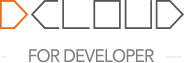
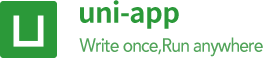
English
what is uts
uts, the full name of uni type script, is a cross-platform, high-performance, strongly typed modern programming language.
It can be compiled into programming languages for different platforms, such as:
uts adopts the same syntax specification as ts and supports most ES6 APIs.
But in order to cross-end, uts has some constraints and platform-specific additions.
In the past, most of the supported syntaxes that ran under the js engine can also be smoothly used in kotlin and swift under the processing of uts. But there are some that cannot be smoothed out and require the use of conditional compilation. Similar to the conditional compilation of uni-app, uts also supports conditional compilation. Written in conditional compilation, you can call platform-specific extended syntax.
uts是一门语言,对标的是js。目前DCloud还未发布基于uts的ui开发框架。所以现阶段使用uts开发ui是很不方便的(就像没有组件和css,拿js开发界面,还不能跨端)。
现阶段uts适合的场景是开发uni-app的原生插件。因为uts可以直接调用Android和iOS的原生API或jar等库。
很快DCloud会推出基于uts的跨平台响应式ui框架uvue
,届时开发者可使用vue语法、uni-app的组件和api,来开发纯原生应用,因为它们都被编译为kotlin和swift,不再有js和webview。未来大概的写法参考hello uni-app x (此项目还未正式发布,公开版的HBuilderX不能编译此项目,仅供参考写法)
This article is an introduction to the basic syntax of uts. If you want to know how to develop uts plug-ins under uni-app, see the document [https://uniapp.dcloud.net.cn/plugin/uts-plugin.html](https://uniapp.dcloud.net.cn/plugin /uts-plugin.html).
js is typeless, TypeScript's type means type, adding a type to js. Its type definition is defined by adding a colon and type after the variable name.
Variables declared in uts can use let or const, see below for details.
Declare a reassignable variable. Syntax let [variable name] : [type] = value;
.
Equivalent to let in TypeScript, var in kotlin
let str :string = "hello"; // 声明一个字符串变量
str = "hello world"; // 重新赋值
In addition to string, more types see below
Declare a read-only constant that can only be assigned a value once. Syntax const [variable name] : [type] = value;
.
Equivalent to const in TypeScript, val in kotlin
const str :string = "hello"; // 声明一个字符串变量
str = "hello world"; // 报错,不允许重新赋值
Precautions:
let str:string
and let str : string
and let str :string
and let str: string
are both valid.In uts, there are certain rules for using variable names.
Note: Unlike TypeScript, uts does not allow variables starting with $
uts has automatic type deduction. If you assign a value directly when defining a variable without using a colon to define the type, it can also run legally.
The following two ways of writing are legal, and both variables are of string type:
let s1 :string = "hello";
let s2 = "hello";
If a variable is defined without a declared type, it is not assigned a value. Then this variable will be regarded as any type. Although it can be used, it is highly discouraged in uts.
let s;
s = "123"
console.log(s) // hello world
name | shorthand operator | meaning |
---|---|---|
Assignment | x = y | x = y |
Addition assignment | x += y | x = x + y |
Subtraction assignment | x -= y | x = x - y |
Multiplication assignment | x *= y | x = x * y |
Division assignment | x /= y | x = x / y |
Remainder assignment | x %= y | x = x % y |
Left shift assignment | x <<= y | x = x << y |
Right shift assignment | x >>= y | x = x >> y |
Unsigned right shift assignment | x >>>= y | x = x >>> y |
Bitwise AND assignment | x &= y | x = x & y |
Bitwise XOR assignment | x ^= y | x = x ^ y |
Bitwise OR assignment | x |= y | x |= y |
operator | description | examples that return true |
---|---|---|
Equal to Equal (==) | Returns true if both operands are equal. | var1==var2 |
不等于 Not equal (!=) | 如果两边操作数不相等时返回 true | var1!=var2 |
引用相等 Reference equal (===) | 两边操作数指向同一个对象返回 true。 | var1===var2 |
Greater than (>) | The left operand is greater than the right operand returns true | var1>var2 |
Greater than or equal (>=) | The left operand is greater than or equal to the right operand returns true | var1>=var2 |
Less than (<) | The left operand is less than the right operand returns true | var1<var2 |
Less than or equal (<=) | Left operand less than or equal to right operand returns true | var1<=var2 |
Operator | Example | Description |
---|---|---|
Remainder (%) | Binary operator. Returns the remainder after division. | |
Increment (++) | Unary operator. Increments the value of the operand by one. If placed before the operand (++x), returns the value after adding one; if placed after the operand (x++) , returns the original value of the operand, and then increments the operand by one. | |
Decrement (--) | Unary operator. Decrements the value of the operand by one. The return value of the two usages of prefix and suffix is similar to the increment operator. |
Operator | Usage | Description |
---|---|---|
Bitwise AND | a & b | In the bit representation of a,b, if each corresponding bit is 1, return 1, otherwise return 0. |
Bitwise OR | a | b | In the bit representation of a,b, if each corresponding bit is 1, it returns 1, otherwise it returns 0. |
Bitwise XOR | a ^ b | In the bit representation of a,b, for each corresponding bit, if the two are different, it returns 1, and if they are the same, it returns 0. |
Bitwise NOT NOT | ~ a | Inverts the bits of the operand. |
shift left | a << b | Shift the binary string of a to the left by b bits and to the right by 0. |
Arithmetic right shift | a >> b | Shift the binary representation of a to the right by b bits, discarding all the shifted bits. ) |
Unsigned right shift (left vacant bits are filled with 0s) | a >>> b | Shift the binary representation of a to the right by b bits, discarding all shifted bits, and filling the left vacant bits with 0s |
Operator | Example | Description |
---|---|---|
logical AND (&&) | expr1 && expr2 | (logical AND) |
Logical OR (||) | expr1 || expr2 | (Logical OR) |
Logical NOT (!) | !expr | (Logical NOT) |
In addition to comparison operators, which can be used on string values, the concatenation operator (+) concatenates two string values, returning another string, which is the union of the two operand strings.
console.log("my " + "string"); // console logs the string "my string".
注意
在iOS平台,连接操作符(+)目前仅支持字符串的连接,即+操作符前后都必须是字符串类型。
The conditional operator is the only operator in uts that requires three operands. The result of the operation takes one of two values according to a given condition. The syntax is:
condition ? value1 : value2
const status = age >= 18 ? "adult" : "minor";
Multiple code statements of uts can be separated by carriage return or semicolon. The semicolon at the end of the line can be omitted. If written on one line, they should be separated by semicolons.
The following code is legal:
let a:number = 1 //行尾可以不加分号
let b:boolean = false; //行尾可以加分号
let c:number = 3 ; let d:number = 4 // 同行多语句需要用分号分割
There are 2 values: true
and false
.
All numbers, integer or floating point, both positive and negative. For example: positive integer 42
or floating point 3.14159
or negative -1
.
let a:number = 42
Notice
In kotlin and swift, the input and return of some system APIs or third-party SDKs forcefully agree on these platform number types, and number cannot be used at this time. In this case, the following method can be used. Although the editor may report syntax errors (subsequent HBuilderX will fix such false positives), it is available when compiling to kotlin and swift.
At present, these platform number types, when declaring the type, are different from number in that the first letter is capitalized
let a:Int = 3 //注意 Int 是首字母大写
let b:Int = 4
let c:Double = a * 1.0 / b
let a:Int = 3
a.toFloat() // 转换为 Float 类型,后续也将支持 new Float(a) 方式转换
a.toDouble() // 转换为 Double 类型,后续也将支持 new Double(a) 方式转换
let a:Int = 3
let b = new Double(a) // 将整型变量 a 转换为 Double 类型
边界情况说明:
Infinity
或 -Infinity
。The value is out of range
。integer literal overflows when stored into Int
。A string is a sequence of characters representing a text value, for example: "hello world"
.
边界情况说明:
Invalid string length
;在 JSCore 中,最大长度为 2^31 - 1,超出限制会报错:Out of memory __ERROR
。java.lang.OutOfMemoryError: char[] of length xxx would overflow
。A date object represents a date, including various dates such as year, month, day, hour, minute, and second. Details see below
A special keyword that indicates a null value.
Sometimes you need to define a nullable string, you can use the |
operator in the type description.
let user: string | null
Note: undefined is not supported when compiling uts to kotlin and swift.
Object (object) refers to an area in memory that can be referenced by an identifier, and is a type of reference. Including Array, Date, Map, Set, JSON, etc., uts has a standard library of built-in objects. Details see below.
Undefined type, i.e. any type. Generally not recommended.
A literal is a constant defined by a grammatical expression; or, a constant defined by a lexical expression consisting of certain words.
In uts, you can use various literals. These literals are fixed values given literally, not variables
An array literal is a list of zero or more expressions enclosed in a pair of square brackets ([]), where each expression represents an element of the array. When you create an array using an array literal, the array is initialized with the specified value as its elements, and its length is set to the number of elements.
The following example generates the array coffees with 3 elements and its length is 3.
const coffees = ["French Roast", "Colombian", "Kona"]
Array literals are also array objects.
The boolean type has two literals: true and false.
Numeric literals include integer literals in multiple bases and floating-point literals in base 10
Integers can be represented in decimal (base 10), hexadecimal (base 16), or binary (base 2).
Decimal integer literals consist of a sequence of numbers without a prefix of 0. For example: 0, 117, -345
Hexadecimal integers start with 0x (or 0X) and can contain numbers (0-9) and letters a~f or A~F. Such as: 0x1123, 0x00111 , -0xF1A7
Binary integers start with 0b (or 0B) and can only contain the digits 0 and 1. For example: 0b11, 0b0011 , -0b11
A floating-point number literal can have the following components:
The exponent part starts with "e" or "E", followed by an integer, which can be signed (i.e. prefixed with "+" or "-"). Floating-point literals have at least one digit, and must have a decimal point or "e" (or uppercase "E").
