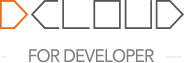
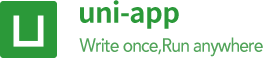
English
Attributes
Platform Difference Description
App | H5 | 微信小程序 | 支付宝小程序 | 百度小程序 | 抖音小程序、飞书小程序 | QQ小程序 | 快手小程序 | 京东小程序 |
---|---|---|---|---|---|---|---|---|
√ | √ | √ | √ | √ | √ | √ | √ | √ |
If you need to use canvas under App-nvue, it has not been packaged as uni API yet, you can refer to documentation to use.
Fill color. The usage is the same as CanvasContext.setFillStyle().
Border color. The usage is the same as CanvasContext.setStrokeStyle().
The shadow's horizontal offset relative to the shape
The vertical offset of the shadow relative to the shape
shade color
Blur level for shadows
The width of the line. The usage is the same as CanvasContext.setLineWidth().
The endpoint style of the line. The usage is the same as CanvasContext.setLineCap().
The intersection style of the line. The usage is the same as CanvasContext.setLineJoin().
Maximum miter length. The usage is the same as CanvasContext.setMiterLimit().
Dashed line offset, the initial value is 0
Properties of the current font style. A DOMString string conforming to CSS font syntax, at least need to provide font size and font family name. The default is 10px sans-serif.
Global brush transparency. The range is 0-1, with 0 being completely transparent and 1 being completely opaque.
The type of compositing operation to apply when drawing new shapes. The current Android version is only applicable to the composition of fill
filling blocks, and the composition effect of stroke
line segments is source-over
.
Currently supported operations are
method
Platform Difference Description
App | H5 | 微信小程序 | 支付宝小程序 | 百度小程序 | 抖音小程序、飞书小程序 | 京东小程序 |
---|---|---|---|---|---|---|
√ | √ | √ | √ | √ | √ | √ |
Draw an arc. To create a circle, you can use the arc()
method to specify the starting arc as 0 and the ending arc as 2 * Math.PI
. Use the stroke()
or fill()
methods to draw arcs in canvas
.
parameter
Parameter | Type | Description |
---|---|---|
x | Number | The x-coordinate of the circle |
y | Number | The y coordinate of the circle |
r | Number | The radius of the circle |
sAngle | Number | Starting radian, unit radian (at 3 o'clock direction) |
eAngle | Number | End Angle |
counterclockwise | Boolean | Optional. Specifies whether the direction of the arc is counterclockwise or clockwise. The default is false, which is clockwise. |
Example Code
const ctx = uni.createCanvasContext('myCanvas')
// Draw coordinates
ctx.arc(100, 75, 50, 0, 2 * Math.PI)
ctx.setFillStyle('#EEEEEE')
ctx.fill()
ctx.beginPath()
ctx.moveTo(40, 75)
ctx.lineTo(160, 75)
ctx.moveTo(100, 15)
ctx.lineTo(100, 135)
ctx.setStrokeStyle('#AAAAAA')
ctx.stroke()
ctx.setFontSize(12)
ctx.setFillStyle('black')
ctx.fillText('0', 165, 78)
ctx.fillText('0.5*PI', 83, 145)
ctx.fillText('1*PI', 15, 78)
ctx.fillText('1.5*PI', 83, 10)
// Draw points
ctx.beginPath()
ctx.arc(100, 75, 2, 0, 2 * Math.PI)
ctx.setFillStyle('lightgreen')
ctx.fill()
ctx.beginPath()
ctx.arc(100, 25, 2, 0, 2 * Math.PI)
ctx.setFillStyle('blue')
ctx.fill()
ctx.beginPath()
ctx.arc(150, 75, 2, 0, 2 * Math.PI)
ctx.setFillStyle('red')
ctx.fill()
// Draw arc
ctx.beginPath()
ctx.arc(100, 75, 50, 0, 1.5 * Math.PI)
ctx.setStrokeStyle('#333333')
ctx.stroke()
ctx.draw()
The three key coordinates for arc(100, 75, 50, 0, 1.5 * Math.PI)
are as follows:
Draws an arc path based on control points and radius.
