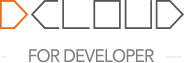
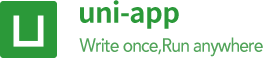
English
Component Type:UniRadioGroupElement
单选组,内部由多个 radio 组成
name | type | default | description |
---|---|---|---|
@change | (event: RadioGroupChangeEvent) => void | - | radio-group 中的选中项发生变化时触发 change 事件,event.detail = {value: 选中项radio的value} |
name | type | required | default | description |
---|---|---|---|---|
value | string | YES | - | - |
name | type | required | default | description |
---|---|---|---|---|
detail | RadioGroupChangeEventDetail | YES | - | - |
type | string | YES | - | 事件类型 |
target | Element | YES | - | 触发事件的组件 |
currentTarget | Element | YES | - | 当前组件 |
timeStamp | number | YES | - | 事件发生时的时间戳 |
name | type | optinal | default | description |
---|---|---|---|---|
ctors | Constructor | YES | - | - |
value | string | YES | - | - |
name | type | required | default | description |
---|---|---|---|---|
stopPropagation | () => void | YES | - | 阻止当前事件的进一步传播 |
preventDefault | () => void | YES | - | 阻止当前事件的默认行为 |
Android version | Android uni-app | Android uni-app-x | iOS version | iOS uni-app | iOS uni-app-x | |
---|---|---|---|---|---|---|
radio-group | 5.0 | √ | √ | 10.0 | √ | x |
@change | 5.0 | √ | √ | 10.0 | √ | x |
Component Type:UniRadioElement
单选项。在1组radio-group中只能选中1个
name | type | default | description |
---|---|---|---|
disabled | boolean | false | 是否禁用 |
value | string | - | <radio/> 标识。当该radio 选中时,radio-group的 change 事件会携带radio的value |
checked | boolean | false | <radio/> 当前是否选中 |
color | string.ColorString | #007AFF | radio的颜色 |
backgroundColor | string.ColorString | #ffffff | radio默认的背景颜色 |
borderColor | string.ColorString | #d1d1d1 | radio默认的边框颜色 |
activeBackgroundColor | string.ColorString | #007AFF | radio选中时的背景颜色,优先级大于color属性 |
activeBorderColor | string.ColorString | radio选中时的边框颜色 | |
iconColor | string.ColorString | #ffffff | radio的图标颜色 |
<script>
type ItemType = {
value : string
name : string
}
export default {
data() {
return {
items: [
{
value: 'CHN',
name: '中国',
},
{
value: 'USA',
name: '美国',
},
{
value: 'BRA',
name: '巴西',
},
{
value: 'JPN',
name: '日本',
},
{
value: 'ENG',
name: '英国',
},
{
value: 'FRA',
name: '法国',
},
] as ItemType[],
current: 0,
value: '',
text: '未选中',
wrapText: 'uni-app x,终极跨平台方案\nuts,大一统语言',
disabled: true,
checked: true,
color: '#007aff',
// 组件属性 autotest
checked_boolean: false,
disabled_boolean: false,
color_input: "#007AFF",
backgroundColor_input: "#ffffff",
borderColor_input: "#d1d1d1",
activeBackgroundColor_input: "#007AFF",
activeBorderColor_input: "",
iconColor_input: "#ffffff"
}
},
methods: {
radioChange(e : RadioGroupChangeEvent) {
const selected = this.items.find((item) : boolean => {
return item.value == e.detail.value
})
uni.showToast({
icon: 'none',
title: '当前选中:' + selected?.name,
})
},
testChange(e : RadioGroupChangeEvent) {
this.value = e.detail.value
},
radio_click() { console.log("组件被点击时触发") },
radio_touchstart() { console.log("手指触摸动作开始") },
radio_touchmove() { console.log("手指触摸后移动") },
radio_touchcancel() { console.log("手指触摸动作被打断,如来电提醒,弹窗") },
radio_touchend() { console.log("手指触摸动作结束") },
radio_tap() { console.log("手指触摸后马上离开") },
radio_longpress() { console.log("如果一个组件被绑定了 longpress 事件,那么当用户长按这个组件时,该事件将会被触发。") },
change_checked_boolean(checked : boolean) { this.checked_boolean = checked },
change_disabled_boolean(checked : boolean) { this.disabled_boolean = checked },
confirm_color_input(value : string) { this.color_input = value },
confirm_backgroundColor_input(value : string) { this.backgroundColor_input = value },
confirm_borderColor_input(value : string) { this.borderColor_input = value },
confirm_activeBackgroundColor_input(value : string) { this.activeBackgroundColor_input = value },
confirm_activeBorderColor_input(value : string) { this.activeBorderColor_input = value },
confirm_iconColor_input(value : string) { this.iconColor_input = value }
}
}
</script>
<template>
<view class="main">
<radio
:disabled="disabled_boolean"
:checked="checked_boolean"
