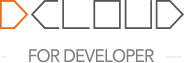
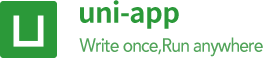
English
This article is suitable for:
The traditional h5 has only one end, the browser. The uni-app can span multiple terminals, although it is still a front-end, which is different from the traditional h5. If you are familiar with h5, you can quickly learn about uni-app through this article.
In the past, most of the web pages were b/s, and the server-side code was mixed in the page;
Now it is c/s, the front and back ends are separated, json data is obtained through js api (similar to uni.request of ajax), and the data is bound to the interface for rendering.
It used to be a .html file, the development was also html, and the operation was also html. Now it is a .vue file, and the development is vue. After compilation, the runtime has become a js file. Modern front-end development rarely uses HTML directly, but basically develops, compiles, and runs. So uni-app has the concept of compiler and runtime.
In the past, there was a large html node with script and style nodes in it;
Now template is a first-level node, used to write tag components, script and style are parallel first-level nodes, that is, there are 3 first-level nodes.
before
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8" />
<title></title>
<script type="text/javascript">
</script>
<style type="text/css">
</style>
</head>
<body>
</body>
</html>
Now. This is called vue single-file component specification sfc
<template>
<view>
注意必须有一个view,且只能有一个根view。所有内容写在这个view下面。
</view>
</template>
<script>
export default {
}
</script>
<style>
</style>
Previously introduced external js and css through script src and link href;
Now it is written in es6, import introduces external js modules (not files) or css
before
<script src="js/jquery-1.10.2.js" type="text/javascript"></script>
<link href="css/bootstrap.css" rel="stylesheet" type="text/css"/>
Now
There is a tool class util.js
in the common directory of hello uni-app, you can search for this example in hello uni-app to view.
<script>
var util = require('../../../common/util.js'); //require这个js模块
var formatedPlayTime = util.formatTime(playTime); //调用js模块的方法
</script>
In this util.js
, the previous function should be encapsulated as an object method
function formatTime(time) {
return time;//这里没写逻辑
}
module.exports = {
formatTime: formatTime
}
Of course there are some advanced usages
var dateUtils = require('../../../common/util.js').dateUtils; //直接使用js模块的属性。在hello uni-app有示例
import * as echarts from '/components/echarts/echarts.simple.min.js'; //将js导入并重命名为echarts,然后使用echarts.来继续执行方法。在hello uni-app有示例
<style>
@import "./common/uni.css";
.uni-hello-text{
color:#7A7E83;
}
</style>
The global style is written in app.vue in the root directory, and each page will load the style in app.vue.
The following is a component library that imports an angle label, which displays an abc on the page and has a digital angle label 1 in the upper right corner, see details
<template>
<view>
<uni-badge text="abc" :inverted="true"></uni-badge><!--3.使用组件-->
</view>
</template>
<script>
import uniBadge from "../../../components/uni-badge.vue";//1.导入组件(这步属于传统vue规范,但在uni-app的easycom下可以省略这步)
export default {
data() {
return {
}
},
components: {
uniBadge //2.注册组件(这步属于传统vue规范,但在uni-app的easycom下可以省略这步)
}
}
</script>
If you need to import vue components globally, that is, each page can be used directly without reference and registration, it is processed in main.js in the project root directory. The following is an example in hello uni-app.
//main.js
import pageHead from './components/page-head.vue' //导入
Vue.component('page-head', pageHead) //注册。注册后在每个vue的page页面里可以直接使用<page-head></page-head>组件。
The above-mentioned component usage methods belong to the concept of traditional vue. After uni-app 2.7, a simpler technology for using components was introduced easycom, no need to reference and register components, and use components directly in the template area That's it.
Formerly html tags, such as <div>
, are now MiniApp components, such as <view>
.
So what's the difference between label
and component
, aren't they all surrounded by angle brackets?
In fact, the label is an old concept, and the label belongs to the built-in thing of the browser. Components, however, can be freely extended.
Similar to you can encapsulate a piece of js into a function or module, you can also encapsulate a ui control into a component.
uni-app
refers to the MiniApp specification and provides a set of built-in components.
The following is the mapping table of html tags and uni-app built-in components:
In addition to the changes, a number of new components commonly used on mobile phones have been added
scroll-view Regionable scroll view container
icon icon
rich-text rich text (not executable js, but can render various text formats and images)
progress progress bar
slider slider indicator
switch switch selector
camera camera
live-player live
map map
cover-view a view container that covers native components
Cover-view needs to be emphasized a few more words. The video, map, canvas, and textarea of the non-h5 end of uni-app are native components, and the level is higher than other components. To cover native components, you need to use the cover-view component. For details, see Level Introduction
In addition to built-in components, there are many open source extension components that encapsulate common operations. DCloud has established a plug-in market to include these extension components. For details, see plug-in market
js changes are divided into three parts: operating environment changes, data binding mode changes, and api changes.
Standard js syntax and api are supported, such as if, for, settimeout, indexOf, etc.
However, browser-specific window, document, navigator, and location objects, including cookie storage, are only available in browsers, and are not supported by apps and MiniApp.
Some people may think that js is equivalent to js in the browser. In fact, js is managed by the ECMAScript organization, and the js in the browser is the w3c organization supplemented with special objects such as window, document, navigator, and location based on the js specification.
In each end of uni-app, except for the h5 end, the js of the other end runs under an independent v8 engine, not in the browser, so the objects of the browser cannot be used. If you have done MiniApp development, you should know this very well.
This means that many HTML libraries that rely on document, such as jquery, cannot be used.
Of course, apps and MiniApp support web-view components, which can load standard HTML. This kind of page still supports browser-specific objects window, document, navigator, and location.
