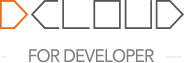
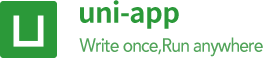
English
Displays the message prompt box.
OBJECT parameter description
Parameter | Type | Required | Instruction | Platform difference description |
---|---|---|---|---|
title | String | 是 | 提示的内容,长度与 icon 取值有关。 | |
icon | String | 否 | 图标,有效值详见下方说明,默认:success。 | |
image | String | 否 | 自定义图标的本地路径(app端暂不支持gif) | App、H5、微信小程序、百度小程序、抖音小程序(2.62.0+) |
mask | Boolean | 否 | 是否显示透明蒙层,防止触摸穿透,默认:false | App、微信小程序、抖音小程序(2.47.0+) |
duration | Number | No | Prompt delay time, in milliseconds, default: 1500 | |
position | String | No | The display position of the plain text light prompt. Only the title attribute takes effect after filling in a valid value, and does not support hiding through uni.hideToast. See below for valid values. | App |
success | Function | No | Callback function for successful interface calling | |
fail | Function | No | Callback function for failed interface calling | |
complete | Function | No | Callback function for closed interface calling (available both for successful and failed calling) |
icon value description
Value | Instruction | Platform difference description |
---|---|---|
success | 显示成功图标,此时 title 文本在小程序 平台最多显示 7 个汉字长度,App 仅支持单行显示。 | 支付宝小程序无长度无限制 |
error | 显示错误图标,此时 title 文本在小程序 平台最多显示 7 个汉字长度,App 仅支持单行显示。 | 支付宝小程序、快手小程序、抖音小程序、百度小程序、京东小程序、QQ小程序不支持 |
fail | 显示错误图标,此时 title 文本无长度显示。 | 支付宝小程序、抖音小程序 |
exception | Display the exception icon. The title text is now displayed without length. | Alipay Mini Program |
loading | 显示加载图标,此时 title 文本在小程序 平台最多显示 7 个汉字长度。 | 支付宝小程序不支持 |
none | 不显示图标,此时 title 文本在小程序 最多可显示两行。 |
Example
uni.showToast({
title: '标题',
duration: 2000
});
Description of position value (valid only in App)
Value | Instruction |
---|---|
top | Display at top |
center | Display at center |
bottom | Display at bottom |
Tips
Hide the message prompt box.
Example
uni.hideToast();
显示 loading 提示框, 需主动调用 uni.hideLoading 才能关闭提示框。
OBJECT parameter description
Parameter | Type | Required | Instruction | Platform difference description |
---|---|---|---|---|
title | String | 是 | 提示的文字内容,显示在loading的下方 | |
mask | Boolean | 否 | 是否显示透明蒙层,防止触摸穿透,默认:false | H5、App、微信小程序、百度小程序、抖音小程序(2.47.0+) |
success | Function | No | Callback function for successful interface calling | |
fail | Function | No | Callback function for failed interface calling | |
complete | Function | No | Callback function for closed interface calling (available both for successful and failed calling) |
Example
uni.showLoading({
title: '加载中'
});
Hide the loading prompt box.
Example
uni.showLoading({
title: '加载中'
});
setTimeout(function () {
uni.hideLoading();
}, 2000);
Display the modal pop-up window with only one OK button or both OK and Cancel buttons. Similar to an API integrating alert and confirm in html.
OBJECT parameter description
Parameter | Type | Required | Instruction | Platform difference description |
---|---|---|---|---|
title | String | No | Prompt title | |
content | String | No | Prompt content | |
showCancel | Boolean | No | Whether to display the Cancel button, with true as default | |
cancelText | String | 否 | 取消按钮的文字,默认为"取消" | |
cancelColor | HexColor | 否 | 取消按钮的文字颜色,默认为"#000000" | H5、微信小程序、百度小程序、抖音小程序(2.62.0+) |
confirmText | String | 否 | 确定按钮的文字,默认为"确定" | |
confirmColor | HexColor | 否 | 确定按钮的文字颜色,H5平台默认为"#007aff",微信小程序平台默认为"#576B95",百度小程序平台默认为"#3c76ff" | H5、微信小程序、百度小程序、抖音小程序(2.62.0+) |
editable | Boolean | 否 | 是否显示输入框 | H5 (3.2.10+)、App (3.2.10+)、微信小程序 (2.17.1+)、抖音小程序(2.62.0+) |
placeholderText | String | 否 | 显示输入框时的提示文本 | H5 (3.2.10+)、App (3.2.10+)、微信小程序 (2.17.1+)、抖音小程序(2.62.0+) |
success | Function | No | Callback function for successful interface calling | |
fail | Function | No | Callback function for failed interface calling | |
complete | Function | No | Callback function for closed interface calling (available both for successful and failed calling) |
Success return parameter description
Parameter | Type | Description | Platform Difference Description |
---|---|---|---|
confirm | Boolean | When is true, it means that the user has clicked the OK button | |
cancel | Boolean | 为 true 时,表示用户点击了取消(用于 Android 系统区分点击蒙层关闭还是点击取消按钮关闭) | |
content | String | editable 为 true 时,用户输入的文本 | H5 (3.2.10+)、App (3.2.10+)、微信小程序 (2.17.1+)、抖音小程序(2.62.0+) |
Example
uni.showModal({
title: '提示',
content: '这是一个模态弹窗',
success: function (res) {
if (res.confirm) {
console.log('用户点击确定');
} else if (res.cancel) {
console.log('用户点击取消');
}
}
});
Notice
cancelText
and confirmText
have a length limit, up to 4 characters;confirm
and cancel
fields, including two cases: { confirm: true, cancel: false }
or { confirm: true }
, but not Affect the use of if to make judgmentsPop up the operation menu from the bottom up
OBJECT parameter description
Parameter | Type | Required | Instruction | Platform difference description |
---|---|---|---|---|
title | String | No | Menu title | App, H5, Alipay applet, DingTalk applet, WeChat applet 3.4.5+ (only valid for real devices) |
alertText | String | 否 | 警示文案(同菜单标题) | 微信小程序(仅真机有效) |
itemList | Array<String> | 是 | 按钮的文字数组 | 微信、百度、抖音小程序数组长度最大为6个 |
itemColor | HexColor | 否 | 按钮的文字颜色,字符串格式,默认为"#000000" | App-iOS、飞书小程序不支持 |
popover | Object | No | On large-screen devices, the display area of the native selection button box is popped up and displayed in the center by default | App-iPad(2.6.6+), H5(2.9.2) |
success | Function | No | Callback function for successful interface calling. See the notices on returning parameter description. | |
fail | Function | No | Callback function for failed interface calling | |
complete | Function | No | Callback function for closed interface calling (available both for successful and failed calling) |
popover value description (only valid for App)
Value | Type | Instruction |
---|---|---|
top | Number | Coordinates of the indication area. When using the native navigationBar, it is generally necessary to add the height of the navigationBar. |
left | Number | Indicate area coordinates |
width | Number | Indicate area width |
height | Number | Indicate area height |
Success return parameter description
Parameter | Type | Instruction |
---|---|---|
tapIndex | Number | The buttons clickable by user, from top to bottom, starting from 0 |
Example
uni.showActionSheet({
itemList: ['A', 'B', 'C'],
success: function (res) {
console.log('选中了第' + (res.tapIndex + 1) + '个按钮');
},
fail: function (res) {
console.log(res.errMsg);
}
});
Tips
Notice