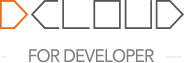
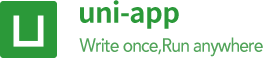
English
Dynamically set the content of a tabBar item
Platform Difference Description
App | H5 | 微信小程序 | 支付宝小程序 | 百度小程序 | 抖音小程序、飞书小程序 | QQ小程序 | 快手小程序 | 京东小程序 |
---|---|---|---|---|---|---|---|---|
√ | √ | √ | √ (DingTalk MiniApp not supported) | √ | √ | √ | √ | √ |
OBJECT parameter description:
Attribute | Type | Default | Required | Description | Platform Difference |
---|---|---|---|---|---|
index | number | which item of | tabBar, counting from the left | ||
text | String | No | button text on the tab | ||
iconPath | String | No | Image path, the icon size is limited to 40kb, and the recommended size is 81px * 81px. When the position is top, this parameter is invalid. WeChat MiniApp 2.7.0+, Alipay MiniApp support network pictures, other platforms do not support network pictures | ||
selectedIconPath | String | No | The image path when selected, the icon size is limited to 40kb, the recommended size is 81px * 81px, when the position is top, this parameter is invalid | ||
pagePath | String | No | The absolute path of the page must be defined first in pages, the replaced pagePath will not become a normal page (you still need to use uni.switchTab to jump Transfer) | App (2.8.4+), H5 (2.8.4+) | |
visible | Boolean | true | No | Whether the item is displayed | App (3.2.10+), H5 (3.2.10+) |
iconfont | Object | No | Font icon, priority is higher than iconPath | App (3.4.4+) | |
success | Function | No | Callback function for successful interface call | ||
fail | Function | No | Callback function for interface call failure | ||
complete | Function | No | The callback function of the end of the interface call (it will be executed when the call succeeds or fails) |
iconfont parameter description:
Attribute | Type | Description |
---|---|---|
text | String | Font Unicode code |
selectedText | String | Unicode code of selected font |
fontSize | String | Font icon font size (px) |
color | String | font icon color |
selectedColor | String | font icon selected color |
Example Code
uni.setTabBarItem({
index: 0,
text: 'text',
iconPath: '/path/to/iconPath',
selectedIconPath: '/path/to/selectedIconPath'
})
Note: When setting the iconfont
property, the pages.json iconfontSrc
needs to specify the font file, refer to the configuration below
// pages.json
{
"tabBar": {
"iconfontSrc":"static/iconfont.ttf",
"list": [
{
"pagePath": "pages/index/index",
"text": "Tab1",
"iconfont": {
"text": "\ue102",
"selectedText": "\ue103",
"fontSize": "17px",
"color": "#000000",
"selectedColor": "#0000ff"
}
}
]
}
}
Dynamically set the overall style of tabBar
Platform Difference Description
App | H5 | 微信小程序 | 支付宝小程序 | 百度小程序 | 抖音小程序、飞书小程序 | QQ小程序 | 快手小程序 | 京东小程序 |
---|---|---|---|---|---|---|---|---|
√ | √ | √ | √ | √ | √ | √ | √ | √ |
OBJECT parameter description:
Attribute | Type | Default | Required | Description |
---|---|---|---|---|
color | String | No | The default color of the text on the tab, HexColor | |
selectedColor | String | No | The color of the selected text on the tab, HexColor | |
backgroundColor | String | No | background color of tab, HexColor | |
backgroundImage | String | No | The image background. Support setting local images or creating linear gradients such as, priority is higher than backgroundColor, only supported by App 2.7.1+ | |
backgroundRepeat | String | 否 | 背景图平铺方式。repeat:背景图片在垂直方向和水平方向平铺;repeat-x:背景图片在水平方向平铺,垂直方向拉伸;repeat-y:背景图片在垂直方向平铺,水平方向拉伸;no-repeat:背景图片在垂直方向和水平方向都拉伸。 默认使用 no-repeat。仅 App 2.7.1+ 支持 | |
borderStyle | String | 否 | tabBar上边框的颜色, 仅支持 black/white,black对应颜色rgba(0,0,0,0.33),white对应颜色rgba(255,255,255,0.33)。 | |
midButton | Object | No | The middle button is only valid when the list item is even Details. HBuilderX 3.6.9+ | |
success | Function | No | Callback function for successful interface call | |
fail | Function | No | Callback function for interface call failure | |
complete | Function | No | The callback function of the end of the interface call (it will be executed when the call succeeds or fails) |
backgroundImage creates a linear gradient description
backgroundImage: linear-gradient(to top, #a80077, #66ff00);
Radial-gradient is currently not supported.
