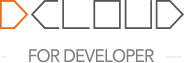
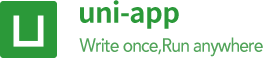
English
Component Type:UniTextareaElement
多行输入框
name | type | default | description |
---|---|---|---|
value | string | 输入框的初始内容 | |
placeholder | string | 输入框为空时占位符 | |
placeholder-style | string | 指定 placeholder 的样式 | |
placeholder-class | string | 指定 placeholder 的样式类 | |
maxlength | number | 140 | 最大输入长度,设置为 -1 的时候不限制最大长度 |
auto-focus | boolean | false | 自动获取焦点 |
focus | boolean | false | 获取焦点 |
cursor | number | 0 | 指定focus时的光标位置 |
confirm-type | string | done | 设置键盘右下角按钮的文字 confirm-type |
confirm-hold | boolean | false | 点击键盘右下角按钮时是否保持键盘不收起 |
auto-height | boolean | false | 是否自动增高,设置auto-height时,style.height不生效 |
cursor-spacing | number | 0 | 指定光标与键盘的距离,单位 px 。取 textarea 距离底部的距离和 cursor-spacing 指定的距离的最小值作为光标与键盘的距离 |
selection-start | number | -1 | 光标起始位置,自动聚集时有效,需与selection-end搭配使用 |
selection-end | number | -1 | 光标结束位置,自动聚集时有效,需与selection-satrt搭配使用 |
adjust-position | boolean | true | 键盘弹起时,是否自动上推页面 |
@confirm | (event: InputConfirmEvent) => void | - | 点击完成时, 触发 confirm 事件,event.detail = {value: value} |
@input | (event: InputEvent) => void | - | 当键盘输入时,触发 input 事件,event.detail = {value, cursor}, @input 处理函数的返回值并不会反映到 textarea 上 |
@linechange | (event: TextareaLineChangeEvent) => void | - | 输入框行数变化时调用,event.detail = {height: 0, heightRpx: 0, lineCount: 0} |
@blur | (event: TextareaBlurEvent) => void | - | 输入框失去焦点时触发,event.detail = {value, cursor} |
@keyboardheightchange | (event: InputKeyboardHeightChangeEvent) => void | - | 键盘高度发生变化的时候触发此事件,event.detail = {height: height, duration: duration} |
@focus | (event: TextareaFocusEvent) => void | - | 输入框聚焦时触发,event.detail = { value, height },height 为键盘高度,在基础库 1.9.90 起支持 |
name | description |
---|---|
send | 发送 |
search | 搜索 |
next | 下一个 |
go | 前往 |
done | 完成 |
name | type | required | default | description |
---|---|---|---|---|
detail | InputConfirmEventDetail | YES | - | - |
type | string | YES | - | 事件类型 |
target | Element | YES | - | 触发事件的组件 |
currentTarget | Element | YES | - | 当前组件 |
timeStamp | number | YES | - | 事件发生时的时间戳 |
name | type | optinal | default | description |
---|---|---|---|---|
value | string | YES | - | 输入框内容 |
name | type | required | default | description |
---|---|---|---|---|
stopPropagation | () => void | YES | - | 阻止当前事件的进一步传播 |
preventDefault | () => void | YES | - | 阻止当前事件的默认行为 |
name | type | required | default | description |
---|---|---|---|---|
detail | InputEventDetail | YES | - | - |
type | string | YES | - | 事件类型 |
target | Element | YES | - | 触发事件的组件 |
currentTarget | Element | YES | - | 当前组件 |
timeStamp | number | YES | - | 事件发生时的时间戳 |
name | type | optinal | default | description |
---|---|---|---|---|
value | string | YES | - | 输入框内容 |
cursor | number | YES | - | 光标的位置 |
keyCode | number | YES | - | 输入字符的Unicode值 |
name | type | required | default | description |
---|---|---|---|---|
stopPropagation | () => void | YES | - | 阻止当前事件的进一步传播 |
preventDefault | () => void | YES | - | 阻止当前事件的默认行为 |
name | type | required | default | description |
---|---|---|---|---|
detail | TextareaLineChangeEventDetail | YES | - | - |
type | string | YES | - | 事件类型 |
target | Element | YES | - | 触发事件的组件 |
currentTarget | Element | YES | - | 当前组件 |
timeStamp | number | YES | - | 事件发生时的时间戳 |
name | type | optinal | default | description |
---|---|---|---|---|
lineCount | number | YES | - | 行数 |
heightRpx | number | YES | - | textarea的高度 |
height | number | YES | - | textarea的高度 |
name | type | required | default | description |
---|---|---|---|---|
stopPropagation | () => void | YES | - | 阻止当前事件的进一步传播 |
preventDefault | () => void | YES | - | 阻止当前事件的默认行为 |
name | type | required | default | description |
