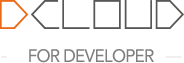
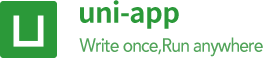
uni-app
uses the syntax of Vue
.
In order to achieve multi-terminal compatibility, factors such as compilation speed and running performance are comprehensively considered. uni-app
has the following development specifications:
Vue.js
specification, while supplementing the life cycle of App and pageA uni-app project, which contains the following directories and files by default:
┌─components ...
│ └─comp-a.vue ...
├─hybrid ...
├─platforms ...
├─pages ...
│ ├─index
│ │ └─index.vue ...
│ └─list
│ └─list.vue ...
├─static ...
├─uni_modules ...
├─main.js ...
├─App.vue ...
├─manifest.json ...
└─pages.json ...
Tips
static
directory will be fully packaged and will not be compiled. Files (vue, js, css, etc.) not in the static
directory will be packaged and compiled only when they are referenced.js
file in the static
directory will not be compiled. If there is es6
code in it, it will report an error on the mobile device if it is run without conversion.css
, less/scss
and other resources should not be placed in the static
directory. It is recommended that these public resources be placed in the self-built common
directory.Valid directory | Instruction |
---|---|
app-plus | App |
h5 | H5 |
When introducing static resources into
template
, such asimage
,video
and other tags of thesrc
attribute, you can use a relative path or an absolute path in the form as follows
<!-- For absolute path, /static refers to the static directory under the root directory. For cli project, /static refers to the static directory under the src directory -->
<image class="logo" src="/static/logo.png"></image>
<image class="logo" src="@/static/logo.png"></image>
<!-- Relative path -->
<image class="logo" src="../../static/logo.png"></image>
Notice
@
will be verified by base64 conversion rulesHBuilderX 2.6.6
, template
supports the introduction of static resources in the path starting with @
, which is not supported by the old version.HBuilderX 2.6.9
on the App platform, when static resource files (such as pictures) are referenced in the template
node, adjust the search strategy to [Search based on the path of the current file], which is consistent with other platformsWhen importing
js
files injs
files orscript
tags (including renderjs, etc.), you can use relative paths and absolute paths in the following form
//Absolute path, @ points to the root directory of the project, and in cli projects @ points to the src directory
import add from '@/common/add.js'
// Relative path
import add from '../../common/add.js'
Notice
/
For
css
files or whencss
files are introduced instyle label
(same for scss and less files), you can use relative or absolute paths (HBuilderX 2.6.6
)
/* Absolute path */
@import url('/common/uni.css');
@import url('@/common/uni.css');
/* Relative path */
@import url('../../common/uni.css');
Notice
HBuilderX 2.6.6
, static resources are imported using absolute paths, which is not supported by the old version.For
css
files or pictures referenced instyle label
, you can use a relative path or an absolute path.
/* Absolute path */
background-image: url(/static/logo.png);
background-image: url(@/static/logo.png);
/* Relative path */
background-image: url(../../static/logo.png);
Tips
@
will be verified by base64 conversion rulesuni-app
supports application life cycle functions such as onLaunch, onShow and onHide. Please refer to Application life cycle for details.
uni-app
supports life cycle functions such as onLoad, onShow and onReady. Please refer to Page life cycle for details.
uni-app
page routing is managed in a unified way as a framework, and developers need to configure the path and page style of each routing page in pages.json. Therefore, the routing usage of uni-app
is different from Vue Router
. If you still want to use Vue Router
to manage routing, you can search for Vue-Router (opens new window) in the plug-in market.
uni-app
has two page routing jump methods: jump through the navigator component, and jump through the API.
The framework manages all current pages in the form of stack. When a route switch occurs, the page stack behaves as follows:
Routing mode | Page stack performance | Trigger timing |
---|---|---|
Initialization | New page onto the stack | The first page opened by uni-app |
Open a new page | New page onto the stack | Call API uni.navigateTo, use component <navigator open-type="navigate"/> |
Page redirection | The current page is out of the stack, and the new page is in the stack | Call API uni.redirectTo, use component <navigator open-type="redirectTo"/> |
Page return | The page is constantly popped until the target returns to the page | Call API uni.navigateBack , use component <navigator open-type="navigateBack"/>, user presses back button in the upper left corner, Android user presses back button |
Tab switching | All the pages are out of the stack, leaving only the new Tab page | Call API uni.switchTab, use component <navigator open-type="switchTab"/>, user switches Tab |
Reload | All the pages are out of the stack, leaving only the new page | Call API uni.reLaunch, Use component <navigator open-type="reLaunch"/> |
You can use process.env.NODE_ENV
to determine whether the current environment of uni-app
is a development environment or a production environment. Generally, it is used for dynamic switching of connecting test server or production server.
if(process.env.NODE_ENV === 'development'){
console.log ('development environment')
}else{
console.log ('production environment')
}
If more environments need to be customized, such as test environment:
Shortcut code block
Type in the code blocks uEnvDev
and uEnvProd
in HBuilderX, then you can quickly generate code for determining the operating environment corresponding to development
and production
.
