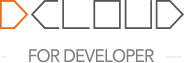
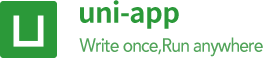
English
On November 18, 2021, both Tencent Cloud and Alibaba Cloud support
Use Tencent Cloud node12 and redis, be sure to read this document carefully: keepRunningAfterReturn
Redis is a key/value based in-memory database. It is usually used in projects as a supplement to disk databases such as MongoDB. The core advantage of Redis over disk databases is that it is fast. Because operating memory is much faster than disk, and Redis only supports key/value data, reading and writing are fast. But Redis does not have the rich query and other functions of the disk database.
Redis generally needs to be used in conjunction with MongoDB, MongoDB is the main storage, and Redis caches some data to meet high-performance demand scenarios.
In uniCloud, Redis also has a feature that it is billed according to the capacity and usage time, and accessing it does not consume the number of reads and writes of the cloud database.
Common usage scenarios of Redis:
Refer to Open redis
Redis的sdk体积不小,没有内置在云函数中。在需要Redis的云函数里,开发者需手动配置Redis扩展库。(Redis没有免费试用,需购买才可以使用)。如何启用扩展库请参考:使用扩展库
// Example of calling Redis in cloud function
'use strict';
const redis = uniCloud.redis()
exports.main = async (event, context) => {
const getResult = await redis.get('my-key')
const setResult = await redis.set('my-key', 'value-test')
return {
getResult,
setResult
}
};
Notice
uni:aa
and uni:bb
, will be displayed as a tree whose root node is uni, with aa and bb after expansion.uni:
, dcloud:
, unicloud:
are official prefixes. Keys required by the developer's own business should avoid using these prefixes.uniCloud.redis()
is called corresponds to a connection. If there is an undisconnected redis instance when called multiple times, this instance is returned. Returns a new redis instance if there is no redis instance or the previous redis instance has been disconnected.Supported since HBuilderX 3.4.10
Because Redis is in the intranet of the cloud function, it can only be accessed in the cloud cloud function, but not in the local cloud function. Every time you debug Redis-related functions, you need to upload cloud functions continuously, which seriously affects the development efficiency. Since HBuilderX 3.4.10, local cloud functions can access cloud Redis through the cloud built-in proxy. If you call Redis on the cloud locally, you will see the words HBuilderX runs and debugs the proxy request of Redis
in the cloud function log.
The data in Redis is stored in the form of key-value, the key is a string, and the value has the following types
String type, which is the simplest Redis type. It should be noted that Redis does not have a number type. If the data stored in the number type will eventually be converted to the string type.
await redis.set('string-key', 1) // 设置string-key的值为字符串"1"
await redis.get('string-key') // 获取string-key的值,"1"
List types, similar to arrays in JavaScript, but with differences. Strictly speaking, List is implemented based on linked lists, and a significant difference compared to arrays in js is the efficiency of header insertion. If you test inserting one bit at the top of an array with a length of one million in the past, you will find that this operation will take a long time. But List does not have this problem. For List, it takes the same time to insert data before and after.
Notice
await redis.lpush('list-key', 1) // 往list-key左侧添加一个元素,不存在则创建
Hash type is similar to Object in js.
await redis.hmset('hash-key', 'key1', 'value1', 'key2', 'value2') // 批量为hash-key添加键值,不存在则创建
await redis.hset('hash-key', 'key1', 'value1') // 为hash-key添加键值,不存在则创建
The collection is a unordered arrangement of String, and the elements in the collection cannot be repeated
await redis.sadd('set-key', 'value1', 'value2') // 往集合内添加数据,不存在则创建
Sorted sets, like sets, are also sets of elements of type string, and do not allow duplicate members. The difference is that each element will have a score of type double (the scores do not have to be consecutive), which is used to sort the elements
await redis.zadd('sorted-set-key', 1, 'value1') // 往有序集合内添加数据并指定分数,不存在则创建
await redis.zadd('sorted-set-key', 2, 'value2')
The number of unique elements in a collection (refers to the aggregation of Object, which can contain repeated elements). For example the set {1,3,5,1,3} has 5 elements but its cardinality is 3.
await redis.pfadd('key', 'element1', 'element2')
Redis transactions can execute multiple commands at once
await redis.multi() // 开启事务
redis.set('key', 'value')
await redis.exec() // 执行事务块内的命令
It is a string of continuous binary arrays (0 and 1), and elements can be positioned by offset (offset). BitMap uses the smallest unit bit to set 0|1 to represent the value or state of an element, and the time complexity is O(1).
await redis.setbit('key', 'offset', 'value') // 设置指定位的值
Only common commands are listed here. For complete command support, please refer to redis official documentation
Used to get data of type string
Interface form
await redis.get(key: string)
Introduction to parameters
Parameters | Description | Required | Description |
---|---|---|---|
key | key | Yes |
return value
This interface returns the obtained data (string type), and returns null to indicate that there is no such key
Example
await redis.get('string-key') // '1'
It is used to set string type data, which can be added or modified.
Interface form
The interface comes in many forms
await redis.set(key: string, value: string, flag: string)
await redis.set(key: string, value: string, mode: string, duration: number)
await redis.set(key: string, value: string, mode: string, duration: number, flag: string)
Introduction to parameters
Parameters | Description | Required | Description |
---|---|---|---|
key | key | Yes | |
value | value | Yes | |
duration | Expire time, automatically delete after expiration | No | |
mode | Identifies the unit of the duration | No (required when duration is not empty) | EX: in seconds, PX: in milliseconds |
flag | Set the status according to the status | No | NX: set only when it does not exist, XX: set it only when it exists |
return value
This interface returns the string type 'OK' to indicate that the operation is successful, and returns null to indicate that it has not been updated
Example
await redis.set('string-key', 1) // redis内存储为字符串"1"
await redis.set('string-key', '1', 'NX') // string-key不存在时设置为1
await redis.set('string-key', '1', 'EX', 100) // string-key 100秒后过期
await redis.set('string-key', '1', 'EX', 100, 'NX') // string-key不存在时设置为1,过期时间设置为100秒
Returns the substring of the string value at key
await redis.getrange(key: string, start: number, end: number)
Entry Instructions
Parameter | Description | Required | Description |
---|---|---|---|
key | key | Yes | |
start | Starting position | Yes | (0 represents the first character, -1 represents the last character, -2 represents the last 2 characters) |
end | End position | Yes | (0 represents the first character, -1 represents the last character, -2 represents the last 2 characters) |
return value
This interface returns the intercepted substring to indicate the operation is successful, returns an empty string to indicate no match
example
await redis.getrange('string-key', 0, 10)
When the key exists, set to the specified string and specify the expiration time
Interface form
await redis.setex(key: string, seconds: number, value: string)
Introduction to parameters
Parameters | Description | Required | Description |
---|---|---|---|
key | key | Yes | |
seconds | Expire Time | Yes | Unit: Seconds |
value | value | Yes |
return value
This interface returns the string type 'OK' to indicate that the operation is successful, and returns null to indicate that it has not been updated
Example
await redis.setex('string-key', 10, 'value') // 值设置为value,过期时间10秒
When the key does not exist, set to the specified string
Interface form
await redis.setnx(key: string, value: string)
Introduction to parameters
Parameters | Description | Required | Description |
---|---|---|---|
key | key | Yes | |
value | value | Yes |
return value
This interface returns the string type 'OK' to indicate that the operation is successful, and returns null to indicate that it has not been updated
Example
await redis.setnx('string-key', 'value') // 键string-key不存在时将值设置为'value'
Get the value of the specified key in batches
Interface form
await redis.mget(key1: string, key2: string, ...)