Now the front-end trend is to de-domize, switch to mvvm mode, write more concisely, greatly reduce the number of lines of code, and at the same time have better differential rendering performance.
uni-app uses vue's data binding method to solve the problem of interaction between js and dom interface.
If you want to change the display content of a dom element, such as the display text of a view:
In the past, the id was set for the view, and then the dom element was obtained through the selector in js, and the assignment operation was further performed through js to modify the attribute or value of the dom element.
The following demonstrates a piece of code. There is a displayed text area and a button on the page. After clicking the button, the value of the text area will be modified.
<html>
<head>
<script type="text/javascript">
document.addEventListener("DOMContentLoaded",function () {
document.getElementById("spana").innerText="456"
})
function changetextvalue () {
document.getElementById("spana").innerText="789"
}
</script>
</head>
<body>
<span id="spana">123</span>
<button type="button" onclick="changetextvalue()">修改为789</button>
</body>
</html>
The current practice is the binding mode of vue, bind a js variable to the dom element, modify the value of the js variable in the script, the dom will automatically change, and the page will automatically update and render
<template>
<view>
<text>{{textvalue}}</text><!-- 这里演示了组件值的绑定 -->
<button :type="buttontype" @click="changetextvalue()">修改为789</button><!-- 这里演示了属性和事件的绑定 -->
</view>
</template>
<script>
export default {
data() {
return {
textvalue:"123",
buttontype:"primary"
};
},
onLoad() {
this.textvalue="456"//这里修改textvalue的值,其实123都来不及显示就变成了456
},
methods: {
changetextvalue() {
this.textvalue="789"//这里修改textvalue的值,页面自动刷新为789
}
}
}
</script>
Note the data(): {return { }}
in export default {}
in the above code.
In the design of Vue, the data that needs to be bound in the page is stored here, and it can be correctly bound and rendered by the interface when it is written in the data.
Note: The vue page of uni-app is the single-file component specification of vue. According to the definition of vue, only functions are accepted and must be wrapped with return.
If you have learned the data binding of MiniApp, but don't know vue, pay attention to:
From the above example, it can also be seen that the writing of events has changed.
onclick="changetextvalue()"
in the above codemethods: {}
in export default {}
of js, and then use @click="changetextvalue()"
in the componentIn js, the level with data and methods, such as onload()
in the above sample code, is called the life cycle. The life cycle in a normal vue page is called the page life cycle. The life cycle in the app.vue file in the project root directory is called the application life cycle.
In addition to onload
, there are many life cycles such as onready
, see uni-app life cycle
In advanced usage, vue supports setting ref (reference mark) to components, which is similar to setting an id to a dom element in html, and then this.$refs.xxx
can also be used to get it in js. as follows:
<template>
<view>
<view ref="testview">11111</view>
<button @click="getTest">获取test节点</button>
</view>
</template>
<script>
export default {
methods: {
getTest() {
console.log(this.$refs.testview)
}
}
};
</script>
Because the api of uni-app refers to the MiniApp, it is very different from the js api of the browser, such as
There are many js apis of uni-app, but they are basically the apis of MiniApp. Just change wx.xxx to uni.xxx. See details
uni-app supports conditional compilation on different sides, and can use the unique api of each side without restriction, see conditional compilation for details
Standard CSS is basically supported.
The selector has 2 changes: * The selector is not supported; there is no body in the element selector, and it is changed to page. This is the case with WeChat MiniApp.
page{
}
In terms of units, px cannot dynamically adapt to screens of different widths, and rem cannot be used for nvue/weex. If you want to use a unit that adapts to the screen width, it is recommended to use rpx, which is fully supported. Dimension Units Documentation
uni-app recommends using flex layout, which is a bit different from traditional flow layout. But the feature of flex is that no matter what technology supports this type of layout, web, MiniApp/ QuickApp, weex/rn, native iOS, and Android development all support flex. It is a new generation layout scheme that takes all ends. For related tutorials, please learn from Baidu.
uni-app's vue file supports all web layout methods, whether streaming or flex. But in nvue, only flex is supported because it is rendered using the native typesetting engine on the app side.
Pay attention to the background image and font file in css, try not to be larger than 40k, because it will affect the performance. On the MiniApp side, if it is larger than 40k, it needs to be placed in the server-side remote reference or imported after base64, and cannot be placed locally as an independent file reference.
The engineering structure of uni-app has separate requirements, [see details](https://uniapp.dcloud.io/frame?id=%e7%9b%ae%e5%bd%95%e7%bb%93%e6 %9e%84)
Every displayable page must be registered in pages.json. If you have developed MiniApp, then pages.json is similar to app.json. If you are familiar with vue, there is no vue routing here, it is all managed in pages.json.
The homepage of the original project is generally index.html or default.html, which is configured in the web server. The home page of uni-app is configured in pages.json, and the first page under the page node is the home page. Usually in the /pages/xx directory.
In the app and MiniApp, in order to improve the experience, the page provides a native navigation bar and bottom tabbar. Note that these configurations are done in pages.json, not in the vue page, but the click event listener is displayed on the vue page. do in.
If you are familiar with MiniApp development, the comparison changes are as follows:
Finally, this article is not a complete tutorial of uni-app. To understand and master uni-app, you need to go through the documentation of uni-app carefully.
To master vue well, you still need to go to vue official website to learn further. Or through the uni-app professional video training course, learn together with vue and uni-app.
According to the data of Tencent Classroom and Job Friend Collection, people who know Vue have a 27% higher salary than ordinary front-end people who do not know Vue.
If you are familiar with MiniApp but not Vue, here is another article that sums it up well: Differences and Comparisons between Vue and WeChat MiniApp