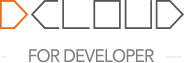
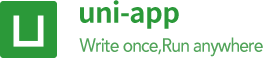
English
iOS has two webviews, UIWebview and WKWebview. Since iOS13, Apple has listed UIWebview as an outdated API.
**App Store will no longer accept new apps using UIWebView from April 2020, and app updates using UIWebView will no longer be accepted from December 2020. **
Since HBuilderX 2.2.5, the default is WKWebview on iOS. Unless the developer manually specifies to use UIWebview in the code, the actual rendered pages are all rendered in WKWebview. However, although the actual page is rendered by WKWebview, there is still an optional reference to UIWebview in the source code of the underlying engine of the App. The Appstore's machine review found that the binary code included a reference to UIWebview, which caused an alert. From HBuilderX 2.6.6, uiWebview is removed from the base engine and becomes an optional module (selected in the manifest). There was no hint from the inspection.
For the old HBuilder and HBuilderX versions before 2.2.5, the App-side strategy is as follows:
**HBuilderX 2.2.5+ version has adjusted the default kernel of all webviews on iOS from UIWebview to WKWebview. **
HBuilderX 2.6.6+ version has removed all UIWebview code in iOS from the basic engine, and is a separate UIWebview module. If you continue to use UIWebview, you need to check the use of UIWebview module in the manifest
hint
Use UIWebview module to submit cloud package to take effect, please use [custom base] when running and debugging on real machine (http://ask.dcloud.net.cn/article/35115) App fails App Store review after using UIWebview module For local offline packaging, please refer to: Configure UIWebview Module
Before HBuilderX 2.2.5, the default webview on iOS is UIWebview, and HBuilderX 2.2.5 and later versions default to WKWebview. If you want to modify the default value, you can configure it in manifest.json. In the manifest.json file source view, set the value of plus -> kernel -> ios to "WKWebview" or "UIWebview":
"plus": {
"kernel": {
"ios": "UIWebview" //或者 "WKWebview"
},
// ...
}
Before HBuilderX 2.2.5, the web-view component in the vue page on iOS or the Webview window created by calling 5+ API defaults to UIWebview, and HBuilderX 2.2.5 and later versions default to WKWebview. If you want to modify the default value, you can configure it in manifest.json. In the manifest.json file source view, set the value of app-plus -> kernel -> ios to "WKWebview" or "UIWebview":
"app-plus": {
"kernel": {
"ios": "UIWebview" //或者 "WKWebview"
},
// ...
}
The web-view component in the nvue page is forced to use WKWebview, which is not configurable
When creating a Webvie window, you can specify the kernel through the kernel property, as follows:
// Specify the kernel of the Webview through the kernel property
var w = plus.webview.create('https://xxx.xxx.xxx', 'id', {
'kernel': 'UIWebview' //或者'WKWebview'
});
For more specifications, refer to [WebviewStyles] of the 5+ API (https://www.html5plus.org/doc/zh_cn/webview.html#plus.webview.WebviewStyles)
Replacing UIWebview with WKWebview will affect the following functionality:
But the advantages of WKWebview are: save memory; lazy-loaded pictures can also be rendered in real time when scrolling, while uiwebview can only display lazy-loaded pictures after scrolling stops.
If you use both ui and wk webviews in an app at the same time, note that cookies, localstorage, and sessions between the two webviews are not shared, but plus.storage is shared.
The js of uni-app runs in independent jscore, not in Webview, so there is no cross-domain problem. The rendering layer of uni-app is mandatory wkwebview under iOS. If you write renderjs code and execute js in the rendering layer, you will also encounter cross-domain problems. At this time, try to put the operations related to cross-domain into the ordinary js logic layer operation. In addition to the rendering layer, there is another issue with web-view components to be aware of:
fail{"statusCode":0,"errMsg":"request:fail abort"}
. However, non-custom components have been discontinued from November 1, 2019.If you need to adjust the rendering kernel settings of the web-view component under uni-app, set the value of app-plus -> kernel -> ios in the manifest.json source view to UIWebview.
The nvue page is not rendered using webview, but the web-view component in it is described below.
At present, UIWebview is still used in the following SDK, whether it is 5+App or uni-app.
**5+App developers recommend to directly upgrade to uni-app, once and for all, there will be no problems such as cross-domain, white screen and inability to encrypt. **
If you are not configured to use the "iOS UIWebview" module and submit to the App Store, you are still prompted to include UIWebview, then please check whether your app uses other native plugins. Generally, it is either a configuration error or a third-party native plug-in. ,