CanvasContext.arcTo(x1, y1, x2, y2, radius)
parameter
Attribute Value | Type | Description |
---|---|---|
x1 | Number | The x-coordinate of the first control point |
y1 | Number | The y-coordinate of the first control point |
x2 | Number | The x-coordinate of the second control point |
y2 | Number | The y-coordinate of the second control point |
radius | Number | Radius of the arc |
Start to create a path, you need to call fill or stroke to use the path to fill or stroke.
Tip: At the beginning, it is equivalent to calling beginPath()
once.
Tip: For multiple setFillStyle()
, setStrokeStyle()
, setLineWidth()
and other settings in the same path, the last setting shall prevail.
Example Code
const ctx = uni.createCanvasContext('myCanvas')
// begin path
ctx.rect(10, 10, 100, 30)
ctx.setFillStyle('yellow')
ctx.fill()
// begin another path
ctx.beginPath()
ctx.rect(10, 40, 100, 30)
// only fill this rect, not in current path
ctx.setFillStyle('blue')
ctx.fillRect(10, 70, 100, 30)
ctx.rect(10, 100, 100, 30)
// it will fill current path
ctx.setFillStyle('red')
ctx.fill()
ctx.draw()
Creates a cubic Bezier path.
Tip: The starting point of the curve is the previous point in the path.
parameter
Parameter | Type | Description |
---|---|---|
cp1x | Number | The x-coordinate of the first Bézier control point |
cp1y | Number | The y-coordinate of the first Bézier control point |
cp2x | Number | The x-coordinate of the second Bézier control point |
cp2y | Number | The y-coordinate of the second Bezier control point |
x | Number | The x-coordinate of the end point |
y | Number | The y-coordinate of the end point |
Example Code
const ctx = uni.createCanvasContext('myCanvas')
// Draw points
ctx.beginPath()
ctx.arc(20, 20, 2, 0, 2 * Math.PI)
ctx.setFillStyle('red')
ctx.fill()
ctx.beginPath()
ctx.arc(200, 20, 2, 0, 2 * Math.PI)
ctx.setFillStyle('lightgreen')
ctx.fill()
ctx.beginPath()
ctx.arc(20, 100, 2, 0, 2 * Math.PI)
ctx.arc(200, 100, 2, 0, 2 * Math.PI)
ctx.setFillStyle('blue')
ctx.fill()
ctx.setFillStyle('black')
ctx.setFontSize(12)
// Draw guides
ctx.beginPath()
ctx.moveTo(20, 20)
ctx.lineTo(20, 100)
ctx.lineTo(150, 75)
ctx.moveTo(200, 20)
ctx.lineTo(200, 100)
ctx.lineTo(70, 75)
ctx.setStrokeStyle('#AAAAAA')
ctx.stroke()
// Draw quadratic curve
ctx.beginPath()
ctx.moveTo(20, 20)
ctx.bezierCurveTo(20, 100, 200, 100, 200, 20)
ctx.setStrokeStyle('black')
ctx.stroke()
ctx.draw()
The three key coordinates for moveTo(20, 20)
bezierCurveTo(20, 100, 200, 100, 200, 20)
are as follows:
Clears the content within the rectangle on the canvas.
parameter
Parameter | Type | Description |
---|---|---|
x | Number | The x coordinate of the upper left corner of the rectangle |
y | Number | The y coordinate of the upper left corner of the rectangle |
width | Number | the width of the rectangular area |
height | Number | the height of the rectangular area |
Example Code
clearRect does not draw a white rectangle in the address area, but clears it. In order to have an intuitive feeling, a layer of background color is added to the canvas.
<canvas canvas-id="myCanvas" id="myCanvas" style="border: 1px solid; background: #123456;"/>
const ctx = uni.createCanvasContext('myCanvas')
ctx.setFillStyle('red')
ctx.fillRect(0, 0, 150, 200)
ctx.setFillStyle('blue')
ctx.fillRect(150, 0, 150, 200)
ctx.clearRect(10, 10, 150, 75)
ctx.draw()
Cut any shape and size from the original canvas. Once a region is clipped, all subsequent drawing is restricted to the clipped region (no access to other regions on the canvas). The current canvas area can be saved by using the save() method before using the clip() method, and restored at any later time (via the restore() method).
Tip: Use setFillStroke() to set the color of the rectangle line, if not set, the default is black.
