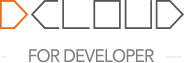
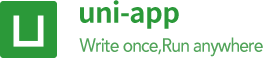
English
uni-app supports ts development, please refer to Vue.js TypeScript Support for instructions.
The type definition file is provided by the @dcloudio/types module. After installation, please configure the compilerOptions > types section in the tsconfig.json file. You can also install other miniprogram platform type definitions, such as: miniprogram-api-typings, mini-types . For missing or wrong type definitions, you can add or modify them locally and report to the official request for updates at the same time.
In the script node of the vue or nvue page, add the attribute lang="ts"
<script lang="ts">
// write ts code here
let s:string = "123"
console.log(s)
</script>
If you need to use vue components, you need import Vue from 'vue'
, specifically see below
You need to specify ts when creating a project. For details, please refer to documentation
Create a tsconfig.json
file in the root directory and perform personalized configuration. The recommended configuration is as follows:
// tsconfig.json
{
"compilerOptions": {
"target": "esnext",
"module": "esnext",
"strict": true,
"jsx": "preserve",
"moduleResolution": "node",
"esModuleInterop": true,
"sourceMap": true,
"skipLibCheck": true,
"importHelpers": true,
"allowSyntheticDefaultImports": true,
"useDefineForClassFields": true,
"resolveJsonModule": true,
"lib": [
"esnext",
"dom"
],
"types": [
"@dcloudio/types"
]
},
"exclude": [
"node_modules",
"unpackage",
"src/**/*.nvue"
]
}
Personalized configuration is optional, without tsconfig.json
it will automatically run with the default configuration.
After declaring lang="ts"
, all vue components imported by this vue/nvue file need to be written in ts.
Sample code
Modification uni-badge.vue
<script lang="ts">
//Only the core code that needs to be modified is displayed. For the complete code, please refer to the original components.
import Vue from 'vue';
export default Vue.extend({
props: {
type: {
type: String,
default: 'default'
},
inverted: {
type: Boolean,
default: false
},
text: {
type: [String, Number],
default: ''
},
size: {
type: String,
default: 'normal'
}
},
computed: {
setClass(): string {
const classList: string[] = ['uni-badge-' + this.type, 'uni-badge-size-' + this.size];
if (this.inverted === true) {
classList.push('uni-badge-inverted')
}
return classList.join(" ")
}
},
methods: {
onClick() {
this.$emit('click')
}
}
})
</script>
Reference the uni-badge component in index.vue
<script lang="ts">
import Vue from 'vue';
import uniBadge from '../../components/uni-badge.vue';
export default Vue.extend({
data() {
return {
title: 'Hello'
}
},
components:{
uniBadge
}
});
</script>