Introduction to parameters
receive a list of keys
return value
This interface returns an array of the acquired data in the incoming order, the existing key returns a string type, and the non-existing key returns null
Example
await redis.mget('key1', 'key2') // '1'
Set key-values in bulk
Interface form
await redis.mset(key1: string, value1: string, key2: string, value2: string, ...)
Introduction to parameters
Receives a list of keys and values
return value
This interface will only return OK
Example
await redis.mset('key1', '1', 'key2', '2') // 'OK'
Returns the length of the string value stored by key.
Interface form
await redis.strlen(key: string)
Entry Instructions
Parameter | Description | Required | Description |
---|---|---|---|
key | key | Yes |
return value
This interface returns the length of the string, and the key that does not exist will return 0
example
await redis.strlen('key')
Batch set key values if and only if all given keys do not exist.
Interface form
await redis.msetnx(key1: string, value1: string, key2: string, value2: string);
Introduction to parameters
Parameter | Description | Required | Description |
---|---|---|---|
key | key | Yes |
return value
This interface returns 1 to indicate successful execution
Example
await redis.msetnx('key1', 'value1', 'key2', 'value2')
Set the key to correspond to the string value, and set the key to expire after the given milliseconds (milliseconds) time
Interface form
await redis.psetex(key: string, milliseconds: number, value: string);
Entry Instructions
Parameter | Description | Required | Description |
---|---|---|---|
key | key | Yes | |
milliseconds | milliseconds | yes | |
value | value | Yes |
return value
This interface returns OK to indicate successful execution
example
await redis.psetex('key', 1666582638076, 'value')
Add 1 to the specified key
Interface form
await redis.incr(key: string)
Introduction to parameters
Parameters | Description | Required | Description |
---|---|---|---|
key | key | Yes |
return value
The interface returns the value after adding one operation (number type)
Notice
When the value of the operation is not in integer form (for example: the string "1" is in integer form, the string "a" is not in integer form), an error will be thrown directly
Example
await redis.set('string-key', '1')
await redis.incr('string-key') // 2
Adds an integer to the specified key
Interface form
await redis.incrby(key: string, increment: number)
Introduction to parameters
Parameters | Description | Required | Description |
---|---|---|---|
key | key | Yes | |
increment | Increased value | Yes |
return value
The interface returns the value after the addition operation (number type)
Notice
When the value of the operation is not in integer form (for example: the string "1" is in integer form, the string "a" is not in integer form), an error will be thrown directly
Example
await redis.set('string-key', '1')
await redis.incrby('string-key', 2) // 3
Adds a float to the specified key
Interface form
await redis.incrbyfloat(key: string, increment: number)
Introduction to parameters
Parameters | Description | Required | Description |
---|---|---|---|
key | key | Yes | |
increment | Increment value, negative values are allowed for subtraction | yes |
return value
The interface returns the value after the addition operation (number type)
Notice
Example
await redis.set('string-key', '1.1')
await redis.incrbyfloat('string-key', 2.2) // 3.30000000000000027
// Doing 0.1 + 0.2 in js will get something like 3.3000000000000003
Decrements the specified key by 1
Interface form
await redis.decr(key: string)
Introduction to parameters
Parameters | Description | Required | Description |
---|---|---|---|
key | key | Yes |
return value
The interface returns the value after subtracting 1 (number type)
Notice
When the value of the operation is not in integer form (for example: the string "1" is in integer form, the string "a" is not in integer form), an error will be thrown directly
Example
await redis.set('string-key', '1')
await redis.decr('string-key') // 0
Subtract an integer from the specified key
Interface form
await redis.decrby(key: string, decrement: number)
Introduction to parameters
Parameters | Description | Required | Description |
---|---|---|---|
key | key | Yes | |
decrement | decrement value | yes |
return value
The interface returns the value after adding one operation (number type)
Notice
When the value of the operation is not in integer form (for example: the string "1" is in integer form, the string "a" is not in integer form), an error will be thrown directly
Example
await redis.set('string-key', '1')
await redis.decrby('string-key', 2) // -1
If the key already exists and is a string, the APPEND command will append the specified value to the end of the key's original value (value).
Interface form
await redis.decrby(key: string, value: string);
Introduction to parameters
Parameter | Description | Required | Description |
---|---|---|---|
key | key | Yes | |
value | The value to be appended | Yes |
return value
This interface returns the length of the successful return value
example
await redis.decrby('key', 10);
key for delete execution
Interface form
await redis.del(key: string)
Introduction to parameters
Parameter | Description | Required | Description |
---|---|---|---|
key | key | Yes |
return value
The interface returns the number 1 to indicate the deletion is successful, and the number 0 indicates that the key does not exist and the deletion fails
example
await redis.del('string-key') // '1'
Used to serialize the given key and return the serialized value.
Interface form
await redis.dump(key: string)
Introduction to parameters
Parameter | Description | Required | Description |
---|---|---|---|
key | key | Yes |
return value
Returns null if the key does not exist, and returns the serialized value if it exists
example
await redis.set('key', 'hello')
await redis.dump('key') // '\u0000\u0005hello\t\u0000����1�C�'
Check if a key exists
Interface form
await redis.exists(key: string)
Entry Instructions
Parameter | Description | Required | Description |
---|---|---|---|
key | key | Yes |
return value
If the key exists, return the number 1, if the key does not exist, return the number 0
example
await redis.exists('string-key') // 0 | 1
Set the expiration time for the specified key
Interface form
await redis.expire(key: string, seconds: number)
Introduction to parameters
Parameter | Description | Required | Description |
---|---|---|---|
key | key | Yes | |
seconds | expiration time | yes | unit: second |
return value
If the expiration time is successfully set, the number 1 is returned, and the number 0 is returned if it fails to exist
example
await redis.expire('key', 600) // 设置key为600秒后过期
The function of EXPIREAT is similar to that of EXPIRE, both are used to set the expiration time for the key. The difference is that the time parameter accepted by the EXPIREAT command is a UNIX timestamp (unix timestamp).