In short, its syntax is:
[(+|-)][digits][.digits][(E|e)[(+|-)]digits]
E.g:
3.14
-.2345789 // -0.23456789
-3.12e+12 // -3.12*10^12
.1e-23 // 0.1*10^(-23)=10^(-24)=1e-24
Regular expressions are expressions in which characters are surrounded by slashes. Below is an example of a regular expression literal.
const re = /ab+c/;
A string literal is zero or more characters enclosed in double quotation marks (") pairs or single quotation marks ('). Strings are bounded between quotation marks of the same kind; that is, they must be pairs of single quotation marks or single quotation marks ('). Double quotes. The following examples are all string literals:
"foo"
'bar'
"1234"
"one line \n another line"
"John's cat"
You can use all the methods of string objects on string literals, and you can also use properties like String.length on string literals:
console.log("John's cat".length)
// will print the number of characters in the string (including spaces)
// result is: 10
Template literals are string literals that allow embedded expressions. You can use multiline strings and string interpolation functions. Also known as "template strings".
// Basic literal string creation
`In uts '\n' is a line-feed.`
// Multiline strings
`In uts this is
not legal.`
// String interpolation
var name = "Bob", time = "today";
`Hello ${name}, how are you ${time}?`
character | meaning |
---|---|
\b | Backspace |
\f | Form feed |
\n | Newline |
\r | carriage return |
\t | Tab |
\' | Single quotes |
\" | Double quotes |
\\ | backslash character |
Use the if statement to execute a statement when a logical condition is true. When the condition is false, use the optional else clause to execute the statement. The if statement looks like this:
if (condition_1) {
statement_1;
} else if (condition_2) {
statement_2;
} else if (condition_n_1) {
statement_n;
} else {
statement_last;
}
Note: Conditional expressions in if and else if must be boolean
The switch statement allows a program to evaluate an expression and try to match the expression's value to a case label. If the match is successful, the program executes the associated statement. The switch statement looks like this:
switch (expression) {
case label_1:
statements_1
[break;]
case label_2:
statements_2
[break;]
default:
statements_def
[break;]
}
The program first looks for a case statement that matches expression, then transfers control to that clause, executing the associated statement. If there is no match, the program looks for the default statement, and if it finds it, control transfers to that clause and the associated statement is executed. If no default is found, the program continues with the statement following the switch statement. The default statement usually appears at the end of the switch statement, although this is not required.
An optional break statement is associated with each case statement, ensuring that the program can break out of the switch after the matching statement has been executed and continue executing the statement following the switch. If break is ignored, the program will continue to the next statement in the switch statement.
uts supports the use of ternary expressions. A condition is followed by a question mark (?), if the condition is true, the expression A following the question mark will be executed; expression A is followed by a colon (😃, if the condition is false, the expression B following the colon will be executed will execute. This operator is often used as a shorthand for an if statement.
function getFee(isMember: boolean): string {
return isMember ? "$2.00" : "$10.00";
}
console.log(getFee(true));
// expected output: "$2.00"
console.log(getFee(false));
// expected output: "$10.00"
console.log(getFee(null));
// expected output: "$10.00"
The ternary operator is right-associative, which means you can chain it like this, similar to an if … else if … else if … else chain:
function example(): string {
return condition1
? value1
: condition2
? value2
: condition3
? value3
: value4;
}
// Equivalent to:
function example(): string {
if (condition1) {
return value1;
} else if (condition2) {
return value2;
} else if (condition3) {
return value3;
} else {
return value4;
}
}
A for loop executes repeatedly until the specified loop condition is false. A for statement looks like this:
for ([initialExpression]; [condition]; [incrementExpression]) {
statement;
}
When a for loop executes, the following process occurs:
Example:
for (let i = 0; i < 10; i++) {
//...
}
The do...while statement repeats until the specified condition evaluates to false. A do...while statement looks like this:
do {
statement;
} while (condition);
statement is executed once before checking the condition. To execute multiple statements (statement blocks), enclose them with block statements ({ ... }). If the condition is true, the statement will execute again. Conditional checks are performed at the end of each execution. When condition is false, execution stops and returns control to the statement following do...while.
Example:
let i = 0;
do {
i += 1;
} while (i < 10);
A while statement executes its block as long as the specified condition evaluates to true. A while statement looks like this:
while (condition) {
statement;
}
If this condition becomes false, the statement inside the loop will stop executing and return control to the code following the while statement.
Condition testing occurs before each statement execution. If the condition returns true, the statement is executed and the condition is tested again immediately afterward. If the condition returns false, execution stops and returns control to the statement following the while.
To execute multiple statements (statement blocks), enclose them with statement blocks ({ ... }).
Example:
let n = 0;
let x = 0;
while (n < 3) {
n++;
x += n;
}
Use the break statement to terminate the loop, switch.
Example:
for (let i = 0; i < 10; i++) {
if (i > 5) {
break;
}
}
let x = 0;
while (true) {
x++;
if (x > 5) {
break;
}
}
Use the continue statement to terminate the current loop and continue the loop on the next iteration.
Example:
for (let i = 0; i < 10; i++) {
if (i > 5) {
continue;
}
}
let x = 0;
while (true) {
x++;
if (x > 5) {
continue;
}
}
You can throw an exception with the throw statement and catch it with the try...catch statement.
Use throw expressions to throw exceptions:
throw new Error("Hi There!");
Use try...catch expressions to catch exceptions:
try {
// some code
} catch (e: Error) {
// handler
} finally {
// optional finally block
}
A function is a common function in programming languages. It can encapsulate a batch of code, receive external parameters, and return a value. The encapsulated logic can be called by different other codes to achieve the purpose of common reuse logic.
Functions are defined with the function keyword, followed by the function name and parentheses.
Also note that defining functions involves scope.
A function definition (also known as a function declaration, or a function statement) consists of a series of content after the function keyword, in order:
Note: The function must clearly indicate the return value type
For example, the following code defines a simple function. The function name is add, there are two parameters x and y, both of type string, and the return value type of the function is also string.
The content of the function is to add the input parameters x and y, assign it to the variable z, and then return z through the return keyword.
function add(x :string, y :string) :string {
let z : string = x + " " + y
return z;
}
If this function does not need to return a value, you need to use the void keyword, and at the same time, there is no need to return at the end of the function to return the content.
function add(x :string, y :string) :void {
let z :string = x + " " + y
console.log(z)
// no need to return
}
While the function declaration above is syntactically a statement, functions can also be created from function expressions. Such a function can be anonymous, it doesn't have to have a name. For example, the function add could also be defined like this:
const add = function (x: string, y: string): string {
return x + " " + y;
};
Notice:
const add = function add(){}
is not allowed.Defining a function does not automatically execute it. Defining a function is just a matter of giving the function a name and specifying what the function should do when called. Calling the function will actually perform the actions with the given parameters.
After defining the function add, you can call it like this:
function add(x :string, y :string) :string {
let z :string = x + " " + y
return z;
}
add("hello", "world"); // 调用add函数
The above statement calls the function by providing the parameters "hello" and "world".
Although the add function was called, the return value was not obtained. If you need to get the return value, you need to reassign:
function add(x :string, y :string) :string {
let z :string = x + " " + y
return z;
}
let s :string = add("hello", "world");
console.log(s) // hello world
Variables defined inside a function cannot be accessed anywhere outside the function, because variables are only defined inside the function's domain. Correspondingly, a function can access any variable and function defined in its scope.
const hello :string = "hello";
const world :string = "world";
function add(): string {
let s1 :string = "123";
return hello + world; // 可以访问到 hello 和 world
}
You can nest a function inside another function. Nested (inner) functions are private to their container (outer) functions. It also forms a closure itself. A closure is an expression (usually a function) that can have its own independent environment and variables.
Since a nested function is a closure, it means that a nested function can "inherit" the parameters and variables of the container function. In other words, the inner function contains the scope of the outer function.
It can be summed up as follows:
Example:
function addSquares(a: number, b: number): number {
function square(x: number): number {
return x * x;
}
return square(a) + square(b);
}
addSquares(2, 3); // returns 13
addSquares(3, 4); // returns 25
addSquares(4, 5); // returns 41
A naming conflict occurs when two parameters or variables in the same closure scope have the same name. Closer scopes have higher precedence, so the closest has the highest priority and the farthest has the lowest. This is the scope chain. The first element of the chain is the innermost scope, and the last element is the outermost scope.
Example:
function outside(): (x: number) => number {
let x = 5;
const inside = function (x: number): number {
return x * 2;
};
return inside;
}
outside()(10); // 返回值为 20 而不是 10
The naming conflict occurs on return x, the inside parameter x and the outside variable x conflict. The scope here is {inside, outside}. So inside x has the highest priority, returning 20 (inside x) instead of 10 (outside x).
uts allows function nesting, and inner functions can access all variables and functions defined in outer functions, and all variables and functions that outer functions can access.
However, the outer function cannot access the variables and functions defined in the inner function. This provides some security to the variables of the inner function.
Also, since the inner function can access the scope of the outer function, when the inner function lifetime is longer than the outer function, the variables and functions defined in the outer function will have a longer lifetime than the inner function execution time. A closure is created when the inner function is accessed in some way by any of the outer function scopes.
Example:
const pet = function (name: string): () => string {
//The external function defines a variable "name"
const getName = function (): string {
//Inner function can access the "name" defined by the outer function
return name;
};
//Return this inner function, thereby exposing it in the outer function scope
return getName;
};
const myPet = pet("Vivie");
myPet(); // 返回结果 "Vivie"
Function parameters can have default values, which are used when the corresponding parameter is omitted.
function multiply(a:number, b:number = 1):number {
return a*b;
}
multiply(5); // 5
Arrow function expressions (also called fat arrow functions) have a shorter syntax than function expressions. Arrow functions are always anonymous.
const arr = ["Hydrogen", "Helium", "Lithium", "Beryllium"];
const a2 = arr.map(function (s): number {
return s.length;
});
console.log(a2); // logs [ 8, 6, 7, 9 ]
const a3 = arr.map((s): number => s.length);
console.log(a3); // logs [ 8, 6, 7, 9 ]
Classes are declared in uts using the keyword class.
A class declaration consists of a class name followed by a class body surrounded by curly braces.
// define Person Class
class Person {
}
A class is an object-oriented concept with properties, methods, and constructors.
object.property name
, or assigned by object.property name=xxx
.object. method name (parameter)
.In the following example, a Person class is defined, which has an attribute name, a constructor function (name cannot be changed), and a method getNameLength.