Example Code
const context = uni.createCanvasContext('myCanvas')
uni.downloadFile({
url: 'https://qiniu-web-assets.dcloud.net.cn/unidoc/zh/uni@2x.png',
success: function (res) {
context.save()
context.beginPath()
context.arc(96, 96, 48, 0, 2 * Math.PI)
context.clip()
context.drawImage(res.tempFilePath, 48, 48)
context.restore()
context.draw()
}
})
Close a path.
Tip: Closing a path connects the start and end points.
Tip: If you close the path without calling fill()
or stroke()
and open a new path, the previous path will not be rendered.
Example Code
const ctx = uni.createCanvasContext('myCanvas')
ctx.moveTo(10, 10)
ctx.lineTo(100, 10)
ctx.lineTo(100, 100)
ctx.closePath()
ctx.stroke()
ctx.draw()
const ctx = uni.createCanvasContext('myCanvas')
// begin path
ctx.rect(10, 10, 100, 30)
ctx.closePath()
// begin another path
ctx.beginPath()
ctx.rect(10, 40, 100, 30)
// only fill this rect, not in current path
ctx.setFillStyle('blue')
ctx.fillRect(10, 70, 100, 30)
ctx.rect(10, 100, 100, 30)
// it will fill current path
ctx.setFillStyle('red')
ctx.fill()
ctx.draw()
Creates a gradient starting at the center of the circle. The returned CanvasGradient object needs to use CanvasGradient.addColorStop()
to specify gradient points, at least two.
parameter
parameter | type | definition |
---|---|---|
x | Number | The x coordinate of the center of the circle |
y | Number | The y coordinate of the center of the circle |
r | Number | Radius of the circle |
Example Code
const ctx = uni.createCanvasContext('myCanvas')
// Create circular gradient
const grd = ctx.createCircularGradient(75, 50, 50)
grd.addColorStop(0, 'red')
grd.addColorStop(1, 'white')
// Fill with gradient
ctx.setFillStyle(grd)
ctx.fillRect(10, 10, 150, 80)
ctx.draw()
Creates a linear gradient of colors. The returned CanvasGradient object needs to use CanvasGradient.addColorStop()
to specify gradient points, at least two.
parameter
parameter | type | definition |
---|---|---|
x0 | Number | The x-coordinate of the starting point |
y0 | Number | The y-coordinate of the starting point |
x1 | Number | The x-coordinate of the end point |
y1 | Number | The y-coordinate of the end point |
Example Code
const ctx = uni.createCanvasContext('myCanvas')
// Create linear gradient
const grd = ctx.createLinearGradient(0, 0, 200, 0)
grd.addColorStop(0, 'red')
grd.addColorStop(1, 'white')
// Fill with gradient
ctx.setFillStyle(grd)
ctx.fillRect(10, 10, 150, 80)
ctx.draw()
Method for creating a pattern for a specified image that repeats the metaimage in the specified direction
parameter
Parameter | Type | Description |
---|---|---|
image | String | Duplicate image source, only supports in-package path and temporary path |
repetition | String | specifies how to repeat the image, valid values are: repeat, repeat-x, repeat-y, no-repeat |
Example Code
const ctx = uni.createCanvasContext('myCanvas')
const pattern = ctx.createPattern('/path/to/image', 'repeat-x')
ctx.fillStyle = pattern
ctx.fillRect(0, 0, 300, 150)
ctx.draw()
Draws the description (path, transform, style) previously in the drawing context to the canvas.
parameter
Parameter | Type | Description | Minimum Version |
---|---|---|---|
reserve | Boolean | Optional. Whether this drawing follows the previous drawing, that is, the reserve parameter is false, and the native layer should clear the canvas before drawing this time before calling drawCanvas; if the reserve parameter is true, the content on the current canvas is reserved, and this call The content drawn by drawCanvas is overlaid on it, the default is false | |
callback | Function | callback after drawing is complete | 1.7.0 |
Example Code
const ctx = uni.createCanvasContext('myCanvas')
ctx.setFillStyle('red')
ctx.fillRect(10, 10, 150, 100)
ctx.draw()
ctx.fillRect(50, 50, 150, 100)
ctx.draw()
const ctx = uni.createCanvasContext('myCanvas')
ctx.setFillStyle('red')
ctx.fillRect(10, 10, 150, 100)
ctx.draw()
ctx.fillRect(50, 50, 150, 100)
ctx.draw(true)
Draw the image to the canvas.