Interface form
await redis.expireat(key: string, timestamp: number)
Introduction to parameters
Parameter | Description | Required | Description |
---|---|---|---|
key | key | Yes | |
seconds | UNIX timestamp | yes | unit: seconds |
return value
If the expiration time is successfully set, the number 1 is returned, and the number 0 is returned if it fails to exist
example
await redis.expireat('key', 1666584328) // 设置key将在2022-10-24 12:05过期
This command is similar to the expire command, but it sets the lifetime of the key in milliseconds instead of seconds like the expire command.
Interface form
await redis.pexpire(key: string, milliseconds: number)
Introduction to parameters
Parameter | Description | Required | Description |
---|---|---|---|
key | key | Yes | |
milliseconds | milliseconds timestamp | yes |
return value
If the expiration time is successfully set, the number 1 is returned, and the number 0 is returned if it fails to exist
example
await redis.pexpire('key', 1500) // 设置key为1500毫秒后过期
Set the timestamp (unix timestamp) of the key expiration time in milliseconds
Interface form
await redis.pexpireat(key: string, timeatamp: number)
Introduction to parameters
Parameter | Description | Required | Description |
---|---|---|---|
key | key | Yes | |
timeatamp | timestamp in milliseconds | yes |
return value
If the expiration time is successfully set, the number 1 is returned, and the number 0 is returned if it fails to exist
example
await redis.pexpireat('key', 1666584328000) // 设置key将在2022-10-24 12:05过期
Find all keys that match the given pattern.
Interface form
await redis.keys(patten: string)
Introduction to parameters
Parameter | Description | Required | Description |
---|---|---|---|
patten | expression | Yes |
return value
List of keys (of type Array) matching the given pattern.
Example
await redis.mset('one', 1, 'two', 2, 'three', 3, 'four', 4)
await redis.keys('*o*') // ['one', 'two', 'four']
await redis.keys('*') // ['one', 'two', 'three', 'four']
Remove the expiration time of the key, and the key will persist.
Interface form
await redis.persist(key: string)
Introduction to parameters
Parameters | Description | Required | Description |
---|---|---|---|
key | key | Yes |
return value
Returns 1 if the expiration time is removed successfully, returns 0 if the key does not exist or the key does not have an expiration time
Example
await redis.set('key', 'ok')
await redis.expire('key', 10) // 10秒后过期
await redis.persist('key') // 移除 key 过期时间
Get the number of milliseconds left in the expiration time
Interface form
await redis.pttl(key: string)
Introduction to parameters
Parameters | Description | Required | Description |
---|---|---|---|
key | key | Yes |
return value
If the expiration time is not set (permanently valid), return the number -1, if it does not exist or has expired, return the number -2, otherwise return the remaining seconds
Example
await redis.pttl('key')
Get the number of seconds left in the expiration time
Interface form
await redis.ttl(key: string)
Entry Instructions
Parameter | Description | Required | Description |
---|---|---|---|
key | key | Yes |
return value
If no expiration time is set (permanently valid), the number -1 is returned, and the number -2 is returned if it does not exist or has expired, otherwise the remaining seconds are returned
example
await redis.ttl('key')
Return a random key from the current database.
Interface form
await redis.randonkey()
Entry Instructions
none
return value
Returns a key when the database is not empty, returns null when the database is empty
example
await redis.randonkey()
Rename key to newkey.
Returns an error when key is the same as newkey, or key does not exist.
When newkey already exists, the rename command will overwrite the old value.
Interface form
await redis.rename(key: string, newKey: string)
Entry Instructions
Parameter | Description | Required | Description |
---|---|---|---|
key | key | Yes | |
newKey | new key name | yes |
return value
Return OK if the modification is successful, and return an error if the key does not exist
example
await redis.rename('key', 'newKey')
Rename key to newkey only if newkey does not exist.
Returns an error when key does not exist.
Interface form
await redis.renamenx(key: string, newKey: string)
Entry Instructions
Parameter | Description | Required | Description |
---|---|---|---|
key | key | Yes | |
newKey | new key name | yes |
return value
If the modification is successful, return 1, if newKey exists, return 0
example
await redis.renamenx('key', 'newKey')
Iterate over the database keys in the current database.
Interface form
await redis.scan(cursor: string)
Entry Instructions
Parameter | Description | Required | Description |
---|---|---|---|
cursor | cursor | yes |
return value
Return data containing two elements [new cursor for next iteration, 0 means it is over, [all iterated data]]
example
await redis.mset('key1', 1, 'key2', '2', 'key3', 3, 'key4', 4, 'key5', 5)
await redis.scan(0) // 返回 [6, ['key1', 'key2', 'key3', 'key4', 'key5']]
Returns the type of value stored by key.
Interface form
await redis.type(key: string)
Entry Instructions
Parameter | Description | Required | Description |
---|---|---|---|
key | key | Yes |
return value
example
await redis.set('key1', 1)
await redis.type('key1') // 返回 string
Append data at the end of List type data
Interface form
await redis.rpush(key: string, value: string)
Entry Instructions
Parameter | Description | Required | Description |
---|---|---|---|
key | key | Yes | |
value | value to append | Yes |
return value
The interface returns the length of the List after performing the append operation
Notice
The usage is the same as rpush
, appending data at the end of the List only when the list exists
Removes the last element of a list and adds that element to another list and returns.
Interface form
await redis.rpoplpush(source: string, destination: string)
Entry Instructions
Parameter | Description | Required | Description |
---|---|---|---|
source | key | Yes | |
destination | another key | Yes |
return value
If the source exists, return the removed element; if the source does not exist, return null
example
await redis.lrange('source', 0, -1) // 返回 ['a', 'b', 'c']
await redis.lrange('destination', 0, -1) // 返回 []
await redis.rpoplpush('source', 'destination') // 返回 c
await redis.lrange('source', 0, -1) // 返回 ['a', 'b']
await redis.lrange('destination', 0, -1) // 返回 ['c']
Delete a piece of data from the end of List type data and return the deleted value
Note: When the length of the List in redis is 0, it will be automatically deleted
Interface form
await redis.rpop(key: string)
Entry Instructions
Parameter | Description | Required | Description |
---|---|---|---|
key | key | Yes |
return value
The interface returns the value deleted by this operation, or null if the key does not exist
Notice
Append data at the beginning of List type data
Interface form
await redis.lpush(key: string, value: string)
Entry Instructions
Parameter | Description | Required | Description |
---|---|---|---|
key | key | Yes | |
value | value to append | Yes |
return value
The interface returns the length of the List after performing the append operation
Notice
The usage is the same as lpush
, only append data at the beginning of the List when the list exists
Delete a piece of data from the beginning of the List type data and return the deleted value
Note: When the length of the List in redis is 0, it will be automatically deleted
Interface form
await redis.lpop(key: string)
Entry Instructions
Parameter | Description | Required | Description |
---|---|---|---|
key | key | Yes |
return value
The interface returns the value deleted by this operation, or null if the key does not exist
Notice
Insert an element before or after the specified element position in the List, and do not insert if the specified element is not matched
Interface form
await redis.linsert(key: string, dir: 'BEFORE' | 'AFTER', pivot: string, value: string)
Entry Instructions
Parameter | Description | Required | Description |
---|---|---|---|
key | key | Yes | |
dir | specifies whether to insert before or after | yes | |
pivot | specifies the element to look for | Yes | |
value | specifies the value to insert | Yes |
return value
The interface returns the length of the inserted list, and -1 is returned when the value to be searched is not matched, and 0 is returned when the key does not exist.