// define Person Class
class Person {
name:string = ""; // 属性name
constructor(newname:string) { // 构造函数,参数newname
console.log("开始实例化");
this.name = newname;
}
getNameLength():number{ // 方法getNameLength
return this.name.length
}
}
After the class is defined, it needs to be instantiated (via the new keyword). Once an instance is defined, the properties and methods of the instance object are available.
A class can be instantiated multiple times as different instances without affecting each other.
//Instantiate the class defined above and call its attribute method
let p = new Person("tom"); // 使用 new 关键字实例化对象时,会自动触发构造函数
console.log(p.name); // 访问p这个对象的属性name,返回值tom
console.log(p.getNameLength()); // 调用p这个对象的方法getNameLength,返回值3
let p2 = new Person("jerry"); // 使用 new 关键字再实例化一个新对象
console.log(p2.name); //jerry
console.log(p2.getNameLength()); //5
The constructor constructor is automatically executed when a new object is created (new), and is used to initialize object properties.
constructor([arguments]) { ... }
You don't have to write a constructor. If no constructor is explicitly specified, the runtime will automatically add a default constructor method.
There can be only one special method named "constructor" in a class. Multiple occurrences of a constructor method in a class will throw a SyntaxError .
class Person {
name:string = "";
constructor(newname:string) {
this.name = newname;
}
}
let person = new Person("tom"); // 使用 new 关键字创建对象时,会自动触发构造函数
console.log(person.name); // tom
You can use the super keyword in a constructor to call a superclass constructor. This involves the concept of inheritance. If you don't understand inheritance, you can see below
class Polygon {
constructor() {
this.name = "Polygon";
}
}
class Square extends Polygon {
constructor() {
super();
}
}
class has instance attributes and static attributes. Instance attributes in uts exist on every instance of the class.
uts can declare properties in the class, which are readable and writable by default.
class Person {
name:string = ""; // 声明实例属性name
city:string = "beijing" // 声明实例属性city
constructor(newname:string) {
this.name = newname; // 在构造函数中对name重新赋值
}
}
let person1 = new Person("tom"); // 使用 new 关键字创建对象时,会自动触发构造函数
console.log(person1.name); //tom
console.log(person1.city); //beijing
let person2 = new Person("jerry"); // 使用 new 关键字创建对象时,会自动触发构造函数
console.log(person2.name); //jerry
console.log(person2.city); //beijing
uts supports intercepting access to object properties through getters/setters. It can be understood as an interceptor for reading/writing attributes.
In the following example, the get and set interception of name is provided for the person object, and the value of name cannot be modified when the paascode is incorrect.
const passcode = "secret passcode";
class Person {
private _name: string = ""; // private是私有的,外部不能访问
get name(): string { // 读取name会触发此拦截器
console.log("start to get person.name");
return this._name;
}
set name(newName: string) { // 给name赋值会触发此拦截器
console.log("start to set person.name");
if (passcode === "secret passcode") { // 校验是否有权修改name的值,这里的条件可以修改以方便测试
this._name = newName;
} else {
console.log("Error: set person.name fail");
}
}
}
let p = new Person()
p.name = "tom" // 会打印"start to set person.name"
console.log(p.name); // 先打印"start to get person.name",然后打印"tom"
uts properties can be made read-only using the readonly keyword. Read-only properties must be initialized at declaration time or in the constructor.
class Person {
readonly name: string;
readonly age: number = 0;
constructor (theName: string) {
this.name = theName;
}
}
let p = new Person("tom");
console.log(p.name);
p.name = "jerry"; // 错误! name 是只读的
p.age = 1 // 错误! age 是只读的
But readonly is more of a syntax check for a development environment. At runtime, this value can often change.
Use the keyword static to declare a property as static. Static properties are not called on the instance, only by the class itself.
class Person {
static age:number = 10; // age是静态属性。不能在实例p中访问,但可以通过类Person访问
getAge():number{
return Person.age
}
}
console.log(Person.age); //10
let p = new Person(); //新建一个实例
console.log(p.getAge()); //10
Instance methods in uts exist in every instance of the class.
uts can declare instance methods in a class.
The following defines a class that calculates the area by multiplying the height by the width.
class Rectangle {
private height:number;
private width:number;
constructor(height: number, width: number) {
this.height = height;
this.width = width;
}
calcArea(): number {
return this.height * this.width;
}
}
To use an instance method, call it on an instance of the class:
const square = new Rectangle(10, 10);
square.calcArea(); // 100
Use the keyword static to declare a method as static. Static methods are not called on the instance, but only by the class itself. They are often utility functions, such as to create or copy objects.
class ClassWithStaticMethod {
static staticMethod(): string {
return "static method has been called.";
}
}
ClassWithStaticMethod.staticMethod(); // 不实例化,直接调用class的方法
uts allows the use of inheritance to extend existing classes. The extended subclass inherits the attribute methods of the parent class, but can add its own unique attribute methods and override the attribute methods defined by the parent class.
The inherited class is called the parent class (also called the super class, base class), and the newly extended class is called the subclass (also called the derived class).
For example, the Person class is defined to store the basic information of a person, and a Developer subclass can be defined to inherit from the Person class, and Developer's unique information can be added to the subclass.
class ChildClass extends ParentClass { ... }
The extends keyword is used to create a subclass of a class.
// define the parent class
class Person {
name:string = "";
constructor(newname:string) {
this.name = newname;
}
}
// define subclass
class Developer extends Person{
likeLanguage:string = "ts"
}
let d = new Developer("tom"); // 实例化。由于子类没有声明和复写自己的构造函数,所以默认继承了父类的构造函数
console.log(d.name); // tom
console.log(d.likeLanguage); // ts
Coverage, also known as duplication, rewriting. In inheritance, it is used to override the methods or properties defined by the parent class in the subclass.
uts requires the explicit modifier override for overridable and overridden members.
class Polygon {
name(): string {
return "Polygon";
}
}
class Square extends Polygon {
override name(): string {
return "Square";
}
}
The override modifier must be added to the Square.name function. If not written, the compiler will report an error.
Properties have the same overriding mechanism as methods. Properties with the same name that have been declared in the parent class must start with override in the subclass, and they must have compatible types (both are strings, or numbers, Boolean values, etc.).
class Shape {
vertexCount: Int = 0
}
class Rectangle extends Shape {
override vertexCount = 4
}
Code in a subclass can call methods of its parent class using the super keyword. The method of the parent class (grandfather class) of the parent class cannot be called across levels.
class Rectangle {
draw() {}
}
class FilledRectangle extends Rectangle {
override draw() {
super.draw();
}
}
Both methods and properties of a class can have visibility modifiers.
There are three visibility modifiers in uts: private, protected, and public. The default visibility is public.
The public members defined in the program can be freely accessed in uts, which is also the default behavior of uts.
When a member is marked private, it cannot be accessed outside the class in which it is declared. for example:
class Person {
private name: string = "Cat";
}
new Person().name; // 错误: 'name' 是私有的.
The protected modifier behaves much like the private modifier, but with one difference, protected members are still accessible in inherited derived classes. for example:
class Person {
protected name: string;
constructor(name: string) {
this.name = name;
}
}
class Employee extends Person {
private department: string;
constructor(name: string, department: string) {
super(name);
this.department = department;
}
public getElevatorPitch(): string {
return `Hello, my name is ${this.name} and I work in ${this.department}.`;
}
}
const howard = new Employee("Howard", "Sales");
console.log(howard.getElevatorPitch());
console.log(howard.name); // 错误
Note that we cannot use the name outside of the Person class, but we can still access it through the instance methods of the Employee class, since Employee is derived from Person.
uts supports splitting programs into separate modules that can be imported on demand, where various types of variables can be imported and exported, such as functions, strings, numbers, booleans, classes, etc.
The export statement can export functions, classes, etc. in a file. for example:
export const name: string = "square";
export function draw() {}
export default class Canvas {} // default 关键词支持默认导出
The import statement can import functions, classes, etc. from another file into the current file. for example:
import { name as name1, draw } from "./canvas.uts" // 支持 as 语法做别名导入
import * as Utils from "./utils.uts" // Test 包含所有 export 的导出
import Canvas from "./canvas.uts" // 对应 export default 的导出
Example
/*-----export [test.js]-----*/
export const name = 'test'
export function test(){
console.log('test')
}
export default class Test{
test(){
console.log('Test.test')
}
}
import { name } from './test.uts'
import * as testModule from './test.uts'
import Test from './test.uts'
console.log(name)
testModule.test()
const test = new Test()
test.test()
可以使用 export 语句将变量或函数导出,以便其他模块可以访问和使用它们。导出的变量可以在模块内共享,并在其他模块中导入和复用。
示例
/*-----export [global.uts]-----*/
// 导出变量
export let count = 1
// 导出函数
export function addCount() {
count++
}
// module1.uts
import { count, addCount } from './global.uts'
console.log(count) // 1
addCount()
console.log(count) // 2
// module2.uts
import { count, addCount } from './global.uts'
console.log(count) // 2
uts has a collection of built-in objects. Regardless of compiling uts to js/kotlin/swfit, these built-in objects can be used cross-platform.
print debug log in console
console.debug(msg1, msg2, msg3)
print error log in console
console.error(msg1, msg2, msg3)
print info log in console
console.info(msg1, msg2, msg3)
print log in console
console.log(msg1, msg2, msg3)
print warn log in console
console.warn(msg1, msg2, msg3)
Array objects are global objects used to construct arrays, which are higher-order objects similar to lists.
the number of elements in the array
const clothing = ['shoes', 'shirts', 'socks', 'sweaters'];
console.log(clothing.length);
// expected output: 4
边界情况说明:
Invalid array length
。java.lang.OutOfMemoryError: Failed to allocate a allocation until OOM
。The concat() method is used to combine two or more arrays. This method does not change the existing array, but returns a new array.
const array1 = ['a', 'b', 'c'];
const array2 = ['d', 'e', 'f'];
const array3 = array1.concat(array2);
console.log(array3);
// expected output: Array ["a", "b", "c", "d", "e", "f"]
The copyWithin() method shallowly copies part of an array to another location in the same array and returns it without changing the length of the original array.
