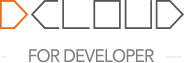
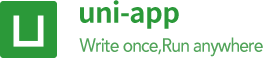
English
The editorContext instance corresponding to the editor component can be accessed through uni.createSelectorQuery.
onEditorReady() {
uni.createSelectorQuery().select('#editor').context((res) => {
this.editorCtx = res.context
}).exec()
}
The Baidu applet Editor
rich text editor dynamic library provides the createEditorContext
method to get it.
onEditorReady() {
this.editorCtx = requireDynamicLib('editorLib').createEditorContext('editor');
}
editorContext
is bound to a <editor>
component through id
, and operates the corresponding <editor>
component.
Platform difference description
App | H5 | 微信小程序 | 支付宝小程序 | 百度小程序 | 抖音小程序、飞书小程序 | QQ小程序 | 快手小程序 | 京东小程序 |
---|---|---|---|---|---|---|---|---|
√ | 2.4.5+ | √ | x | A dynamic library needs to be imported | x | x | x | x |
Baidu applet introduces dynamic library
dynamicLib
to manifest.json
"mp-baidu" : {
"appid" : "",
"setting" : {
"urlCheck" : true
},
"dynamicLib": {//引入百度小程序动态库
"editorLib": {
"provider": "swan-editor"
}
}
}
pages.json
file as follows:{
"pages": [
{
"path": "pages/index/index",
"style": {
"navigationBarTitleText": "uni-app",
"usingComponents": {
"editor": "dynamicLib://editorLib/editor"
}
}
}
]
}
Modify style
Parameter | Type | Instruction |
---|---|---|
name | String | Attribute |
value | String | Value |
List of supported styles
name | value | Platform Difference Description |
---|---|---|
bold | ||
italic | ||
underline | ||
strike | ||
ins | ||
script | sub / super | |
header | H1 / H2 / h3 / H4 / h5 / H6 | |
direction | rtl | |
indent | -1 / +1 | |
list | ordered / bullet / check | |
color | hex color | |
backgroundColor | hex color | |
margin/marginTop/marginBottom/marginLeft/marginRight | css style | Baidu applet does not support |
padding/paddingTop/paddingBottom/paddingLeft/paddingRight | css style | Baidu applet does not support |
font/fontSize/fontStyle/fontVariant/fontWeight/fontFamily | css style | Baidu applet does not support |
lineHeight | css style | Baidu applet does not support |
letterSpacing | css style | Baidu applet does not support |
textDecoration | css style | Baidu applet does not support |
textIndent | css style | Baidu applet does not support |
wordWrap | css style | Baidu applet does not support |
wordBreak | css style | Baidu applet does not support |
whiteSpace | css style | Baidu applet does not support |
If applying to the selected area with style, the current style will be canceled. css style represents the allowable values specified in css.
Insert dividing line
OBJECT parameter description
Attribute | Type | Defaults | Required | Instruction |
---|---|---|---|---|
success | Function | No | Callback function for successful interface calling | |
fail | Function | No | Callback function for failed interface calling | |
complete | Function | No | Callback function for closed interface calling (available both for successful and failed calling) |
Insert image.
When the WeChat applet platform address is a temporary file, the content in the html format of the editor obtained is <img>
The attribute data-local is added to the tag, and the data-local field is added to the image attributes attribute in the delta format content, which is the address of the incoming temporary file.
Developers can choose to upload images to the server during the submission stage, and replace them after obtaining the network address. When replacing, the html content should be replaced with <img> The src value of
, which should be substituted for the insert { image: abc }
value for delta content.
OBJECT parameter description
Attribute | Type | Defaults | Required | Instruction |
---|---|---|---|---|
src | String | Yes | Image address | |
alt | String | No | Alternative text when the image cannot be displayed. | |
width | String | No | Width of image (pixels/percentage). Supported in 2.6.5+. | |
height | String | No | Height of image (pixels/percentage). Supported in 2.6.5+. | |
extClass | String | No | Class name that added to img tags. Supported in 2.6.5+. | |
data | Object | No | data area serialized to name=value;name1=value2 and mounted to the attribute data-custom. Supported in 2.6.5+. | |
success | Function | No | Callback function for successful interface calling | |
fail | Function | No | Callback function for failed interface calling | |
complete | Function | No | Callback function for closed interface calling (available both for successful and failed calling) |
Override the current selected area and set a paragraph.