parameter
Parameter | Type | Description |
---|---|---|
imageResource | String | The image resource to be drawn |
dx | Number | The X-axis position of the upper left corner of the image on the target canvas |
dy | Number | The Y-axis position of the upper left corner of the image on the target canvas |
dWidth | Number | The width of the image drawn on the target canvas, allowing scaling of the drawn image |
dHeight | Number | The height of the image drawn on the target canvas, allowing scaling of the drawn image |
sx | Number | The X coordinate of the upper left corner of the selection rectangle of the source image |
sy | Number | The Y coordinate of the upper left corner of the selection rectangle of the source image |
sWidth | Number | The width of the selection rectangle of the source image |
sHeight | Number | The height of the selection rectangle of the source image |
There are three versions of writing:
drawImage(dx, dy)
drawImage(dx, dy, dWidth, dHeight)
drawImage(sx, sy, sWidth, sHeight, dx, dy, dWidth, dHeight)
Example Code
const ctx = uni.createCanvasContext('myCanvas')
uni.chooseImage({
success: function(res){
ctx.drawImage(res.tempFilePaths[0], 0, 0, 150, 100)
ctx.draw()
}
})
Fill in the content of the current path. The default fill color is black.
Tip: If the current path is not closed, the fill()
method will connect the starting point and the ending point, and then fill it. See example 1 for details.
Tip: fill()
fills the path from beginPath()
, but does not include fillRect()
, details See example two.
Example Code
const ctx = uni.createCanvasContext('myCanvas')
ctx.moveTo(10, 10)
ctx.lineTo(100, 10)
ctx.lineTo(100, 100)
ctx.fill()
ctx.draw()
const ctx = uni.createCanvasContext('myCanvas')
// begin path
ctx.rect(10, 10, 100, 30)
ctx.setFillStyle('yellow')
ctx.fill()
// begin another path
ctx.beginPath()
ctx.rect(10, 40, 100, 30)
// only fill this rect, not in current path
ctx.setFillStyle('blue')
ctx.fillRect(10, 70, 100, 30)
ctx.rect(10, 100, 100, 30)
// it will fill current path
ctx.setFillStyle('red')
ctx.fill()
ctx.draw()
Fills a rectangle.
Tip: Use setFillStyle()
to set the fill color of the rectangle, if not set, the default is black.
parameter
parameter | type | definition |
---|---|---|
x | Number | The x-coordinate of the upper left corner of the rectangle path |
y | Number | The y coordinate of the upper left corner of the rectangle path |
width | Number | Width of the rectangular path |
height | Number | the height of the rectangle path |
Example Code
const ctx = uni.createCanvasContext('myCanvas')
ctx.setFillStyle('red')
ctx.fillRect(10, 10, 150, 75)
ctx.draw()
Draw the filled text on the canvas.
parameter
Parameter | Type | Description |
---|---|---|
text | String | The text to output on the canvas |
x | Number | The x coordinate position of the upper left corner of the drawn text |
y | Number | The y coordinate position of the upper left corner of the drawn text |
maxWidth | Number | The maximum width to be drawn, optional |
Example Code
const ctx = uni.createCanvasContext('myCanvas')
ctx.setFontSize(20)
ctx.fillText('Hello', 20, 20)
ctx.fillText('MINA', 100, 100)
ctx.draw()
Adds a new point, then creates a line from the last specified point to the target point.
Tip: Use the stroke()
method to draw lines
parameter
parameter | type | description |
---|---|---|
x | Number | The x-coordinate of the target location |
y | Number | The y-coordinate of the target location |
Example Code
const ctx = uni.createCanvasContext('myCanvas')
ctx.moveTo(10, 10)
ctx.rect(10, 10, 100, 50)
ctx.lineTo(110, 60)
ctx.stroke()
ctx.draw()
Measure text size information, currently only returns text width. synchronous interface. (App 2.8.12+ support)
parameter
Parameter | Type | Description |
---|---|---|
text | String | Text to measure |
return
Return TextMetrics
object, the structure is as follows:
Parameter | Type | Description |
---|---|---|
width | Number | the width of the text |
Example Code
const ctx = uni.createCanvasContext('myCanvas')
ctx.font = 'italic bold 20px cursive'
const metrics = ctx.measureText('Hello World')
console.log(metrics.width)
Moves the path to the specified point in the canvas without creating a line. Use the stroke()
method to draw lines.