const array1 = ['a', 'b', 'c', 'd', 'e'];
// copy to index 0 the element at index 3
console.log(array1.copyWithin(0, 3, 4));
// expected output: Array ["d", "b", "c", "d", "e"]
// copy to index 1 all elements from index 3 to the end
console.log(array1.copyWithin(1, 3));
// expected output: Array ["d", "d", "e", "d", "e"]
The every() method tests whether all elements in an array pass the test of a specified function. It returns a boolean value.
const isBelowThreshold = (currentValue:number):boolean => currentValue < 40;
const array1 = [1, 30, 39, 29, 10, 13];
console.log(array1.every(isBelowThreshold));
// expected output: true
The fill() method fills all elements in an array from the start index to the end index with a fixed value. Termination index is not included.
const array1 = [1, 2, 3, 4];
// fill with 0 from position 2 until position 4
console.log(array1.fill(0, 2, 4));
// expected output: [1, 2, 0, 0]
// fill with 5 from position 1
console.log(array1.fill(5, 1));
// expected output: [1, 5, 5, 5]
console.log(array1.fill(6));
// expected output: [6, 6, 6, 6]
The filter() method creates a new array containing all the elements of the test implemented by the provided function.
const words = ['spray', 'limit', 'elite', 'exuberant', 'destruction', 'present'];
const result = words.filter((word:string):boolean => word.length > 6);
console.log(result);
// expected output: Array ["exuberant", "destruction", "present"]
The find() method returns the value of the first element in the array that satisfies the provided test function.
const array1 = [5, 12, 8, 130, 44];
const found = array1.find((element:number):boolean => element > 10);
console.log(found);
// expected output: 12
The findIndex() method returns the index of the first element in the array that satisfies the provided test function. Returns -1 if no corresponding element is found.
const array1 = [5, 12, 8, 130, 44];
const isLargeNumber = (element:number):boolean => element > 13;
console.log(array1.findIndex(isLargeNumber));
// expected output: 3
The flat() method recursively traverses the array to a specified depth, and returns all elements combined with the elements in the traversed subarrays into a new array.
const arr1 = [0, 1, 2, [3, 4]];
console.log(arr1.flat());
// expected output: [0, 1, 2, 3, 4]
const arr2 = [0, 1, 2, [[[3, 4]]]];
console.log(arr2.flat(2));
// expected output: [0, 1, 2, [3, 4]]
The forEach() method executes the given function once for each element of the array.
const array1 = ['a', 'b', 'c'];
array1.forEach(element => console.log(element));
// expected output: "a"
// expected output: "b"
// expected output: "c"
The includes() method is used to determine whether an array contains a specified value. According to the situation, it returns true if it contains, otherwise it returns false.
const array1 = [1, 2, 3];
console.log(array1.includes(2));
// expected output: true
const pets = ['cat', 'dog', 'bat'];
console.log(pets.includes('cat'));
// expected output: true
console.log(pets.includes('at'));
// expected output: false
The indexOf() method returns the first index in the array at which a given element can be found, or -1 if it does not exist.
const beasts = ['ant', 'bison', 'camel', 'duck', 'bison'];
console.log(beasts.indexOf('bison'));
// expected output: 1
// start from index 2
console.log(beasts.indexOf('bison', 2));
// expected output: 4
console.log(beasts.indexOf('giraffe'));
// expected output: -1
The join() method joins all elements of an array into a string and returns the string. If the array has only one item, then that item is returned without a delimiter.
const elements = ['Fire', 'Air', 'Water'];
console.log(elements.join());
// expected output: "Fire,Air,Water"
console.log(elements.join(''));
// expected output: "FireAirWater"
console.log(elements.join('-'));
// expected output: "Fire-Air-Water"
The lastIndexOf() method returns the index of the last specified element in the array, or -1 if it does not exist. Search forward from the back of the array, starting at fromIndex.
const animals = ['Dodo', 'Tiger', 'Penguin', 'Dodo'];
console.log(animals.lastIndexOf('Dodo'));
// expected output: 3
console.log(animals.lastIndexOf('Tiger'));
// expected output: 1
The map() method creates a new array consisting of the return value of calling the provided function once for each element in the original array.
const array1 = [1, 4, 9, 16];
// pass a function to map
const map1 = array1.map((x:number):number => x * 2);
console.log(map1);
// expected output: Array [2, 8, 18, 32]
The pop() method removes the last element from the array and returns the value of that element. This method changes the length of the array.
const plants = ['broccoli', 'cauliflower', 'cabbage', 'kale', 'tomato'];
console.log(plants.pop());
// expected output: "tomato"
console.log(plants);
// expected output: Array ["broccoli", "cauliflower", "cabbage", "kale"]
plants.pop();
console.log(plants);
// expected output: Array ["broccoli", "cauliflower", "cabbage"]
The push() method adds one or more elements to the end of an array and returns the new length of the array.
const animals = ['pigs', 'goats', 'sheep'];
const count = animals.push('cows');
console.log(count);
// expected output: 4
console.log(animals);
// expected output: Array ["pigs", "goats", "sheep", "cows"]
animals.push('chickens', 'cats', 'dogs');
console.log(animals);
// expected output: Array ["pigs", "goats", "sheep", "cows", "chickens", "cats", "dogs"]
The reduce() method executes a reducer function you provide in order for each element in the array, each run of the reducer passes in the result of the previous element's calculation as a parameter, and finally aggregates its results into a single return value.
When the callback function is executed for the first time, there is no "last calculation result". If you want the callback function to execute from the element at index 0 of the array, you need to pass the initial value. Otherwise, the element at index 0 of the array will be used as initialValue, and the iterator will be executed from the second element (index 1 instead of 0).
const array1 = [1, 2, 3, 4];
// 0 + 1 + 2 + 3 + 4
const initialValue = 0;
const sumWithInitial = array1.reduce(
(previousValue:number, currentValue:number):number => previousValue + currentValue,
initialValue
);
console.log(sumWithInitial);
// expected output: 10
The shift() method removes the first element from the array and returns the value of that element. This method changes the length of the array.
const array1 = [1, 2, 3];
const firstElement = array1.shift();
console.log(array1);
// expected output: Array [2, 3]
console.log(firstElement);
// expected output: 1
The slice() method returns a new array object that is a shallow copy of the original array determined by begin and end (including begin, but not end). The original array will not be altered.
const animals = ['ant', 'bison', 'camel', 'duck', 'elephant'];
console.log(animals.slice(2));
// expected output: Array ["camel", "duck", "elephant"]
console.log(animals.slice(2, 4));
// expected output: Array ["camel", "duck"]
console.log(animals.slice(1, 5));
// expected output: Array ["bison", "camel", "duck", "elephant"]
console.log(animals.slice(-2));
// expected output: Array ["duck", "elephant"]
console.log(animals.slice(2, -1));
// expected output: Array ["camel", "duck"]
console.log(animals.slice());
// expected output: Array ["ant", "bison", "camel", "duck", "elephant"]
The some() method tests whether at least 1 element in the array passes the provided function test. It returns a value of type Boolean.
const array = [1, 2, 3, 4, 5];
// checks whether an element is even
const even = (element:number):boolean=> element % 2 == 0;
console.log(array.some(even));
// expected output: true
The sort() method sorts the elements of an array and returns the array.
Platform Difference Description
JavaScript | Kotlin | Swift |
---|---|---|
√ | √ | x |
const array2 = [5, 1, 4, 2, 3];
array2.sort((a: number, b: number):number => a - b);
// expect(array2).toEqual([1, 2, 3, 4, 5]);
The splice() method modifies an array by removing or replacing existing elements or adding new elements in place, and returns the modified contents as an array. This method changes the original array.
const months = ['Jan', 'March', 'April', 'June'];
months.splice(1, 0, 'Feb');
// inserts at index 1
console.log(months);
// expected output: Array ["Jan", "Feb", "March", "April", "June"]
months.splice(4, 1, 'May');
// replaces 1 element at index 4
console.log(months);
// expected output: Array ["Jan", "Feb", "March", "April", "May"]
The unshift() method adds one or more elements to the beginning of an array and returns the new length of the array (this method modifies the original array).
const array1 = [1, 2, 3];
console.log(array1.unshift(4, 5));
// expected output: 5
console.log(array1);
// expected output: Array [4, 5, 1, 2, 3]
const fruits = ['Apple', 'Banana']
console.log(fruits.length)
const first = fruits[0]
// Apple
const last = fruits[fruits.length - 1]
// Banana
fruits.forEach(function(item, index, array) {
console.log(item, index)
})
// Apple 0
// Banana 1
const newLength = fruits.push('Orange')
// ["Apple", "Banana", "Orange"]
const last = fruits.pop() // remove Orange (from the end)
// ["Apple", "Banana"]
const first = fruits.shift() // remove Apple from the front
// ["Banana"]
const newLength = fruits.unshift('Strawberry') // add to the front
// ["Strawberry", "Banana"]
fruits.push('Mango')
// ["Strawberry", "Banana", "Mango"]
const pos = fruits.indexOf('Banana')
// 1
const removedItem = fruits.splice(pos, 1) // this is how to remove an item
// ["Strawberry", "Mango"]
const vegetables = ['Cabbage', 'Turnip', 'Radish', 'Carrot']
console.log(vegetables)
// ["Cabbage", "Turnip", "Radish", "Carrot"]
const pos = 1
const n = 2
const removedItems = vegetables.splice(pos, n)
// this is how to remove items, n defines the number of items to be removed,
// starting at the index position specified by pos and progressing toward the end of array.
console.log(vegetables)
// ["Cabbage", "Carrot"] (the original array is changed)
console.log(removedItems)
// ["Turnip", "Radish"]
const shallowCopy = fruits.slice() // this is how to make a copy
// ["Strawberry", "Mango"]
The index of the array is 0-based, the index of the first element is 0, and the index of the last element is equal to the length of the array minus 1.