---|---|---|---|---|
detail | TextareaBlurEventDetail | YES | - | - |
type | string | YES | - | 事件类型 |
target | Element | YES | - | 触发事件的组件 |
currentTarget | Element | YES | - | 当前组件 |
timeStamp | number | YES | - | 事件发生时的时间戳 |
name | type | optinal | default | description |
---|---|---|---|---|
value | string | YES | - | 输入框内容 |
cursor | number | YES | - | 选择区域的起始位置 |
name | type | required | default | description |
---|---|---|---|---|
stopPropagation | () => void | YES | - | 阻止当前事件的进一步传播 |
preventDefault | () => void | YES | - | 阻止当前事件的默认行为 |
name | type | required | default | description |
---|---|---|---|---|
detail | InputKeyboardHeightChangeEventDetail | YES | - | - |
type | string | YES | - | 事件类型 |
target | Element | YES | - | 触发事件的组件 |
currentTarget | Element | YES | - | 当前组件 |
timeStamp | number | YES | - | 事件发生时的时间戳 |
name | type | optinal | default | description |
---|---|---|---|---|
height | number | YES | - | 键盘高度 |
duration | number | YES | - | 持续时间 |
name | type | required | default | description |
---|---|---|---|---|
stopPropagation | () => void | YES | - | 阻止当前事件的进一步传播 |
preventDefault | () => void | YES | - | 阻止当前事件的默认行为 |
name | type | required | default | description |
---|---|---|---|---|
detail | TextareaFocusEventDetail | YES | - | - |
type | string | YES | - | 事件类型 |
target | Element | YES | - | 触发事件的组件 |
currentTarget | Element | YES | - | 当前组件 |
timeStamp | number | YES | - | 事件发生时的时间戳 |
name | type | optinal | default | description |
---|---|---|---|---|
height | number | YES | - | 键盘高度 |
value | string | YES | - | 输入框内容 |
name | type | required | default | description |
---|---|---|---|---|
stopPropagation | () => void | YES | - | 阻止当前事件的进一步传播 |
preventDefault | () => void | YES | - | 阻止当前事件的默认行为 |
<script>
import { type ItemType } from '@/components/enum-data/enum-data.vue'
export default {
data() {
return {
adjust_position_boolean: false,
show_confirm_bar_boolean: false,
fixed_boolean: false,
auto_height_boolean: false,
confirm_hold_boolean: false,
focus_boolean: false,
auto_focus_boolean: false,
default_value:"",
maxlength:-1,
confirm_type_enum: [{"value":0,"name":"send"},{"value":1,"name":"search"},{"value":2,"name":"next"},{"value":3,"name":"go"},{"value":4,"name":"done"}] as ItemType[],
confirm_type_enum_current: 0,
inputmode_enum: [{"value":0,"name":"none"},{"value":1,"name":"text"},{"value":2,"name":"decimal"},{"value":3,"name":"numeric"},{"value":4,"name":"tel"},{"value":5,"name":"search"},{"value":6,"name":"email"},{"value":7,"name":"url"}] as ItemType[],
inputmode_enum_current: 0
}
},
methods: {
textarea_click() { console.log("组件被点击时触发") },
textarea_touchstart() { console.log("手指触摸动作开始") },
textarea_touchmove() { console.log("手指触摸后移动") },
textarea_touchcancel() { console.log("手指触摸动作被打断,如来电提醒,弹窗") },
textarea_touchend() { console.log("手指触摸动作结束") },
textarea_tap() { console.log("手指触摸后马上离开") },
textarea_longpress() { console.log("如果一个组件被绑定了 longpress 事件,那么当用户长按这个组件时,该事件将会被触发。") },
textarea_confirm() { console.log("点击完成时, 触发 confirm 事件,event.detail = {value: value}") },
textarea_input() { console.log("当键盘输入时,触发 input 事件,event.detail = {value, cursor}, @input 处理函数的返回值并不会反映到 textarea 上") },
textarea_linechange() { console.log("输入框行数变化时调用,event.detail = {height: 0, heightRpx: 0, lineCount: 0}") },
textarea_blur() { console.log("输入框失去焦点时触发,event.detail = {value, cursor}") },
textarea_keyboardheightchange() { console.log("键盘高度发生变化的时候触发此事件,event.detail = {height: height, duration: duration}") },
textarea_focus() { console.log("输入框聚焦时触发,event.detail = { value, height },height 为键盘高度,在基础库 1.9.90 起支持") },
change_adjust_position_boolean(checked : boolean) { this.adjust_position_boolean = checked },