parameter
parameter | type | description |
---|---|---|
x | Number | The x-coordinate of the target location |
y | Number | The y-coordinate of the target location |
Example Code
const ctx = uni.createCanvasContext('myCanvas')
ctx.moveTo(10, 10)
ctx.lineTo(100, 10)
ctx.moveTo(10, 50)
ctx.lineTo(100, 50)
ctx.stroke()
ctx.draw()
Creates a quadratic Bézier path. The starting point of the curve is the previous point in the path.
parameter
Parameter | Type | Description |
---|---|---|
cpx | Number | x-coordinate of the Bezier control point |
cpy | Number | The y-coordinate of the Bezier control point |
x | Number | The x-coordinate of the end point |
y | Number | The y coordinate of the end point |
Example Code
const ctx = uni.createCanvasContext('myCanvas')
// Draw points
ctx.beginPath()
ctx.arc(20, 20, 2, 0, 2 * Math.PI)
ctx.setFillStyle('red')
ctx.fill()
ctx.beginPath()
ctx.arc(200, 20, 2, 0, 2 * Math.PI)
ctx.setFillStyle('lightgreen')
ctx.fill()
ctx.beginPath()
ctx.arc(20, 100, 2, 0, 2 * Math.PI)
ctx.setFillStyle('blue')
ctx.fill()
ctx.setFillStyle('black')
ctx.setFontSize(12)
// Draw guides
ctx.beginPath()
ctx.moveTo(20, 20)
ctx.lineTo(20, 100)
ctx.lineTo(200, 20)
ctx.setStrokeStyle('#AAAAAA')
ctx.stroke()
// Draw quadratic curve
ctx.beginPath()
ctx.moveTo(20, 20)
ctx.quadraticCurveTo(20, 100, 200, 20)
ctx.setStrokeStyle('black')
ctx.stroke()
ctx.draw()
The three key coordinates for moveTo(20, 20)
quadraticCurveTo(20, 100, 200, 20)
are as follows:
Create a rectangle.
Tip: Use the fill() or stroke() method to actually draw the rectangle into the canvas.
parameter
Parameter | Type | Description |
---|---|---|
x | Number | The x-coordinate of the upper left corner of the rectangle path |
y | Number | The y coordinate of the upper left corner of the rectangle path |
width | Number | Width of the rectangular path |
height | Number | the height of the rectangle path |
Example Code
const ctx = uni.createCanvasContext('myCanvas')
ctx.rect(10, 10, 150, 75)
ctx.setFillStyle('red')
ctx.fill()
ctx.draw()
Restores a previously saved drawing context.
Example Code
const ctx = uni.createCanvasContext('myCanvas')
// save the default fill style
ctx.save()
ctx.setFillStyle('red')
ctx.fillRect(10, 10, 150, 100)
// restore to the previous saved state
ctx.restore()
ctx.fillRect(50, 50, 150, 100)
ctx.draw()
Centered on the origin, the origin can be modified with the translate method. Rotate the current axis clockwise. Call rotate multiple times, the angle of rotation will be superimposed.
parameter
Parameter | Type | Description |
---|---|---|
rotate | Number | Rotation angle, in radians (degrees * Math.PI/180; the range of degrees is 0~360) |
Example Code
const ctx = uni.createCanvasContext('myCanvas')
ctx.strokeRect(100, 10, 150, 100)
ctx.rotate(20 * Math.PI / 180)
ctx.strokeRect(100, 10, 150, 100)
ctx.rotate(20 * Math.PI / 180)
ctx.strokeRect(100, 10, 150, 100)
ctx.draw()
Save the current drawing context.
