couponItem description
Parameter Name | Type | Description | Supported Platforms |
---|---|---|---|
couponType | String | Coupon Type | - |
couponRefundId | String | Refund coupon ID | - |
couponRefundFee | String | Refund amount of a single coupon | - |
refundRoyaltysItem Description
Parameter Name | Type | Description | Supported Platforms |
---|---|---|---|
fundChannel | String | The funding channel used by the transaction | - |
bankCode | String | Bank code when paying by bank card | - |
amount | Number | Amount used by this payment instrument type | - |
realAmount | Number | The actual payment amount of the channel | - |
fundType | String | The type of funds used by the channel. Currently, this information is only returned when the fund channel (fund_channel) is a bank card channel (BANKCARD) (DEBIT_CARD: debit card, CREDIT_CARD: credit card, MIXED_CARD: debit and credit in one card) | - |
Use example
exports.main = async function (event) {
let res = await unipayIns.refundQuery({
outTradeNo: '商户订单号',
outRefundNo: '商户退款单号', // 支付宝必填
})
return res
}
unipayIns.downloadBill
, merchants can download the historical transaction list through this interface. Only supported by WeChat Pay
Notice:
Introduction to parameters
Parameter Name | Type | Required | Default Value | Description |
---|---|---|---|---|
billDate | String | Mandatory | - | The date of downloading the statement, format: 2014-06-03 |
billType | String | Optional | ALL | ALL (default), returns all order information on the day (excluding recharge and refund orders), SUCCESS, returns orders successfully paid on the day (excluding recharge and refund orders), REFUND, returns Same-day refund order (excluding recharge and refund order), RECHARGE_REFUND, return the same-day recharge and refund order |
Return value description
Parameter Name | Type | Description | Supported Platforms |
---|---|---|---|
content | String | The data returned in the form of a text table | - |
An example of content
is as follows
All orders of the day Transaction Time, Public Account ID, Merchant ID, Sub-Merchant ID, Device ID, WeChat Order ID, Merchant Order ID, User ID, Transaction Type, Transaction Status, Payment Bank, Currency Type, Total Amount, Voucher or Instant Discount Amount , WeChat refund order number, merchant refund order number, refund amount, voucher or instant discount refund amount, refund type, refund status, product name, merchant data package, handling fee, rate
Orders successfully paid on the same day Transaction Time, Public Account ID, Merchant ID, Sub-Merchant ID, Device ID, WeChat Order ID, Merchant Order ID, User ID, Transaction Type, Transaction Status, Payment Bank, Currency Type, Total Amount, Voucher or Instant Discount Amount , commodity name, merchant data package, handling fee, rate
Same-day refund orders Transaction Time, Public Account ID, Merchant ID, Sub-Merchant ID, Device ID, WeChat Order ID, Merchant Order ID, User ID, Transaction Type, Transaction Status, Payment Bank, Currency Type, Total Amount, Voucher or Instant Discount Amount ,Refund application time,Refund success time,WeChat refund order number, Merchant refund order number, Refund amount, Voucher or instant discount refund amount, Refund type, Refund status, Product name, Merchant data package, fee, rate
From the second line onwards, it is a data record, each parameter is separated by a comma, and a ` symbol is added before the parameter, which is the character of the left key of standard keyboard 1, and the field order is consistent with the table header.
