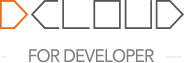
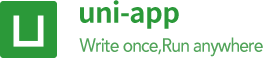
English
The download address of the uni-config-center
plugin: https://ext.dcloud.net.cn/plugin?id=4425
In actual development, many plug-ins/cloud functions/public modules require configuration files to run normally, such as various appkeys and secrets. If it is stored in the database, it will drag down the performance of cloud functions and increase database requests. If each is configured separately, it will produce the following directory structure.
cloudfunctions
├─cf1 // cf1云函数
│ │─index.js
│ └─config.json // cf1使用的配置文件
└─cf2 // cf2云函数
│─index.js
└─config.json // cf2使用的配置文件
It can be seen that there are many problems with this:
uni-config-center
solves the above problem. Through independent public modules and unified management of configuration files, configuration and code are separated, updated code will not overwrite configuration, and configuration can be shared among multiple cloud functions.
The directory structure after using uni-config-center
is as follows
cloudfunctions
├─common
│ ├─uni-config-center
│ │ ├─cf1 // 插件配置目录
│ │ │ └─config.json // 插件配置文件
│ │ └─cf2 // 插件配置目录
│ │ └─config.json // 插件配置文件
├─cf1 // cf1云函数
└─cf2 // cf2云函数
注意
如果你只是使用别人提供的插件要在uni-config-center内放配置文件,则无需关注下面的内容。只要按照插件作者约定的插件配置目录存放你的配置文件即可
For example, to use uni-config-center
inside the cloud function cf2
, the steps are as follows:
uni-config-center
公共模块到项目内,存放路径是 uniCloud/cloudfunctions/common/uni-config-center。一般uniCloud项目创建时会自动导入这个基础插件,如项目没有该插件,请在插件市场下载https://ext.dcloud.net.cn/plugin?id=4425cf2
上右键选择管理公共模块依赖
,弹窗中勾选uni-config-center
公共模块cloudfunctions/common/uni-config-center
目录新建插件配置目录,在本示例中我们使用share-config
作为插件配置目录名(uni-config-center下不同的配置,被称为插件配置。这个插件的概念容易和插件市场的插件混淆,请注意区分)share-config
目录下创建配置文件config.json
(这个文件的名称不能自定义),在config.json
里编写基于json的配置内容。So far, the shared configuration of multi-cloud functions/public modules has been created, and the directory structure is as follows
cloudfunctions
├─common
│ └─uni-config-center
│ └─share-config // 插件配置目录
│ └─config.json // 配置文件
└─cf2 // 引用share-config的云函数
Notice
share-config
in the above example) be as unambiguous as possible. If you only provide a configuration for one cloud function, it is recommended that the name of this configuration be the same as the name of the cloud function. Plug-in authors, in particular, should pay attention to the fact that the name of the plug-in configuration contains the plug-in prefix to prevent conflicts with user code.uni-config-center
of the two projects will overwrite each other when uploading. It is recommended to put the cloud code in the same project, and other projects are associated with the service space of the project where the cloud code is stored.Taking the above directory structure as an example, obtain the configuration content in the share-config
configuration directory in the cloud function cf2
. The sample code is as follows
// cf2/index.js
const createConfig = require('uni-config-center')
const shareConfig = createConfig({ // 获取配置实例
pluginId: 'share-config' // common/uni-config-center下的插件配置目录名
})
const Config = shareConfig.config() // 获取common/uni-config-center/share-config/config.json的内容
exports.main = async function(event, context) {
console.log(Config) //打印配置
}
Notice
uni-config-center
are not isolated. A cloud function or public module, after the introduction of uni-config-center
, all the plugin configurations below can be accesseduni-config-center
is read-only. It won't change unless you re-upload to update the uniCloud service space. If you need to modify the configuration while the code is running, you need to use redis insteadFor detailed interface description, please read the document below.
The configuration instance can be obtained through the createConfig
interface. There are some methods on this instance, such as: obtaining configuration content, splicing directory paths, etc.
Interface form
const createConfig = require('uni-config-center')
createConfig({
pluginId,
defaultConfig,
customMerge
})
Parameter Description
Parameter Name | Type | Required | Description |
---|---|---|---|
pluginId | string | Yes | the name of the configuration directory under common/uni-config-center |
defaultConfig | Object | No | DefaultConfig |
customMerge | Function | No | Customize the merge rule of default configuration and user configuration, if not set, the default configuration and user configuration will be deeply merged |
CODE EXAMPLE
const createConfig = require('uni-config-center')
const shareConfig = createConfig({
pluginId: 'share-config', // 同common/uni-config-center下的配置目录名
defaultConfig: { // 默认配置
tokenExpiresIn: 7200,
tokenExpiresThreshold: 600,
},
customMerge: function(defaultConfig, userConfig) { // 自定义默认配置和用户配置的合并规则,不设置的情况下会对默认配置和用户配置进行深度合并
// defaudltConfig default configuration
// Configuration file content in userConfig config-center
return Object.assign(defaultConfig, userConfig)
}
})
The interface for obtaining configuration content has the following forms. If defaultConfig
is passed in when createConfig is created, it will be merged with config.json in the configuration directory as a complete configuration file.
// get configuration
shareConfig.config() // 获取全部配置,没有获取到配置时返回空对象
shareConfig.config('tokenExpiresIn') // 传入参数名获取指定配置
shareConfig.config('service.sms.codeExpiresIn') // 传入参数路径获取指定配置
shareConfig.config('tokenExpiresThreshold', 600) // 获取指定配置,如果不存在则取传入的默认值
shareConfig.resolve('custom-token.js') // 获取uni-config-center/share-config/custom-token.js文件的路径
shareConfig.requireFile('custom-token.js') // 使用require方式引用uni-config-center/share-config/custom-token.js文件。文件不存在时返回undefined,文件内有其他错误导致require失败时会抛出错误。
shareConfig.hasFile('custom-token.js') // 配置目录是否包含某文件,true: 文件存在,false: 文件不存在