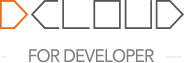
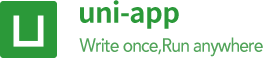
English
获取 APP 授权设置。
Type |
---|
GetAppAuthorizeSettingResult |
name | type | optinal | default | description |
---|---|---|---|---|
cameraAuthorized | string | YES | - | 允许 App 使用摄像头的开关 - authorized: 已经获得授权,无需再次请求授权 - denied: 请求授权被拒绝,无法再次请求授权;(此情况需要引导用户打开系统设置,在设置页中打开权限) - not determined: 尚未请求授权,会在App下一次调用系统相应权限时请求;(仅 iOS 会出现。此种情况下引导用户打开系统设置,不展示开关) - config error: Android平台:表示没有授予 android.permission.CAMERA 权限;iOS平台没有该值 |
locationAuthorized | string | YES | - | 允许 App 使用定位的开关 - authorized: 已经获得授权,无需再次请求授权 - denied: 请求授权被拒绝,无法再次请求授权;(此情况需要引导用户打开系统设置,在设置页中打开权限) - not determined: 尚未请求授权,会在App下一次调用系统相应权限时请求;(仅 iOS 会出现。此种情况下引导用户打开系统设置,不展示开关) - config error: Android平台:表示没有授予 android.permission.ACCESS_COARSE_LOCATION 权限;iOS平台:表示没有在 manifest.json -> App模块配置 中配置 Geolocation(定位) 模块 |
locationAccuracy | string | NO | - | 定位准确度。true 表示模糊定位,false 表示精确定位 - reduced: 模糊定位 - full: 精准定位 - unsupported: 不支持(包括用户拒绝定位权限和没有在 manifest.json -> App模块配置 中配置 Geolocation(定位) 模块) |
microphoneAuthorized | string | YES | - | 允许 App 使用麦克风的开关 - authorized: 已经获得授权,无需再次请求授权 - denied: 请求授权被拒绝,无法再次请求授权;(此情况需要引导用户打开系统设置,在设置页中打开权限) - not determined: 尚未请求授权,会在App下一次调用系统相应权限时请求;(仅 iOS 会出现。此种情况下引导用户打开系统设置,不展示开关) - config error: Android平台:表示没有授予 android.permission.RECORD_AUDIO 权限;iOS平台没有该值 |
notificationAuthorized | string | YES | - | 允许 App 通知的开关 - authorized: 已经获得授权,无需再次请求授权 - denied: 请求授权被拒绝,无法再次请求授权;(此情况需要引导用户打开系统设置,在设置页中打开权限) - not determined: 尚未请求授权,会在App下一次调用系统相应权限时请求;(仅 iOS 会出现。此种情况下引导用户打开系统设置,不展示开关) - config error: Android平台没有该值;iOS平台:表示没有在 manifest.json -> App模块配置 中配置 Push(推送) 模块 |
Android version | Android uni-app | Android uni-app-x | iOS version | iOS uni-app | iOS uni-app-x |
---|---|---|---|---|---|
4.4 | √ | 3.9+ | 9.0 | √ | x |
<template>
<view>
<page-head :title="title"></page-head>
<view class="uni-common-mt">
<view class="uni-list">
<view class="uni-list-cell">
<view class="uni-pd">
<view class="uni-label" style="width:180px;">是否授权使用摄像头</view>
</view>
<view class="uni-list-cell-db">
<input class="uni-input" type="text" :disabled="true" placeholder="未获取" :value="cameraAuthorized"/>
</view>
</view>
<view class="uni-list-cell">
<view class="uni-pd">
<view class="uni-label" style="width:180px;">是否授权使用定位</view>
</view>
<view class="uni-list-cell-db">
<input class="uni-input" type="text" :disabled="true" placeholder="未获取" :value="locationAuthorized"/>
</view>
</view>
<view class="uni-list-cell">
<view class="uni-pd">
<view class="uni-label" style="width:180px;">定位准确度</view>
</view>
<view class="uni-list-cell-db">
<input class="uni-input" type="text" :disabled="true" placeholder="未获取" :value="locationAccuracy"/>
</view>
</view>
<view class="uni-list-cell">
<view class="uni-pd">
<view class="uni-label" style="width:180px;">是否授权使用麦克风</view>
</view>
<view class="uni-list-cell-db">
<input class="uni-input" type="text" :disabled="true" placeholder="未获取" :value="microphoneAuthorized"/>
</view>
</view>
<view class="uni-list-cell">
<view class="uni-pd">
<view class="uni-label" style="width:180px;">是否授权通知</view>
</view>
<view class="uni-list-cell-db">
<input class="uni-input" type="text" :disabled="true" placeholder="未获取" :value="notificationAuthorized"/>
</view>
</view>
</view>
<view class="uni-padding-wrap">
<view class="uni-btn-v">
<button type="primary" @tap="getAppAuthorizeSetting">获取App授权设置</button>
</view>
</view>
</view>
</view>
</template>
<script>
export default {
data() {
return {
title: 'getAppAuthorizeSetting',
cameraAuthorized:"",
locationAuthorized:"",
locationAccuracy:"",
microphoneAuthorized:"",
notificationAuthorized:""
}
},
onUnload:function(){
},
methods: {
getAppAuthorizeSetting: function () {
const res = uni.getAppAuthorizeSetting();
this.cameraAuthorized = res.cameraAuthorized;
this.locationAuthorized = res.locationAuthorized;
this.locationAccuracy = res.locationAccuracy ?? "unsupported";
this.microphoneAuthorized = res.microphoneAuthorized;
this.notificationAuthorized = res.notificationAuthorized;
}
}
}
</script>
<style>
.uni-pd {
padding-left: 30rpx;
}
</style>
name | type | optinal | default | description |
---|---|---|---|---|
errMsg | string | YES | - | 错误信息 |