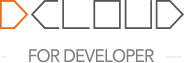
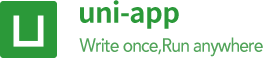
English
Array 对象是用于构造数组的全局对象,数组是类似于列表的高阶对象。
length 是 Array 的实例属性,表示该数组中元素的个数。该值是一个无符号 32 位整数,并且其数值总是大于数组最大索引。
const clothing = ['shoes', 'shirts', 'socks', 'sweaters'];
console.log(clothing.length);
// expected output: 4
Compatibility
Android version | Android uni-app | Android uni-app-x | iOS version | iOS uni-app | iOS uni-app-x |
---|---|---|---|---|---|
4.4 | √ | 3.9.0 | 9.0 | √ | x |
边界情况说明:
Invalid array length
。java.lang.OutOfMemoryError: Failed to allocate a allocation until OOM
。Returns the value of the first element in the array where predicate is true, and undefined otherwise.
Parameters
name | type | required | description |
---|---|---|---|
predicate | (value : T, index : number, obj : T[]) => value is S | YES | find calls predicate once for each element of the array, in ascending order, until it finds one where predicate returns true. If such an element is found, find immediately returns that element value. Otherwise, find returns undefined. |
thisArg | any | NO | If provided, it will be used as the this value for each invocation of predicate. If it is not provided, undefined is used instead. |
Return value
Type |
---|
S | null |
const array1 = [5, 12, 8, 130, 44];
const found = array1.find((element:number):boolean => element > 10);
console.log(found);
// expected output: 12
Compatibility
Android version | Android uni-app | Android uni-app-x | iOS version | iOS uni-app | iOS uni-app-x |
---|---|---|---|---|---|
4.4 | √ | 3.9.0 | 9.0 | √ | x |
Returns the index of the first element in the array where predicate is true, and -1 otherwise.
Parameters
name | type | required | description |
---|---|---|---|
predicate | (value : T, index : number, obj : T[]) => unknown | YES | find calls predicate once for each element of the array, in ascending order, until it finds one where predicate returns true. If such an element is found, findIndex immediately returns that element index. Otherwise, findIndex returns -1. |
thisArg | any | NO | If provided, it will be used as the this value for each invocation of predicate. If it is not provided, undefined is used instead. |
Return value
Type |
---|
number |
const array1 = [5, 12, 8, 130, 44];
const isLargeNumber = (element:number):boolean => element > 13;
console.log(array1.findIndex(isLargeNumber));
// expected output: 3
Compatibility
Android version | Android uni-app | Android uni-app-x | iOS version | iOS uni-app | iOS uni-app-x |
---|---|---|---|---|---|
4.4 | √ | 3.9.0 | 9.0 | √ | x |
Changes all array elements from start
to end
index to a static value
and returns the modified array
Parameters
name | type | required | description |
---|---|---|---|
value | T | YES | value to fill array section with |
start | number | NO | index to start filling the array at. If start is negative, it is treated as length+start where length is the length of the array. |
end | number | NO | index to stop filling the array at. If end is negative, it is treated as length+end. |
Return value