If the specified index is an invalid value, an IndexOutOfBoundsException will be thrown
The following writing is wrong, and a SyntaxError exception will be thrown at runtime because of the use of an illegal property name:
console.log(arr.0) // a syntax error
Create a Date instance that renders a moment in time. Date objects are based on the Unix Time Stamp, which is the number of milliseconds elapsed since January 1, 1970 (UTC).
new Date();
new Date(value);
new Date(year, monthIndex [, day [, hours [, minutes [, seconds [, milliseconds]]]]]);
Represents the number of milliseconds since the beginning of the UNIX epoch (January 1, 1970 00:00:00 (UTC)) to the current time.
Platform Difference Description
JavaScript | Kotlin | Swift |
---|---|---|
√ | √ | √ (3.6.11+) |
// this example takes 2 seconds to run
const start = Date.now()
console.log('starting timer...')
// expected output: starting timer...
setTimeout(() => {
const millis = Date.now() - start
console.log(`seconds elapsed = ${Math.floor(millis / 1000)}`)
// expected output: seconds elapsed = 2
}, 2000)
Returns the day of the month (from 1--31) for a specified date object according to local time.
Platform Difference Description
JavaScript | Kotlin | Swift |
---|---|---|
√ | √ | √ (3.6.11+) |
Returns the day of the week for a specific date, according to local time, with 0 for Sunday. for the day of the month
Platform Difference Description
JavaScript | Kotlin | Swift |
---|---|---|
√ | √ | √ (3.6.11+) |
Returns the year of the specified date according to local time.
Returns the hour of a specified date object according to local time.
Platform Difference Description
JavaScript | Kotlin | Swift |
---|---|---|
√ | √ | √ (3.6.11+) |
Returns the number of milliseconds for a specified date object according to local time.
Platform Difference Description
JavaScript | Kotlin | Swift |
---|---|---|
√ | √ | √ (3.6.11+) |
Returns the number of minutes in a specified date object according to local time.
Platform Difference Description
JavaScript | Kotlin | Swift |
---|---|---|
√ | √ | √ (3.6.11+) |
The month of the specified date object, as a 0-based value (0 represents the first month of the year).
Platform Difference Description
JavaScript | Kotlin | Swift |
---|---|---|
√ | √ | √ (3.6.11+) |
Returns the number of seconds in a specified date object according to local time.
Platform Difference Description
JavaScript | Kotlin | Swift |
---|---|---|
√ | √ | √ (3.6.11+) |
Returns the GMT value of a time.
Platform Difference Description
JavaScript | Kotlin | Swift |
---|---|---|
√ | √ | √ (3.6.11+) |
Specifies the number of days in a date object according to local time.
Platform Difference Description
JavaScript | Kotlin | Swift |
---|---|---|
√ | √ | √ (3.6.11+) |
Sets the year for a date object based on local time.
Platform Difference Description
JavaScript | Kotlin | Swift |
---|---|---|
√ | √ | √ (3.6.11+) |
Sets the hour for a Date object according to local time, returning the number of milliseconds from 1970-01-01 00:00:00 UTC to the time represented by the updated Date object instance.
Sets the milliseconds of a date object according to local time.
Sets the minutes for a date object according to local time.
Sets the month for a date object according to local time.
Sets the seconds for a date object according to local time.
Sets the time for a Date object as a number of milliseconds representing the time since 1970-1-1 00:00:00 UTC.
Platform Difference Description
JavaScript | Kotlin | Swift |
---|---|---|
√ | √ | √ (3.6.11+) |
The Error object is thrown when a runtime error occurs. Error objects can also be used as base objects for user-defined exceptions.
wrong information. For user-created Error objects, this is the string provided as the first parameter of the constructor.
try {
throw new Error('Whoops!')
} catch (e) {
console.error(e.message)
}
The JSON.parse() method is used to parse a JSON string and construct a UTSJSONObject described by the string.
const json = `{"result":true, "count":42}`;
const obj = JSON.parse(json);
console.log(obj["count"]);
// expected output: 42
console.log(obj["result"]);
// expected output: true
Notice
The JSON.stringify() method converts a uts object or value to a JSON string
console.log(JSON.stringify({ x: 5, y: 6 }));
// expected output: "{"x":5,"y":6}"
console.log(JSON.stringify([3, 'false', boolean]));
// expected output: "[3,"false",false]"
console.log(JSON.stringify(new Date(2006, 0, 2, 15, 4, 5)));
// expected output: ""2006-01-02T15:04:05.000Z""
Map objects hold key-value pairs. Any value (object or primitive type) can be a key or a value.
Returns the number of members of the Map object.
const map1 = new Map<string,string>();
map1.set('a', 'alpha');
map1.set('b', 'beta');
map1.set('g', 'gamma');
console.log(map1.size);
// expected output: 3
Removes all elements from the Map object.
const map1 = new Map<string,string>();
map1.set('bar', 'baz');
map1.set(1, 'foo');
console.log(map1.size);
// expected output: 2
map1.clear();
console.log(map1.size);
// expected output: 0
Used to remove the specified element in the Map object.
const map1 = new Map<string,string>();
map1.set('bar', 'foo');
console.log(map1.delete('bar'));
// expected result: true
// (true indicates successful removal)
console.log(map1.has('bar'));
// expected result: false
Returns a specified element in a Map object.
const map1 = new Map<string,string>();
map1.set('bar', 'foo');
console.log(map1.get('bar'));
// expected output: "foo"
Returns a boolean value indicating whether the specified element exists in the Map.
const map1 = new Map<string,string>();
map1.set('bar', 'foo');
console.log(map1.has('bar'));
// expected output: true
console.log(map1.has('baz'));
// expected output: false
Adds or updates a (new) key-value pair specifying a key and a value.
const map1 = new Map<string,string>();
map1.set('bar', 'foo');
console.log(map1.get('bar'));
// expected output: "foo"
console.log(map1.get('baz'));
// expected output: null
Math is a built-in object that has some properties of mathematical constants and methods of mathematical functions.
The Math.E property represents the base (or base) of natural logarithms, e, approximately equal to 2.718.
Platform Difference Description
JavaScript | Kotlin | Swift |
---|---|---|
√ | √ | √ (3.7.1+) |
function getNapier():number {
return Math.E;
}
console.log(getNapier());
// expected output: 2.718281828459045
The Math.LN10 property represents the natural logarithm of 10, approximately 2.302.
Platform Difference Description
JavaScript | Kotlin | Swift |
---|---|---|
√ | √ | √ (3.7.1+) |
function getNatLog10():number {
return Math.LN10;
}
console.log(getNatLog10());
// expected output: 2.302585092994046
The Math.LN2 property represents the natural logarithm of 2, which is approximately 0.693.
Platform Difference Description
JavaScript | Kotlin | Swift |
---|---|---|
√ | √ | √ (3.7.1+) |
function getNatLog2():number {
return Math.LN2;
}
console.log(getNatLog2());
// expected output: 0.6931471805599453
The Math.LOG10E property represents the base 10 logarithm of e, approximately 0.434.
Platform Difference Description
JavaScript | Kotlin | Swift |
---|---|---|
√ | √ | √ (3.7.1+) |
function getLog10e():number {
return Math.LOG10E;
}
console.log(getLog10e());
// expected output: 0.4342944819032518
The Math.LOG2E property represents the base 2 logarithm of e, approximately 1.442.
Platform Difference Description
JavaScript | Kotlin | Swift |
---|---|---|
√ | √ | √ (3.7.1+) |
function getLog2e():number {
return Math.LOG2E;
}
console.log(getLog2e());
// expected output: 1.4426950408889634
Math.PI represents the ratio of a circle's circumference to its diameter, which is approximately 3.14159.
Platform Difference Description
JavaScript | Kotlin | Swift |
---|---|---|
√ | √ | √ (3.7.1+) |
function calculateCircumference (radius:number):number {
return 2 * Math.PI * radius;
}
console.log(calculateCircumference(1));
// expected output: 6.283185307179586
The Math.SQRT1_2 property represents the square root of 1/2, which is approximately 0.707.
Platform Difference Description
JavaScript | Kotlin | Swift |
---|---|---|
√ | √ | √ (3.7.1+) |
function getRoot1_2():number {
return Math.SQRT1_2;
}
console.log(getRoot1_2());
// expected output: 0.7071067811865476
The Math.SQRT2 property represents the square root of 2, approximately 1.414.
Platform Difference Description
JavaScript | Kotlin | Swift |
---|---|---|
√ | √ | √ (3.7.1+) |
function getRoot2():number {
return Math.SQRT2;
}
console.log(getRoot2());
// expected output: 1.4142135623730951
The Math.abs(x) function returns the absolute value of a number.
Platform Difference Description
JavaScript | Kotlin | Swift |
---|---|---|
√ | √ | √ (3.7.1+) |
function difference(a:number, b:number):number {
return Math.abs(a - b);
}
console.log(difference(3, 5));
// expected output: 2
console.log(difference(5, 3));
// expected output: 2
console.log(difference(1.23456, 7.89012));
// expected output: 6.6555599999999995
Math.acos() Returns the arccosine of a number in radians.
Platform Difference Description
JavaScript | Kotlin | Swift |
---|---|---|
√ | √ | √ (3.7.1+) |
console.log(Math.acos(-1));
// expected output: 3.141592653589793
console.log(Math.acos(0));
// expected output: 1.5707963267948966
console.log(Math.acos(1));
// expected output: 0
The Math.acosh() function returns the inverse hyperbolic cosine of a number.
Platform Difference Description
JavaScript | Kotlin | Swift |
---|---|---|
√ | √ | √ (3.7.1+) |
console.log(Math.acosh(1));
// expected output: 0
console.log(Math.acosh(2));
// expected output: 1.3169578969248166
console.log(Math.acosh(2.5));
// expected output: 1.566799236972411
The Math.asin() method returns the arcsine of a number in radians.
Platform Difference Description
JavaScript | Kotlin | Swift |
---|---|---|
√ | √ | √ (3.7.1+) |
console.log(Math.asin(-1));
// expected output: -1.5707963267948966 (-pi/2)
console.log(Math.asin(0));
// expected output: 0
console.log(Math.asin(0.5));
// expected output: 0.5235987755982989
console.log(Math.asin(1));
// expected output: 1.5707963267948966
Math.asinh() Returns the inverse hyperbolic sine of a number.
