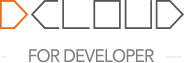
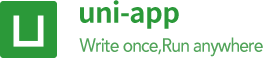
English
Make a network request.
When each MiniApp platform is running, network-related APIs need to be configured with a domain name whitelist before use.
OBJECT parameter description
Parameter Name | Type | Required | Default Value | Description | Platform Difference Description | |
---|---|---|---|---|---|---|
url | String | Yes | Developer server interface address | |||
data | Object/String/ArrayBuffer | No | Request parameter | App 3.3.7 or later does not support the ArrayBuffer type | ||
header | Object | No | Set the header of the request, Referer cannot be set in the header | App and H5 end will automatically bring cookies, and H5 end cannot be manually modified | ||
method | String | No | GET | Please refer to the description below for valid values | ||
timeout | Number | 否 | 60000 | 超时时间,单位 ms | H5(HBuilderX 2.9.9+)、APP(HBuilderX 2.9.9+)、微信小程序(2.10.0)、支付宝小程序 | |
dataType | String | 否 | json | 如果设为 json,会对返回的数据进行一次 JSON.parse,非 json 不会进行 JSON.parse | ||
responseType | String | No | text | Sets the data type of the response. Legal values: text, arraybuffer | Alipay MiniApp not support | |
sslVerify | Boolean | No | true | Verify ssl certificate | Only supported by App Android (HBuilderX 2.3.3+), does not support offline packaging | |
withCredentials | Boolean | No | false | Whether to carry credentials (cookies) when making cross-domain requests | Only supported by H5 (HBuilderX 2.6.15+) | |
firstIpv4 | Boolean | No | false | Use ipv4 first when DNS resolution | Only App-Android support (HBuilderX 2.8.0+) | |
enableHttp2 | Boolean | No | false | Enable http2 | WeChat MiniApp | |
enableQuic | Boolean | 否 | false | 开启 quic | 微信小程序 | |
enableCache | Boolean | 否 | false | 开启 cache | 微信小程序、抖音小程序 2.31.0+ | |
enableHttpDNS | Boolean | No | false | Whether to enable HttpDNS service. If enabled, you need to fill in httpDNSServiceId at the same time. For details on HttpDNS usage, see Mobile Resolution HttpDNS | WeChatMiniApp | |
httpDNSServiceId | String | No | HttpDNS service provider Id. For details on HttpDNS usage, see Mobile Resolution HttpDNS | WeChatMiniApp | ||
enableChunked | Boolean | No | false | Enable transfer-encoding chunked | WeChat MiniApp | |
forceCellularNetwork | Boolean | No | false | Use mobile network to send requests under wifi | WeChat MiniApp | |
enableCookie | Boolean | No | false | The cookie can be edited in the headers after enabling | Alipay MiniApp 10.2.33+ | |
cloudCache | Object/Boolean | 否 | false | 是否开启云加速(详见云加速服务) | 百度小程序 3.310.11+ | |
cloudCache | Object/Boolean | No | false | Whether to enable cloud acceleration (see [Cloud Acceleration Service](https://smartprogram.baidu.com/docs/develop/extended/component-codeless/cloud-speed/introduction/for details) <a href="https://smartprogram.baidu.com/docs/develop/extended/component-codeless/cloud-speed/introduction/)) | 百度小程序">)) | Baidu MiniApp 3.310.11+ |
defer | Boolean | No | false | Controls whether the current request is delayed until the content of the first screen is rendered | Baidu MiniApp 3.310.11+ | |
success | Function | No | received the callback function successfully returned by the developer server | |||
fail | Function | No | Interface call failure callback function | |||
complete | Function | No | The callback function for the end of the interface call (it will be executed when the call succeeds or fails) |
method Valid Values
Note: The effective value of method must be capitalized. The effective value of method supported by each platform is different. See the table below for details.
