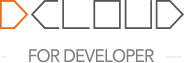
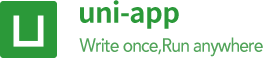
English
Apply to activate
WeChat payment function in the application details, fill in the information according to the page prompts, and submit for reviewmerchant ID
(PartnerID, required for payment orders) and login password
merchant ID
and login password
, go to "Account Center" > "API Security" ” > “Set APIv2 Key” Set the API key (used by the server to generate orders). For details, please refer to API certificate and keyFor more information, please refer to the official WeChat document APP payment access application process guidelines, and the server access related information please refer to Preparation for APP payment access
Notice
Before invoking payment on the app side, a payment order needs to be generated on the business server, please refer to:
For more information, please refer to WeChat payment official document APP payment unified order
wxpay
, and the value of the orderInfo attribute is the order objectObject object type
Attribute | Type | Required | Description |
---|---|---|---|
appid | String | Yes | Application ID (AppID), please log in to WeChat Open Platform to view, note that it is different from the APPID of the official account |
partnerid | String | Yes | The merchant ID (PartnerID) allocated by WeChat Pay |
prepayid | String | Yes | Prepay transaction session ID |
package | String | Yes | Extended field, fixed value "Sign=WXPay" |
noncestr | String | yes | random string, no longer than 32 bits |
timestamp | String | Yes | Timestamp, standard Beijing time, the time zone is Dongba District, the number of seconds since 0:00:00 on January 1, 1970 |
sign | String | Yes | Signature, see Signature Generation Algorithm |
For more information, please refer to the official WeChat payment document APP payment calls up payment interface
//Order object, obtained from the server
var orderInfo = {
"appid": "wx499********7c70e", // 应用ID(AppID)
"partnerid": "148*****52", // 商户号(PartnerID)
"prepayid": "wx202254********************fbe90000", // 预支付交易会话ID
"package": "Sign=WXPay", // 固定值
"noncestr": "c5sEwbaNPiXAF3iv", // 随机字符串
"timestamp": 1597935292, // 时间戳(单位:秒)
"sign": "A842B45937F6EFF60DEC7A2EAA52D5A0" // 签名,这里用的 MD5 签名
};
uni.getProvider({
service: 'payment',
success: function (res) {
console.log(res.provider)
if (~res.provider.indexOf('wxpay')) {
uni.requestPayment({
"provider": "wxpay", //固定值为"wxpay"
"orderInfo": orderInfo,
success: function (res) {
var rawdata = JSON.parse(res.rawdata);
console.log("支付成功");
},
fail: function (err) {
console.log('支付失败:' + JSON.stringify(err));
}
});
}
}
});
//Order object, obtained from the server
var orderInfo = {
"appid": "wx499********7c70e", // 应用ID(AppID)
"partnerid": "148*****52", // 商户号(PartnerID)
"prepayid": "wx202254********************fbe90000", // 预支付交易会话ID
"package": "Sign=WXPay", // 固定值
"noncestr": "c5sEwbaNPiXAF3iv", // 随机字符串
"timestamp": 1597935292, // 时间戳(单位:秒)
"sign": "A842B45937F6EFF60DEC7A2EAA52D5A0" // 签名,这里用的 MD5 签名
};
//get payment channel
var wxpaySev = null;
plus.payment.getChannels(function(channels){
for (var i in channels) {
var channel = channels[i];
if (channel.id === 'wxpay') {
wxpaySev = channel;
}
}
//Initiate payment
plus.payment.request(wxpaySev, orderInfo, function(result) {
var rawdata = JSON.parse(result.rawdata);
console.log("支付成功");
}, function(e) {
console.log("支付失败:" + JSON.stringify(e));
});
}, function(e){
console.log("获取支付渠道失败:" + JSON.stringify(e));
});