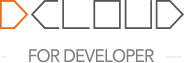
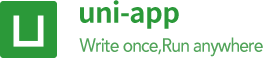
English
Supported by HBuilderX 2.0.0+, User Guide
When global custom events are triggered, additional parameters will be passed to the listener callback function.
Attribute | Type | Describe |
---|---|---|
eventName | String | Event name |
OBJECT | Object | Additional parameters carried by triggering events |
Code example
uni.$emit('update',{msg:'页面更新'})
listen to the global custom event triggered by uni.$emit
, and the callback function receives the parameters passed in to the event trigger function.
Attribute | Type | Describe |
---|---|---|
eventName | String | Event name |
callback | Function | Event callback function |
Code example
uni.$on('update',function(data){
console.log('监听到事件来自 update ,携带参数 msg 为:' + data.msg);
})
listen to the global custom events generated by uni.$emit
, but only once, and remove the listener after the first trigger.
Attribute | Type | Describe |
---|---|---|
eventName | String | Event name |
callback | Function | Event callback function |
Code example
uni.$once('update',function(data){
console.log('监听到事件来自 update ,携带参数 msg 为:' + data.msg);
})
Remove the global custom event listener.
Attribute | Type | Describe |
---|---|---|
eventName | Array<String> | Event name |
callback | Function | Event callback function |
Tips
Code example
$emit
, $on
and $off
are commonly used for cross-page and cross-component communication, and are placed on the same page for easy demonstration
<template>
<view class="content">
<view class="data">
<text>{{val}}</text>
</view>
<button type="primary" @click="comunicationOff">结束监听</button>
</view>
</template>
<script>
export default {
data() {
return {
val: 0
}
},
onLoad() {
setInterval(()=>{
uni.$emit('add', {
data: 2
})
},1000)
uni.$on('add', this.add)
},
methods: {
comunicationOff() {
uni.$off('add', this.add)
},
add(e) {
this.val += e.data
}
}
}
</script>
<style>
.content {
display: flex;
flex-direction: column;
align-items: center;
justify-content: center;
}
.data {
text-align: center;
line-height: 40px;
margin-top: 40px;
}
button {
width: 200px;
margin: 20px 0;
}
</style>
Precautions