The penultimate line is the title of the order statistics, and the last line is the statistics
Total number of transactions, total transaction amount, total refund amount, total voucher or instant discount refund amount, total handling fee amount
An example is as follows:
交易时间,公众账号ID,商户号,子商户号,设备号,微信订单号,商户订单号,用户标识,交易类型,交易状态,付款银行,货币种类,总金额,代金券或立减优惠金额,微信退款单号,商户退款单号,退款金额,代金券或立减优惠退款金额,退款类型,退款状态,商品名称,商户数据包,手续费,费率
`2014-11-10 16:33:45,`wx2421b1c4370ec43b,`10000100,`0,`1000,`1001690740201411100005734289,`1415640626,`085e9858e3ba5186aafcbaed1,`MICROPAY,`SUCCESS,`OTHERS,`CNY,`0.01,`0.0,`0,`0,`0,`0,`,`,`被扫支付测试,`订单额外描述,`0,`0.60%
`2014-11-10 16:46:14,`wx2421b1c4370ec43b,`10000100,`0,`1000,`1002780740201411100005729794,`1415635270,`085e9858e90ca40c0b5aee463,`MICROPAY,`SUCCESS,`OTHERS,`CNY,`0.01,`0.0,`0,`0,`0,`0,`,`,`被扫支付测试,`订单额外描述,`0,`0.60%
总交易单数,总交易额,总退款金额,总代金券或立减优惠退款金额,手续费总金额
`2,`0.02,`0.0,`0.0,`0
Use example
exports.main = async function (event) {
let res = await unipayIns.downloadBill({
billDate: '20200202',
})
return res
}
unipayIns.downloadFundflow
, merchants can download historical fund flow bills from June 1, 2017 through this interface. WeChat support only
illustrate:
Introduction to parameters
Parameter Name | Type | Required | Default Value | Description |
---|---|---|---|---|
billDate | String | Mandatory | - | The date of downloading the statement, format: 2014-06-03 |
accountType | String | Optional | Basic | Account for the source of funds for the bill: Basic account, Operation account, Fees fee account |
Return value description
Parameter Name | Type | Description | Supported Platforms |
---|---|---|---|
content | String | The data returned in the form of a text table | - |
An example of content
is as follows
Accounting time, WeChat payment business order number, capital flow order number, business name, business type, income and expenditure type, income and expenditure amount (yuan), account balance (yuan), fund change submission applicant, remarks, business voucher number
From the second line onwards, it is the capital flow data, each parameter is separated by a comma, and the ` symbol is added before the parameter, which is the character of the left key of standard keyboard 1, and the field order is consistent with the table header
The penultimate row is the title of the capital bill statistics
The total number of capital flows, the number of income, the amount of income, the number of expenditures, the amount of expenditure
An example bill is as follows:
记账时间,微信支付业务单号,资金流水单号,业务名称,业务类型,收支类型,收支金额(元),账户结余(元),资金变更提交申请人,备注,业务凭证号
`2018-02-01 04:21:23,`50000305742018020103387128253,`1900009231201802015884652186,`退款,`退款,`支出,`0.02,`0.17,`system,`缺货,`REF4200000068201801293084726067
资金流水总笔数,收入笔数,收入金额,支出笔数,支出金额
`20.0,`17.0,`0.35,`3.0,`0.18
Use example
exports.main = async function (event) {
let res = await unipayIns.downloadFundflow({
billDate: '20200202',
})
return res
}
Note: Alipay will also send a notification to the notify_url set at the time of payment in the event of a partial refund
unipayIns.verifyPaymentNotify
for verifying and processing payment results within cloud functions using cloud function URLization.
Introduction to parameters
Only receive the event
corresponding to the cloud function as a parameter
Return value description
Parameter Name | Type | Description | Supported Platforms |
---|---|---|---|
totalFee | Number | Total order amount | - |
cashFee | Number | Cash payment amount | - |
feeType | String | Currency Type | - |
outTradeNo | String | Merchant order number | - |
transactionId | String | Platform order number | - |
timeEnd | String | Payment completion time in the format yyyyMMddHHmmss | - |
openid | String | user id | - |
returnCode | String | When the value is SUCCESS, the payment is successful. Usually, parameters such as order amount need to be verified | - |
Use example
exports.main = async function (event) {
let res = await unipayIns.verifyPaymentNotify(event)
// Finish other business
// Note that if the processing is successful, it must be returned in strict accordance with the following format, otherwise the manufacturer will continue to notify
// After the WeChat payment V3 is processed successfully
return {
mpserverlessComposedResponse: true,
statusCode: 200,
headers: {
'content-type': 'application/json'
},
body: JSON.stringify({
code: 'SUCCESS',
message: '成功'
})
}
// After the WeChat payment V2 is processed successfully
return {
mpserverlessComposedResponse: true,
statusCode: 200,
headers: {
'content-type': 'text/xml;charset=utf-8'
},
body: `<xml><return_code><![CDATA[SUCCESS]]></return_code><return_msg><![CDATA[OK]]></return_msg></xml>`
}
// Alipay processed successfully
return {
mpserverlessComposedResponse: true,
statusCode: 200,
headers: {
'content-type': 'text/plain'
},
body: "success"
}
}
Note: Alipay will only send a notification when it is not fully refunded, and the notification address is the notify_url set at the time of payment
uni-pay 1.0.17 version added support for Alipay refund result notification
unipayIns.verifyRefundNotify
for verifying and processing payment results within cloud functions using cloud function URLization.