Type |
---|
this |
const array1 = [1, 2, 3, 4];
// fill with 0 from position 2 until position 4
console.log(array1.fill(0, 2, 4));
// expected output: [1, 2, 0, 0]
// fill with 5 from position 1
console.log(array1.fill(5, 1));
// expected output: [1, 5, 5, 5]
console.log(array1.fill(6));
// expected output: [6, 6, 6, 6]
Compatibility
Android version | Android uni-app | Android uni-app-x | iOS version | iOS uni-app | iOS uni-app-x |
---|---|---|---|---|---|
4.4 | √ | 3.9.0 | 9.0 | √ | x |
Returns the this object after copying a section of the array identified by start and end to the same array starting at position target
Parameters
name | type | required | description |
---|---|---|---|
target | number | YES | If target is negative, it is treated as length+target where length is the length of the array. |
start | number | NO | If start is negative, it is treated as length+start. If end is negative, it is treated as length+end. If start is omitted, 0 is used. |
end | number | NO | If not specified, length of the this object is used as its default value. |
Return value
Type |
---|
this |
const array1 = ['a', 'b', 'c', 'd', 'e'];
// copy to index 0 the element at index 3
console.log(array1.copyWithin(0, 3, 4));
// expected output: Array ["d", "b", "c", "d", "e"]
// copy to index 1 all elements from index 3 to the end
console.log(array1.copyWithin(1, 3));
// expected output: Array ["d", "d", "e", "d", "e"]
Compatibility
Android version | Android uni-app | Android uni-app-x | iOS version | iOS uni-app | iOS uni-app-x |
---|---|---|---|---|---|
4.4 | √ | 3.9.0 | 9.0 | √ | x |
pop() 方法从数组中删除最后一个元素,并返回该元素的值。此方法会更改数组的长度。
Return value
Type |
---|
T | null |
const plants = ['broccoli', 'cauliflower', 'cabbage', 'kale', 'tomato'];
console.log(plants.pop());
// expected output: "tomato"
console.log(plants);
// expected output: Array ["broccoli", "cauliflower", "cabbage", "kale"]
plants.pop();
console.log(plants);
// expected output: Array ["broccoli", "cauliflower", "cabbage"]
Compatibility
Android version | Android uni-app | Android uni-app-x | iOS version | iOS uni-app | iOS uni-app-x |
---|---|---|---|---|---|
4.4 | √ | 3.9.0 | 9.0 | √ | x |
push() 方法将指定的元素添加到数组的末尾,并返回新的数组长度。
Parameters
name | type | required | description |
---|---|---|---|
items | T[] | YES | 添加到数组末尾的元素。 |
Return value
Type | description |
---|---|
number | 调用方法的对象的新 length 属性。 |
const animals = ['pigs', 'goats', 'sheep'];
const count = animals.push('cows');
console.log(count);
// expected output: 4
console.log(animals);
// expected output: Array ["pigs", "goats", "sheep", "cows"]
animals.push('chickens', 'cats', 'dogs');
console.log(animals);
// expected output: Array ["pigs", "goats", "sheep", "cows", "chickens", "cats", "dogs"]
Compatibility
Android version | Android uni-app | Android uni-app-x | iOS version | iOS uni-app | iOS uni-app-x |
---|---|---|---|---|---|
4.4 | √ | 3.9.0 | 9.0 | √ | x |
concat() 方法用于合并两个或多个数组。此方法不会更改现有数组,而是返回一个新数组。
Parameters
name | type | required | description |
---|---|---|---|