Notice
Get the element with the specified subscript in the List
Interface form
await redis.lindex(key: string, index: number)
Entry Instructions
Parameter | Description | Required | Description |
---|---|---|---|
key | key | Yes | |
index | specified subscript | yes |
return value
The interface returns the value corresponding to the specified subscript in the list, or null if the key does not exist
Notice
Returns the length of the List
Interface form
await redis.llen(key: string)
Entry Instructions
Parameter | Description | Required | Description |
---|---|---|---|
key | key | Yes |
return value
The interface returns the length of the list, or 0 if the key does not exist
Notice
Returns the elements in the list key within the specified range specified by offsets start and stop.
Interface form
await redis.lrange(key: string, strar: number, end: number)
Entry Instructions
Parameter | Description | Required | Description |
---|---|---|---|
key | key | Yes | |
start | start subscript | yes | |
end | end subscript | yes |
return value
Returns the specified element, the type is Array
example
await redis.rpush('key', 'a')
await redis.rpush('key', 'b')
await redis.rpush('key', 'c')
await redis.lrange('key', 0, 1) // 返回 ['a', 'b']
According to the value of the parameter count, remove the elements in the list that are equal to the parameter value.
Interface form
await redis.lrem(key: string, count: number, value: string)
Entry Instructions
Parameter | Description | Required | Description |
---|---|---|---|
key | key | Yes | |
count | Number of removals | Yes | |
value | value | Yes |
count Description
The value of count can be one of the following:
return value
Returns the number of removed elements if the key exists, or 0 if the key does not exist
example
await redis.rpush('key', 'a')
await redis.lrem('key', 0, 'a') // 返回 1
Sets the value of the element at index index of the list to value .
Interface form
await redis.lset(key: string, index: number, value: string)
Entry Instructions
Parameter | Description | Required | Description |
---|---|---|---|
key | key | Yes | |
index | the subscript of the list value | Yes | |
value | value | Yes |
return value
Returns OK if the key exists, returns an error if the key does not exist
example
await redis.rpush('key', 'a')
await redis.lset('key', 0, 'b') // 返回 OK
Trim (trim) an existing list, so that the list will only contain the specified elements in the specified range
Interface form
await redis.ltrim(key: string, start: number, end: number)
Entry Instructions
Parameter | Description | Required | Description |
---|---|---|---|
key | key | Yes | |
start | starting position | yes | |
end | end position | Yes |
Notice subscript out of range
Out-of-range subscript values do not cause an error.
If the start subscript is greater than the list's largest subscript end ( llen list minus 1 ), or if start > stop , ltrim returns an empty list (because ltrim has already emptied the entire list).
If the stop subscript is greater than the end subscript, Redis sets the value of stop to end .
return value
Execute successfully and return OK
example
await redis.ltrim('key', 0, -1) // 返回 OK
Move out and get the first element of the list. If there is no element in the list, the list will be blocked until the wait times out or a pop-up element is found.
Returns a nil if the list is empty. Otherwise, returns a list with two elements, the first element is the key to which the popped element belongs, and the second element is the value of the popped element.
Interface form
await redis.blpop(key: string, timeout: number)
Entry Instructions
Parameter | Description | Required | Description |
---|---|---|---|
key | key | Yes | |
timeout | waiting timeout; unit second | yes |
return value
Returns null if the list is empty, otherwise returns an array [key, value] with two elements
example
await redis.rpush('key', 'a')
await redis.blpop('key', 10) // 返回 ['key', 'a']
Move out and get the last element of the list. If there is no element in the list, the list will be blocked until the wait times out or a pop-up element is found.
If no elements are popped within the specified time, return a nil and wait time. Otherwise, return a list with two elements, the first element is the key to which the popped element belongs, and the second element is the value of the popped element.
Interface form
await redis.brpop(key: string, timeout: number)
Entry Instructions
Parameter | Description | Required | Description |
---|---|---|---|
key | key | Yes | |
timeout | waiting timeout; unit second | yes |
return value
If no element is popped within the specified time, return null and the waiting time, otherwise return an array [key name, value] containing two elements
example
await redis.rpush('key', 'a')
await redis.brpop('key', 10) // 返回 ['key', 'a']
Take the last element from source and insert it into the head of destination; if there is no element in the list, the list will be blocked until the wait times out or a pop-up element is found.
Interface form
await redis.brpoplpush(source: string, destination: string, timeout: number)
Entry Instructions
Parameter | Description | Required | Description |
---|---|---|---|
source | key | Yes | |
destination | another key | Yes | |
timeout | wait timeout | yes |
return value
If no element is popped within the specified time, return null and the waiting time, otherwise return an array [key name, value] containing two elements
example
await redis.brpoplpush('source', 'destination', 10)
Set the value of the specified field in key.
Interface form
await redis.hset(key: string, field: string, value: string)
Entry Instructions
Parameter | Description | Required | Description |
---|---|---|---|
key | key | Yes | |
field | field name | Yes | |
value | value | Yes |
Notice
If the key does not exist, a new hash table will be created and hseted.
If the field already exists in the hashtable, the old value will be overwritten.
return value
A newly created field returns 1, and an overwritten field returns 0
example
await redis.hset('key', 'field', 'value')
Returns the value of the specified field at key.