Introduction to parameters
Only receive the event
corresponding to the cloud function as a parameter
Return value description
Parameter Name | Type | Description | Supported Platforms |
---|---|---|---|
totalFee | Number | Total order amount | - |
refundFee | Number | Refund amount requested | - |
settlementTotalFee | Number | The amount of the order to be settled, Alipay does not return it | - |
settlementRefundFee | Number | Refund amount, Alipay will not return | - |
outTradeNo | String | Merchant order number | - |
transactionId | String | Platform order number | - |
refundId | String | Platform refund order number, Alipay will not return it | - |
outRefundNo | String | Merchant refund order number | - |
refundStatus | String | SUCCESS - successful refund, CHANGE - abnormal refund, REFUNDCLOSE - refund closed | - |
refundAccount | String | Refund source, Alipay does not return | - |
refundRecvAccout | String | The refund is credited to the account, and Alipay does not return it | - |
Use example
exports.main = async function (event) {
let res = await unipayIns.verifyRefundNotify(event)
// Note that if the processing is successful, it needs to be returned in strict accordance with the following format, otherwise the manufacturer will continue to notify
// After the WeChat processing is successful
return {
mpserverlessComposedResponse: true,
statusCode: 200,
headers: {
'content-type': 'text/xml;charset=utf-8'
},
body: `<xml><return_code><![CDATA[SUCCESS]]></return_code><return_msg><![CDATA[OK]]></return_msg></xml>`
}
// Alipay processed successfully
return {
mpserverlessComposedResponse: true,
statusCode: 200,
headers: {
'content-type': 'text/plain'
},
body: "success"
}
}
Added in uni-id 1.0.17
unipayIns.checkNotifyType
, which is used to check the type of the current notification within the cloud function URLized using the cloud function. Since Alipay payment will call the notify_url set at the time of payment when it is not fully refunded, you can use this interface to determine the notification type before calling the verification notification
Introduction to parameters
Only receive the event
corresponding to the cloud function as a parameter
Return value description
This interface will return a string, the possible values are as follows
refund
: is currently a refund notificationpayment
: is currently a payment result notificationUse example
exports.main = async function (event) {
let res = await unipayIns.checkNotifyType(event)
if(res === 'refund') {
// refund notification
} else if(res === 'payment') {
// Payment result notification
}
}
unipayIns.verifyReceipt
, verifies the iap payment receipt and returns the transaction information.
Introduction to parameters
Parameter Name | Type | Required | Default Value | Description |
---|---|---|---|---|
receiptData | String | Yes | - | Payment receipt |
Return value description
Parameter name | Type | Description | Supported platforms |
---|---|---|---|
transactionId | String | Transaction ID | - |
tradeState | String | Order status, WeChat payment: SUCCESS—successful payment, REFUND—transferred and refunded, NOTPAY—unpaid, PAYERROR—payment failure (other reasons, such as bank return failure), SUCCESS (transaction payment successful). | - |
totalFee | Number | Price amount, unit: cents | - |
settlementTotalFee | Number | The amount of the order to be settled, unit: cent | - |
receipt | Object | Receipt information | - |
Use example
exports.main = async function (event) {
let res = await unipayIns.verifyReceipt({
receiptData: 'transactionReceipt',
})
return res
}