items | ConcatArray<T>[] | YES | 数组和/或值,将被合并到一个新的数组中。如果省略了所有 valueN 参数,则 concat 会返回调用此方法的现存数组的一个浅拷贝。详情请参阅下文描述。 |
Return value
Type | description |
---|---|
T[] | 新的 Array 实例。 |
const array1 = ['a', 'b', 'c'];
const array2 = ['d', 'e', 'f'];
const array3 = array1.concat(array2);
console.log(array3);
// expected output: Array ["a", "b", "c", "d", "e", "f"]
Compatibility
Android version | Android uni-app | Android uni-app-x | iOS version | iOS uni-app | iOS uni-app-x |
---|---|---|---|---|---|
4.4 | √ | 3.9.0 | 9.0 | √ | x |
concat() 方法用于合并两个或多个数组。此方法不会更改现有数组,而是返回一个新数组。
Parameters
name | type | required | description |
---|---|---|---|
items | (T | ConcatArray<T>)[] | YES | 数组和/或值,将被合并到一个新的数组中。如果省略了所有 valueN 参数,则 concat 会返回调用此方法的现存数组的一个浅拷贝。详情请参阅下文描述。 |
Return value
Type | description |
---|---|
T[] | 新的 Array 实例。 |
Compatibility
Android version | Android uni-app | Android uni-app-x | iOS version | iOS uni-app | iOS uni-app-x |
---|---|---|---|---|---|
4.4 | √ | 3.9.0 | 9.0 | √ | x |
join() 方法将一个数组(或一个类数组对象)的所有元素连接成一个字符串并返回这个字符串,用逗号或指定的分隔符字符串分隔。如果数组只有一个元素,那么将返回该元素而不使用分隔符。
Parameters
name | type | required | description |
---|---|---|---|
separator | string | NO | 指定一个字符串来分隔数组的每个元素。如果需要,将分隔符转换为字符串。如果省略,数组元素用逗号(,)分隔。如果 separator 是空字符串(""),则所有元素之间都没有任何字符。 |
Return value
Type | description |
---|---|
string | 一个所有数组元素连接的字符串。如果 arr.length 为 0,则返回空字符串。 |
const elements = ['Fire', 'Air', 'Water'];
console.log(elements.join());
// expected output: "Fire,Air,Water"
console.log(elements.join(''));
// expected output: "FireAirWater"
console.log(elements.join('-'));
// expected output: "Fire-Air-Water"
Compatibility
Android version | Android uni-app | Android uni-app-x | iOS version | iOS uni-app | iOS uni-app-x |
---|---|---|---|---|---|
4.4 | √ | 3.9.0 | 9.0 | √ | x |
reverse() 方法就地反转数组中的元素,并返回同一数组的引用。数组的第一个元素会变成最后一个,数组的最后一个元素变成第一个。换句话说,数组中的元素顺序将被翻转,变为与之前相反的方向。
Return value
Type | description |
---|---|
T[] | 原始数组反转后的引用。注意,数组是就地反转的,并且没有复制。 |
Compatibility
Android version | Android uni-app | Android uni-app-x | iOS version | iOS uni-app | iOS uni-app-x |
---|---|---|---|---|---|
4.4 | √ | 3.9.0 | 9.0 | √ | x |
shift() 方法从数组中删除第一个元素,并返回该元素的值。此方法更改数组的长度。
Return value
Type | description |
---|---|
T | null | 从数组中删除的元素;如果数组为空则返回 undefined。 |
const array1 = [1, 2, 3];
const firstElement = array1.shift();
console.log(array1);
// expected output: Array [2, 3]
console.log(firstElement);
// expected output: 1
Compatibility
Android version | Android uni-app | Android uni-app-x | iOS version | iOS uni-app | iOS uni-app-x |
---|---|---|---|---|---|
4.4 | √ | 3.9.0 | 9.0 | √ | x |
slice() 方法返回一个新的数组对象,这一对象是一个由 start 和 end 决定的原数组的浅拷贝(包括 start,不包括 end),其中 start 和 end 代表了数组元素的索引。原始数组不会被改变。
Parameters
name | type | required | description |
---|---|---|---|
start | number | NO | 提取起始处的索引(从 0 开始),会转换为整数。 |
end | number | NO | 提取终止处的索引(从 0 开始),会转换为整数。slice() 会提取到但不包括 end 的位置。 |
Return value
Type |
---|
T[] |
const animals = ['ant', 'bison', 'camel', 'duck', 'elephant'];
console.log(animals.slice(2));
// expected output: Array ["camel", "duck", "elephant"]