Example Code
const ctx = wx.createCanvasContext('myCanvas')
// save the default fill style
ctx.save()
ctx.setFillStyle('red')
ctx.fillRect(10, 10, 150, 100)
// restore to the previous saved state
ctx.restore()
ctx.fillRect(50, 50, 150, 100)
ctx.draw()
After calling the scale
method, the horizontal and vertical coordinates of the created path will be scaled. Calling scale
multiple times, the multiples will be multiplied.
parameter
Parameter | Type | Description |
---|---|---|
scaleWidth | Number | Multiplier of abscissa scaling (1 = 100%, 0.5 = 50%, 2 = 200%) |
scaleHeight | Number | Multiplier of vertical axis scaling (1 = 100%, 0.5 = 50%, 2 = 200%) |
Example Code
const ctx = uni.createCanvasContext('myCanvas')
ctx.strokeRect(10, 10, 25, 15)
ctx.scale(2, 2)
ctx.strokeRect(10, 10, 25, 15)
ctx.scale(2, 2)
ctx.strokeRect(10, 10, 25, 15)
ctx.draw()
Set the fill color, if no fillStyle is set, the default color is black.
grammar
canvasContext.setFillStyle(color)
canvasContext.fillStyle = color
parameter
parameter | type | definition | description |
---|---|---|---|
color | Color | Gradient Object | fill color |
Example Code
const ctx = uni.createCanvasContext('myCanvas')
ctx.setFillStyle('red')
ctx.fillRect(10, 10, 150, 75)
ctx.draw()
Sets the size of the font.
Parameter | Type | Description |
---|---|---|
fontSize | Number | Font size |
Example Code
const ctx = uni.createCanvasContext('myCanvas')
ctx.setFontSize(20)
ctx.fillText('20', 20, 20)
ctx.setFontSize(30)
ctx.fillText('30', 40, 40)
ctx.setFontSize(40)
ctx.fillText('40', 60, 60)
ctx.setFontSize(50)
ctx.fillText('50', 90, 90)
ctx.draw()
Sets the global brush transparency.
parameter
parameter | type | range | description |
---|---|---|---|
alpha | Number | 0~1 | Transparency, 0 means completely transparent, 1 means completely opaque |
Example Code
const ctx = uni.createCanvasContext('myCanvas')
ctx.setFillStyle('red')
ctx.fillRect(10, 10, 150, 100)
ctx.setGlobalAlpha(0.2)
ctx.setFillStyle('blue')
ctx.fillRect(50, 50, 150, 100)
ctx.setFillStyle('yellow')
ctx.fillRect(100, 100, 150, 100)
ctx.draw()
Sets the endpoint style of the line.
parameter
Parameter | Type | Description | |
---|---|---|---|
lineCap | String | 'butt', 'round', 'square' | End cap style of the line |
Example Code
const ctx = uni.createCanvasContext('myCanvas')
ctx.beginPath()
ctx.moveTo(10, 10)
ctx.lineTo(150, 10)
ctx.stroke()
ctx.beginPath()
ctx.setLineCap('butt')
ctx.setLineWidth(10)
ctx.moveTo(10, 30)
ctx.lineTo(150, 30)
ctx.stroke()
ctx.beginPath()
ctx.setLineCap('round')
ctx.setLineWidth(10)
ctx.moveTo(10, 50)
ctx.lineTo(150, 50)
ctx.stroke()
ctx.beginPath()
ctx.setLineCap('square')
ctx.setLineWidth(10)
ctx.moveTo(10, 70)
ctx.lineTo(150, 70)
ctx.stroke()
ctx.draw()
Sets the line width.
parameter
parameter | type | definition |
---|---|---|
pattern | Array | An array of numbers describing the lengths of alternately drawn line segments and spacing (coordinate space units) |
offset | Number | Dashed line offset |
Example Code
const ctx = uni.createCanvasContext('myCanvas')
ctx.setLineDash([10, 20], 5);
ctx.beginPath();
ctx.moveTo(0,100);
ctx.lineTo(400, 100);
ctx.stroke();
ctx.draw()
Sets the intersection style for lines.
parameter
parameter | type | range | description |
---|---|---|---|
lineJoin | String | 'bevel', 'round', 'miter' | End intersection style for lines |
Example Code
const ctx = uni.createCanvasContext('myCanvas')
ctx.beginPath()
ctx.moveTo(10, 10)
ctx.lineTo(100, 50)
ctx.lineTo(10, 90)
ctx.stroke()
ctx.beginPath()
ctx.setLineJoin('bevel')
ctx.setLineWidth(10)
ctx.moveTo(50, 10)
ctx.lineTo(140, 50)
ctx.lineTo(50, 90)
ctx.stroke()
ctx.beginPath()
ctx.setLineJoin('round')
ctx.setLineWidth(10)
ctx.moveTo(90, 10)
ctx.lineTo(180, 50)
ctx.lineTo(90, 90)
ctx.stroke()
ctx.beginPath()
ctx.setLineJoin('miter')
ctx.setLineWidth(10)
ctx.moveTo(130, 10)
ctx.lineTo(220, 50)
ctx.lineTo(130, 90)
ctx.stroke()
ctx.draw()
Sets the width of the line.