OBJECT parameter description
Attribute | Type | Defaults | Required | Instruction |
---|---|---|---|---|
text | String | No | Text content | |
success | Function | No | Callback function for successful interface calling | |
fail | Function | No | Callback function for failed interface calling | |
complete | Function | No | Callback function for closed interface calling (available both for successful and failed calling) |
Initialize the editor content, only delta takes effect when html and delta exist at the same time
OBJECT parameter description
Attribute | Type | Defaults | Required | Instruction |
---|---|---|---|---|
html | String | No | HTML content with tags | |
delta | Object | No | Delta object that represents the content | |
success | Function | No | Callback function for successful interface calling | |
fail | Function | No | Callback function for failed interface calling | |
complete | Function | No | Callback function for closed interface calling (available both for successful and failed calling) |
Get editor content
OBJECT parameter description
Attribute | Type | Defaults | Required | Instruction |
---|---|---|---|---|
success | Function | No | Callback function for successful interface calling | |
fail | Function | No | Callback function for failed interface calling | |
complete | Function | No | Callback function for closed interface calling (available both for successful and failed calling) |
object.success callback function
property | type | description |
---|---|---|
html | string | Tagged HTML content |
text | string | Plain text content |
delta | Object | A delta object representing the content |
Clear editor content
OBJECT parameter description
Attribute | Type | Defaults | Required | Instruction |
---|---|---|---|---|
success | Function | No | Callback function for successful interface calling | |
fail | Function | No | Callback function for failed interface calling | |
complete | Function | No | Callback function for closed interface calling (available both for successful and failed calling) |
Clear the style of the current selection
OBJECT parameter description
Attribute | Type | Defaults | Required | Instruction |
---|---|---|---|---|
success | Function | No | Callback function for successful interface calling | |
fail | Function | No | Callback function for failed interface calling | |
complete | Function | No | Callback function for closed interface calling (available both for successful and failed calling) |
Revoke
OBJECT parameter description
Attribute | Type | Defaults | Required | Instruction |
---|---|---|---|---|
success | Function | No | Callback function for successful interface calling | |
fail | Function | No | Callback function for failed interface calling | |
complete | Function | No | Callback function for closed interface calling (available both for successful and failed calling) |
Restore
OBJECT parameter description
Attribute | Type | Defaults | Required | Instruction |
---|---|---|---|---|
success | Function | No | Callback function for successful interface calling | |
fail | Function | No | Callback function for failed interface calling | |
complete | Function | No | Callback function for closed interface calling (available both for successful and failed calling) |
The editor is out of focus and the keyboard is retracted at the same time.
Platform difference description
App | H5 | 微信小程序 | 支付宝小程序 | 百度小程序 | 抖音小程序、飞书小程序 | QQ小程序 | 快手小程序 | 京东小程序 |
---|---|---|---|---|---|---|---|---|
√HBuilderX 3.0.3 | √HBuilderX 3.0.3 | √Basic library 2.8.3 | x | √ | x | x | x | x |
OBJECT parameter description
Attribute | Type | Defaults | Required | Instruction |
---|---|---|---|---|
success | Function | No | Callback function for successful interface calling | |
fail | Function | No | Callback function for failed interface calling | |
complete | Function | No | Callback function for closed interface calling (available both for successful and failed calling) |
Make the editor cursor scroll to the visible area of the window.
Platform difference description
App | H5 | 微信小程序 | 支付宝小程序 | 百度小程序 | 抖音小程序、飞书小程序 | QQ小程序 | 快手小程序 | 京东小程序 |
---|---|---|---|---|---|---|---|---|
√HBuilderX 3.0.3 | √HBuilderX 3.0.3 | √Basic library 2.8.3 | x | √ | x | x | x | x |
OBJECT parameter description
Attribute | Type | Defaults | Required | Instruction |
---|---|---|---|---|
success | Function | No | Callback function for successful interface calling | |
fail | Function | No | Callback function for failed interface calling | |
complete | Function | No | Callback function for closed interface calling (available both for successful and failed calling) |
Get the plain text content within the selected area of the editor. When the editor is out of focus or no interval is selected, noting is returned.
Platform difference description
App | H5 | 微信小程序 | 支付宝小程序 | 百度小程序 | 抖音小程序、飞书小程序 | QQ小程序 | 快手小程序 | 京东小程序 |
---|---|---|---|---|---|---|---|---|
√HBuilderX 3.0.3 | √HBuilderX 3.0.3 | √Basic library 2.10.2 | x | √ | x | x | x | x |
OBJECT parameter description
Attribute | Type | Defaults | Required | Instruction |
---|---|---|---|---|
success | Function | No | Callback function for successful interface calling | |
fail | Function | No | Callback function for failed interface calling | |
complete | Function | No | Callback function for closed interface calling (available both for successful and failed calling) |
Success return parameter description:
Parameter | Type | Instruction |
---|---|---|
errMsg | String | Interface call result |
text | String | Plain text content |