console.log(animals.slice(2, 4));
// expected output: Array ["camel", "duck"]
console.log(animals.slice(1, 5));
// expected output: Array ["bison", "camel", "duck", "elephant"]
console.log(animals.slice(-2));
// expected output: Array ["duck", "elephant"]
console.log(animals.slice(2, -1));
// expected output: Array ["camel", "duck"]
console.log(animals.slice());
// expected output: Array ["ant", "bison", "camel", "duck", "elephant"]
Compatibility
Android version | Android uni-app | Android uni-app-x | iOS version | iOS uni-app | iOS uni-app-x |
---|---|---|---|---|---|
4.4 | √ | 3.9.0 | 9.0 | √ | x |
sort() 方法就地对数组的元素进行排序,并返回对相同数组的引用。默认排序是将元素转换为字符串,然后按照它们的 UTF-16 码元值升序排序。
Parameters
name | type | required | description |
---|---|---|---|
compareFn | (a : T, b : T) => number | NO | 定义排序顺序的函数。返回值应该是一个数字,其正负性表示两个元素的相对顺序。该函数使用以下参数调用: a:第一个用于比较的元素。不会是 undefined。 b:第二个用于比较的元素。不会是 undefined。 如果省略该函数,数组元素会被转换为字符串,然后根据每个字符的 Unicode 码位值进行排序。 |
Return value
Type | description |
---|---|
this | 经过排序的原始数组的引用。注意数组是就地排序的,不会进行复制。 |
const array2 = [5, 1, 4, 2, 3];
array2.sort((a: number, b: number):number => a - b);
// expect(array2).toEqual([1, 2, 3, 4, 5]);
Compatibility
Android version | Android uni-app | Android uni-app-x | iOS version | iOS uni-app | iOS uni-app-x |
---|---|---|---|---|---|
4.4 | √ | 3.9.0 | 9.0 | √ | x |
splice() 方法通过移除或者替换已存在的元素和/或添加新元素就地改变一个数组的内容。
Parameters
name | type | required | description |
---|---|---|---|
start | number | YES | 从 0 开始计算的索引,表示要开始改变数组的位置,它会被转换成整数。 |
items | T[] | YES | - |
Return value
Type | description |
---|---|
T[] | 一个包含了删除的元素的数组。 |
const months = ['Jan', 'March', 'April', 'June'];
months.splice(1, 0, 'Feb');
// inserts at index 1
console.log(months);
// expected output: Array ["Jan", "Feb", "March", "April", "June"]
months.splice(4, 1, 'May');
// replaces 1 element at index 4
console.log(months);
// expected output: Array ["Jan", "Feb", "March", "April", "May"]
Compatibility
Android version | Android uni-app | Android uni-app-x | iOS version | iOS uni-app | iOS uni-app-x |
---|---|---|---|---|---|
4.4 | √ | 3.9.0 | 9.0 | √ | x |
unshift() 方法将指定元素添加到数组的开头,并返回数组的新长度。
Parameters
name | type | required | description |
---|---|---|---|
items | T[] | YES | 添加到 arr 开头的元素。 |
Return value
Type |
---|
number |
const array1 = [1, 2, 3];
console.log(array1.unshift(4, 5));
// expected output: 5
console.log(array1);
// expected output: Array [4, 5, 1, 2, 3]
Compatibility
Android version | Android uni-app | Android uni-app-x | iOS version | iOS uni-app | iOS uni-app-x |
---|---|---|---|---|---|
4.4 | √ | 3.9.0 | 9.0 | √ | x |
indexOf() 方法返回数组中第一次出现给定元素的下标,如果不存在则返回 -1。
Parameters
name | type | required | description |
---|---|---|---|
searchElement | T | YES | 数组中要查找的元素。 |
fromIndex | number | NO | 开始搜索的索引(从零开始),会转换为整数。 |
Return value
Type |
---|
number |
const beasts = ['ant', 'bison', 'camel', 'duck', 'bison'];
console.log(beasts.indexOf('bison'));
// expected output: 1
// start from index 2
console.log(beasts.indexOf('bison', 2));
// expected output: 4
console.log(beasts.indexOf('giraffe'));
