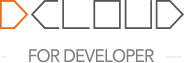
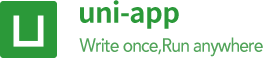
English
Component Type:UniButtonElement
按钮
name | type | default | description |
---|---|---|---|
disabled | boolean | false | 是否禁用 |
hover-class | string.ClassString | button-hover | 指定按下去的样式类。当 hover-class="none" 时,没有点击态效果 |
hover-start-time | number | 20 | 按住后多久出现点击态,单位毫秒 |
hover-stay-time | number | 70 | 手指松开后点击态保留时间,单位毫秒 |
size | string | default | 按钮的大小 size |
type | string | default | 按钮的样式类型 type |
plain | boolean | false | 按钮是否镂空,背景色透明 |
name | description |
---|---|
default | 默认大小 |
mini | 小尺寸 |
name | description |
---|---|
default | 白色 |
primary | 蓝色 |
warn | 红色 |
<script>
import { type ItemType } from '@/components/enum-data/enum-data.vue'
export default {
data() {
return {
plain_boolean: false,
disabled_boolean: false,
default_style: false,
size_enum: [{ "value": 0, "name": "default" }, { "value": 1, "name": "mini" }] as ItemType[],
size_enum_current: 0,
type_enum: [{ "value": 0, "name": "default" }, { "value": 1, "name": "primary" }, { "value": 2, "name": "warn" }] as ItemType[],
type_enum_current: 0,
count: 0,
text: 'uni-app-x',
style: 'color:#ffffff;backgroundColor:#1AAD19;borderColor:#1AAD19;'
}
},
methods: {
button_click() {
console.log("组件被点击时触发")
this.count++
},
button_touchstart() { console.log("手指触摸动作开始") },
button_touchmove() { console.log("手指触摸后移动") },
button_touchcancel() { console.log("手指触摸动作被打断,如来电提醒,弹窗") },
button_touchend() { console.log("手指触摸动作结束") },
button_tap() { console.log("手指触摸后马上离开") },
button_longpress() { console.log("如果一个组件被绑定了 longpress 事件,那么当用户长按这个组件时,该事件将会被触发。") },
change_plain_boolean(checked : boolean) { this.plain_boolean = checked },
change_disabled_boolean(checked : boolean) { this.disabled_boolean = checked },
change_default_style(checked : boolean) { this.default_style = checked },
radio_change_size_enum(checked : number) { this.size_enum_current = checked },
radio_change_type_enum(checked : number) { this.type_enum_current = checked },
confirm_text_input(value : string) { this.text = value }
}
}
</script>
<template>
<view class="main">
<button :disabled="disabled_boolean" :size="size_enum[size_enum_current].name"
:type="type_enum[type_enum_current].name" :plain="plain_boolean" @click="button_click"
@touchstart="button_touchstart" @touchmove="button_touchmove" @touchcancel="button_touchcancel"
@touchend="button_touchend" @tap="button_tap" @longpress="button_longpress" class="btn"
:style="default_style ? style : ''" :hover-class="default_style ? 'is-hover' : 'button-hover'">
{{ text }}
</button>
</view>
<!-- #ifdef APP -->
<scroll-view style="flex: 1">
<!-- #endif -->
<view class="content">
<boolean-data :defaultValue="false" title="按钮是否镂空,背景色透明" @change="change_plain_boolean"></boolean-data>
<boolean-data :defaultValue="false" title="是否禁用" @change="change_disabled_boolean"></boolean-data>
<boolean-data :defaultValue="false" title="修改默认样式和点击效果(高优先)" @change="change_default_style"></boolean-data>
<enum-data :items="size_enum" title="按钮的大小" @change="radio_change_size_enum"></enum-data>
<enum-data :items="type_enum" title="按钮的类型" @change="radio_change_type_enum"></enum-data>
<input-data :defaultValue="text" title="按钮的文案" type="text" @confirm="confirm_text_input"></input-data>
</view>
<!-- #ifdef APP -->
</scroll-view>
<!-- #endif -->
</template>
<style>
.main {
padding: 10rpx 0;
border-bottom: 1px solid rgba(0, 0, 0, 0.06);
flex-direction: row;
justify-content: center;
}
.main .list-item {
width: 100%;
height: 200rpx;
border: 1px solid #666;
}
.is-hover {
color: rgba(255, 255, 255, 0.6);
background-color: #179b16;
border-color: #179b16;
}
</style>
Android version | Android uni-app | Android uni-app-x | iOS version | iOS uni-app | iOS uni-app-x | |
---|---|---|---|---|---|---|
button | 5.0 | √ | √ | 10.0 | √ | x |
disabled | 5.0 | √ | √ | 10.0 | √ | x |
hover-class | 5.0 | √ | √ | 10.0 | √ | x |
hover-start-time | 5.0 | √ | √ | 10.0 | √ | x |
hover-stay-time | 5.0 | √ | √ | 10.0 | √ | x |
size | 5.0 | √ | √ | 10.0 | √ | x |
type | 5.0 | √ | √ | 10.0 | √ | x |
plain | 5.0 | √ | √ | 10.0 | √ | x |
button在元素的text区域直接写文字,和text组件一样。可以这么理解,button是一个特殊的text组件,文字样式可以直接写在button组件的style或class上。
button组件属性中的size和type,属于预置样式,方便开发者使用。开发者也可以通过style和class来自定义样式。
button虽然可以内嵌text组件,但不建议通过text组件来修改button样式,因为会导致hove-class不生效。尤其是uvue中样式不继承。建议button组件text区域直接写文字,然后在button组件的style或class属性编写样式。
style和class的优先级,高于size和type属性。
<template>
<button size="default" type="default"
style="color:#ffffff;backgroundColor:#1AAD19;borderColor:#1AAD19"
hover-class="is-hover">按钮</button>
</template>
<style>
.is-hover {
color: rgba(255, 255, 255, 0.6);
background-color: #179b16;
border-color: #179b16;
}
</style>
button 组件的点击遵循 vue 标准的 @click事件。
button 组件没有 url 属性,如果要跳转页面,可以在@click中编写,也可以在button组件外面套一层 navigator 组件。举例,如需跳转到about页面,可按如下几种代码写法执行:
<template>
<view>
<navigator url="/pages/about/about"><button>通过navigator组件跳转到about页面</button></navigator>
<button @click="goto('/pages/about/about')">通过方法跳转到about页面</button>
</view>
</template>
<script>
export default {
methods: {
goto(url:string) {
uni.navigateTo({
url:url
})
}
}
}
</script>
\n
方式换行,会直接显示 \n
字符。微信小程序下 \n
会变成一个空格