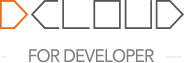
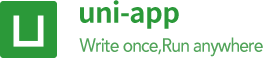
English
HBuilderX from version 2.4.4, uni-app iOS side TabBar supports Gaussian blur effect (frosted glass effect) Since HBuilderX version 3.4.10, the uni-app Android TabBar supports Gaussian blur effect (frosted glass effect)
Here's how to use the Gaussian blur effect, and some precautions.
Effect
Example demo address https://github.com/dcloudio/BlurEffectDemo (click in, remember to star)
The implementation principle is very simple. After enabling the Gaussian blur effect, the page will become taller (increase the height of the tabBar), the page layout will extend below the tabBar, and the framework will automatically add a Gaussian blur effect view on the tabBar, and then look through this view. The following content will see the blur effect.
Notes when using the Gaussian Blur effect:
After enabling the Gaussian blur effect, it is not recommended to set the backgroundColor of the tabBar. If you have to set it, you need to use rgba
to set the transparency, otherwise the blur effect will not be seen;
Since the page height has changed, some precautions should be taken in the page layout (very simple). The following will explain how vue
and nvue
pages are adapted; and a demo will be provided for your reference;
After enabling the Gaussian blur effect, do not dynamically set the hiding of the tabBar, otherwise it will affect the page layout;
In order to facilitate developers to adapt to multiple platforms, the framework has provided a method to obtain the height of the tabBar, and will return different values windowBottom
according to different platforms; the usage method is as follows
// use it directly in css in vue
.fixed1{
position: fixed;
left: 0;
bottom: var(--window-bottom);
}
// nvue does not support css writing, please use js method to get
uni.getSystemInfoSync().windowBottom
First, you need to add the configuration of safearea
under the app-plus
node of manifest.json
, and set the offset
of bottom
to none
, so that the platform will automatically handle the height adaptation of iPhoneX and other full-screen models. Otherwise, the page may be blocked on the full-screen model.
// manifest.json
"app-plus": {
...
"safearea" : {
"bottom" : {
"offset" : "none"
}
}
...
}
Then add the configuration information of tabBar
in pages.json
and configure the value of blurEffect
{
...
...
"tabBar": {
"blurEffect":"extralight",
"color": "#999999",
"borderStyle": "#000000",
// "backgroundColor": "rgba(0,255,51,0.3)",
"spacing": "5px",
"height": "50px",
"selectedColor": "#0062cc",
"list": [
{
"text" : "HELLO",
"iconPath" : "static/ic_tabbar_home_nor.png",
"selectedIconPath" : "static/ic_tabbar_home_sel.png",
"pagePath": "pages/index/index"
},
{
"text" : "WORLD",
"iconPath" : "static/ic_tabbar_group_nor.png",
"selectedIconPath" : "static/ic_tabbar_group_sel.png",
"pagePath": "pages/index/page"
}]
}
}
blurEffect corresponds to the configuration of Gaussian blur, which can take values:
Example (for source code, please refer to index.vue of the example project)
<template>
<view class="content">
<image v-for="index in 3" :key='index' src="../../static/test.png" style="width: 750rpx; height: 1136rpx;" mode="scaleToFill"></image>
<!-- Add a placeholder view at the bottom of the page, the height is equal to the height of the tabBar -->
<view class="edgeInsetBottom"></view>
<!-- Absolutely positioned views need to consider the issue of tabBar occlusion, bottom should add the height of tabBar -->
<view class="fixedView"></view>
</view>
</template>
<script>
export default {
data() {
return {
tabbarHeight:0
}
},
onReady() {
// Get the height of the tabBar
this.tabbarHeight = uni.getSystemInfoSync().windowBottom;
}
}
</script>
<style>
.content {
background-color: #FFFFFF;
line-height: 0;
}
.edgeInsetBottom {
width: 750rpx;
height: var(--window-bottom);
background-color: #FFFFFF;
}
.fixedView{
position: fixed;
width: 750rpx;
height: 30px;
background-color: #4CD964;
bottom: var(--window-bottom);
}
</style>
Because the nvue page is a pure native layout, when the frosted glass effect is enabled, the native frame can automatically adjust the contentInset bottom value of the scroll view, which is equivalent to offsetting the height of the tabbar at the bottom of the page, so that the original elements of the page are not will be occluded, and the position of the scrollbar will be handled automatically. Note: The offset position shows the background color of the scroll view;
Note android does not support adjustBottom temporarily
Example
<template>
<!-- The root node of the page is the scroll view, and add adjustBottom="true" -->
<scroll-view class="content" scroll-y="true" adjustBottom="true">
<image v-for="index in 3" :key='index' src="../../static/test.png" style="width: 750rpx; height: 1136rpx;" mode="scaleToFill"></image>
<!-- Absolutely positioned views need to consider the issue of tabBar occlusion, bottom should add the height of tabBar -->
<view class="fixedView" :style="{ bottom : tabbarHeight + 'px'}"></view>
</scroll-view>
</template>
<script>
export default {
data() {
return {
tabbarHeight: 0
}
},
onReady() {
// Get the height of the tabBar
this.tabbarHeight = uni.getSystemInfoSync().windowBottom;
}
}
</script>
<style>
.content {
background-color: #FFFFFF;
line-height: 0;
}
.fixedView{
position: fixed;
width: 750rpx;
height: 30px;
background-color: #4CD964;
}
</style>
Since HBuilderX version 2.4.4, the uni-app iOS navigationBar supports Gaussian blur effect (frosted glass effect) Since HBuilderX version 3.4.10, the uni-app Android navigationBar supports Gaussian blur effect (frosted glass effect)
Add the blurEffect
attribute to the page style --> app-plus --> titleNView to enable the Gaussian blur effect
Example
{
"pages": [ //pages数组中第一项表示应用启动页,参考:https://uniapp.dcloud.io/collocation/pages
{
"path": "pages/index/index",
"style": {
"navigationBarTitleText": "vue",
"app-plus":{
"bounce":"vertical",
"titleNView": {
"blurEffect":"extralight",
"backgroundColor": "#00ffffff",
"type" : "float"
}
}
}
}
}
Note: backgroundColor needs to be set with a transparent color to see the Gaussian blur effect. You can't see the Gaussian blur effect without setting the backgroundColor!
Since HBuilderX version 2.4.8+, the nvue view component on iOS supports Gaussian blur effect (frosted glass effect) The Android platform is not currently supported
Effect
Add the blurEffect
property to the view component to enable the Gaussian blur effect, the value is the same as the TabBar
Precautions
rgba
to set the transparency, otherwise the blur effect will not be seen;Example
<template>
<view class="container">
<image src="../../static/3.jpg" class="img" mode="aspectFill"></image>
<view class="blur" blurEffect="light">
</view>
</view>
</template>
<script>
export default {
data() {
return {
}
}
}
</script>
<style>
.container{
flex: 1;
}
.img {
flex: 1;
}
.blur{
position: fixed;
top: 300rpx;
bottom: 300rpx;
left: 20px;
right: 20px;
/* background-color: rgba(152,245,255,0.3); */
}
</style>