Interface form
await redis.hget(key: string, field: string)
Entry Instructions
Parameter | Description | Required | Description |
---|---|---|---|
key | key | Yes | |
field | field name | Yes |
return value
Returns null when the field does not exist, and returns the field value if it exists
example
await redis.hset('key', 'field', 'value')
await redis.hget('key', 'field') // 返回 value
Set multiple field-values in a key at the same time
Interface form
await redis.hmset(key: string, field1: string, value1: string, field2: string, value2: string)
Entry Instructions
Parameter | Description | Required | Description |
---|---|---|---|
key | key | Yes | |
field1 | field name | Yes | |
value1 | value | Yes |
return value
An error is returned when the key is not a hash type, and OK is returned if the execution is successful
example
await redis.hmset('key', 'field1', 'value1', 'field2', 'value2')
Return multiple fields in a key
Interface form
await redis.hmget(key: string, field1: string, field2: string)
Entry Instructions
Parameter | Description | Required | Description |
---|---|---|---|
key | key | Yes | |
field1 | field name | Yes |
return value
Returns the obtained field list, type Array
example
await redis.hmget('key', 'field1', 'field2')
Return all fields and values of key
Interface form
await redis.hgetall(key: string)
Entry Instructions
Parameter | Description | Required | Description |
---|---|---|---|
key | key | Yes |
return value
Return field and value, type Array
example
await redis.hset('key', 'field1', 'value1')
await redis.hset('key', 'field2', 'value2')
await redis.hgetall('key') // 返回 ['field1', 'value1', 'field2', 'value2']
Delete one or more fields specified in key
Interface form
await redis.hdel(key: string, field1: string)
await redis.hdel(key: string, field1: string, field2: string)
Entry Instructions
Parameter | Description | Required | Description |
---|---|---|---|
key | key | Yes | |
field1 | field | Yes |
return value
Returns the number of deleted fields
example
await redis.hdel('key') // 返回 0
Check if field exists in key
Interface form
await redis.hexists(key: string, field: string)
Entry Instructions
Parameter | Description | Required | Description |
---|---|---|---|
key | key | Yes | |
field | field | Yes |
return value
Returns 1 if the field exists, returns 0 if the key does not exist or the field does not exist
example
await redis.hexists('key', 'field') // 返回 0
Adds an integer increment to the value of the field specified in key
Interface form
await redis.hincrby(key: string, field: string, increment: number)
Entry Instructions
Parameter | Description | Required | Description |
---|---|---|---|
key | key | Yes | |
field | field | Yes | |
increment | Increment integer; >0 increases, <0 decreases | Yes |
return value
Returns the incremented value
example
await redis.hincrby('key', 'field', 10) // 返回 10
Add the floating point increment increment to the value of the field specified in key
Interface form
await redis.hincrbyfloat(key: string, field: string, increment: number)
Entry Instructions
Parameter | Description | Required | Description |
---|---|---|---|
key | key | Yes | |
field | field | Yes | |
increment | Incremental float; >0 increases, <0 decreases | Yes |
return value
Returns the incremented value
example
await redis.hincrbyfloat('key', 'field', 2.5) // 返回 2.5
Return all fields in key
Interface form
await redis.hkeys(key: string)
Entry Instructions
Parameter | Description | Required | Description |
---|---|---|---|
key | key | Yes |
return value
Return all fields; type Array, return an empty array if the key does not exist
example
await redis.hkeys('key') // 返回 []
Returns the number of fields in the key
Interface form
await redis.hlen(key: string)
Entry Instructions
Parameter | Description | Required | Description |
---|---|---|---|
key | key | Yes |
return value
Returns the number of fields, and returns 0 if the key does not exist
example
await redis.hlen('key') // 返回 0
Set the value when the field in the key does not exist, and the operation is invalid when the field already exists.
Interface form
await redis.hsetnx(key: string, field: string, value: string)
Entry Instructions
Parameter | Description | Required | Description |
---|---|---|---|
key | key | Yes | |
field | field | Yes | |
value | value | Yes |
return value
Returns 1 if the setting is successful, returns 0 if the field already exists
example
await redis.hsetnx('key', 'field', 'value') // 返回 1
Return the value of all fields in key
Interface form
await redis.hvals(key: string)
Entry Instructions
Parameter | Description | Required | Description |
---|---|---|---|
key | key | Yes |
return value
Return the value of all fields, the type is Array, and return an empty array when the key does not exist
example
await redis.hvals('key') // 返回 []
Iterate over the key-value pairs in the hash key
Interface form
await redis.hscan(key: string, cursor: number)
Entry Instructions
Parameter | Description | Required | Description |
---|---|---|---|
key | key | Yes | |
cursor | cursor | yes |
return value
Return data containing two elements [new cursor for next iteration, 0 means it is over, [all iterated data]]
example
await redis.hscan('key', 0) // 返回 []
Add one or more members to the collection key, the member elements already in the collection will be ignored.
Interface form
await redis.sadd(key: string, member: string)
await redis.sadd(key: string, member1: string, member2: string)
Entry Instructions
Parameter | Description | Required | Description |
---|---|---|---|
key | key | Yes | |
member | member | Yes |
return value
Returns the number of new elements added to the collection, excluding ignored elements.
example
await redis.sadd('key', 'm1', 'm2') // 返回 2
Returns the cardinality of the key stored in the collection (the number of elements in the collection)
Interface form
await redis.scard(key: string)
Entry Instructions
Parameter | Description | Required | Description |
---|---|---|---|
key | key | Yes |
return value
Returns the cardinality of the key stored in the set, or returns 0 if the key does not exist
example
await redis.sadd('key', 'a', 'b')
await redis.scard('key') // 返回 2
Returns all members of a set that is the difference between all given sets.
A key that does not exist is considered an empty set.
Interface form
await redis.sdiff(key1: string, key2: string)
await redis.sdiff(key1: string, key2: string, key3: string)
Entry Instructions
Parameter | Description | Required | Description |
---|---|---|---|
key1 | the key of the collection | Yes | |
key2 | the key of the set | Yes |
return value
Returns a list of subtraction members. Type Array
example
await redis.sadd('key1', 'a', 'b', 'c')
await redis.sadd('key2', 'c', 'd', 'f')
await redis.sdiff('key1', 'key2') // 返回 ['b', 'a']
This command works like sdiff, but it saves the results to the destination collection instead of simply returning the result set.
If the destination collection already exists, it is overwritten.
destination can be the key itself.
Interface form
await redis.sdiffstore(destination: string, key1: string, key2: string)
await redis.sdiffstore(destination: string, key1: string, key2: string, key3: string)
Entry Instructions
Parameter | Description | Required | Description |
---|---|---|---|
destination | The key name that needs to be stored | Yes | |
key1 | the key of the collection | Yes | |
key2 | the key of the set | Yes |
return value
Returns the number of elements in the result set
example
await redis.sadd('key1', 'a', 'b', 'c')
await redis.sadd('key2', 'c', 'd', 'f')
await redis.sdiff('destination', 'key1', 'key2') // 返回 2
Returns all members of a set that is the intersection of all given sets.
A key that does not exist is considered an empty set.
When there is an empty set among the given sets, the result is also an empty set (by the laws of set operations).
Interface form
await redis.sinter(key1: string, key2: string)
await redis.sinter(key1: string, key2: string, key3: string)
Entry Instructions
Parameter | Description | Required | Description |
---|---|---|---|
key1 | the key of the collection | Yes | |
key2 | the key of the set | Yes |
return value
Returns a list of intersection members, of type Array
example
await redis.sadd('key1', 'a', 'b', 'c')
await redis.sadd('key2', 'c', 'd', 'f')
await redis.sinter('key1', 'key2') // 返回 ['c']
This command is similar to the sinter command, but it saves the results to the destination collection instead of simply returning a result set.
If the destination collection already exists, it is overwritten.
destination can be the key itself.
