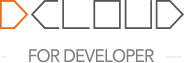
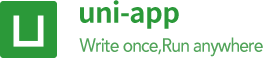
English
The following is the api document of uni-push2.0, business introduction details reference
uni-push
has a server API and a client API.
Obtain the unique push ID of the client
Note: This is an asynchronous method and only supports uni-push2.0;
OBJECT parameter description
Parameter Name | Type | Required | Description |
---|---|---|---|
success | Function | Yes | the callback function called by the interface, see the return parameter description for details |
fail | Function | No | Callback function for interface call failure |
complete | Function | No | The callback function for the end of the interface call (it will be executed when the call succeeds or fails) |
success return parameter description
Parameter | Type | Description |
---|---|---|
cid | String | A push client push id, corresponding to the push_clientid of the uni-id-device table |
errMsg | String | error description |
fail return parameter description
Parameter | Type | Description |
---|---|---|
errMsg | String | error description |
getPushClientId:fail register fail: {\"errorCode\":1,\"errorMsg\":\"\"}
请检查:
示例代码:
uni.getPushClientId({
success: (res) => {
console.log(res.cid);
},
fail(err) {
console.log(err)
}
})
Start listening to push message events 代码示例:
uni.onPushMessage((res)=>{
console.log(res)
})
Name | Type | Description |
---|---|---|
type | String | event type, "click" - the click message from the system push service starts the application event; "receive" - the application receives the push message event from the push server. |
data | String, Object | message content |
Close the push message listening event Sample code:
let callback = (res)=>{
console.log(res)
}
//启动推送事件监听
uni.onPushMessage(callback);
//关闭推送事件监听
uni.offPushMessage(callback);
获取通知渠道管理器,Android 8系统以上才可以设置通知渠道。
返回值说明
类型 |
---|
ChannelManager |
Android 系统版本 | Android | iOS | 其他 |
---|---|---|---|
8.0 | 4.02 | x | x |
渠道管理器
设置推送渠道
名称 | 类型 | 必填 |
---|---|---|
options | SetPushChannelOptions | 是 |
名称 | 类型 | 必备 | 默认值 | 描述 |
---|---|---|---|---|
soundName | string | 否 | null | 声音文件名(不能带文件后缀),需要放置声音文件到Android原生的/res/raw/ 目录下 原生资源配置 |
channelId | string | 是 | - | 通知渠道id |
channelDesc | string | 是 | - | 通知渠道描述 |
enableLights | boolean | 否 | false | 呼吸灯闪烁 |
enableVibration | boolean | 否 | false | 震动 |
importance | number | 否 | 3 | 通知的重要性级别,可选范围IMPORTANCE_LOW:2、IMPORTANCE_DEFAULT:3、IMPORTANCE_HIGH:4 |
lockscreenVisibility | number | 否 | -1000 | 锁屏可见性,可选范围VISIBILITY_PRIVATE:0、VISIBILITY_PUBLIC:1、VISIBILITY_SECRET:-1、VISIBILITY_NO_OVERRIDE:-1000 |
const manager = uni.getChannelManager()
manager.setPushChannel({
channelId: "xxx",
channelDesc: "通知渠道描述",
soundName: "pushsound" // 已经把声音文件存储到/res/raw/pushsound.mp3
})
Android 系统版本 | Android | iOS | 其他 |
---|---|---|---|
8.0 | 4.02 | x | x |
获取当前应用注册的所有的通知渠道。
类型 |
---|
Array |
Android 系统版本 | Android | iOS | 其他 |
---|---|---|---|
8.0 | 4.02 | x | x |
setPushChannel
来进行channel的创建,通过此Api来创建渠道进行推送。客户端创建渠道成功后,即可通过云函数进行推送,uni-push2服务端文档。setPushChannel
时,第一次的设置参数是{"channelId":"test","soundName":"pushsound"}
, 这时你想切换铃音,你的channelId就不能再叫test了,而应该为{"channelId":"test2","soundName":"ring"}
,此时会新建一个渠道。Create a local notification bar message (supported from HBuilderX 3.5.2)
Platform Difference Description
App | H5 | 快应用 | 微信小程序 | 支付宝小程序 | 百度小程序 | 抖音小程序、飞书小程序 | QQ小程序 | 快手小程序 | 京东小程序 |
