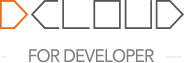
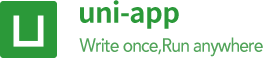
English
预览图片
name | type | required | default | description |
---|---|---|---|---|
options | PreviewImageOptions | YES | - | - |
name | type | optinal | default | description |
---|---|---|---|---|
current | any | NO | - | current 为当前显示图片的链接/索引值,不填或填写的值无效则为 urls 的第一张。 |
urls | Array<string> | YES | - | 需要预览的图片链接列表 |
indicator | string | NO | - | 图片指示器样式 - default: 底部圆点指示器 - number: 顶部数字指示器 - none: 不显示指示器 |
loop | boolean | NO | - | 是否可循环预览 |
success | (callback: PreviewImageSuccess) => void | NO | - | 接口调用成功的回调函数 |
fail | (callback: IMediaError) => void | NO | - | 接口调用失败的回调函数 |
complete | (callback: any) => void | NO | - | 接口调用结束的回调函数(调用成功、失败都会执行) |
name | type | optinal | default | description |
---|---|---|---|---|
errSubject | string | YES | - | 调用API的名称 |
errMsg | string | YES | - | 描述信息 |
name | type | optinal | default | description |
---|---|---|---|---|
errCode | 1101001 | 1101002 | 1101003 | 1101004 | 1101005 | 1101006 | 1101007 | 1101008 | 1101009 | 1101010 | YES | - | 错误码 - 1101001 用户取消 - 1101002 urls至少包含一张图片地址 - 1101003 文件不存在 - 1101004 图片加载失败 - 1101005 未获取权限 - 1101006 图片或视频保存失败 - 1101007 图片裁剪失败 - 1101008 拍照或录像失败 - 1101009 图片压缩失败 - 1101010 其他错误 |
errSubject | string | YES | - | 统一错误主题(模块)名称 |
data | any | NO | - | 错误信息中包含的数据 |
errMsg | string | YES | - | - |
Android version | Android uni-app | Android uni-app-x | iOS version | iOS uni-app | iOS uni-app-x |
---|---|---|---|---|---|
4.4 | √ | 3.9+ | - | - | - |
关闭图片预览
name | type | required | default | description |
---|---|---|---|---|
options | ClosePreviewImageOptions | YES | - | - |
name | type | optinal | default | description |
---|---|---|---|---|
success | (callback: ClosePreviewImageSuccess) => void | NO | - | 接口调用成功的回调函数 |
fail | (callback: IMediaError) => void | NO | - | 接口调用失败的回调函数 |
complete | (callback: any) => void | NO | - | 接口调用结束的回调函数(调用成功、失败都会执行) |
name | type | optinal | default | description |
---|---|---|---|---|
errMsg | string | YES | - | 错误信息 |
name | type | optinal | default | description |
---|---|---|---|---|
errCode | 1101001 | 1101002 | 1101003 | 1101004 | 1101005 | 1101006 | 1101007 | 1101008 | 1101009 | 1101010 | YES | - | 错误码 - 1101001 用户取消 - 1101002 urls至少包含一张图片地址 - 1101003 文件不存在 - 1101004 图片加载失败 - 1101005 未获取权限 - 1101006 图片或视频保存失败 - 1101007 图片裁剪失败 - 1101008 拍照或录像失败 - 1101009 图片压缩失败 - 1101010 其他错误 |
errSubject | string | YES | - | 统一错误主题(模块)名称 |
data | any | NO | - | 错误信息中包含的数据 |
errMsg | string | YES | - | - |
Android version | Android uni-app | Android uni-app-x | iOS version | iOS uni-app | iOS uni-app-x |
---|---|---|---|---|---|
4.4 | √ | 3.9+ | - | - | - |
<template>
<!-- #ifdef APP -->
<scroll-view style="flex: 1">
<!-- #endif -->
<view style="padding-left: 16rpx; padding-right: 16rpx">
<view>
<text class="text-desc">图片指示器样式</text>
<radio-group
class="cell-ct"
style="background-color: white"
@change="onIndicatorChanged"
>
<view
class="indicator-it"
v-for="(item, index) in indicator"
:key="item.value"
>
<radio :checked="index == 0" :value="item.value">{{
item.name
}}</radio>
</view>
</radio-group>
</view>
<view>
<checkbox-group
@change="onCheckboxChange"
style="margin-top: 32rpx; margin-bottom: 32rpx; margin-left: 16rpx"
>
<checkbox :checked="isLoop" style="margin-right: 30rpx"
>循环播放</checkbox
>
</checkbox-group>
</view>
<view style="background-color: white">
<text class="text-desc">点击图片开始预览</text>
<view class="cell-ct" style="background-color: #eeeeee; padding: 16rpx">
<view
class="cell cell-choose-image"
v-for="(image, index) in imageList"
:key="index"
>
<image
style="width: 210rpx; height: 210rpx"
mode="aspectFit"
:src="image"
@click="previewImage(index)"
></image>
</view>
<image
class="cell cell-choose-image"
src="/static/plus.png"
@click="chooseImage"
>
<view></view>
</image>
</view>
</view>
</view>
<!-- #ifdef APP -->
</scroll-view>
<!-- #endif -->
</template>
<script>
type ItemType = {
value: string,
name: string
}
export default {
data() {
return {
imageList: ["https://web-assets.dcloud.net.cn/unidoc/zh/uni@2x.png", "/static/uni.png"],
indicator: [{
value: "default",
name: "圆点"
}, {
value: "number",
name: "数字"
}, {
value: "none",
name: "不显示"
}] as ItemType[],
currentIndicator: "default",
isLoop: true
}
},
methods: {
previewImage(index: number) {
uni.previewImage({
urls: this.imageList,
current: index,
indicator: this.currentIndicator,
loop: this.isLoop
})
},
chooseImage() {
uni.chooseImage({
sourceType: ['album'],
success: (e) => {
this.imageList = this.imageList.concat(e.tempFilePaths)
},
fail(_) {
}
})
},
onIndicatorChanged(e: RadioGroupChangeEvent) {
this.currentIndicator = e.detail.value
},
onCheckboxChange(_: CheckboxGroupChangeEvent) {
this.isLoop = !this.isLoop
}
}
}
</script>
<style>
.text-desc {
margin-top: 32rpx;
margin-left: 16rpx;
margin-bottom: 32rpx;
font-weight: bold;
}
.cell-ct {
display: flex;
flex-wrap: wrap;
flex-direction: row;
}
.cell {
margin-left: 14rpx;
width: 210rpx;
height: 210rpx;
}
.cell-choose-image {
border-width: 1px;
border-style: solid;
border-color: lightgray;
}
.indicator-it {
margin: 16rpx;
}
</style>
name | type | optinal | default | description |
---|---|---|---|---|
errMsg | string | YES | - | 错误信息 |