Interface form
await redis.sinterstore(destination: string, key1: string, key2: string)
await redis.sinterstore(destination: string, key1: string, key2: string, key3: string)
Entry Instructions
Parameter | Description | Required | Description |
---|---|---|---|
destination | The key name that needs to be stored | Yes | |
key1 | the key of the collection | Yes | |
key2 | the key of the set | Yes |
return value
Returns the number of members in the result set.
example
await redis.sadd('key1', 'a', 'b', 'c')
await redis.sadd('key2', 'c', 'd', 'f')
await redis.sinterstore('destination', 'key1', 'key2') // 返回 1
Determine whether the member element is a member of the set key.
Interface form
await redis.sismember(key: string, member: string)
Entry Instructions
Parameter | Description | Required | Description |
---|---|---|---|
key | the key of the collection | Yes | |
member | member | Yes |
return value
Return 1 if the member element is a member of the set, return 0 if it is not a member of the set or the key does not exist
example
await redis.sadd('key', 'a', 'b', 'c')
await redis.sismember('key', 'a') // 返回 1
Returns all members in the set key.
Interface form
await redis.smembers(key: string)
Entry Instructions
Parameter | Description | Required | Description |
---|---|---|---|
key | the key of the collection | Yes |
return value
Returns all members in the collection, type Array example
await redis.sadd('key', 'a', 'b', 'c')
await redis.smembers('key', 'a') // 返回 ['a', 'b', 'c']
Moves the member element from the source collection to the destination collection.
Interface form
await redis.smove(source: string, destination: string, member: string)
Entry Instructions
Parameter | Description | Required | Description |
---|---|---|---|
source | the key of the collection | Yes | |
destination | the key of the collection | Yes | |
member | The member to move | Yes |
return value
If the member is removed successfully, return 1, if the member is not a member of the source collection, and there is no operation performed on the destination collection, then return 0.
example
await redis.sadd('source', 'a', 'b', 'c')
await redis.smove('source', 'destination', 'a') // 返回 1
Removes and returns a random element from the collection.
Interface form
await redis.spop(key: string)
Entry Instructions
Parameter | Description | Required | Description |
---|---|---|---|
key | the key of the collection | Yes |
return value
Returns the random element that was removed. Returns null when key does not exist or key is an empty set.
example
await redis.spop('key')
Randomly get one or count elements in the collection key
Interface form
await redis.srandmember(key: string)
await redis.srandmember(key: string, count: number)
Entry Instructions
Parameter | Description | Required | Description |
---|---|---|---|
key | the key of the collection | Yes | |
count | how many elements to return | no |
count Description
return value
Returns an element when only the key parameter is provided, or nil if the collection is empty.
Returns an array if the count parameter is provided, or an empty array if the collection is empty.
example
await redis.srandmember('key')
Remove one or more member elements in the collection key, and the non-existing member elements will be ignored.
Interface form
await redis.srem(key: string, member: string)
await redis.srem(key: string, member1: string, member2: string)
Entry Instructions
Parameter | Description | Required | Description |
---|---|---|---|
key | the key of the collection | Yes | |
member | member | Yes |
return value
Returns the number of elements that were successfully removed, excluding ignored elements.
example
await redis.srem('key', 'member')
Returns all members of a set that is the union of all given sets.
Interface form
await redis.sunion(key: string)
await redis.sunion(key1: string, key2: string)
Entry Instructions
Parameter | Description | Required | Description |
---|---|---|---|
key | the key of the collection | Yes | |
member | member | Yes |
return value
Returns a list of union members.
example
await redis.sunion('key1', 'key2')
This command is similar to the sunion command, but it saves the results to the destination collection instead of simply returning a result set.
If destination already exists, it is overwritten.
destination can be the key itself.
Interface form
await redis.sunionstore(destination: string, key: string)
await redis.sunionstore(destination: string, key1: string, key2: string)
Entry Instructions
Parameter | Description | Required | Description |
---|---|---|---|
destination | the key of the collection | Yes | |
key | the key of the collection | Yes |
return value
Returns the number of elements in the result set.
example
await redis.sunionstore('destination', 'key')
Iterate over the elements in the collection key.
Interface form
await redis.sscan(key: string, cursor: number)
Entry Instructions
Parameter | Description | Required | Description |
---|---|---|---|
key | the key of the collection | Yes | |
cursor | cursor | yes |
return value
Return data containing two elements [new cursor for next iteration, 0 means it is over, [all iterated data]]
example
await redis.sscan('key', 0)
Add one or more member elements and their score values to the sorted set key.
If a member is already a member of the ordered set, update the score value of the member and reinsert the member element to ensure that the member is in the correct position.
Interface form
await redis.zadd(key: string, score: string, member: string)
await redis.zadd(key: string, score1: string, member1: string, score2: string, member2: string)
Entry Instructions
Parameter | Description | Required | Description |
---|---|---|---|
key | the key of the collection | Yes | |
score | score; can be an integer value or a double precision floating point number | Yes | |
member | element | Yes |
return value
Returns the number of new members that were successfully added, excluding existing members that were updated.
example
await redis.zadd('key', '0', 'a')
Returns the cardinality of the sorted set key.
Interface form
await redis.zcard(key: string)
Entry Instructions
Parameter | Description | Required | Description |
---|---|---|---|
key | the key of the collection | Yes |
return value
Returns the cardinality of the sorted set when key exists and is of sorted set type. Returns 0 when the key does not exist.
example
await redis.zcard('key')
Returns the number of members whose score value is between min and max (default includes score equal to min or max ) in the sorted set key.
Interface form
await redis.zcount(key: string, min: number, max: number)
Entry Instructions
Parameter | Description | Required | Description |
---|---|---|---|
key | the key of the collection | Yes | |
min | minimum score | yes | |
max | maximum score | yes |
return value
Returns the number of members with score values between min and max.
example
await redis.zcount('key', 0, -1)
Adds increment to the score value of member member of sorted set key.
Interface form
await redis.zincrby(key: string, increment: number, member: number)
Entry Instructions
Parameter | Description | Required | Description |
---|---|---|---|
key | the key of the collection | Yes | |
increment | incremental score | yes | |
member | member | Yes |
return value
Returns the new score value for the member member, represented as a string.
example
await redis.zincrby('key', 10, 'member')
Computes the intersection of the given one or more sorted sets, where the number of given keys must be specified with the numkeys parameter, and stores the intersection (result set) in destination .
Interface form
await redis.zinterstore(destination: string, numkeys: number, key: string)
await redis.zinterstore(destination: string, numkeys: number, key1: string, key2: string)
Entry Instructions
Parameter | Description | Required | Description |
---|---|---|---|
destination | the key of the collection | Yes | |
numkeys | number of sets | yes | |
key | Collection key name | Yes |
return value
Returns the cardinality of the result set saved to destination.
example
await redis.zinterstore('destination', 1, 'key')
Returns the members in the specified range in the ordered set key.