---|---|---|---|---|---|---|---|---|---|
√ | x | x | x | x | x | x | x | x | x |
OBJECT parameter description
Parameter name | Type | Required | Description |
---|---|---|---|
title | string | No | The title of the push message, the title of the notification message displayed in the system message center, the default value is the name of the program. Android - ALL (supported) iOS - 5.0+ (not supported): It does not support setting the title of the message, it is fixed as the name of the program. |
content | string | yes | The content displayed in the message, the text content displayed in the system notification center. |
payload | string, Object | No | The data carried by the message can be customized according to the business logic. |
icon | string | 否 | 推送消息的图标 本地图片地址,相对路径 - 相对于当前页面的host位置,如"a.jpg",注意当前页面为网络地址则不支持; 绝对路径 - 系统绝对路径,如Android平台"/sdcard/logo.png",此类路径通常通过其它5+ API获取的; 扩展相对路径URL(RelativeURL) - 以"_"开头的相对路径,如"_www/a.jpg"; 本地路径URL - 以“file://”开头,后面跟随系统绝对路径。 Android - 2.3+ (支持) iOS - ALL (不支持): 不支持自定义图片,固定使用应用图标。 |
sound | string | 否 | 显示消息时播放的提示音; 可取值: system 表示使用系统通知提示音,none 表示不使用提示音;(默认值为system)。注意:当程序在前台运行时,提示音不生效。 注:通常应该设置延迟时间,当程序切换到后台才创建本地推送消息时生效 支持的版本:Android 2.3+,iOS - 5.1+。 |
cover | boolean | 否 | 是否覆盖上一次提示的消息 可取值: true 或false ,true为覆盖,false不覆盖,默认为permission中设置的cover值Android - ALL (支持) iOS - 5.0+ (不支持): 不支持覆盖消息,只能创建新的消息。 |
delay | number | No | Delayed display time of the prompt message When the device receives the push message, it may not be displayed immediately, but displayed after a period of delay. The delay time unit is s, and the default is 0s, which is displayed immediately. |
when | Date | 否 | 消息上显示的提示时间 默认为当前时间,如果延迟显示则使用延时后显示消息的时间。 Android - ALL (支持) iOS - 5.0+ (不支持): 不支持设定消息的显示时间,由系统自动管理消息的创建时间。 |
channelId | string | 否 | 渠道id, 支持的版本:HBuilder X 4.02+ |
category | string | 否 | 通知类别,支持的版本:HBuilder X 4.02+ |
success | Function | No | Callback function for successful interface call |
fail | Function | 否 | 接口调用失败的回调函数 |
complete | Function | 否 | 接口调用结束的回调函数(调用成功、失败都会执行) |
Other Related Resources
The similar concept of the MiniApp platform is called Template Message
, and some platforms have renamed it Subscription Message
.
Taking WeChat as an example, the developer's server sends a message to the WeChat server, and the WeChat server sends a subscription message, which is folded into the service notification in the WeChat message list. It belongs to the background development and has nothing to do with the mobile terminal.
If you use uniCloud to send WeChat and Alipay subscription messages, refer to: https://ext.dcloud.net.cn/plugin?id=1810
WeChat subscription message document: [https://developers.weixin.qq.com/miniprogram/dev/framework/open-ability/subscribe-message.html](https://developers.weixin.qq.com/miniprogram/dev /framework/open-ability/subscribe-message.html)
Alipay template message document: https://docs.alipay.com/mini/introduce/message
Baidu template message document: https://smartprogram.baidu.com/docs/develop/third/api/
QQ MiniApp subscription message document: [https://q.qq.com/wiki/develop/miniprogram/frame/open_ability/open_message.html#%E8%AE%A2%E9%98%85%E6%B6%88%E6%81%AF](https://q.qq.com/wiki/develop/miniprogram/frame/open_ability/open_message.html#%E8%AE%A2%E9%98%85%E6%B6%88 %E6%81%AF)
Huawei QuickApp push document: https://developer.huawei.com/consumer/cn/doc/development/quickApp-References/webview-api-hwpush