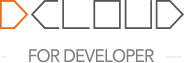
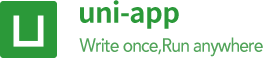
English
Component Type:UniProgressElement
进度条
name | type | default | description |
---|---|---|---|
duration | number | 30 | 进度增加1%所需毫秒数 |
percent | number | 0 | 百分比0~100 |
show-info | boolean | false | 在进度条右侧显示百分比 |
border-radius | number | 0 | 圆角大小 |
font-size | number | 16 | 右侧百分比字体大小 |
stroke-width | number | 6 | 进度条线的宽度,单位px |
color | string.ColorString | - | 进度条颜色 (请使用 activeColor) |
activeColor | string.ColorString | #09BB07 | 已选择的进度条的颜色 |
backgroundColor | string.ColorString | #EBEBEB | 未选择的进度条的颜色 |
active | boolean | false | 进度条从左往右的动画 |
active-mode | string | backwards | backwards: 动画从头播;forwards:动画从上次结束点接着播 active-mode |
@activeend | (event: ProgressActiveendEvent) => void | - | 动画完成事件 |
name | description |
---|---|
backwards | 动画从头播 |
forwards | 动画从上次结束点接着播 |
name | type | required | default | description |
---|---|---|---|---|
value | number | YES | - | - |
name | type | required | default | description |
---|---|---|---|---|
detail | ProgressActiveendEventDetail | YES | - | - |
type | string | YES | - | 事件类型 |
target | Element | YES | - | 触发事件的组件 |
currentTarget | Element | YES | - | 当前组件 |
timeStamp | number | YES | - | 事件发生时的时间戳 |
name | type | optinal | default | description |
---|---|---|---|---|
ctors | Constructor | YES | - | - |
curPercent | number | YES | - | - |
name | type | required | default | description |
---|---|---|---|---|
stopPropagation | () => void | YES | - | 阻止当前事件的进一步传播 |
preventDefault | () => void | YES | - | 阻止当前事件的默认行为 |
<script>
import { type ItemType } from '@/components/enum-data/enum-data.vue'
export default {
data() {
return {
title: 'progress',
pgList: [0, 0, 0, 0] as number[],
curPercent: 0,
showInfo: true,
borderRadius: 0,
fontSize: 16,
strokeWidth: 3,
backgroundColor: '#EBEBEB',
// 组件属性 autotest
active_boolean: false,
show_info_boolean: false,
duration_input: 30,
percent_input: 0,
stroke_width_input: 6,
activeColor_input: "#09BB07",
backgroundColor_input: "#EBEBEB",
active_mode_enum: [{ "value": 0, "name": "backwards" }, { "value": 1, "name": "forwards" }] as ItemType[],
active_mode_enum_current: 0
}
},
methods: {
setProgress() {
this.pgList = [20, 40, 60, 80] as number[]
},
clearProgress() {
this.pgList = [0, 0, 0, 0] as number[]
},
activeend(e : ProgressActiveendEvent) {
this.curPercent = e.detail.curPercent
},
progress_touchstart() { console.log("手指触摸动作开始") },
progress_touchmove() { console.log("手指触摸后移动") },
progress_touchcancel() { console.log("手指触摸动作被打断,如来电提醒,弹窗") },
progress_touchend() { console.log("手指触摸动作结束") },
progress_tap() { console.log("手指触摸后马上离开") },
change_active_boolean(checked : boolean) { this.active_boolean = checked },
change_show_info_boolean(checked : boolean) { this.show_info_boolean = checked },
confirm_duration_input(value : number) { this.duration_input = value },
confirm_percent_input(value : number) { this.percent_input = value },
confirm_stroke_width_input(value : number) { this.stroke_width_input = value },
confirm_activeColor_input(value : string) { this.activeColor_input = value },
confirm_backgroundColor_input(value : string) { this.backgroundColor_input = value },
radio_change_active_mode_enum(checked : number) { this.active_mode_enum_current = checked }
}
}
</script>
<template>
<view class="main">
<progress
:duration="duration_input"
:percent="percent_input"
:show-info="show_info_boolean"