// expected output: -1
Compatibility
Android version | Android uni-app | Android uni-app-x | iOS version | iOS uni-app | iOS uni-app-x |
---|---|---|---|---|---|
4.4 | √ | 3.9.0 | 9.0 | √ | x |
lastIndexOf() 方法返回数组中给定元素最后一次出现的索引,如果不存在则返回 -1。该方法从 fromIndex 开始向前搜索数组。
Parameters
name | type | required | description |
---|---|---|---|
searchElement | T | YES | 被查找的元素。 |
fromIndex | number | NO | 以 0 起始的索引,表明反向搜索的起始位置,会被转换为整数。 |
Return value
Type |
---|
number |
const animals = ['Dodo', 'Tiger', 'Penguin', 'Dodo'];
console.log(animals.lastIndexOf('Dodo'));
// expected output: 3
console.log(animals.lastIndexOf('Tiger'));
// expected output: 1
Compatibility
Android version | Android uni-app | Android uni-app-x | iOS version | iOS uni-app | iOS uni-app-x |
---|---|---|---|---|---|
4.4 | √ | 3.9.0 | 9.0 | √ | x |
every() 方法测试一个数组内的所有元素是否都能通过指定函数的测试。它返回一个布尔值。
Parameters
name | type | required | description |
---|---|---|---|
predicate | (value : T, index : number, array : T[]) => unknown | YES | 为数组中的每个元素执行的函数。它应该返回一个真值以指示元素通过测试,否则返回一个假值。该函数被调用时将传入以下参数: value:数组中当前正在处理的元素。 index:正在处理的元素在数组中的索引。 array:调用了 every() 的数组本身。 |
thisArg | any | NO | 执行 callbackFn 时用作 this 的值 |
Return value
Type |
---|
boolean |
const isBelowThreshold = (currentValue:number):boolean => currentValue < 40;
const array1 = [1, 30, 39, 29, 10, 13];
console.log(array1.every(isBelowThreshold));
// expected output: true
Compatibility
Android version | Android uni-app | Android uni-app-x | iOS version | iOS uni-app | iOS uni-app-x |
---|---|---|---|---|---|
4.4 | √ | 3.9.0 | 9.0 | √ | x |
some() 方法测试数组中是否至少有一个元素通过了由提供的函数实现的测试。如果在数组中找到一个元素使得提供的函数返回 true,则返回 true;否则返回 false。它不会修改数组。
Parameters
name | type | required | description |
---|---|---|---|
predicate | (value : T, index : number, array : T[]) => unknown | YES | 为数组中的每个元素执行的函数。它应该返回一个真值以指示元素通过测试,否则返回一个假值。该函数被调用时将传入以下参数: value:数组中当前正在处理的元素。 index:正在处理的元素在数组中的索引。 array:调用了 some() 的数组本身。 |
thisArg | any | NO | 执行 callbackFn 时用作 this 的值。 |
Return value
Type | description |
---|---|
boolean | 如果回调函数对数组中至少一个元素返回一个真值,则返回 true。否则返回 false。 |
const array = [1, 2, 3, 4, 5];
// checks whether an element is even
const even = (element:number):boolean=> element % 2 == 0;
console.log(array.some(even));
// expected output: true
Compatibility
Android version | Android uni-app | Android uni-app-x | iOS version | iOS uni-app | iOS uni-app-x |
---|---|---|---|---|---|
4.4 | √ | 3.9.0 | 9.0 | √ | x |
forEach() 方法对数组的每个元素执行一次给定的函数。
Parameters
name | type | required | description |
---|---|---|---|
callbackfn | (value : T, index : number, array : T[]) => void | YES | 为数组中每个元素执行的函数。并会丢弃它的返回值。该函数被调用时将传入以下参数: value:数组中正在处理的当前元素。 index:数组中正在处理的当前元素的索引。 array:调用了 forEach() 的数组本身。 |
thisArg | any | NO | 执行 callbackFn 时用作 this 的值。 |
Return value
Type |
---|
void |
const array1 = ['a', 'b', 'c'];
array1.forEach(element => console.log(element));
// expected output: "a"
// expected output: "b"
// expected output: "c"
特别注意: 不可在 forEach 的 callbackFn 里添加或者删除原数组元素,此行为是危险的,在 Android 平台会造成闪退,在 iOS 平台会造成行为不符合预期。如果想实现该效果,请用 while 循环。