Platform Difference Description
JavaScript | Kotlin | Swift |
---|---|---|
√ | √ | √ (3.7.1+) |
console.log(Math.asinh(1));
// expected output: 0.881373587019543
console.log(Math.asinh(0));
// expected output: 0
console.log(Math.asinh(-1));
// expected output: -0.881373587019543
console.log(Math.asinh(2));
// expected output: 1.4436354751788103
The Math.atan() function returns the arctangent of a number in radians.
Platform Difference Description
JavaScript | Kotlin | Swift |
---|---|---|
√ | √ | √ (3.7.1+) |
console.log(Math.atan(1));
// expected output: 0.7853981633974483
console.log(Math.atan(0));
// expected output: 0
Math.atan2() returns the plane angle (in radians) between the line segment from the origin (0,0) to the point (x,y) and the positive x-axis, that is, Math.atan2(y,x).
Platform Difference Description
JavaScript | Kotlin | Swift |
---|---|---|
√ | √ | √ (3.7.1+) |
console.log(Math.atan2(90, 15));
// expected output: 1.4056476493802699
console.log(Math.atan2(15, 90));
// expected output: 0.16514867741462683
The Math.atanh() function returns a numeric inverse hyperbolic tangent.
Platform Difference Description
JavaScript | Kotlin | Swift |
---|---|---|
√ | √ | √ (3.7.1+) |
console.log(Math.atanh(0));
// expected output: 0
console.log(Math.atanh(0.5));
// expected output: 0.5493061443340548
The Math.cbrt() function returns the cube root of any number.
Platform Difference Description
JavaScript | Kotlin | Swift |
---|---|---|
√ | √ | √ (3.7.1+) |
console.log(Math.cbrt(-1));
// expected output: -1
console.log(Math.cbrt(0));
// expected output: 0
console.log(Math.cbrt(1));
// expected output: 1
console.log(Math.cbrt(2));
// expected output: 1.2599210498948732
The Math.ceil() function always rounds up and returns the smallest integer greater than or equal to the given number.
Platform Difference Description
JavaScript | Kotlin | Swift |
---|---|---|
√ | √ | √ (3.7.1+) |
console.log(Math.ceil(0.95));
// expected output: 1
console.log(Math.ceil(4));
// expected output: 4
console.log(Math.ceil(7.004));
// expected output: 8
console.log(Math.ceil(-7.004));
// expected output: -7
The Math.clz32() function returns the number of leading zeros after a number is converted to the binary form of 32 unsigned integers.
Platform Difference Description
JavaScript | Kotlin | Swift |
---|---|---|
√ | √ | √ (3.7.1+) |
console.log(Math.clz32(1));
// expected output: 31
console.log(Math.clz32(1000));
// expected output: 22
console.log(Math.clz32());
// expected output: 32
console.log(Math.clz32(3.5));
// expected output: 30
The Math.cos() function returns the cosine of a number.
Platform Difference Description
JavaScript | Kotlin | Swift |
---|---|---|
√ | √ | √ (3.7.1+) |
console.log(Math.cos(0));
// expected output: 1
console.log(Math.cos(1));
// expected output: 0.5403023058681398
The Math.cosh() function returns the hyperbolic cosine function of a number.
Platform Difference Description
JavaScript | Kotlin | Swift |
---|---|---|
√ | √ | √ (3.7.1+) |
console.log(Math.cosh(0));
// expected output: 1
console.log(Math.cosh(1));
// expected output: 1.5430806348152437
console.log(Math.cosh(-1));
// expected output: 1.5430806348152437
The Math.exp() function returns e^x, x represents the parameter, and e is Euler's constant (Euler's constant), the base of natural logarithms.
Platform Difference Description
JavaScript | Kotlin | Swift |
---|---|---|
√ | √ | √ (3.7.1+) |
console.log(Math.exp(-1));
// expected output: 0.36787944117144233
console.log(Math.exp(0));
// expected output: 1
console.log(Math.exp(1));
// expected output: 2.718281828459045
The Math.expm1() function returns E^x - 1, where x is the argument to the function and E is the base of the natural logarithm 2.718281828459045.
Platform Difference Description
JavaScript | Kotlin | Swift |
---|---|---|
√ | √ | √ (3.7.1+) |
console.log(Math.expm1(1));
// expected output: 1.718281828459045
console.log(Math.expm1(-38));
// expected output: -1
Math.floor() 函数总是返回小于等于一个给定数字的最大整数。
Platform Difference Description
JavaScript | Kotlin | Swift |
---|---|---|
√ | √ | √ (3.7.1+) |
console.log(Math.floor(5.95));
// expected output: 5
console.log(Math.floor(5.05));
// expected output: 5
console.log(Math.floor(5));
// expected output: 5
console.log(Math.floor(-5.05));
// expected output: -6
Math.fround() converts any number to its nearest single-precision floating-point number.
Platform Difference Description
JavaScript | Kotlin | Swift |
---|---|---|
√ | √ | x |
console.log(Math.fround(1.5));
// expected output: 1.5
console.log(Math.fround(1.337));
// expected output: 1.3370000123977661
The Math.hypot() function returns the square root of the sum of squares of all arguments.
Platform Difference Description
JavaScript | Kotlin | Swift |
---|---|---|
√ | x | √ (3.7.1+) |
console.log(Math.hypot(3, 4));
// expected output: 5
console.log(Math.hypot(5, 12));
// expected output: 13
console.log(Math.hypot(3, 4, 5));
// expected output: 7.0710678118654755
console.log(Math.hypot(-5));
// expected output: 5
This function converts the two arguments to 32-bit integers and returns the 32-bit result when multiplied.
Platform Difference Description
JavaScript | Kotlin | Swift |
---|---|---|
√ | x | √ (3.7.1+) |
console.log(Math.imul(3, 4));
// expected output: 12
console.log(Math.imul(-5, 12));
// expected output: -60
The Math.log() function returns the natural logarithm of a number.
Platform Difference Description
JavaScript | Kotlin | Swift |
---|---|---|
√ | √ | √ (3.7.1+) |
console.log(Math.log(1));
// expected output: 0
console.log(Math.log(10));
// expected output: 2.302585092994046
The Math.log10() function returns the base 10 logarithm of a number.
Platform Difference Description
JavaScript | Kotlin | Swift |
---|---|---|
√ | √ | √ (3.7.1+) |
console.log(Math.log10(10));
// expected output: 1
console.log(Math.log10(100));
// expected output: 2
console.log(Math.log10(1));
// expected output: 0
The Math.log1p() function returns the natural logarithm (base E) of a number plus 1, which is log(x+1).
Platform Difference Description
JavaScript | Kotlin | Swift |
---|---|---|
√ | √ | √ (3.7.1+) |
console.log(Math.log1p(Math.E-1));
// expected output: 1
console.log(Math.log1p(0));
// expected output: 0
The Math.log2() function returns the base 2 logarithm of a number.
Platform Difference Description
JavaScript | Kotlin | Swift |
---|---|---|
√ | √ | √ (3.7.1+) |
console.log(Math.log2(2));
// expected output: 1
console.log(Math.log2(1024));
// expected output: 10
console.log(Math.log2(1));
// expected output: 0
The Math.max() function returns the largest number given as an input parameter.
Platform Difference Description
JavaScript | Kotlin | Swift |
---|---|---|
√ | √ | √ (3.7.1+) |
console.log(Math.max(1, 3, 2));
// expected output: 3
console.log(Math.max(-1, -3, -2));
// expected output: -1
The Math.min() function returns the smallest of the numbers given as input parameters.
Platform Difference Description
JavaScript | Kotlin | Swift |
---|---|---|
√ | √ | √ (3.7.1+) |
console.log(Math.min(2, 3, 1));
// expected output: 1
console.log(Math.min(-2, -3, -1));
// expected output: -3
The Math.pow() function returns the base (base) raised to the power of the exponent (exponent), ie base^exponent.
Platform Difference Description
JavaScript | Kotlin | Swift |
---|---|---|
√ | √ | √ (3.7.1+) |
console.log(Math.pow(7, 3));
// expected output: 343
console.log(Math.pow(4, 0.5));
// expected output: 2
The Math.random() function returns a floating-point number, a pseudo-random number in the range from 0 to less than 1.
Platform Difference Description
JavaScript | Kotlin | Swift |
---|---|---|
√ | √ | √ (3.7.1+) |
function getRandomInt(max:number):number {
return Math.floor(Math.random() * max);
}
console.log(getRandomInt(3));
// expected output: 0, 1 or 2
console.log(getRandomInt(1));
// expected output: 0
console.log(Math.random());
// expected output: a number from 0 to <1
The Math.round() function returns a number rounded to the nearest integer.
Platform Difference Description
JavaScript | Kotlin | Swift |
---|---|---|
√ | √ | √ (3.7.1+) |
console.log(Math.round(20.49));
// expected output: 20
console.log(Math.round(20.5));
// expected output: 21
console.log(Math.round(-20.5));
// expected output: -20
console.log(Math.round(-20.51));
// expected output: -21
Math.sign() 函数返回一个数字的符号,分别是 1、-1、0,代表的各是正数、负数、零。
Platform Difference Description
JavaScript | Kotlin | Swift |
---|---|---|
√ | √ | √ (3.7.1+) |
console.log(Math.sign(3));
// expected output: 1
console.log(Math.sign(-3));
// expected output: -1
console.log(Math.sign(0));
// expected output: 0
The Math.sin() function returns the sine of a number.
Platform Difference Description
JavaScript | Kotlin | Swift |
---|---|---|
√ | √ | √ (3.7.1+) |
console.log(Math.sin(0));
// expected output: 0
console.log(Math.sin(1));
// expected output: 0.8414709848078965
The Math.sinh() function returns the hyperbolic sine of a number (in degrees).
Platform Difference Description
JavaScript | Kotlin | Swift |
---|---|---|
√ | √ | √ (3.7.1+) |
console.log(Math.sinh(0));
// expected output: 0
console.log(Math.sinh(1));
// expected output: 1.1752011936438014
The Math.sqrt() function returns the square root of a number.
Platform Difference Description
JavaScript | Kotlin | Swift |
---|---|---|
√ | √ | √ (3.7.1+) |
function calcHypotenuse(a:number, b:number):number {
return (Math.sqrt((a * a) + (b * b)));
}
console.log(calcHypotenuse(3, 4));
// expected output: 5
console.log(calcHypotenuse(5, 12));
// expected output: 13
console.log(calcHypotenuse(0, 0));
// expected output: 0
The Math.tan() method returns the tangent of a number.