:stroke-width="stroke_width_input"
:activeColor="activeColor_input"
:backgroundColor="backgroundColor_input"
:active="active_boolean"
:active-mode="active_mode_enum[active_mode_enum_current].name"
@touchstart="progress_touchstart"
@touchmove="progress_touchmove"
@touchcancel="progress_touchcancel"
@touchend="progress_touchend"
@tap="progress_tap"
style="width: 80%"
>
<text>uni-app-x</text>
</progress>
</view>
<!-- #ifdef APP -->
<scroll-view style="flex: 1">
<!-- #endif -->
<view class="content nvue">
<page-head title="组件属性"></page-head>
<boolean-data
:defaultValue="false"
title="进度条从左往右的动画"
@change="change_active_boolean"
></boolean-data>
<boolean-data
:defaultValue="false"
title="在进度条右侧显示百分比"
@change="change_show_info_boolean"
></boolean-data>
<input-data
defaultValue="30"
title="进度增加1%所需毫秒数"
type="number"
@confirm="confirm_duration_input"
></input-data>
<input-data
defaultValue="0"
title="百分比0~100"
type="number"
@confirm="confirm_percent_input"
></input-data>
<input-data
defaultValue="6"
title="进度条线的宽度,单位px"
type="number"
@confirm="confirm_stroke_width_input"
></input-data>
<input-data
defaultValue="#09BB07"
title="已选择的进度条的颜色"
type="text"
@confirm="confirm_activeColor_input"
></input-data>
<input-data
defaultValue="#EBEBEB"
title="未选择的进度条的颜色"
type="text"
@confirm="confirm_backgroundColor_input"
></input-data>
<enum-data
:items="active_mode_enum"
title="backwards: 动画从头播;forwards:动画从上次结束点接着播"
@change="radio_change_active_mode_enum"
></enum-data>
</view>
<view>
<page-head title="默认及使用"></page-head>
<view class="uni-padding-wrap uni-common-mt">
<view class="progress-box">
<progress
:percent="pgList[0]"
:active="true"
:border-radius="borderRadius"
:show-info="showInfo"
:font-size="fontSize"
:stroke-width="strokeWidth"
:background-color="backgroundColor"
class="progress p"
@activeend="activeend"
/>
</view>
<view class="progress-box">
<progress
:percent="pgList[1]"
:stroke-width="3"
class="progress p1"
/>
</view>
<view class="progress-box">
<progress
:percent="pgList[2]"
:stroke-width="3"
class="progress p2"
/>
</view>
<view class="progress-box">
<progress
:percent="pgList[3]"
activeColor="#10AEFF"
:stroke-width="3"
class="progress p3"
/>
</view>
<view class="progress-control">
<button type="primary" @click="setProgress" class="button">
设置进度
</button>
<button type="warn" @click="clearProgress" class="button">
清除进度
</button>
</view>
</view>
</view>
<!-- #ifdef APP -->
</scroll-view>
<!-- #endif -->
</template>
<style>
.main {
max-height: 500rpx;
padding: 10rpx 0;
border-bottom: 1px solid rgba(0, 0, 0, 0.06);
flex-direction: row;
justify-content: center;
}
.main .list-item {
width: 100%;
height: 200rpx;
border: 1px solid #666;
}
.progress-box {
height: 50rpx;
margin-bottom: 60rpx;
}
.progress-cancel {
margin-left: 40rpx;
}
.button {
margin-top: 20rpx;
}
</style>
Android version | Android uni-app | Android uni-app-x | iOS version | iOS uni-app | iOS uni-app-x | |
---|---|---|---|---|---|---|
progress | 5.0 | √ | √ | 10.0 | √ | x |
duration | 5.0 | √ | √ | 10.0 | √ | x |
percent | 5.0 | √ | √ | 10.0 | √ | x |
show-info | 5.0 | √ | √ | 10.0 | √ | x |
border-radius | 5.0 | √ | √ | 10.0 | √ | x |
font-size | 5.0 | √ | √ | 10.0 | √ | x |
stroke-width | 5.0 | √ | √ | 10.0 | √ | x |
activeColor | 5.0 | √ | √ | 10.0 | √ | x |
backgroundColor | 5.0 | √ | √ | 10.0 | √ | x |
active | 5.0 | √ | √ | 10.0 | √ | x |
active-mode | 5.0 | √ | √ | 10.0 | √ | x |
@activeend | 5.0 | x | √ | 10.0 | x | x |