const array1 = ['a', 'b', 'c'];
array1.forEach(element => {
console.log(element)
array1.pop() // 此行为在 Android 平台会造成闪退,在 iOS 平台会输出 'a', 'b', 'c', 而 JS 会输出 'a', 'b'
});
// 如果想让上述行为正常运行,可以用 while 循环实现:
let array1 = ['a', 'b', 'c'];
let index = 0;
while (index < array1.length) {
console.log(array1[index]);
array1.pop();
index += 1;
}
Compatibility
Android version | Android uni-app | Android uni-app-x | iOS version | iOS uni-app | iOS uni-app-x |
---|---|---|---|---|---|
4.4 | √ | 3.9.0 | 9.0 | √ | x |
map() 方法创建一个新数组,这个新数组由原数组中的每个元素都调用一次提供的函数后的返回值组成。
Parameters
name | type | required | description |
---|---|---|---|
thisArg | any | NO | An object to which the this keyword can refer in the callbackfn function. If thisArg is omitted, undefined is used as the this value. |
Return value
Type |
---|
U[] |
const array1 = [1, 4, 9, 16];
// pass a function to map
const map1 = array1.map((x:number):number => x * 2);
console.log(map1);
// expected output: Array [2, 8, 18, 32]
Compatibility
Android version | Android uni-app | Android uni-app-x | iOS version | iOS uni-app | iOS uni-app-x |
---|---|---|---|---|---|
4.4 | √ | 3.9.0 | 9.0 | √ | x |
filter() 方法创建给定数组一部分的浅拷贝,其包含通过所提供函数实现的测试的所有元素。
Parameters
name | type | required | description |
---|---|---|---|
predicate | (value : T, index : number, array : T[]) => unknown | YES | 为数组中的每个元素执行的函数。它应该返回一个真值以将元素保留在结果数组中,否则返回一个假值。该函数被调用时将传入以下参数: value:数组中当前正在处理的元素。 index:正在处理的元素在数组中的索引。 array:调用了 filter() 的数组本身。 |
thisArg | any | NO | 执行 callbackFn 时用作 this 的值 |
Return value
Type |
---|
T[] |
const words = ['spray', 'limit', 'elite', 'exuberant', 'destruction', 'present'];
const result = words.filter((word:string):boolean => word.length > 6);
console.log(result);
// expected output: Array ["exuberant", "destruction", "present"]
Compatibility
Android version | Android uni-app | Android uni-app-x | iOS version | iOS uni-app | iOS uni-app-x |
---|---|---|---|---|---|
4.4 | √ | 3.9.0 | 9.0 | √ | x |
reduce() 方法对数组中的每个元素按序执行一个提供的 reducer 函数,每一次运行 reducer 会将先前元素的计算结果作为参数传入,最后将其结果汇总为单个返回值。
Parameters
name | type | required | description |
---|---|---|---|
callbackfn | (previousValue : T, currentValue : T, currentIndex : number, array : T[]) => T | YES | 为数组中每个元素执行的函数。其返回值将作为下一次调用 callbackFn 时的 accumulator 参数。对于最后一次调用,返回值将作为 reduce() 的返回值。该函数被调用时将传入以下参数: previousValue:上一次调用 callbackFn 的结果。在第一次调用时,如果指定了 initialValue 则为指定的值,否则为 array[0] 的值。 currentValue:当前元素的值。在第一次调用时,如果指定了 initialValue,则为 array[0] 的值,否则为 array[1]。 currentIndex:currentValue 在数组中的索引位置。在第一次调用时,如果指定了 initialValue 则为 0,否则为 1 array:调用了 reduce() 的数组本身。 |
Return value
Type |
---|
T |
const array1 = [1, 2, 3, 4];
// 0 + 1 + 2 + 3 + 4
const initialValue = 0;
const sumWithInitial = array1.reduce(
(previousValue:number, currentValue:number):number => previousValue + currentValue,
initialValue
);
console.log(sumWithInitial);
// expected output: 10
Compatibility
Android version | Android uni-app | Android uni-app-x | iOS version | iOS uni-app | iOS uni-app-x |
---|---|---|---|---|---|
4.4 | √ | 3.9.0 | 9.0 | √ | x |
