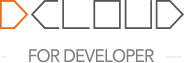
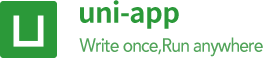
English
uni-app 内置了 Pinia 。Vue 2 项目暂不支持
注意事项
使用 HBuilder X
HBuilder X 已内置了 Pinia,无需手动安装,按照下方示例使用即可。
App 升级时,如果之前使用 HBuilder X 版本 < 4.14
打包,现在使用 HBuilder X 版本 >= 4.14
,更新时需要整包更新不可使用wgt更新(在 4.14
时升级了 vue
版本,低版本的基座和高版本 wgt 资源包会导致使用 Pinia 时报错)
使用 CLI
4.14 之前
:执行 yarn add pinia@2.0.36
或 npm install pinia@2.0.36
安装,要固定版本
4.14 之后
:执行 yarn add pinia
或 npm install pinia
安装,可不指定版本
Pinia (pronounced /piːnjʌ/
, like peenya
in English) is a repository for Vue that allows you to share state across components, pages. On the server side as well as in small single page applications, you can also get a lot of benefits from using Pinia:
├── pages
├── static
└── stores
└── counter.js
├── App.vue
├── main.js
├── manifest.json
├── pages.json
└── uni.scss
Write the following code in main.js
:
import { createSSRApp } from 'vue';
import * as Pinia from 'pinia';
export function createApp() {
const app = createSSRApp(App);
app.use(Pinia.createPinia());
return {
app,
Pinia, // 此处必须将 Pinia 返回
};
}
首先创建一个 Store:
// stores/counter.js
import { defineStore } from 'pinia';
export const useCounterStore = defineStore('counter', {
state: () => {
return { count: 0 };
},
// state: () => ({ count: 0 })
actions: {
increment() {
this.count++;
},
},
});
and then use it in the component:
<script setup>
import { useCounterStore } from '@/stores/counter'
const counter = useCounterStore()
counter.count++
// 自动补全! ✨
counter.$patch({ count: counter.count + 1 })
// 或使用 action 代替
counter.increment()
</script>
<template>
<!-- 直接从 store 中访问 state -->
<div>Current Count: {{ counter.count }}</div>
</template>
为实现更多高级用法,你甚至可以使用一个函数 (与组件 setup()
类似) 来定义一个 Store:
export const useCounterStore = defineStore('counter', () => {
const count = ref(0);
function increment() {
count.value++;
}
return { count, increment };
});
如果你还不熟悉 setup() 函数和组合式 API,Pinia 也提供了一组类似 Vuex 的 映射 state 的辅助函数。
你可以用和之前一样的方式来定义 Store,然后通过 mapStores()、mapState() 或 mapActions() 访问:
const useCounterStore = defineStore('counter', {
state: () => ({ count: 0 }),
getters: {
double: (state) => state.count * 2,
},
actions: {
increment() {
this.count++
},
},
})
const useUserStore = defineStore('user', {
// ...
})
// 使用
import { mapState, mapStores, mapActions } from 'pinia'
export default defineComponent({
computed: {
// ...
// 允许访问 this.counterStore 和 this.userStore
...mapStores(useCounterStore, useUserStore)
// 允许读取 this.count 和 this.double
...mapState(useCounterStore, ['count', 'double']),
},
methods: {
// 允许读取 this.increment()
...mapActions(useCounterStore, ['increment']),
},
})