// uEnvDev
if (process.env.NODE_ENV === 'development') {
// TODO
}
// uEnvProd
if (process.env.NODE_ENV === 'production') {
// TODO
}
There are 2 scenarios for platform judgment, one is at compile time and the other is at run time.
// #ifdef H5
alert ("Only H5 platform has alert method")
// #endif
The above code will only be compiled to the distribution package of H5 (not other platforms).
uni.getSystemInfoSync().platform
to judge whether the client environment is Android or iOS.switch(uni.getSystemInfoSync().platform){
case 'android':
console.log ('Run on Android')
break;
case 'ios':
console.log ('Run on iOS')
break;
default:
Console.log ('Run on developer tools')
break;
}
If necessary, you can also define a variable and assign it different values in conditional compilation. Dynamically judge the environment in the subsequent running code.
For the definition of other environment variables, please refer to Environment variables.
css of uni-app is basically the same as that of web. css usage is not covered in this article. Based on your knowledge of web css, this article also describes some style-related considerations.
There are vue page and nvue page in uni-app. vue page is rendered by webview, and that of the app side is rendered natively. Styles are more restricted in nvue pages than in the web. See also Nvue style specific document
In this article, attention is placed on the style considerations of the vue page.
Common css units supported by uni-app
include px, rpx
vue page supports the following common H5 units, but not support in nvue:
nvue does not support percentage units yet.
On the App side, only px is supported for the units involved in titleNView in pages.json. Note that rpx is not supported at this time
In nvue, px and rpx can be used in uni-app mode (Introduction to different compilation modes of nvue (opens new window)), with the same performance as in vue. weex mode currently follows the unit of weex, and its unit is a little bit special:
The following is a detailed description of rpx
:
Designers generally only provide drawing with one resolution while providing design drawing.
If you develop strictly according to the px marked by the design icon, the interface is easily deformed on mobile phones of different widths.
Width deformation is dominant. Generally, the height is not easy to go wrong on account of the scroll bar. As a result, a strong demand for dynamic width unit is triggered.
uni-app
supports rpx
on both the App side and H5 side, and configures different screen width calculation methods. For details, please refer to: rpx calculation configuration (opens new window).
rpx is a unit relative to the reference width, which can be adapted to the screen width. uni-app
The specified screen reference width is 750rpx.
Developers can calculate the rpx value of page elements based on the reference width of design draft. The conversion formula between design draft 1px and frame style 1rpx is as follows:
Design draft 1px / design draft baseline width = frame style 1rpx / 750rpx
In other words, the formula for calculating the width of the page element width in uni-app
is as follows:
750 * element width in design draft/Design draft baseline width
For example:
uni-app
should be set to: 750 * 100 / 750
, the result is: 100rpx.uni-app
should be set to: 750 * 100 / 640
, the result is: 117rpx.uni-app
should be set to: 750 * 200 / 375
, the result is: 400rpx.Tips
The @import
sentence can be used to import an external style sheet, @import
is followed by the relative path of the external style sheet that needs to be imported, and ;
indicates the end of the sentence.
Sample code:
<style>
@import "../../common/uni.css";
.uni-card {
box-shadow: none;
}
</style>
Frame components support the use of style and class attributes to control the style of components.
<view :style="{color:color}" />
<view class="normal_view" />
Currently supported selectors are:
Selector | Example | Example description |
---|---|---|
.class | .intro | Select all components with class="intro" |
#id | #firstname | Select the component with id="firstname" |
element | view | Select all view components |
element, element | view, checkbox | Select view component of all documents and all checkbox components |
::after | view::after | Insert content behind the view component, Only vue page is valid |
::before | view::before | Insert content in front of the view component, Only vue page is effective |
Notice:
You cannot use the *
selector in uni-app
.
page
is equivalent to the body
node, for example:
<!-- Set the background color of the page. Using scoped may cause invalidation -->
page {
background-color:#ccc;
}
The styles defined in App.vue are global styles, which act on every page. The styles defined in the vue file in the pages directory are local styles, which only act on the corresponding pages and will cover the same selectors in App.vue.