method | App | H5 | 微信小程序 | 支付宝小程序 | 百度小程序 | 抖音小程序、飞书小程序 | 快手小程序 | 京东小程序 |
---|---|---|---|---|---|---|---|---|
GET | √ | √ | √ | √ | √ | √ | √ | √ |
POST | √ | √ | √ | √ | √ | √ | √ | √ |
PUT | √ | √ | √ | x | √ | √ | x | x |
DELETE | √ | √ | √ | x | √ | x | x | x |
CONNECT | x | √ | √ | x | x | x | x | x |
HEAD | √ | √ | √ | x | √ | x | x | x |
OPTIONS | √ | √ | √ | x | √ | x | x | x |
TRACE | x | √ | √ | x | x | x | x | x |
success return parameter description
Parameter | Type | Description |
---|---|---|
data | Object/String/ArrayBuffer | Data returned by the developer server |
statusCode | Number | The HTTP status code returned by the developer server |
header | Object | HTTP Response Header returned by the developer server |
cookies | Array.<string> | The cookies returned by the developer server, in the format of a string array |
data data description
The final data sent to the server is of String type. If the incoming data is not of String type, it will be converted to String. The conversion rules are as follows:
GET
method, the data will be converted to a query string. For example, { name: 'name', age: 18 }
converts to name=name&age=18
.POST
method data with header['content-type']
as application/json
, JSON serialization will be performed.POST
method and header['content-type']
is application/x-www-form-urlencoded
, the data will be converted to query string.example
uni.request({
url: 'https://www.example.com/request', //仅为示例,并非真实接口地址。
data: {
text: 'uni.request'
},
header: {
'custom-header': 'hello' //自定义请求头信息
},
success: (res) => {
console.log(res.data);
this.text = 'request success';
}
});
return value
If you want to return a requestTask
object, you need to pass in at least one of the success / fail / complete parameters. For example:
var requestTask = uni.request({
url: 'https://www.example.com/request', //仅为示例,并非真实接口地址。
complete: ()=> {}
});
requestTask.abort();
If no success / fail / complete parameter is passed in, the encapsulated Promise object will be returned: Promise Encapsulation
With requestTask
, the request task can be interrupted.
list of methods of the requestTask object
Method | Parameter | Description |
---|---|---|
abort | interrupt request task | |
offHeadersReceived | Cancel listening to HTTP Response Header events, only supported by WeChat MiniApp Platform , document details | |
onHeadersReceived | Listen to HTTP Response Header events. It will be earlier than the request completion event, only supported by WeChat MiniApp Platform , document details |
example
const requestTask = uni.request({
url: 'https://www.example.com/request', //仅为示例,并非真实接口地址。
data: {
name: 'name',
age: 18
},
success: function(res) {
console.log(res.data);
}
});
// interrupt request task
requestTask.abort();
Tips
content-type
in the header
of the request defaults to application/json
.header
, or use encodeURIComponent to encode, otherwise an error will be reported in the Baidu MiniApp. (From: Quick Dog taxi front-end team)timeout time
of network requests can be uniformly configured in manifest.json
networkTimeout.sslVerify
configurationhttps request configuration self-signed certificate
App | H5 | 微信小程序 | 支付宝小程序 | 百度小程序 | 抖音小程序、飞书小程序 | QQ小程序 | 快手小程序 | 京东小程序 |
---|---|---|---|---|---|---|---|---|
√(3.2.7+) | x | x | x | x | x | x | x | x |
OBJECT parameter description
Parameter | Type | Required | Description |
---|---|---|---|
certificates | Array<certificate > | Yes | certificates is an array, which supports configuring self-signed certificates for multiple domain names |
success | Function(callbackObject ) | No | Callback function for successful interface call |
fail | Function(callbackObject ) | No | Callback function for interface call failure |
complete | Function | No | The callback function for the end of the interface call (it will be executed when the call succeeds or fails) |
certificate parameter description Certificate Configuration Items
Parameter | Type | Required | Description |
---|---|---|---|
host | String | yes | The domain name corresponding to the request (note: do not need the protocol part) |
client | String | No | Client certificate (this item needs to be configured when the server side needs to verify the client certificate. For the format requirements, please refer to the certificate format description below. Note that the iOS platform client certificate only supports .p12 type Certificate) |
clientPassword | String | 否 | 客户端证书对应的密码(客户端证书存在时必须配置此项) |
server | Array<String> | 否 | 服务器端证书(客户端需要对服务器端证书做校验时需要配置此项,通常使用自签名证书时才需要配置,格式要求请参考下面的证书格式说明,注意 iOS 平台服务器端证书只支持 .cer 类型的证书,并且证书要包含完整的信息) |
证书格式说明
证书支持两种格式,文件格式和 Base64字符串格式
'/static/client.p12'
Base64String
格式说明:将证书文件的二进制转换为 Base64String
字符串,然后在字符串前面添加'data:cert/pem;base64,'
前缀,示例:'data:cert/pem;base64,xxx'
xxx 代表真实的证书 base64StringcallbackObject parameter description
Attribute | Type | Description |
---|---|---|
code | Number | Returns 0 for success, returns the corresponding code for failure |
example
uni.configMTLS({
certificates: [{
'host': 'www.test.com',
'client': '/static/client.p12',
'clientPassword': '123456789',
'server': ['/static/server.cer'],
}],
success ({code}) {}
});