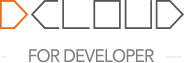
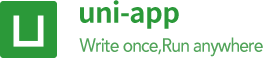
English
Component Type:UniFormElement
表单
name | type | default | description |
---|---|---|---|
disabled | boolean | - | 是否禁用 |
@submit | (event: FormSubmitEvent) => void | - | 携带 form 中的数据触发 submit 事件,event.detail = {value : {'name': 'value'}} |
@reset | (event: FormResetEvent) => void | - | 表单重置时会触发 reset 事件 |
name | type | required | default | description |
---|---|---|---|---|
value | UTSJSONObject | YES | - | - |
name | type | required | default | description |
---|---|---|---|---|
detail | FormSubmitEventDetail | YES | - | - |
type | string | YES | - | 事件类型 |
target | Element | YES | - | 触发事件的组件 |
currentTarget | Element | YES | - | 当前组件 |
timeStamp | number | YES | - | 事件发生时的时间戳 |
name | type | optinal | default | description |
---|---|---|---|---|
ctors | Constructor | YES | - | - |
value | UTSJSONObject | YES | - | - |
name | type | required | default | description |
---|---|---|---|---|
stopPropagation | () => void | YES | - | 阻止当前事件的进一步传播 |
preventDefault | () => void | YES | - | 阻止当前事件的默认行为 |
name | type | required | default | description |
---|---|---|---|---|
detail | FormResetEventDetail | YES | - | - |
type | string | YES | - | 事件类型 |
target | Element | YES | - | 触发事件的组件 |
currentTarget | Element | YES | - | 当前组件 |
timeStamp | number | YES | - | 事件发生时的时间戳 |
name | type | optinal | default | description |
---|---|---|---|---|
ctors | Constructor | YES | - | - |
name | type | required | default | description |
---|---|---|---|---|
stopPropagation | () => void | YES | - | 阻止当前事件的进一步传播 |
preventDefault | () => void | YES | - | 阻止当前事件的默认行为 |
<template>
<!-- #ifdef APP -->
<scroll-view class="page">
<!-- #endif -->
<form @submit="onFormSubmit" @reset="onFormReset">
<view class="uni-form-item">
<view class="title">姓名</view>
<input class="uni-input" name="nickname" :value="nickname" placeholder="请输入姓名" />
</view>
<view class="uni-form-item">
<view class="title">性别</view>
<radio-group name="gender" class="flex-row">
<view class="group-item">
<radio :value="0" :checked="gender=='0'" /><text>男</text>
</view>
<view class="group-item">
<radio :value="1" :checked="gender=='1'" /><text>女</text>
</view>
</radio-group>
</view>
<view class="uni-form-item">
<view class="title">爱好</view>
<checkbox-group name="loves" class="flex-row">
<view class="group-item">
<checkbox value="0" :checked="loves.indexOf('0')>-1" /><text>读书</text>
</view>
<view class="group-item">
<checkbox value="1" :checked="loves.indexOf('1')>-1" /><text>写字</text>
</view>
</checkbox-group>
</view>
<view class="uni-form-item">
<view class="title">年龄</view>
<slider name="age" :value="age" :show-value="true"></slider>
</view>
<view class="uni-form-item">
<view class="title">保留选项</view>
<view>
<switch name="switch" :checked="switch" />
</view>
</view>
<view class="uni-btn-v flex-row">
<button class="btn btn-submit" form-type="submit" type="primary">Submit</button>
<button class="btn btn-reset" type="default" form-type="reset">Reset</button>
</view>
</form>
<view class="result">提交的表单数据</view>
<textarea class="textarea" :value="formDataText"></textarea>
<!-- #ifdef APP -->
</scroll-view>
<!-- #endif -->
</template>
<script>
export default {
data() {
return {
nickname: '',
gender: '0',
age: 18,
loves: ['0'],
switch: true,
formData: {} as UTSJSONObject
}
},
computed: {
formDataText() : string {
return JSON.stringify(this.formData)
}
},
methods: {
onFormSubmit: function (e : FormSubmitEvent) {
this.formData = e.detail.value
},
onFormReset: function (_ : FormResetEvent) {
this.formData = {}
}
}
}
</script>
<style>
.page {
flex: 1;
padding: 15px;
}
.flex-row {
flex-direction: row;
}
.uni-form-item {
padding: 15px 0;
}
.title {
margin-bottom: 10px;
}
.group-item {
flex-direction: row;
margin-right: 20px;
}
.btn {
flex: 1;
}
.btn-submit {
margin-right: 5px;
}
.btn-reset {
margin-left: 5px;
}
.result {
margin-top: 30px;
}
.textarea {
margin-top: 5px;
background-color: #fff;
}
</style>
Android version | Android uni-app | Android uni-app-x | iOS version | iOS uni-app | iOS uni-app-x | |
---|---|---|---|---|---|---|
form | 5.0 | √ | 3.97+ | 9.0 | √ | x |
disabled | 5.0 | √ | 3.97+ | 9.0 | √ | x |
form 组件的表单提交,微信小程序的实现策略,与浏览器W3C的策略略有差异。目前uni-app在app和web上的实现参考了微信小程序。具体是:
{"name": "value"}
。而浏览器标准form是数组,每项为 pair,pair[0] 对应name,pair[1] 对应value 。注意uni-app编译到web平台,也是按uni-app的策略,而不是浏览器的策略。uni-app 的 web平台使用 uni-app 自己的 form 组件,而不是浏览器的 form 标签。
reset在浏览器W3C的策略是还原、重置。
在uni-app中,不同平台的策略不同,有的是还原
,有的是清空
。
各平台策略如下:
uni-app-x App | uni-app App | Web | 微信小程序 | 支付宝小程序 | 百度小程序 | 抖音小程序 |
---|---|---|---|---|---|---|
还原(3.97+) | 清空 | 清空 | 清空 | 还原 | 清空 | 清空 |
<!-- reset 后为 name -->
<input name="input1" value="name" />
<!-- reset 后为 true -->
<switch name="switch1" :checked="true" />
<!-- reset 后为 50 -->
<slider name="slider1" :value="50" :min="10" :max="110" />
<!-- reset 后为 "写字" 被 checked -->
<checkbox-group name="loves">
<view>
<checkbox value="0" /><text>读书</text>
</view>
<view>
<checkbox value="1" :checked="true" /><text>写字</text>
</view>
</checkbox-group>
<!-- reset 后为 "" -->
<input name="input1" value="name" />
<!-- reset 后为 false -->
<switch name="switch1" :checked="true" />
<!-- reset 后为 最小值10 -->
<slider name="slider1" :value="50" :min="10" :max="110" />
<!-- reset 后为 无任何 checked -->
<checkbox-group name="loves">
<view>
<checkbox value="0" /><text>读书</text>
</view>
<view>
<checkbox value="1" :checked="true" /><text>写字</text>
</view>
</checkbox-group>