Currently only supports gradients of two colors, and the gradient direction is as follows:
Example Code
uni.setTabBarStyle({
color: '#FF0000',
selectedColor: '#00FF00',
backgroundColor: '#0000FF',
borderStyle: 'white'
})
hide tabBar
Platform Difference Description
App | H5 | 微信小程序 | 支付宝小程序 | 百度小程序 | 抖音小程序、飞书小程序 | QQ小程序 | 快手小程序 | 京东小程序 |
---|---|---|---|---|---|---|---|---|
√ | √ | √ | √ | √ | √ | √ | √ | √ |
OBJECT parameter description:
Attribute | Type | Default | Required | Description |
---|---|---|---|---|
animation | boolean | false | 否 | 是否需要动画效果,仅微信小程序、支付宝小程序、百度小程序、抖音小程序、飞书小程序、QQ小程序、快手小程序、京东小程序支持 |
success | Function | No | Callback function for successful interface call | |
fail | Function | No | Callback function for interface call failure | |
complete | Function | No | The callback function of the end of the interface call (it will be executed when the call succeeds or fails) |
show tabBar
Platform Difference Description
App | H5 | 微信小程序 | 支付宝小程序 | 百度小程序 | 抖音小程序、飞书小程序 | QQ小程序 | 快手小程序 | 京东小程序 |
---|---|---|---|---|---|---|---|---|
√ | √ | √ | √ | √ | √ | √ | √ | √ |
OBJECT parameter description:
Attribute | Type | Default | Required | Description |
---|---|---|---|---|
animation | boolean | false | 否 | 是否需要动画效果,仅微信小程序、支付宝小程序、百度小程序、抖音小程序、飞书小程序、QQ小程序、快手小程序、京东小程序支持 |
success | Function | No | Callback function for successful interface call | |
fail | Function | No | Callback function for interface call failure | |
complete | Function | No | The callback function of the end of the interface call (it will be executed when the call succeeds or fails) |
Add text to the upper right corner of an item in the tabBar.
Platform Difference Description
App | H5 | 微信小程序 | 支付宝小程序 | 百度小程序 | 抖音小程序、飞书小程序 | QQ小程序 | 快手小程序 | 京东小程序 |
---|---|---|---|---|---|---|---|---|
√ | √ | √ | √ | √ | √ | √ | √ | √ |
OBJECT parameter description:
Parameter | Type | Required | Description |
---|---|---|---|
index | Number | which item of | tabBar, counting from the left |
text | String | Yes | the displayed text, no more than 3 half-width characters |
success | Function | No | Callback function for successful interface call |
fail | Function | No | Callback function for interface call failure |
complete | Function | No | The callback function for the end of the interface call (it will be executed when the call succeeds or fails) |
Example Code
uni.setTabBarBadge({
index: 0,
text: '1'
})
Remove the text in the upper right corner of an item in the tabBar.
Platform Difference Description
App | H5 | 微信小程序 | 支付宝小程序 | 百度小程序 | 抖音小程序、飞书小程序 | QQ小程序 | 快手小程序 | 京东小程序 |
---|---|---|---|---|---|---|---|---|
√ | √ | √ | √ | √ | √ | √ | √ | √ |
OBJECT parameter description:
Parameter | Type | Required | Description |
---|---|---|---|
index | Number | which item of | tabBar, counting from the left |
success | Function | No | Callback function for successful interface call |
fail | Function | No | Callback function for interface call failure |
complete | Function | No | The callback function for the end of the interface call (it will be executed when the call succeeds or fails) |
Displays a red dot in the upper right corner of an item in the tabBar.
Platform Difference Description
App | H5 | 微信小程序 | 支付宝小程序 | 百度小程序 | 抖音小程序、飞书小程序 | QQ小程序 | 快手小程序 | 京东小程序 |
---|---|---|---|---|---|---|---|---|
√ | √ | √ | √ | √ | √ | √ | √ | √ |
OBJECT parameter description:
Parameter | Type | Required | Description |
---|---|---|---|
index | Number | which item of | tabBar, counting from the left |
success | Function | No | Callback function for successful interface call |
fail | Function | No | Callback function for interface call failure |
complete | Function | No | The callback function for the end of the interface call (it will be executed when the call succeeds or fails) |
Hide the red dot in the upper right corner of an item in the tabBar.
Platform Difference Description
App | H5 | 微信小程序 | 支付宝小程序 | 百度小程序 | 抖音小程序、飞书小程序 | QQ小程序 | 快手小程序 | 京东小程序 |
---|---|---|---|---|---|---|---|---|
√ | √ | √ | √ | √ | √ | √ | √ | √ |
OBJECT parameter description:
Parameter | Type | Required | Description |
---|---|---|---|
index | Number | which item of | tabBar, counting from the left |
success | Function | No | Callback function for successful interface call |
fail | Function | No | Callback function for interface call failure |
complete | Function | No | The callback function for the end of the interface call (it will be executed when the call succeeds or fails) |
Listen to the click event of the middle button
Platform Difference Description
App | H5 | 微信小程序 | 支付宝小程序 | 百度小程序 | 抖音小程序、飞书小程序 | QQ小程序 | 快手小程序 | 京东小程序 |
---|---|---|---|---|---|---|---|---|
√(HBuilderX 2.3.4+) | √ | x | x | x | x | x | x | x |
Tip