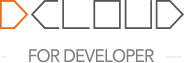
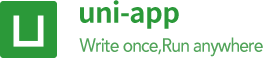
English
获取当前的地理位置、速度
name | type | required | default | description |
---|---|---|---|---|
options | GetLocationOptions | YES | - | - |
name | type | optinal | default | description |
---|---|---|---|---|
type | string | NO | wgs84 | 默认为 wgs84 返回 gps 坐标,gcj02 返回可用于uni.openLocation的坐标 |
altitude | boolean | NO | false | 传入 true 会返回高度信息,由于获取高度需要较高精确度,会减慢接口返回速度 |
geocode | boolean | NO | false | 传入 true 会解析地址 |
highAccuracyExpireTime | number | NO | 3000 | 高精度定位超时时间(ms),指定时间内返回最高精度,该值3000ms以上高精度定位才有效果 |
isHighAccuracy | boolean | NO | false | 开启高精度定位 |
success | (result: GetLocationSuccess) => void | NO | - | 接口调用成功的回调函数 |
fail | (result: IGetLocationFail) => void | NO | - | 接口调用失败的回调函数 |
complete | (result: any) => void | NO | - | 接口调用结束的回调函数(调用成功、失败都会执行) |
name | type | optinal | default | description |
---|---|---|---|---|
latitude | number | YES | 0 | 纬度,浮点数,范围为-90~90,负数表示南纬 |
longitude | number | YES | 0 | 经度,范围为-180~180,负数表示西经 |
speed | number | YES | 0 | 速度,浮点数,单位m/s |
accuracy | number | YES | - | 位置的精确度 |
altitude | number | YES | 0 | 高度,单位 m |
verticalAccuracy | number | YES | 0 | 垂直精度,单位 m(Android 无法获取,返回 0) |
horizontalAccuracy | number | YES | 0 | 水平精度,单位 m |
address | any | NO | null | 地址信息 |
name | type | optinal | default | description |
---|---|---|---|---|
errCode | 1505004 | 1505021 | 1505022 | 1505023 | 1505024 | YES | - | 错误码 - 1505004 缺失权限 - 1505021 超时 - 1505022 不支持的定位类型 - 1505023 不支持逆地理编码 - 1505024 没有找到具体的定位引擎,请定位开关是否已打开 |
errSubject | string | YES | - | 统一错误主题(模块)名称 |
data | any | NO | - | 错误信息中包含的数据 |
errMsg | string | YES | - | - |
Android version | Android uni-app | Android uni-app-x | iOS version | iOS uni-app | iOS uni-app-x |
---|---|---|---|---|---|
4.4.4 | √ | 3.9.0 | x | x | x |
uni-app x的定位,默认是系统定位,system。
system的定位仅支持wgs84坐标系、不支持逆地址解析、且某些老型号国产Android机因gms问题不支持系统定位、国产Rom可能不支持高度信息。
如需更强的定位能力,需加载专业定位sdk。
真机运行基座不包含三方定位sdk。
三方定位sdk方面,暂不支持高德、百度,但支持腾讯定位。
可下载腾讯定位插件,在插件中配置key打包后生效。
上述腾讯定位插件属于ext api插件,引用到工程后,会覆盖uni.getLocation的实现,替换掉系统定位。
如需其他定位,请在插件市场搜索定位相关的uts插件。
获取手机端app是否拥有定位权限,请使用API uni.getAppAuthorizeSetting
不管系统定位、还是三方sdk定位,都有很多注意事项,包括gms、坐标系、隐私和权限等,请仔细阅读下面的参考链接。
<template>
<view>
<page-head :title="title"></page-head>
<view style="padding: 4px;">
<text class="hello-text">
真机运行标准基座仅包含系统定位,即system。\n
部分手机因gms兼容不好可能导致无法定位。\n
gcj国标、逆地理信息等功能需三方sdk定位。如果需要类似能力可以下载腾讯定位插件,打包自定义基座。参考示例:</text>
<u-link :href="'https://ext.dcloud.net.cn/plugin?id=14569'" :text="'https://ext.dcloud.net.cn/plugin?id=14569'" :inWhiteList="true"></u-link>
</view>
<view class="uni-padding-wrap uni-common-mt">
<view class="uni-list">
<radio-group @change="radioChange">
<radio class="uni-list-cell uni-list-cell-pd" v-for="(item, index) in items" :key="item.value"
:class="index < items.length - 1 ? 'uni-list-cell-line': ''" :value="item.value"
:checked="index === current">
{{item.name}}
</radio>
</radio-group>
</view>
<view class="uni-list-cell uni-list-cell-pd">
<view class="uni-list-cell-db">高度信息</view>
<switch :checked="altitudeSelect" @change="altitudeChange" />
</view>
<view class="uni-list-cell uni-list-cell-pd">
<view class="uni-list-cell-db">开启高精度定位</view>
<switch :checked="isHighAccuracySelect" @change="highAccuracySelectChange" />
</view>
<view class="uni-list-cell uni-list-cell-pd">
<view class="uni-list-cell-db">是否解析地址信息</view>
<switch :checked="geocodeSelect" @change="geocodeChange" />
</view>
<text>{{exeRet}}</text>
<view class="uni-btn-v">
<button class="uni-btn" type="default" @tap="getLocationTap">获取定位</button>
</view>
</view>
</view>
</template>
<script lang="uts">
type ItemType = {
value : string,
name : string,
}
export default {
data() {
return {
title: 'get-location',
altitudeSelect: false,
isHighAccuracySelect: false,
geocodeSelect: false,
exeRet: '',
items: [
{
value: 'wgs84',
name: 'wgs84'
},
{
value: 'gcj02',
name: 'gcj02'
}
] as ItemType[],
current: 0,
}
},
methods: {
altitudeChange: function (e : SwitchChangeEvent) {
this.altitudeSelect = e.detail.value
},
geocodeChange: function (e : SwitchChangeEvent) {
this.geocodeSelect = e.detail.value
},
highAccuracySelectChange: function (e : SwitchChangeEvent) {
this.isHighAccuracySelect = e.detail.value
},
radioChange(e : RadioGroupChangeEvent) {
for (let i = 0; i < this.items.length; i++) {
if (this.items[i].value === e.detail.value) {
this.current = i;
break;
}
}
},
getLocationTap: function () {
uni.showLoading({
title: '定位中'
})
uni.getLocation(({
type: this.items[this.current].value,
altitude: this.altitudeSelect,
isHighAccuracy: this.isHighAccuracySelect,
geocode: this.geocodeSelect,
success: (res : any) => {
uni.hideLoading()
console.log(res);
this.exeRet = JSON.stringify(res)
},
fail: (res : any) => {
uni.hideLoading()
console.log(res);
this.exeRet = JSON.stringify(res)
},
complete: (res : any) => {
uni.hideLoading()
console.log(res);
this.exeRet = JSON.stringify(res)
}
}));
}
}
}
</script>
<style>
@import '@/common/uni-uvue.css';
</style>
name | type | optinal | default | description |
---|---|---|---|---|
errMsg | string | YES | - | 错误信息 |