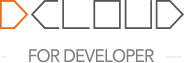
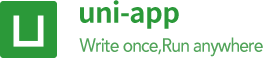
English
This plugin is used to run uni-app automated tests in HBuilderX, and supports H5, WeChat applet, android, ios automated tests.
The main functions are:
uni-app common project
and uniapp-cli project
. For the uniapp-cli project, to run automated tests, you need to install automated test dependencies under the current project.ios phone
.iOS模拟器
,不支持ios真机,测试iOS模拟器,需要电脑装安装XCode。chrome
浏览器,不支持其它浏览器。directly
run the test to the currently connected device. When you have multiple
devices, a pop-up window will ask you to select a phone.built-in node
. On the contrary, if the node is installed on the machine, the node of the machine is used.As shown in the figure below, in the plug-in market, enter the [plug-in details page] (https://ext.dcloud.net.cn/plugin?id=5708), click [Import Plug-in], and the locally installed HBuilderX will be automatically launched.
Special Note: Plug-in installation depends on HBuilderX terminal plug-in.
插件依赖:
puppeteer
, adbkit
, node-simctl
, jest
, playwright
. When running the plugin, if this dependency is not installed, a pop-up window will be installed automatically.Note
: This plug-in version 0.0.3 and below, node: When node is not installed on this machine, the built-in node of HBuilderX will be used to run the test. On the contrary, if the node is installed on the machine, the node of the machine is used.Note
: This plugin version 0.0.4+, new configuration items support custom settings which node version to use for uni-app compilationpay attention:
hbuilderx-for-uniapp-test
.For uni-app ordinary projects, when initializing the test environment
or running the test
, if the relevant dependencies are not installed, they will be installed automatically.
As shown in the figure below, the project manager, select the project, right-click menu [initialize the test environment]
注意:安装环境依赖时,如果检测到项目下不存在测试配置文件 env.js
和jest.config.js,则会自动创建测试配置文件。
The uniapp-cli project, automated test running, will use the dependent library under the project.
Open the command line, enter the project directory, and enter the following command to install:
npm install --save cross-env puppeteer adbkit node-simctl jest playwright @playwright/test
uni-app project, pages page, right-click menu, create test cases
After creating a test case, select the project, right-click the menu [Run uni-app automated test], and select the running platform to start running the test.
Note: If you want to run the specified test case, please select the use case to be run in the project manager, right-click menu [Run current test case]
ios phone
ios simulator
, does not support ios real machine.chrome
browser, does not support other browsers.As shown in the figure below, when running the test, you can select the corresponding platform.
如果无法获取到设备信息,请参考
Click the menu [Settings] [Plugin Configuration], find the hbuilderx-for-uniapp-test item, and you can see the setting items.
As pictured above
true
. After removing the check, testMatch will no longer be automatically modified.built-in Node
, or use operating system
installed Node for uni-app compilation.uni-app automated testing, using the common jest testing library in the industry.
The following will use a simplest example to explain the composition of test cases.
# 求和测试
function sum(a, b) {
return a + b;
};
describe("sum test", () => {
it('adds 1 + 2 to equal 3', () => {
expect(sum(1, 2)).toBe(3);
});
test('adds 1 + 1 to equal 3', () => {
expect(sum(1, 1)).toBe(3);
});
})
Take the uni-app [default template] index page as an example.
Write a test case that checks the index.vue
page, if the title is Hello
describe('test title', () => {
let page;
beforeAll(async () => {
page = await program.currentPage();
await page.waitFor(3000);
});
it('check page title', async () => {
const el = await page.$('.title');
const titleText = await el.text();
expect(titleText).toEqual('Hello');
});
});
扩展:如上测试代码中,使用了beforeAll
函数,它用于在所有测试之前执行。了解jest更多钩子函数
Often when writing tests, you need to do some setup work before the test runs, and some finishing work after the test runs. You can use Jest's hook function to solve this problem.
4 hook functions in jest
Documentation extension: jest setup-teardown
Using the following code, let's see the function execution order
describe('test Run Sequence', () => {
beforeAll(() => {
console.log('1 - beforeAll');
});
afterAll(() => {
console.log('1 - afterAll');
});
beforeEach(() => {
console.log('1 - beforeEach');
});
afterEach(() => {
console.log('1 - afterEach');
});
test('test', () => {
console.log('1 - test')
});
});
operation result
test Run Sequence
✓ test (4 ms)
console.log
1 - beforeAll
console.log
1 - beforeEach
console.log
1 - test
console.log
1 - afterEach
console.log
1 - afterAll
Test Suites: 1 passed, 1 total
Tests: 1 passed, 1 total
Snapshots: 0 total
Time: 0.454 s
In order to write test cases more quickly, this plugin has built-in jest part of the code block
prefix | code block |
---|---|
describe | describe('', () => {}); |
test | test('', () => {}); |
ta | test('', async () => {await}); |
beforeAll | beforeAll(() => {}); |
afterEach | afterEach(() => {}); |
afterAll | afterAll(() => {}); |
beforeAll | beforeAll(() => {}); |