reduceRight() 方法对累加器(accumulator)和数组的每个值(按从右到左的顺序)应用一个函数,并使其成为单个值。
Parameters
name | type | required | description |
---|---|---|---|
callbackfn | (previousValue : T, currentValue : T, currentIndex : number, array : T[]) => T | YES | 为数组中的每个元素执行的函数。其返回值将作为下一次调用 callbackFn 时的 accumulator 参数。对于最后一次调用,返回值将成为 reduceRight() 的返回值。该函数被调用时将传入以下参数: previousValue:上一次调用 callbackFn 的结果。在第一次调用时,如果指定了 initialValue 则为指定的值,否则为数组最后一个元素的值。 currentValue:数组中当前正在处理的元素。 index:正在处理的元素在数组中的索引。 array:调用了 reduceRight() 的数组本身。 |
Return value
Type |
---|
T |
Compatibility
Android version | Android uni-app | Android uni-app-x | iOS version | iOS uni-app | iOS uni-app-x |
---|---|---|---|---|---|
4.4 | √ | 3.9.0 | 9.0 | √ | x |
Array.isArray() 静态方法用于确定传递的值是否是一个 Array。
Parameters
name | type | required | description |
---|---|---|---|
arg | any | YES | 需要检测的值。 |
Return value
Type | description |
---|---|
arg is any[] | 如果 value 是 Array,则为 true;否则为 false。如果 value 是 TypedArray 实例,则总是返回 false。 |
console.log(Array.isArray([1, 3, 5]));
// Expected output: true
console.log(Array.isArray('[]'));
// Expected output: false
console.log(Array.isArray(new Array(5)));
// Expected output: true
console.log(Array.isArray(new Int16Array([15, 33])));
// Expected output: false
Compatibility
Android version | Android uni-app | Android uni-app-x | iOS version | iOS uni-app | iOS uni-app-x |
---|---|---|---|---|---|
4.4 | √ | 3.9.0 | 9.0 | √ | x |
const fruits = ['Apple', 'Banana']
console.log(fruits.length)
const first = fruits[0]
// Apple
const last = fruits[fruits.length - 1]
// Banana
fruits.forEach(function(item, index, array) {
console.log(item, index)
})
// Apple 0
// Banana 1
注意:数组遍历不推荐使用 for in 语句,因为在 ts 中 for in 遍历的是数组的下标,而在 Swift 和 Kottlin 中遍历的是数组的元素,存在行为不一致。
添加元素到数组的末尾
const newLength = fruits.push('Orange')
// ["Apple", "Banana", "Orange"]
const last = fruits.pop() // remove Orange (from the end)
// ["Apple", "Banana"]
const first = fruits.shift() // remove Apple from the front
// ["Banana"]
const newLength = fruits.unshift('Strawberry') // add to the front
// ["Strawberry", "Banana"]
fruits.push('Mango')
// ["Strawberry", "Banana", "Mango"]
const pos = fruits.indexOf('Banana')
// 1
const removedItem = fruits.splice(pos, 1) // this is how to remove an item
// ["Strawberry", "Mango"]
const vegetables = ['Cabbage', 'Turnip', 'Radish', 'Carrot']
console.log(vegetables)
// ["Cabbage", "Turnip", "Radish", "Carrot"]
const pos = 1
const n = 2
const removedItems = vegetables.splice(pos, n)
// this is how to remove items, n defines the number of items to be removed,
// starting at the index position specified by pos and progressing toward the end of array.
console.log(vegetables)
// ["Cabbage", "Carrot"] (the original array is changed)
console.log(removedItems)
// ["Turnip", "Radish"]
const shallowCopy = fruits.slice() // this is how to make a copy
// ["Strawberry", "Mango"]
数组的索引是从 0 开始的,第一个元素的索引为 0,最后一个元素的索引等于该数组的 长度 减 1。
如果指定的索引是一个无效值,将会抛出 IndexOutOfBoundsException 异常
下面的写法是错误的,运行时会抛出 SyntaxError 异常,而原因则是使用了非法的属性名:
console.log(arr.0) // a syntax error