Interface form
await redis.zrange(key: string, start: number, stop: number)
await redis.zrange(key: string, start: number, stop: number, 'WITHSCORES')
Entry Instructions
Parameter | Description | Required | Description |
---|---|---|---|
key | Collection key name | Yes | |
start | starting position | yes | |
stop | end position | yes |
return value
Returns a list of ordered set members with score value (optional) within the specified range, type Array
example
await redis.zrange('key', 0, -1)
Returns the rank of member member in the sorted set key. Among them, the members of the ordered set are arranged in the order of increasing score value (from small to large).
Interface form
await redis.zrank(key: string, member: string)
Entry Instructions
Parameter | Description | Required | Description |
---|---|---|---|
key | Collection key name | Yes | |
member | member | Yes |
return value
If member is a member of the sorted set key, returns the rank of member.
Returns null if member is not a member of the sorted set key.
example
await redis.zrank('key', 'member')
Remove one or more members from the sorted set key, non-existing members will be ignored.
Interface form
await redis.zrem(key: string, member: string)
await redis.zrem(key: string, member1: string, member2: string)
Entry Instructions
Parameter | Description | Required | Description |
---|---|---|---|
key | Collection key name | Yes | |
member | member | Yes |
return value
Returns the number of members successfully removed, excluding ignored members.
example
await redis.zrem('key', 'member')
Returns the members in the specified interval in the ordered set key, where the positions of the members are arranged in descending order of score value (from large to small).
Interface form
await redis.zrevrange(key: string, start: number, stop: number)
await redis.zrevrange(key: string, start: number, stop: number, 'WITHSCORES')
Entry Instructions
Parameter | Description | Required | Description |
---|---|---|---|
key | Collection key name | Yes | |
start | starting position | yes | |
stop | end position | yes |
return value
Returns a list of ordered set members with score value (optional) within the specified range, type Array.
example
await redis.zrevrange('key', 0,-1)
Return the members of the ordered set (with paging function) through the dictionary interval (the set is sorted from small to large by score)
Interface form
await redis.zrevrangebyscore(key: string, min: number, max: number)
await redis.zrevrangebyscore(key: string, min: number, max: number, withscores: string, limit: string, offset: number, count: number)
Entry Instructions
Parameter | Description | Required | Description |
---|---|---|---|
key | Collection key name | Yes | |
min | Members with smaller sorting positions in the dictionary must start with "[", or start with "(", you can use "-" instead | Yes | |
max | The member with a larger sorting position in the dictionary must start with "[" or "(", and "+" can be used instead | Yes | |
withscores | WITHSCORES | no | |
limit | Whether the returned result should be paginated, offset and count must be entered after LIMIT is included in the command | No | |
offset | Return the starting position of the result | No | |
count | Return the number of results | No |
return value
Returns a list of sorted set members with score values (optional) in the specified range.
example
await redis.zrevrangebyscore('key', 1000,200)
Returns the rank of the member member in the sorted set key, where the sorted set members are arranged from high to low according to the score value.
The ranking starts from 0, that is, the member with the highest score is ranked 0.
Interface form
await redis.zrevrank(key: string, member: string)
Entry Instructions
Parameter | Description | Required | Description |
---|---|---|---|
key | Collection key name | Yes | |
member | member | Yes |
return value
If member is a member of the sorted set key, returns the rank of member. Returns null if member is not a member of the sorted set key.
example
await redis.zrevrank('key', 'member')
Get the score value of the member member in the ordered set key.
Interface form
await redis.zscore(key: string, member: string)
Entry Instructions
Parameter | Description | Required | Description |
---|---|---|---|
key | Collection key name | Yes | |
member | member | Yes |
return value
Return the score value of the member member, expressed in string form, if the key does not exist or the member is not a member of the key, return null .
example
await redis.zscore('key', 'member')
Computes the union of the given one or more sorted sets, where the number of given keys must be specified with the numkeys parameter, and stores the union (result set) in destination .
Interface form
await redis.zunionstore(destination: string, numkeys: string, key: string)
await redis.zunionstore(destination: string, numkeys: string, key1: string, key2: string)
Entry Instructions
Parameter | Description | Required | Description |
---|---|---|---|
destination | collection key name | yes | |
numkeys | number of sets | yes | |
key | Collection key name | Yes |
return value
Returns the cardinality of the result set saved to destination.
example
await redis.zunionstore('destination', 1, 'key')
Iterate over the elements in an ordered collection (including element members and element scores)
Interface form
await redis.zscan(key: string, cursor: string)
Entry Instructions
Parameter | Description | Required | Description |
---|---|---|---|
key | Collection key name | Yes | |
cursor | cursor | yes |
return value
Return data containing two elements [new cursor for next iteration, 0 means it is over, [all iterated data]]
example
await redis.zscan('key', 0)
Add the specified element to HyperLogLog.
Interface form
await redis.pfadd(key: string, element1: string, element2: string)
Entry Instructions
Parameter | Description | Required | Description |
---|---|---|---|
key | key name | Yes | |
element1 | element 1 | Yes | |
element2 | element 2 | Yes |
return value
Returns 1 if at least one element was added, 0 otherwise.
example
await redis.pfadd('key', 'element1', 'element2')
Returns the cardinality estimate for the given HyperLogLog.
Interface form
await redis.pfcount(key: string)
Entry Instructions
Parameter | Description | Required | Description |
---|---|---|---|
key | key name | Yes |
return value
Returns the base value for a given HyperLogLog, or the sum of base estimates if multiple HyperLogLogs.
example
await redis.pfadd('key', 'a', 'b', 'c', 'b', 'c')
await redis.pfcount('key') // 返回 3
Merge multiple HyperLogLogs into one HyperLogLog
Interface form
await redis.pfmerge(destkey: string, sourcekey: string)
await redis.pfmerge(destkey: string, sourcekey1: string, sourceKey2: string)
Entry Instructions
Parameter | Description | Required | Description |
---|---|---|---|
destKey | destination key name | yes | |
sourceKey | key name | yes |
return value
Returns OK on success
example
await redis.pfadd('dest', 'a', 'b', 'c', 'b', 'c')
await redis.pfadd('source', 'a', 'b', 'c', 'b', 'c')
await redis.pfmerge('key', 'dest', 'source')
await redis.pfcount('key') // 返回 3
Execute multiple instructions as a single atom.
example
const multi = redis.multi()
multi.set('key1', 'value1')
multi.set('key2', 'value2')
multi.set('key3', 'value3')
multi.set('key4', 'value4')
const res = await multi.exec()
// if the execution was successful
res = ['OK','OK','OK','OK']
// An error occurred in an operation
res = ['OK','OK', error, 'OK'] // error为 Error对象的实例
Executes the commands inside all transaction blocks.
Interface form
await redis.exec()
Cancel the transaction, abandoning the execution of all commands within the transaction block.