Platform Difference Description
JavaScript | Kotlin | Swift |
---|---|---|
√ | √ | √ (3.7.1+) |
console.log(Math.tan(0));
// expected output: 0
console.log(Math.tan(1));
// expected output: 1.5574077246549023
The Math.tanh() function will return the value of the hyperbolic tangent of a number.
Platform Difference Description
JavaScript | Kotlin | Swift |
---|---|---|
√ | √ | √ (3.7.1+) |
console.log(Math.tanh(-1));
// Expected output: -0.7615941559557649
console.log(Math.tanh(0));
// Expected output: 0
console.log(Math.tanh(1));
// Expected output: 0.7615941559557649
The Math.trunc() method will remove the decimal part of the number and keep only the integer part.
Platform Difference Description
JavaScript | Kotlin | Swift |
---|---|---|
√ | √ | √ (3.7.1+) |
console.log(Math.trunc(13.37));
// Expected output: 13
console.log(Math.trunc(42.84));
// Expected output: 42
console.log(Math.trunc(0.123));
// Expected output: 0
Number objects are encapsulated objects that allow you to work with numeric values.
The toFixed() method formats a number using fixed-point notation.
Platform Difference Description
JavaScript | Kotlin | Swift |
---|---|---|
√ | √ | √ (3.6.11+) |
function financial(x: Number): String {
return x.toFixed(2);
}
console.log(financial(123.456));
// expected output: "123.46"
console.log(financial(0.004));
// expected output: "0.00"
RegExp 对象用于将文本与一个模式匹配。
dotAll 属性表明是否在正则表达式中一起使用"s"修饰符。
平台差异说明
JavaScript | Kotlin | Swift |
---|---|---|
√ | x | x |
const regex1 = new RegExp('foo', 's');
console.log(regex1.dotAll);
// expected output: true
const regex2 = new RegExp('bar');
console.log(regex2.dotAll);
// expected output: false
flags 属性属性返回一个字符串,由当前正则表达式对象的标志组成。
平台差异说明
JavaScript | Kotlin | Swift |
---|---|---|
√ | x | x |
console.log(/foo/ig.flags);
// expected output: "gi"
console.log(/bar/myu.flags);
// expected output: "muy"
global 属性表明正则表达式是否使用了 "g" 标志。
平台差异说明
JavaScript | Kotlin | Swift |
---|---|---|
√ | x | x |
var regex = new RegExp("foo", "g")
console.log(regex.global) // true
// expected output: "muy"
hasIndices 属性指示 "d" 标志是否与正则表达式一起使用。
平台差异说明
JavaScript | Kotlin | Swift |
---|---|---|
√ | x | x |
const regex1 = new RegExp('foo', 'd');
console.log(regex1.hasIndices);
// expected output: true
const regex2 = new RegExp('bar');
console.log(regex2.hasIndices);
// expected output: false
lastIndex 是正则表达式的一个可读可写的整型属性,用来指定下一次匹配的起始索引。
平台差异说明
JavaScript | Kotlin | Swift |
---|---|---|
√ | x | x |
const regex1 = new RegExp('foo', 'g');
const str1 = 'table football, foosball';
regex1.test(str1);
console.log(regex1.lastIndex);
// expected output: 9
regex1.test(str1);
console.log(regex1.lastIndex);
// expected output: 19
multiline 属性表明正则表达式是否使用了 "m" 标志。
平台差异说明
JavaScript | Kotlin | Swift |
---|---|---|
√ | x | x |
var regex = new RegExp("foo", "m");
console.log(regex.multiline);
// expected output: true
source 属性返回一个值为当前正则表达式对象的模式文本的字符串,该字符串不会包含正则字面量两边的斜杠以及任何的标志字符。
平台差异说明
JavaScript | Kotlin | Swift |
---|---|---|
√ | x | x |
const regex1 = /fooBar/ig;
console.log(regex1.source);
// expected output: "fooBar"
console.log(new RegExp().source);
// expected output: "(?:)"
sticky 属性反映了搜索是否具有粘性(仅从正则表达式的 lastIndex 属性表示的索引处搜索)。
平台差异说明
JavaScript | Kotlin | Swift |
---|---|---|
√ | x | x |
const str1 = 'table football';
const regex1 = new RegExp('foo', 'y');
regex1.lastIndex = 6;
console.log(regex1.sticky);
// expected output: true
console.log(regex1.test(str1));
// expected output: true
console.log(regex1.test(str1));
// expected output: false
unicode 属性表明正则表达式带有"u" 标志。
平台差异说明
JavaScript | Kotlin | Swift |
---|---|---|
√ | x | x |
var regex = new RegExp('\u{61}', 'u');
console.log(regex.unicode);
// expected output: true
exec() 方法在一个指定字符串中执行一个搜索匹配。返回一个结果数组或 null。
const regex1 = RegExp('foo*', 'g');
const str1 = 'table football, foosball';
let array1;
while ((array1 = regex1.exec(str1)) !== null) {
console.log(`Found ${array1[0]}. Next starts at ${regex1.lastIndex}.`);
// expected output: "Found foo. Next starts at 9."
// expected output: "Found foo. Next starts at 19."
}
test() 方法执行一个检索,用来查看正则表达式与指定的字符串是否匹配。返回 true 或 false。
const str = 'table football';
const regex = new RegExp('foo*');
const globalRegex = new RegExp('foo*', 'g');
console.log(regex.test(str));
// expected output: true
console.log(globalRegex.lastIndex);
// expected output: 0
console.log(globalRegex.test(str));
// expected output: true
console.log(globalRegex.lastIndex);
// expected output: 9
console.log(globalRegex.test(str));
// expected output: false
toString() 返回一个表示该正则表达式的字符串。
console.log(new RegExp('a+b+c'));
// expected output: /a+b+c/
console.log(new RegExp('a+b+c').toString());
// expected output: "/a+b+c/"
console.log(new RegExp('bar', 'g').toString());
// expected output: "/bar/g"
console.log(new RegExp('\n', 'g').toString());
// expected output (if your browser supports escaping): "/\n/g"
console.log(new RegExp('\\n', 'g').toString());
// expected output: "/\n/g"
A Set object is a collection of values, and you can iterate over its elements in the order they were inserted. The elements in the Set will only appear once, that is, the elements in the Set are unique.
Returns the number of elements in the Set object.
Platform Difference Description
JavaScript | Kotlin | Swift |
---|---|---|
√ | √ | √ (3.6.11+) |
const set1 = new Set<Any>();
set1.add(42);
set1.add('forty two');
set1.add('forty two');
console.log(set1.size);
// expected output: 2
The add() method is used to add a specified value to the end of a Set object.
const set1 = new Set<number>();
set1.add(42);
set1.add(42);
set1.add(13);
set1.forEach((item)=>{
console.log(item);
// expected output: 42
// expected output: 13
})
The clear() method is used to clear all elements in a Set object.
Platform Difference Description
JavaScript | Kotlin | Swift |
---|---|---|
√ | √ | √ (3.6.11+) |
const set1 = new Set<any>();
set1.add(1);
set1.add('foo');
console.log(set1.size);
// expected output: 2
set1.clear();
console.log(set1.size);
// expected output: 0
The delete() method deletes the specified element from a Set object.
Platform Difference Description
JavaScript | Kotlin | Swift |
---|---|---|
√ | √ | √ (3.6.11+) |
const map1 = new Map<string,string>();
map1.set('bar', 'foo');
console.log(map1.delete('bar'));
// expected result: true
// (true indicates successful removal)
console.log(map1.has('bar'));
// expected result: false
The forEach method executes the provided callback function in sequence according to the insertion order of the elements in the collection.
const set1 = new Set<number>([42, 13]);
set1.forEach((item)=>{
console.log(item);
// expected output: 42
// expected output: 13
})
The has() method returns a boolean value indicating whether the corresponding value value exists in the Set object.
Platform Difference Description
JavaScript | Kotlin | Swift |
---|---|---|
√ | √ | √ (3.6.11+) |
const set1 = new Set<number>([1, 2, 3, 4, 5]);
console.log(set1.has(1));
// expected output: true
console.log(set1.has(5));
// expected output: true
console.log(set1.has(6));
// expected output: false
The String global object is a constructor for strings or a sequence of characters.
String literals take the following form:
'string text'
"string text"
"中文/汉语"
"español"
"English "
"हिन्दी"
"العربية"
"português"
"বাংলা"
"русский"
"日本語"
"ਪੰਜਾਬੀ"
"한국어"
The length property represents the length of a string.
const x = "Mozilla";
const empty = "";
console.log("Mozilla is " + x.length + " code units long");
/* "Mozilla is 7 code units long" */
console.log("The empty string is has a length of " + empty.length);
/* "The empty string is has a length of 0" */
The at() method accepts an integer value and returns a new String consisting of a single UTF-16 code unit at the specified offset. This method allows positive and negative integers. Negative integers count down from the last character in the string.
const sentence = 'The quick brown fox jumps over the lazy dog.';
let index = 5;
console.log(`Using an index of ${index} the character returned is ${sentence.at(index)}`);
// expected output: "Using an index of 5 the character returned is u"
index = -4;
console.log(`Using an index of ${index} the character returned is ${sentence.at(index)}`);
// expected output: "Using an index of -4 the character returned is d"
The charAt() method returns the specified character from a string.
const anyString = "Brave new world";
console.log("The character at index 0 is '" + anyString.charAt(0) + "'");
// The character at index 0 is 'B'
console.log("The character at index 1 is '" + anyString.charAt(1) + "'");
// The character at index 1 is 'r'
console.log("The character at index 2 is '" + anyString.charAt(2) + "'");
// The character at index 2 is 'a'
console.log("The character at index 3 is '" + anyString.charAt(3) + "'");
// The character at index 3 is 'v'
console.log("The character at index 4 is '" + anyString.charAt(4) + "'");
// The character at index 4 is 'e'
console.log("The character at index 999 is '" + anyString.charAt(999) + "'");
// The character at index 999 is ''
The charCodeAt() method returns an integer between 0 and 65535 representing the UTF-16 code unit at the given index
const sentence = 'The quick brown fox jumps over the lazy dog.';
const index = 4;
console.log(`The character code ${sentence.charCodeAt(index)} is equal to ${sentence.charAt(index)}`);
// expected output: "The character code 113 is equal to q"
The concat() method concatenates one or more strings with the original string to form a new string and returns it.