change_show_confirm_bar_boolean(checked : boolean) { this.show_confirm_bar_boolean = checked },
change_fixed_boolean(checked : boolean) { this.fixed_boolean = checked },
change_auto_height_boolean(checked : boolean) { this.auto_height_boolean = checked },
change_confirm_hold_boolean(checked : boolean) { this.confirm_hold_boolean = checked },
change_focus_boolean(checked : boolean) { this.focus_boolean = checked },
change_auto_focus_boolean(checked : boolean) { this.auto_focus_boolean = checked },
radio_change_confirm_type_enum(checked : number) { this.confirm_type_enum_current = checked },
radio_change_inputmode_enum(checked : number) { this.inputmode_enum_current = checked }
}
}
</script>
<template>
<view class="main">
<textarea
:value="default_value"
class="uni-textarea"
:auto-focus="auto_focus_boolean"
:focus="focus_boolean"
:confirm-type="confirm_type_enum[confirm_type_enum_current].name"
:confirm-hold="confirm_hold_boolean"
:auto-height="auto_height_boolean"
:fixed="fixed_boolean"
:show-confirm-bar="show_confirm_bar_boolean"
:adjust-position="adjust_position_boolean"
:inputmode="inputmode_enum[inputmode_enum_current].name"
:maxlength="maxlength"
@click="textarea_click"
@touchstart="textarea_touchstart"
@touchmove="textarea_touchmove"
@touchcancel="textarea_touchcancel"
@touchend="textarea_touchend"
@tap="textarea_tap"
@longpress="textarea_longpress"
@confirm="textarea_confirm"
@input="textarea_input"
@linechange="textarea_linechange"
@blur="textarea_blur"
@keyboardheightchange="textarea_keyboardheightchange"
@focus="textarea_focus"
style="padding: 20rpx; border: 1px solid #666"
/>
</view>
<!-- #ifdef APP -->
<scroll-view style="flex: 1">
<!-- #endif -->
<view class="content nvue">
<boolean-data
:defaultValue="false"
title="键盘弹起时,是否自动上推页面"
@change="change_adjust_position_boolean"
></boolean-data>
<!-- #ifdef !APP -->
<boolean-data
:defaultValue="false"
title="是否显示键盘上方带有”完成“按钮那一栏"
@change="change_show_confirm_bar_boolean"
></boolean-data>
<boolean-data
:defaultValue="false"
title="如果 textarea 是在一个 position:fixed 的区域,需要显示指定属性 fixed 为 true"
@change="change_fixed_boolean"
></boolean-data>
<!-- #endif -->
<boolean-data
:defaultValue="false"
title="是否自动增高,设置auto-height时,style.height不生效"
@change="change_auto_height_boolean"
></boolean-data>
<boolean-data
:defaultValue="false"
title="点击键盘右下角按钮时是否保持键盘不收起"
@change="change_confirm_hold_boolean"
></boolean-data>
<boolean-data
:defaultValue="focus_boolean"
title="获取焦点"
@change="change_focus_boolean" class="textarea-focus"
></boolean-data>
<boolean-data
:defaultValue="false"
title="自动获取焦点"
@change="change_auto_focus_boolean"
></boolean-data>
<enum-data class="textarea-confirm"
:items="confirm_type_enum"
title="设置键盘右下角按钮的文字"
@change="radio_change_confirm_type_enum"
></enum-data>
<enum-data
:items="inputmode_enum" class="textarea-input"
title="是一个枚举属性,它提供了用户在编辑元素或其内容时可能输入的数据类型的提示。在符合条件的高版本webview里,uni-app的 web 和 app-vue 平台中可使用本属性。"
@change="radio_change_inputmode_enum"
></enum-data>
</view>
<!-- #ifdef APP -->
</scroll-view>
<!-- #endif -->
</template>
<style>
.main {
max-height: 500rpx;
padding: 10rpx 0;
border-bottom: 1px solid rgba(0, 0, 0, 0.06);
flex-direction: row;
justify-content: center;
}
.main .list-item {
width: 100%;
height: 200rpx;
border: 1px solid #666;
}
</style>
Android version | Android uni-app | Android uni-app-x | iOS version | iOS uni-app | iOS uni-app-x | |
---|---|---|---|---|---|---|
textarea | 5.0 | √ | 3.9+ | 9.0 | √ | - |
@confirm | 5.0 | √ | 3.9+ | - | - | - |
@input | 5.0 | √ | 3.9+ | - | - | - |
@linechange | 5.0 | √ | 3.9+ | - | - | - |
@blur | 5.0 | √ | 3.9+ | - | - | - |
@keyboardheightchange | 5.0 | √ | 3.9+ | - | - | - |
@focus | 5.0 | √ | 3.9+ | - | - | - |
Can't nest components