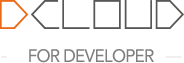
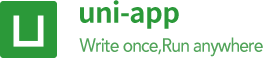
uni-app
supports the following application life cycle functions:
Function name | Instruction |
---|---|
onLaunch | Triggerred when the initialization of uni-app is completed (only triggered once globally) |
onShow | Displayed when uni-app starts or enters the foreground from the background |
onHide | When uni-app enters the background from the foreground |
onError | Triggered when uni-app reports an error |
onUniNViewMessage | listen to the data sent from nvue page, refer to Nvue to vue communication (opens new window) |
onUnhandledRejection | Listening function for unprocessed Promise reject events (2.8.1+) |
onThemeChange | listen to system theme changes |
Notice
App.vue
, and listening on other pages is invalid.Sample code
<script>
// You can only listen to the application's life cycle in App.vue
export default {
onLaunch: function() {
console.log('App Launch')
},
onShow: function() {
console.log('App Show')
},
onHide: function() {
console.log('App Hide')
}
}
</script>
uni-app
supports the following page life cycle functions:
Function name | Instruction | Platform difference description | Minimum version |
---|---|---|---|
onLoad | listen to the page loading, whose parameter is the data transferred from the previous page, and the parameter type is Object (used for page parameter transmission)), refer toExample | ||
onShow | listen to page display. Triggered every time a page appears on the screen, including clicking back from the lower level page to return to the current page | ||
onReady | The first rendering of the listening page is completed. Note that if the rendering speed is fast, it will be triggered before the page enters the animation. | ||
onHide | listen to page hiding | ||
onUnload | listen to page uninstall | ||
onResize | listen to window size changes | App | |
onPullDownRefresh | listen to the user's pull-down action, which is generally used for pull-down refresh, refer toExample | ||
onReachBottom | The event that a page scrolls to the bottom (not scroll-view to the bottom) is often used to pull down the data on the next page. See the precautions below for details | ||
onTabItemTap | Triggered when tab is clicked with the parameter of Object. See the notes below for details | H5, App | |
onPageScroll | Listen to page scrolling with the parameter of Object | not supported by nvue temporarily | |
onNavigationBarButtonTap | Listen to the click event of native title bar button with the parameter of Object | App, H5 | |
onBackPress | listen to page return, return event = {from:backbutton, navigateBack}. backbutton indicates that the source is the return button in the upper left corner or the android return button. navigateBack indicates that the source is uni.navigateBack. For detailed description and usage, see: onBackPress details (opens new window). | app, H5 | |
onNavigationBarSearchInputChanged | Listen to the input content change event of search input box of the native title bar | App, H5 | 1.6.0 |
onNavigationBarSearchInputConfirmed | Listen to the search event of search input box of the native title bar, which is triggered when the user clicks the "Search" button on the soft keyboard. | App, H5 | 1.6.0 |
onNavigationBarSearchInputClicked | Listen to the native title bar search input box click event | App, H5 | 1.6.0 |
onReachBottom
Notes for Use:
The trigger distance at the bottom of a specific page can be defined in pages.json onReachBottomDistance. For example, if the distance is set to 50, the onReachBottom event will be triggered when the page is scrolled to 50px from the bottom.
If using scroll-view causes no scrolling in the page, the scrolling to the bottom event will not be triggered. Please refer to the documentation of scroll-view for the scrolling to the bottom event of scroll-view
onPageScroll
(listen to scroll, scroll listening, scroll event) parameter description:
Attribute | Type | Instruction |
---|---|---|
scrollTop | Number | The distance (in px) that the page has scrolled over vertically |
Notice
onPageScroll
, such as frequently modifying pages. Since this life cycle is triggered in the rendering layer and on the non-h5 side, js is executed in the logical layer, and the communication between the two layers is lossy. If the data exchange between the two layers is frequently triggered during the rolling process, it may cause a lag.async
is not available on onBackPress
, which will prevent the default returnonPageScroll : function(e) {
//nvue does not support scrolling listener at the moment, but bindingx can be used instead
console.log("scrolling distance: " + e.scrollTop);
},
Description of the json object returned by onTabItemTap
:
Attribute | Type | Instruction |
---|---|---|
index | String | The serial number of the clicked tabItem, starting from 0 |
pagePath | String | The page path of the clicked tabItem |
text | String | The buttom text of the clicked tabItem |
Notice
onTabItemTap : function(e) {
console.log(e);
// Return format of e is json object: {"index":0,"text":"Home page","pagePath":"pages/index/index"}
},
Description of onNavigationBarButtonTap
parameter:
Attribute | Type | Instruction |
---|---|---|
index | Number | Subscript of native title bar button array |
onNavigationBarButtonTap : function (e) {
console.log(e);
// The return format of e is a json object: {"text":"test","index":0}
}
Description of onBackPress
callback parameter object:
Attribute | Type | Instruction |
---|---|---|
from | String | Sources of triggering return behavior: 'backbutton' - navigation bar button in the upper left corner and Android return key; 'navigateback' - uni. navigateback() method. |
export default {
data() {
return {};
},
onBackPress(options) {
console.log('from:' + options.from)
}
}
Notice
The life cycle supported by the uni-app
component is the same as the life cycle of the vue standard component. There is no page-level lifecycle such as onLoad:
Function name | Instruction | Platform difference description | Minimum version |
---|---|---|---|
beforeCreate | Called after the instance is initialized. See details (opens new window) | ||
created | Called immediately after the instance is created. See details (opens new window) | ||
beforeMount | Called before mounting. See details (opens new window) | ||
mounted | Called after being mounted to the instance. See details (opens new window) Note: It is not certain that all the sub-components are mounted here. If you need to perform operations after sub-components are fully mounted, you can use $nextTick Vue official documents (opens new window) | ||
beforeUpdate | Called when the data is updated, which occurs before the virtual DOM is patched. See details (opens new window) | Only supported by H5 platform | |
updated | The virtual DOM is re-rendered and patched due to data changes, after which the hook will be called. See details (opens new window) | Only supported by H5 platform | |
beforeDestroy | Called before the instance is destroyed. At this step, the instance is still fully available. See details (opens new window) | ||
destroyed | Call after the Vue instance is destroyed. After calling, everything indicated by Vue instance will be unbound. All event listeners will be removed, and all sub-instances will be destroyed. See details (opens new window) |