Interface form
await redis.discard()
For the string value stored by the key, set or clear the bit at the specified offset offset
The bit is set or cleared depending on the value value, i.e. 1 or 0
Interface form
await redis.setbit(key: string, offset: number, value: number)
Entry Instructions
Parameter | Description | Required | Description |
---|---|---|---|
key | key name | Yes | |
offset | bits | yes | |
value | the value of the bit; 0 or 1 | Yes |
return value
Returns the original value at offset offset
example
await redis.setbit('key', 0, 1)
Return the string corresponding to the key, the bit at the offset position (bit)
Interface form
await redis.getbit(key: string, offset: number)
Entry Instructions
Parameter | Description | Required | Description |
---|---|---|---|
key | key name | Yes | |
offset | bits | yes |
return value
Returns the bit value at the offset offset, and returns 0 when the key does not exist or when the offset is greater than the length of the value
example
await redis.setbit('key', 1, 1)
await redis.getbit('key', 1) // 返回 1
Count the number of bits with 1 in the given string
Interface form
await redis.bitcount(key: string, start: number, end: number)
Entry Instructions
Parameter | Description | Required | Description |
---|---|---|---|
key | key name | Yes | |
start | start bit | yes | |
end | end bit | yes |
return value
Returns the number with a bit value of 1, if the key does not exist, returns 0
example
await redis.setbit('key', 1, 1)
await redis.bitcount('key', 0, -1) // 返回 1
Perform bit operations on one or more string keys that hold binary bits, and store the result on destkey.
Interface form
await redis.bitop(operation: string, destkey: string, key: string)
await redis.bitop(operation: string, destkey: string, key1: string, key2: string)
Entry Instructions
Parameter | Description | Required |
---|---|---|
operation | operation; one of AND, OR, NOT, XOR | Yes |
destkey | key name | yes |
key | key name | Yes |
return value
Returns the length of the string saved to destkey (equal to the length of the longest string in the key given by the parameter)
example
await redis.bitop('AND', 'andkey', 'key1', 'key2')
Returns the offset of the first bit value of bit (0 or 1) from left to right in the string
Interface form
await redis.bitpos(key: string, value: string, start: number, end: number)
Entry Instructions
Parameter | Description | Required |
---|---|---|
key | key name | Yes |
value | value; 0 or 1 | yes |
start | start byte, note that 1 byte = 8 bits | yes |
end | end byte, note that 1 byte = 8 bits | yes |
return value
Returns the offset where the first bit value is the specified bit (0 or 1)
example
await redis.set('key', 'a')
await redis.bitpos('key', 1) // 返回 1
Interface form
await redis.eval(String script, Number argsCount, String key1, String key2 , ... , String arg1, String arg2, ...)
Parameter Description
Parameter | Type | Required | Description |
---|---|---|---|
script | String | Yes | lua script content |
argsCount | Number | Yes | Number of parameters, if there is no parameter, pass 0 |
key1, key2... | String | No | Starting from the third parameter of eval, it represents those Redis keys (keys) used in the script. These key name parameters can be passed through the global variable KEYS array in Lua , with 1-based access ( KEYS[1] , KEYS[2] , and so on) |
arg1, agr2... | Number | yes | Additional parameters, accessed in Lua through the global variable ARGV array, in a similar way to the KEYS variable ( ARGV[1] , ARGV[2] , etc.) |
In some cases, complex atomic operations need to be used to avoid the problem of chaotic data modification under high concurrency. This requirement can generally be achieved by executing lua scripts. As shown in the following example, when it is judged that key-test does not exist in redis, set its value to 1; if it exists and less than 10, add one operation; if it is greater than or equal to 10, no operation is performed and returned directly.
{0, 1}
is the table type in lua. When returning to the cloud function, it will be converted to an array with the corresponding value of [0, 1]
const [operationType, currentValue] = await redis.eval(`local val = redis.call('get','key-test')
local valNum = tonumber(val)
if (val == nil) then
redis.call('set', 'key-test', 1)
return {0, 1}
end
if (valNum < 10) then
redis.call('incrby', 'key-test', 1)
return {1, valNum + 1}
else
return {2, valNum}
end
`, 0)
Disconnecting the redis connection will wait for the redis request to complete before disconnecting
Interface form
await redis.quit()
Introduction to parameters
none
return value
Returns OK
string after successful call
Notice
uniCloud.redis()
method to establish a connectionconnection between cloud functions and redis
Different from traditional development, cloud function instances are not interoperable, that is to say, each function instance using redis will establish a connection with redis, and this connection will also be reused when the cloud function instance is reused.
The atomic operation of redis can be used to ensure that it will not be oversold under high concurrency. The following is a simple example
You can synchronize the product inventory to redis before the snap-buying event starts. In actual business, you can load all the products by visiting the snap-buying page once in advance. The following is achieved through a simple demo code
const redis = uniCloud.redis()
const goodsList = [{ // 商品库存信息
id: 'g1',
stock: 100
}, {
id: 'g2',
stock: 200
}, {
id: 'g3',
stock: 400
}]
const stockKeyPrefix = 'stock_'
async function init() { // 抢购活动开始前在redis初始化库存
for (let i = 0; i < goodsList.length; i++) {
const {
id,
stock
} = goodsList[i];
await redis.set(`${stockKeyPrefix}${id}`, stock)
}
}
init()
See the following code for the logic of snapping
exports.main = async function (event, context) {
// Some logic for judging whether the snap-purchase activity starts/finishes, and returns directly if it does not start
const cart = [{ // 购物车信息,此处演示购物车抢购的例子,如果抢购每次下单只能购买一件商品,可以适当调整代码
id: 'g1', // 商品id
amount: parseInt(Math.random() * 5 + 5) // 购买数量
}, {
id: 'g2',
amount: parseInt(Math.random() * 5 + 5)
}]
/**
* 使用redis的eval方法执行lua脚本判断各个商品库存能否满足购物车购买的数量
* 如果不满足,返回库存不足的商品id
* 如果满足,返回一个空数组
*
* eval为原子操作可以在高并发下保证不出错
**/
let checkAndSetStock = `
local cart = {${cart.map(item => `{id='${item.id}',amount=${item.amount}}`).join(',')}}
local amountList = redis.call('mget',${cart.map(item => `'${stockKeyPrefix}${item.id}'`).join(',')})
local invalidGoods = {}
for i,cartItem in ipairs(cart) do
if (cartItem['amount'] > tonumber(amountList[i])) then
table.insert(invalidGoods, cartItem['id'])
break
end
end
if(#invalidGoods > 0) then
return invalidGoods
end
for i,cartItem in ipairs(cart) do
redis.call('decrby', '${stockKeyPrefix}'..cartItem['id'], cartItem['amount'])
end
return invalidGoods
`
const invalidGoods = await redis.eval(checkAndSetStock, 0)
if (invalidGoods.length > 0) {
return {
code: -1,
message: `以下商品库存不足:${invalidGoods.join(',')}`, // 此处为简化演示代码直接返回商品id给客户端
invalidGoods // 返回库存不足的商品列表
}
}
// The redis inventory has been deducted, and the inventory is synchronized to the database. Create an order for a user
// Code omitted here...
return {
code: 0,
message: '下单成功,跳转订单页面'
}
}
```