parameter
Parameter | Type | Description |
---|---|---|
lineWidth | Number | The width of the line (unit is px) |
Example Code
const ctx = uni.createCanvasContext('myCanvas')
ctx.beginPath()
ctx.moveTo(10, 10)
ctx.lineTo(150, 10)
ctx.stroke()
ctx.beginPath()
ctx.setLineWidth(5)
ctx.moveTo(10, 30)
ctx.lineTo(150, 30)
ctx.stroke()
ctx.beginPath()
ctx.setLineWidth(10)
ctx.moveTo(10, 50)
ctx.lineTo(150, 50)
ctx.stroke()
ctx.beginPath()
ctx.setLineWidth(15)
ctx.moveTo(10, 70)
ctx.lineTo(150, 70)
ctx.stroke()
ctx.draw()
Sets the maximum miter length, which is the distance between the inner and outer corners where two lines meet. Only valid when setLineJoin()
is miter. If it exceeds the maximum slant length, the join will be displayed with lineJoin as bevel.
parameter
Parameter | Type | Description |
---|---|---|
miterLimit | Number | Maximum miter length |
Example Code
const ctx = uni.createCanvasContext('myCanvas')
ctx.beginPath()
ctx.setLineWidth(10)
ctx.setLineJoin('miter')
ctx.setMiterLimit(1)
ctx.moveTo(10, 10)
ctx.lineTo(100, 50)
ctx.lineTo(10, 90)
ctx.stroke()
ctx.beginPath()
ctx.setLineWidth(10)
ctx.setLineJoin('miter')
ctx.setMiterLimit(2)
ctx.moveTo(50, 10)
ctx.lineTo(140, 50)
ctx.lineTo(50, 90)
ctx.stroke()
ctx.beginPath()
ctx.setLineWidth(10)
ctx.setLineJoin('miter')
ctx.setMiterLimit(3)
ctx.moveTo(90, 10)
ctx.lineTo(180, 50)
ctx.lineTo(90, 90)
ctx.stroke()
ctx.beginPath()
ctx.setLineWidth(10)
ctx.setLineJoin('miter')
ctx.setMiterLimit(4)
ctx.moveTo(130, 10)
ctx.lineTo(220, 50)
ctx.lineTo(130, 90)
ctx.stroke()
ctx.draw()
Sets the shadow style. If not set, the default value of offsetX is 0, the default value of offsetY is 0, the default value of blur is 0, and the default value of color is black.
parameter
Parameter | Type | Definition | Description |
---|---|---|---|
offsetX | Number | The horizontal offset of the shadow relative to the shape | |
offsetY | Number | The vertical offset of the shadow relative to the shape | |
blur | Number | 0~100 | Blur level of the shadow, the larger the value, the more blurred |
color | Color | the color of the shadow |
Example Code
const ctx = uni.createCanvasContext('myCanvas')
ctx.setFillStyle('red')
ctx.setShadow(10, 50, 50, 'blue')
ctx.fillRect(10, 10, 150, 75)
ctx.draw()
Set border color. If fillStyle is not set, the default color is black.
