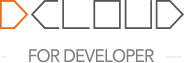
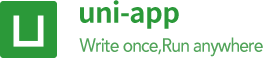
English
Form, <switch>
<input>
<checkbox>
<slider>
<radio>
``within the component
Submit.
When clicking the <button>
component whose formType is submit in the <form>
form, the value in the form component will be submitted, and name needs to be added to the form component as the key.
Attribute Description
Property Name | Type | Description | Platform Difference Description |
---|---|---|---|
report-submit | Boolean | Whether to return formId for sending template message | WeChatMiniApp, Alipay MiniApp |
report-submit-timeout | number | Wait for a period of time (in milliseconds) to confirm whether the formId is valid. If this parameter is not specified, the formId has a small probability of being invalid (such as in the case of a network failure). Specify this parameter to detect whether the formId is valid, and use the time of this parameter as the timeout of this detection. If it fails, it will return the formId starting with requestFormId:fail | WeChat MiniApp 2.6.2 |
@submit | EventHandle | Carry the data in the form to trigger the submit event, event.detail = {value : {'name': 'value'} , formId: ''}, formId will be returned only when report-submit is true | |
@reset | EventHandle | The reset event will be triggered when the form is reset |
Example View Demo
The following sample code comes from hello uni-app project. It is recommended to use HBuilderX to create a new uni-app project and select the hello uni-app template to directly experience the complete example.
Template
Script
Style
<!-- This example does not contain the complete css, please refer to the above to get the external link css, check it in the hello uni-app project -->
<template>
<view>
<view>
<form @submit="formSubmit" @reset="formReset">
<view class="uni-form-item uni-column">
<view class="title">switch</view>
<view>
<switch name="switch" />
</view>
</view>
<view class="uni-form-item uni-column">
<view class="title">radio</view>
<radio-group name="radio">
<label>
<radio value="radio1" /><text>选项一</text>
</label>
<label>
<radio value="radio2" /><text>选项二</text>
</label>
</radio-group>
</view>
<view class="uni-form-item uni-column">
<view class="title">checkbox</view>
<checkbox-group name="checkbox">
<label>
<checkbox value="checkbox1" /><text>选项一</text>
</label>
<label>
<checkbox value="checkbox2" /><text>选项二</text>
</label>
</checkbox-group>
</view>
<view class="uni-form-item uni-column">
<view class="title">slider</view>
<slider value="50" name="slider" show-value></slider>
</view>
<view class="uni-form-item uni-column">
<view class="title">input</view>
<input class="uni-input" name="input" placeholder="这是一个输入框" />
</view>
<view class="uni-btn-v">
<button form-type="submit">Submit</button>
<button type="default" form-type="reset">Reset</button>
</view>
</form>
</view>
</view>
</template>
Use built-in behaviors
When the MiniApp has an input
form control in a custom component in form
, or implements a form control with a common label, such as score
, etc., it cannot get the component in the submit
event of form
In the form control value, you can use behaviors
at this time.
For form components, the following built-in behaviors are currently automatically recognized:
uni://form-field
Currently only supports WeChat MiniApp, QQ MiniApp, Baidu MiniApp, and h5.
uni://form-field
Make custom components behave like form controls. The form component can recognize these custom components, and return the component's field name and its corresponding field value in the submit event. This will add the following two properties to it.
Attribute Name | Type | Description |
---|---|---|
name | String | Field name in the form |
value | any | field value in the form |
Examples are as follows:
<!-- /pages/index/index.vue -->
<template>
<view class="content">
<form @submit="onSubmit">
<compInput name="test" v-model="testValue"></compInput>
<button form-type="submit">Submit</button>
</form>
</view>
</template>
<script>
export default {
data() {
return {
testValue: 'Hello'
}
},
methods: {
onSubmit(e) {
console.log(e)
}
}
}
</script>
Vue2
Vue3
<!-- /components/compInput/compInput.vue -->
<template>
<view>
<input name="test" style="border: solid 1px #999999;height: 80px;" type="text" @input="onInput" :value="value" />
</view>
</template>
<script>
export default {
name: 'compInput',
behaviors: ['uni://form-field'],
data(){
return{
value:""
}
},
methods: {
onInput(e) {
this.$emit('input', e.detail.value)
}
}
}
</script>
Enhanced uni-forms components
<uni-forms>
component, reference: [https://ext.dcloud.net.cn/plugin?id=2773](https://ext.dcloud. net.cn/plugin?id=2773)DB Schema
, configure the format of the field in the schema, the front-end form validation and server input parameter validation will be able to reuse the rules, without the need to repeatedly develop form validation in the front-end and back-end. See detailsDB Schema
of uniCloud, you can automatically generate a full set of forms, including interface, verification logic, and submit to storage, see details.