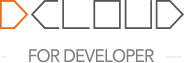
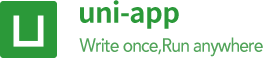
English
Most of the error messages of the MiniApp platform are returned in the format of errCode and errMsg. However, different MiniApp platforms may return different errCode.
In actual development, the uni-app engine, third-party plug-ins, and the developer's own business code will all return errors, but errCode may also conflict with each other.
In unified error interception and uni statistics, confusing errCode will cause many problems.
Many errors are caused by lower-level errors, but only the outermost errors are exposed, which is difficult to troubleshoot, and lower-level errors need to be exposed.
In order to further standardize the error message format, uni-app defines a more complete error specification:
From 2022-11-11, all new APIs added by DCloud will use this set of uni error specifications. At the same time, we recommend that all plugin authors also use this specification and declare their own plugin id in errSubject.
All asynchronous APIs should return errors through the callback callback, and the error information should be included in the callback function parameters, and the callback function parameters should be of type UniError
The full error types are defined as follows:
// source error message
interface SourceError {
message: string,
subject?: string,
code?: number,
cause?: SourceError | UniAggregateError
}
//Uni聚合源错误信息
interface UniAggregateError extends SourceError {
errors: Array<SourceError|UniAggregateError>
}
//uni error message
interface UniError {
errSubject: string,
errCode: number,
errMsg: string,
data?: Object,
cause?: SourceError | UniAggregateError
}
//Callback
function CallBack(err:UniError){
//console.log(JSON.stringify(res));
}
Used to save the source error that caused the error, such as the error information of the third-party SDK on the app side, including the following attributes:
Notice
The source error can be expanded to other attributes according to the business situation. For example, in uni-AD, slotId can be added to indicate the aggregated three-party advertising slot identifier
用于保存多个源错误,如app端某个错误可能是由多个三方SDK的错误引起,可将多个源错误组成UniAggregateError对象。 Includes the following properties:
Uni unified error information, used to unify the error information of each platform (terminal)
When there are multiple source errors, SourceError needs to be encapsulated into an AggregateError object, and the SourceError array can be obtained as follows:
function CallBack(err:UniError){
var cerrs:SourceError[] = err.cause.errors;
}
The errSubject attribute value indicates the name of the calling module that returned the error.
Module Name | Description |
---|---|
uni-runtime | App side SDK runtime environment error |
uni-ad | uni-AD |
uni-push | UniPush |
uni-login | OAuth (login authentication) |
uni-verify | One-key login |
Notice
在uni-app、uni-app x中的错误信息建议统一使用UniError对象,以便在发生错误时统一捕获处理,特别是以下情况:
在App端,UniError和SourceError都是从uts的Error继承。
UniError对象必须通过 new 操作符构造
语法
new UniError()
new UniError(errSubject:string, errCode:number, errMsg:string)
参数
示例
//创建一个UniError
let error = new UniError("uni-apidName", 60000, "Custom uni error");
//设置data数据(可选)
error.data = {
"dataName": "custom data value"
};
当错误信息是有三方SDK或其它模块引起时,可以将三方SDK或其它模块的错误信息封装在SourceError中作为UniError的源错误
语法
new SourceError()
new SourceError(message:string)
参数
示例
//创建一个SourceError
let sourceError = new SourceError("Third SDK error message");
//创建一个UniError
let error = new UniError("uni-apidName", 60000, "Custom uni error");
//设置源错误
error.cause = sourceError;
当错误是由多个SourceError源错误引起时,可以将多个源错误放到一个UniAggregateError对象中
语法
new UniAggregateError(errors:Array<SourceError>)
参数
示例
//创建UniAggregateError
let aggregateError = new UniAggregateError([new SourceError("First 3rd SDK error message"), new SourceError("Second 3rd SDK error message")]);
//创建一个UniError
let error = new UniError("uni-apidName", 60000, "Custom uni error");
//设置源错误
error.cause = aggregateError;