Notice:
@import
statement, which also applies to every page.uni-app provides built-in CSS variables
CSS variables | Describe | App | H5 |
---|---|---|---|
--status-bar-height | Height of system status bar | See below for height of system status bar and attentions for nvue | 0 |
--window-top | Distance of the content area from the top | 0 | Height of NavigationBar |
--window-bottom | Distance of the content area from the bottom | 0 | Height of TabBar |
Notice:
var(--status-bar-height)
represents the height of the actual status bar of the phone in the App."navigationStyle":"custom"
to cancel the native navigation bar, the status bar position is occupied because the window is immersive. At this time, a view with a height of var(--status-bar-height)
can be placed at the top of the page to prevent the page content from appearing in the status bar.--window-bottom
, no matter which end it is, it is fixed above the tabbar.--status-bar-height
variable on the App side. The alternative is to obtain the status bar height through uni.getSystemInfoSync().statusBarHeight when the page is onLoad, and then set the height of the placeholder view through style binding. Sample code is provided belowCode block
How to quickly write css variables: type hei in css to display 3 css variables in the candidate assistant. (supported by HBuilderX 1.9.6+)
Example 1 - use css variables on ordinary pages:
<template>
<!-- page-meta is added to HBuilderX 2.6.3+. See details: https://uniapp.dcloud.io/component/page-meta -->
<page-meta>
<navigation-bar />
</page-meta>
<view>
<view class="status_bar">
<!-- Here is the status bar -->
</view>
<view> Text under the status bar </view>
</view>
</template>
<style>
.status_bar {
height: var(--status-bar-height);
width: 100%;
}
</style>
<template>
<view>
<view class="toTop">
<!-- An up arrow can be put here, which shifts up 10px from the bottom tabbar-->
</view>
</view>
</template>
<style>
.toTop {
bottom: calc(var(--window-bottom) + 10px)
}
</style>
Example 2 - get the height of status bar on nvue page
<template>
<view class="content">
<view :style="{ height: iStatusBarHeight + 'px'}"></view>
</view>
</template>
<script>
export default {
data() {
return {
iStatusBarHeight:0
}
},
onLoad() {
this.iStatusBarHeight = uni.getSystemInfoSync().statusBarHeight
}
}
</script>
The height of the following components in uni-app
is fixed and cannot be modified:
Component | Describe | App | H5 |
---|---|---|---|
NavigationBar | Navigation bar | 44px | 44px |
TabBar | Bottom tab | 56px before HBuilderX 2.3.4. From 2.3.4+, it was in line with H5, unified to 50px. BBut you can change the height voluntarily) | 50px |
To support cross-platform, it is recommended that the framework adopts Flex layout. For Flex layout, please refer to external documents A Complete Guide to Flexbox (opens new window), Ruan Yifeng's flex tutorial (opens new window), etc.
uni-app
supports being used to set a background image in css in the same way as ordinary web
projects, but the following points should be noted:
uni-app
will be automatically converted to base64 format when compiled to a platform that does not support local background images; .test2 {
background-image: url('~@/static/logo.png');
}
uni-app
supports the use of font icons in the same way as ordinary web
projects, but the following points should be noted:
https
.uni-app
will automatically convert it to base64 format; @font-face {
font-family: test1-icon;
src: url('~@/static/iconfont.ttf');
}
You cannot import font files in nvue
directly using CSS. Instead, you need to import font files in js using the following method. nvue does not support importing fonts from local paths, please use network links or the form of base64
. In the src
field, single quotation marks must be used within the brackets of url
.
var domModule = weex.requireModule('dom');
domModule.addRule('fontFace', {
'fontFamily': "fontFamilyName",
'src': "url('https://...')"
})
Example:
<template>
<view>
<view>
<text class="test"></text>
<text class="test"></text>
<text class="test"></text>
</view>
</view>
</template>
<style>
@font-face {
font-family: 'iconfont';
src: url('https://at.alicdn.com/t/font_865816_17gjspmmrkti.ttf') format('truetype');
}
.test {
font-family: iconfont;
margin-left: 20rpx;
}
</style>
<template/>
and <block/>
uni-app
supports nesting of <template/>
and <block/>
in the template for list rendering and conditional rendering.
<template/>
and <block/>
are not a component but just a packaging element. They will not be rendered on the page and only accept control attributes.
<block/>
has certain differences in performance on different platforms. It is recommended to use <template/>
uniformly.
Code example
<template>
<view>
<template v-if="test">
<view>Display when test is true</view>
</template>
<template v-else>
<view>Display when test is false</view>
</template>
</view>
</template>
<template>
<view>
<block v-for="(item,index) in list" :key="index">
<view>{{item}} - {{index}}</view>
</block>
</view>
</template>
uni-app supports not only most ES6 API, but also await/async of ES7.