:color="color_input"
:backgroundColor="backgroundColor_input"
:borderColor="borderColor_input"
:activeBackgroundColor="activeBackgroundColor_input"
:activeBorderColor="activeBorderColor_input"
:iconColor="iconColor_input"
@click="radio_click"
@touchstart="radio_touchstart"
@touchmove="radio_touchmove"
@touchcancel="radio_touchcancel"
@touchend="radio_touchend"
@tap="radio_tap"
@longpress="radio_longpress"
>
<text>uni-app-x</text>
</radio>
</view>
<!-- #ifdef APP -->
<scroll-view style="flex: 1">
<!-- #endif -->
<view class="content nvue">
<page-head title="组件属性"></page-head>
<boolean-data
:defaultValue="false"
title="<radio/> 当前是否选中"
@change="change_checked_boolean"
></boolean-data>
<boolean-data
:defaultValue="false"
title="是否禁用"
@change="change_disabled_boolean"
></boolean-data>
<input-data
defaultValue="#007AFF"
title="radio的颜色"
type="text"
@confirm="confirm_color_input"
></input-data>
<input-data
defaultValue="#ffffff"
title="radio默认的背景颜色"
type="text"
@confirm="confirm_backgroundColor_input"
></input-data>
<input-data
defaultValue="#d1d1d1"
title="radio默认的边框颜色"
type="text"
@confirm="confirm_borderColor_input"
></input-data>
<input-data
defaultValue="#007AFF"
title="radio选中时的背景颜色,优先级大于color属性"
type="text"
@confirm="confirm_activeBackgroundColor_input"
></input-data>
<input-data
defaultValue=""
title="radio选中时的边框颜色"
type="text"
@confirm="confirm_activeBorderColor_input"
></input-data>
<input-data
defaultValue="#ffffff"
title="radio的图标颜色"
type="text"
@confirm="confirm_iconColor_input"
></input-data>
</view>
<view>
<page-head title="默认及使用"></page-head>
<view class="uni-padding-wrap">
<view class="uni-title uni-common-mt">
<text class="uni-title-text"> 默认样式 </text>
</view>
<radio-group
class="uni-flex uni-row radio-group"
@change="testChange"
style="flex-wrap: wrap"
>
<radio
value="r"
:checked="checked"
:color="color"
style="margin-right: 30rpx"
class="radio r"
>选中
</radio>
<radio value="r1" style="margin-right: 30rpx" class="radio r1">{{
text
}}</radio>
<radio value="r2" :disabled="disabled" class="radio r2">禁用</radio>
<radio value="r3" class="radio r3" style="margin-top: 20rpx">{{
wrapText
}}</radio>
</radio-group>
</view>
<view class="uni-padding-wrap">
<view class="uni-title uni-common-mt">
<text class="uni-title-text"> 不同颜色和尺寸的radio </text>
</view>
<radio-group class="uni-flex uni-row radio-group">
<radio
value="r1"
:checked="true"
color="#FFCC33"
style="transform: scale(0.7); margin-right: 30rpx"
class="radio"
>选中
</radio>
<radio
value="r2"
color="#FFCC33"
style="transform: scale(0.7)"
class="radio"
>未选中</radio
>
</radio-group>
</view>
<view class="uni-padding-wrap">
<view class="uni-title uni-common-mt">
<text class="uni-title-text"> 推荐展示样式 </text>
</view>
</view>
<view class="uni-list uni-common-pl">
<radio-group @change="radioChange" class="radio-group">
<radio
class="uni-list-cell uni-list-cell-pd radio"
v-for="(item, index) in items"
:key="item.value"
:class="index < items.length - 1 ? 'uni-list-cell-line' : ''"
:value="item.value"
:checked="index === current"
>
{{ item.name }}
</radio>
</radio-group>
</view>
</view>
<!-- #ifdef APP -->
</scroll-view>
<!-- #endif -->
</template>
<style>
.main {
max-height: 500rpx;
padding: 10rpx 0;
border-bottom: 1px solid rgba(0, 0, 0, 0.06);
flex-direction: row;
justify-content: center;
}
.main .list-item {
width: 100%;
height: 200rpx;
border: 1px solid #666;
}
.uni-list-cell {
justify-content: flex-start;
}
</style>
Android version | Android uni-app | Android uni-app-x | iOS version | iOS uni-app | iOS uni-app-x | |
---|---|---|---|---|---|---|
radio | 5.0 | √ | √ | 10.0 | √ | x |
disabled | 5.0 | √ | √ | 10.0 | √ | x |
value | 5.0 | √ | √ | 10.0 | √ | x |
checked | 5.0 | √ | √ | 10.0 | √ | x |
color | 5.0 | √ | √ | 10.0 | √ | x |
backgroundColor | 5.0 | x | √ | 10.0 | x | x |
borderColor | 5.0 | x | √ | 10.0 | x | x |
activeBackgroundColor | 5.0 | x | √ | 10.0 | x | x |
activeBorderColor | 5.0 | x | √ | 10.0 | x | x |
iconColor | 5.0 | x | √ | 10.0 | x | x |