parameter
Parameter | Type | Definition | Description |
---|---|---|---|
color | Color | Gradient Object | fill color |
Example Code
const ctx = uni.createCanvasContext('myCanvas')
ctx.setStrokeStyle('red')
ctx.strokeRect(10, 10, 150, 75)
ctx.draw()
Used to set the alignment of text
parameter | type | definition |
---|---|---|
align | String | Optional values 'left', 'center', 'right' |
Example Code
const ctx = uni.createCanvasContext('myCanvas')
ctx.setStrokeStyle('red')
ctx.moveTo(150, 20)
ctx.lineTo(150, 170)
ctx.stroke()
ctx.setFontSize(15)
ctx.setTextAlign('left')
ctx.fillText('textAlign=left', 150, 60)
ctx.setTextAlign('center')
ctx.fillText('textAlign=center', 150, 80)
ctx.setTextAlign('right')
ctx.fillText('textAlign=right', 150, 100)
ctx.draw()
Used to set the horizontal alignment of the text
parameter
parameter | type | description |
---|---|---|
textBaseline | String | Optional values 'top', 'bottom', 'middle', 'normal' |
Example Code
const ctx = uni.createCanvasContext('myCanvas')
ctx.setStrokeStyle('red')
ctx.moveTo(5, 75)
ctx.lineTo(295, 75)
ctx.stroke()
ctx.setFontSize(20)
ctx.setTextBaseline('top')
ctx.fillText('top', 5, 75)
ctx.setTextBaseline('middle')
ctx.fillText('middle', 50, 75)
ctx.setTextBaseline('bottom')
ctx.fillText('bottom', 120, 75)
ctx.setTextBaseline('normal')
ctx.fillText('normal', 200, 75)
ctx.draw()
Method to reset (overwrite) the current transform using a matrix
grammar
canvasContext.setTransform(scaleX, skewX, skewY, scaleY, translateX, translateY)
parameter
Parameter | Type | Description |
---|---|---|
scaleX | Number | Horizontal scaling |
skewX | Number | Horizontal skew |
skewY | Number | vertical skew |
scaleY | Number | Vertical scaling |
translateX | Number | Horizontal translation |
translateY | Number | vertical translation |
Draws a border around the current path. The default color is black.
Tip: The path drawn by stroke()
is calculated from beginPath()
, but will not include strokeRect()
, see the example for details two.
Example Code
const ctx = uni.createCanvasContext('myCanvas')
ctx.moveTo(10, 10)
ctx.lineTo(100, 10)
ctx.lineTo(100, 100)
ctx.stroke()
ctx.draw()
const ctx = uni.createCanvasContext('myCanvas')
// begin path
ctx.rect(10, 10, 100, 30)
ctx.setStrokeStyle('yellow')
ctx.stroke()
// begin another path
ctx.beginPath()
ctx.rect(10, 40, 100, 30)
// only stoke this rect, not in current path
ctx.setStrokeStyle('blue')
ctx.strokeRect(10, 70, 100, 30)
ctx.rect(10, 100, 100, 30)
// it will stroke current path
ctx.setStrokeStyle('red')
ctx.stroke()
ctx.draw()
Draw a rectangle (not filled). Use setFillStroke()
to set the border color, if not set, the default is black.
parameter
parameter | type | range | description |
---|---|---|---|
x | Number | The x-coordinate of the upper left corner of the rectangle path | |
y | Number | The y coordinate of the upper left corner of the rectangle path | |
width | Number | Width of the rectangular path | |
height | Number | height of the rectangle path |
Example Code
const ctx = uni.createCanvasContext('myCanvas')
ctx.setStrokeStyle('red')
ctx.strokeRect(10, 10, 150, 75)
ctx.draw()
Method to draw a text stroke at a given (x, y) position
grammar
canvasContext.strokeText(text, x, y, maxWidth)
parameter
Parameter | Type | Description |
---|---|---|
text | String | The text to draw |
x | Number | The x-axis coordinate of the starting point of the text |
y | Number | The y-axis coordinate of the starting point of the text |
maxWidth | Number | The maximum width to be drawn, optional |
Method to overlay the current transform multiple times using a matrix.
parameter
Parameter | Type | Description |
---|---|---|
scaleX | Number | Horizontal scaling |
skewX | Number | Horizontal skew |
skewY | Number | vertical skew |
scaleY | Number | Vertical scaling |
translateX | Number | Horizontal translation |
translateY | Number | vertical translation |
Transform the origin (0, 0) of the current coordinate system. The default coordinate system origin is the upper left corner of the page.
parameter
Parameter | Type | Description |
---|---|---|
x | Number | Horizontal coordinate translation |
y | Number | Vertical coordinate translation |
Example Code
const ctx = uni.createCanvasContext('myCanvas')
ctx.strokeRect(10, 10, 150, 100)
ctx.translate(20, 20)
ctx.strokeRect(10, 10, 150, 100)
ctx.translate(20, 20)
ctx.strokeRect(10, 10, 150, 100)
ctx.draw()