For the support of ES6 API, see the following table for details (x
means no support, and no special instructions means support):
String | iOS8 | iOS9 | iOS10 | Android |
---|---|---|---|---|
codePointAt | ||||
normalize | x | x | Only NFD/NFC is supported | |
includes | ||||
startsWith | ||||
endsWith | ||||
repeat | ||||
String.fromCodePoint |
Array | iOS8 | iOS9 | iOS10 | Android |
---|---|---|---|---|
copyWithin | ||||
find | ||||
findIndex | ||||
fill | ||||
entries | ||||
keys | ||||
values | x | x | ||
includes | x | |||
Array.from | ||||
Array.of |
Number | iOS8 | iOS9 | iOS10 | Android |
---|---|---|---|---|
isFinite | ||||
isNaN | ||||
parseInt | ||||
parseFloat | ||||
isInteger | ||||
EPSILON | ||||
isSafeInteger |
Math | iOS8 | iOS9 | iOS10 | Android |
---|---|---|---|---|
trunc | ||||
sign | ||||
cbrt | ||||
clz32 | ||||
imul | ||||
fround | ||||
hypot | ||||
expm1 | ||||
log1p | ||||
log10 | ||||
log2 | ||||
sinh | ||||
cosh | ||||
tanh | ||||
asinh | ||||
acosh | ||||
atanh |
Object | iOS8 | iOS9 | iOS10 | Android |
---|---|---|---|---|
is | ||||
assign | ||||
getOwnPropertyDescriptor | ||||
keys | ||||
getOwnPropertyNames | ||||
getOwnPropertySymbols |
Other | iOS8 | iOS9 | iOS10 | Android |
---|---|---|---|---|
Symbol | ||||
Set | ||||
Map | ||||
Proxy | x | x | x | |
Reflect | ||||
Promise |
Notice
Uni-app supports the use of npm to install third-party packages.
This document requires developers to have a certain understanding of npm, so the basic functions of npm will not be introduced. If you do not have access to npm before, please refer to the NPM official document (opens new window).
Initialize npm project
If the npm management dependency has not been used before in the project (no package.json file in the project root directory), please initialize the npm project by executing the command in the project root directory first:
npm init -y
cli project already has package.json by default. The project created by HBuilderX is not available by default and needs to be created by initialization command.
Installation dependencies
Execute the command to install npm package in the root directory of the project:
npm install packageName --save
Usage
You can use npm package after installation, and introduce npm package into js:
import package from 'packageName'
const package = require('packageName')
Notice
To develop using ts in uni-app, please refer to Vue.js TypeScript Support (opens new window).
The type definition file is provided by the @dcloudio/types module. Please pay attention to the compilerOptions> types section in the configuration tsconfig.json file after installation. Missing or wrong type definitions can be added or modified locally and reported to the official in the meantime to ask for updates.
Please note the following when using ts in uni-app.
After declaring lang="ts"
, all vue components imported from the vue file need to be written in ts.
Sample code
Transform uni-badge.vue
<script lang="ts">
//Only the core code that needs to be modified is displayed. For the complete code, please refer to the original components.
import Vue from 'vue';
export default Vue.extend({
props: {
type: {
type: String,
default: 'default'
},
inverted: {
type: Boolean,
default: false
},
text: {
type: [String, Number],
default: ''
},
size: {
type: String,
default: 'normal'
}
},
computed: {
setClass(): string {
const classList: string[] = ['uni-badge-' + this.type, 'uni-badge-size-' + this.size];
if (this.inverted === true) {
classList.push('uni-badge-inverted')
}
return classList.join(" ")
}
},
methods: {
onClick() {
this.$emit('click')
}
}
})
</script>
Reference the uni-badge component in index.vue
<script lang="ts">
import Vue from 'vue';
import uniBadge from '../../components/uni-badge.vue';
export default Vue.extend({
data() {
return {
title: 'Hello'
}
},
components:{
uniBadge
}
});
</script>
renderjs
is a js running in the view layer. Only supported by app-vue and h5.
renderjs
has two main functions:
Platform difference description
App | H5 |
---|---|
√(2.5.5+, only vue is supported) | √ |
Set lang of script node to renderjs
<script module="test" lang="renderjs">
export default {
mounted() {
// ...
},
methods: {
// ...
}
}
</script>
echarts
(opens new window) on app and h5 through renderjsIn addition to the separation of the logic layer and the view layer has many advantages, there is a side effect of blocking communication between the two layers.
renderjs
runs on the view layer and can be used to directly manipulate the elements of the view layer to avoid communication loss.
In the canvas example of hello uni-app, the App side uses renderjs
, and the renderjs
running on the view layer directly manipulates the canvas of the view layer to achieve a smooth canvas animation example. You can experience it in the hello uni-app (opens new window) example.
renderjs
to solve it.In the app-vue environment, the view layer is rendered by the webview, and renderjs
runs on the view layer, which can naturally operate dom and window.
This is an example of running the full version of echart based on renderjs
: renderjs version of echart (opens new window)
In the same way, libraries such as f2
and threejs
can all be used in this way.
uni-app
uses the vue
grammar to develop multi-terminal applications. Sincere thanks should be given to the Vue
team!!