Platform Difference Description
JavaScript | Kotlin | Swift |
---|---|---|
√ | √ | √ (3.6.11+) |
let hello = 'Hello, '
console.log(hello.concat('Kevin', '. Have a nice day.'))
// Hello, Kevin. Have a nice day.
The endsWith() method is used to judge whether the current string "ends" with another given substring, and returns true or false according to the judgment result.
Platform Difference Description
JavaScript | Kotlin | Swift |
---|---|---|
√ | √ | √ (3.6.11+) |
const str1 = 'Cats are the best!';
console.log(str1.endsWith('best!'));
// expected output: true
console.log(str1.endsWith('best', 17));
// expected output: true
const str2 = 'Is this a question?';
console.log(str2.endsWith('question'));
// expected output: false
The includes() method is used to determine whether a string is included in another string, and returns true or false according to the situation.
Platform Difference Description
JavaScript | Kotlin | Swift |
---|---|---|
√ | √ | √ (3.6.11+) |
const str = 'To be, or not to be, that is the question.';
console.log(str.includes('To be')); // true
console.log(str.includes('question')); // true
console.log(str.includes('nonexistent')); // false
console.log(str.includes('To be', 1)); // false
console.log(str.includes('TO BE')); // false
The indexOf() method returns the index of the first occurrence of the specified value in the String object on which it was called, searching from fromIndex. Returns -1 if the value is not found.
Platform Difference Description
JavaScript | Kotlin | Swift |
---|---|---|
√ | √ | √ (3.6.11+) |
const paragraph = 'The quick brown fox jumps over the lazy dog. If the dog barked, was it really lazy?';
const searchTerm = 'dog';
const indexOfFirst = paragraph.indexOf(searchTerm);
console.log(`The index of the first "${searchTerm}" from the beginning is ${indexOfFirst}`);
// expected output: "The index of the first "dog" from the beginning is 40"
console.log(`The index of the 2nd "${searchTerm}" is ${paragraph.indexOf(searchTerm, (indexOfFirst + 1))}`);
// expected output: "The index of the 2nd "dog" is 52"
The padEnd() method pads the current string with a string (repeatedly if necessary), and returns a string of the specified length after padding. Padding starts from the end (right side) of the current string.
Platform Difference Description
JavaScript | Kotlin | Swift |
---|---|---|
√ | √ | √ (3.6.11+) |
const str1 = 'Breaded Mushrooms';
console.log(str1.padEnd(25, '.'));
// expected output: "Breaded Mushrooms........"
const str2 = '200';
console.log(str2.padEnd(5));
// expected output: "200 "
The padStart() method pads the current string with another string (repeated as many times as necessary) so that the resulting string reaches the given length. Padding starts from the left of the current string.
Platform Difference Description
JavaScript | Kotlin | Swift |
---|---|---|
√ | √ | √ (3.6.11+) |
const str1 = '5';
console.log(str1.padStart(2, '0'));
// expected output: "05"
repeat() constructs and returns a new string containing the specified number of copies of the string concatenated together.
Platform Difference Description
JavaScript | Kotlin | Swift |
---|---|---|
√ | √ | √ (3.6.11+) |
"abc".repeat(0) // ""
"abc".repeat(1) // "abc"
"abc".repeat(2) // "abcabc"
"abc".repeat(3.5) // "abcabcabc" 参数 count 将会被自动转换成整数。
The replace() method returns a new string with some or all pattern matches replaced by the replacement value. The pattern can be a string or a regular expression, and the replacement value can be a string or a callback function to be called for each match. If pattern is a string, only the first match is replaced. The original string will not be changed.
Platform Difference Description
JavaScript | Kotlin | Swift |
---|---|---|
√ | √ | √ (3.6.11+) |
const p = 'The quick brown fox jumps over the lazy dog. If the dog reacted, was it really lazy?';
console.log(p.replace('dog', 'monkey'));
// expected output: "The quick brown fox jumps over the lazy monkey. If the dog reacted, was it really lazy?"
const regex = /Dog/i;
console.log(p.replace(regex, 'ferret'));
// expected output: "The quick brown fox jumps over the lazy ferret. If the dog reacted, was it really lazy?"
The search() method performs a search match between the regular expression and the String object.
Platform Difference Description
JavaScript | Kotlin | Swift |
---|---|---|
√ | √ | √ (3.6.11+) |
const paragraph = 'The quick brown fox jumps over the lazy dog. If the dog barked, was it really lazy?';
// any character that is not a word character or whitespace
const regex = /[^\w\s]/g;
console.log(paragraph.search(regex));
// expected output: 43
console.log(paragraph[paragraph.search(regex)]);
// expected output: "."
The slice() method extracts part of a string and returns a new string without changing the original string.
Platform Difference Description
JavaScript | Kotlin | Swift |
---|---|---|
√ | √ | √ (3.6.11+) |
const str = 'The quick brown fox jumps over the lazy dog.';
console.log(str.slice(31));
// expected output: "the lazy dog."
console.log(str.slice(4, 19));
// expected output: "quick brown fox"
The split() method splits a String object into an array of substrings using the specified delimiter string, and uses a specified split string to determine the position of each split.
Platform Difference Description
JavaScript | Kotlin | Swift |
---|---|---|
√ | √ | √ (3.6.11+) |
const str = 'The quick brown fox jumps over the lazy dog.';
const words = str.split(' ');
console.log(words[3]);
// expected output: "fox"
const chars = str.split('');
console.log(chars[8]);
// expected output: "k"
toLowerCase() converts the string value that calls this method to lowercase and returns it.
Platform Difference Description
JavaScript | Kotlin | Swift |
---|---|---|
√ | √ | √ (3.6.11+) |
console.log('中文简体 zh-CN || zh-Hans'.toLowerCase());
// Simplified Chinese zh-cn || zh-hans
console.log( "ALPHABET".toLowerCase() );
// "alphabet"
The toUpperCase() method converts the string that calls this method to uppercase and returns it (if the method is called with a value other than a string, it will be coerced).
Platform Difference Description
JavaScript | Kotlin | Swift |
---|---|---|
√ | √ | √ (3.6.11+) |
const sentence = 'The quick brown fox jumps over the lazy dog.';
console.log(sentence.toUpperCase());
// expected output: "THE QUICK BROWN FOX JUMPS OVER THE LAZY DOG."
Set a timer. Execute the registered callback function after the timer expires
setTimeout(() => {
console.log("Delayed for 1 second.")
}, 1000)
Cancels the timer set by setTimeout.
const timer = setTimeout(() => {
console.log("Delayed for 1 second.")
}, 1000)
clearTimeout(timer)
Set a timer. Execute the registered callback function according to the specified period (in milliseconds)
setInterval(() => {
console.log(Date.now())
}, 1000)
Cancels the timer set by setInterval.
const timer = setInterval(() => {
console.log(Date.now())
}, 1000)
clearInterval(timer)
注意:暂不支持在变量没有定义完成(赋值的过程中)就访问。比如:需要在setInterval内执行clearInterval。
例如:
let index = 0;
let interval : number = setInterval(() => {
index ++
if (index > 3) {
clearInterval(interval)
}
}, 6)
暂时需要使用如下写法(后续会优化抹平):
let index = 0;
let interval : number = 0;
interval = setInterval(() => {
index ++
if (index > 3) {
clearInterval(interval)
}
}, 6)
as
break
case
switch
.catch
try
to catch program exceptions.class
const
continue
debugger
default
switch
to match what to do if it doesn't exist, and can also be used in export
statements.delete
Javascript
platform)do
else
if
.export
extends
class
inheritance.finally
try-catch
.for
function
if
import
in
instanceof
new
class
instance.return
super
switch
this
throw
try
]
typeof
Javascript
platform)var
void
while
with
Javascript
platform)yield
Javascript
platform)enum
implements
interface
let
package
private
protected
public
static
await
abstract
boolean
byte
char
double
final
float
goto
int
long
native
short
synchronized
transient
volatile
+
+=
=
&
&=
~
|
|=
^
^=
?
--
/
/=
==
>
>=
++
!=
<<
<<=
<
<=
&&
&&=
!
??=
||
||=
*
*=
??
?.
%
%=
>>
>>=
===
!==
-
-=
>>>
>>>=
JavaScript is a very flexible programming language:
This flexibility, on the one hand, allows JavaScript to flourish, on the other hand, it also makes its code quality uneven and expensive to maintain.
The type system of uts can largely make up for the shortcomings of JavaScript.
uts is a static type
The type system is classified according to the "timing of type checking", which can be divided into dynamic type and static type.
Dynamic typing means that type checking happens at runtime, and type errors in this language often lead to runtime errors. JavaScript is an interpreted language, there is no compilation phase, so it is dynamically typed, and the following code will report an error at runtime:
let foo = 1;
foo.split(' ');
// Uncaught TypeError: foo.split is not a function
// An error will be reported at runtime (foo.split is not a function), causing an online bug
Static typing means that the type of each variable can be determined at the compile stage, and type errors in this language often lead to syntax errors. uts will perform type checking during the compilation phase, so uts is a static type, and this uts code will report an error during the compilation phase:
let foo = 1;
foo.split(' ');
// Property 'split' does not exist on type 'number'.
// An error will be reported when compiling (the number does not have a split method) and cannot be compiled
Most JavaScript code can be turned into uts code with only a few modifications and type annotations, which is very close to ts.
Example:
// js sums two numbers
function add(left, right) {
return left + right;
}
After adding type annotations, it can be turned into uts code
// uts sum
function add(left: number, right: number): number {
return left + right;
}
hello uts
At present, we can develop uts plugin through [Development uts plugin](https://uniapp.dcloud.net.cn/plugin/uts-plugin.html#_3-%E5%BC%80%E5%8F%91uts%E5%8E%9F %E7%94%9F%E6%8F%92%E4%BB%B6) to learn uts.