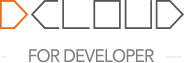
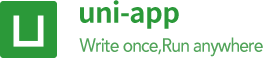
English
Get the current location and speed.
OBJECT parameter description
Parameter Name | Type | Required | Description | Platform Difference Description |
---|---|---|---|---|
type | String | 否 | 默认为 wgs84 返回 gps 坐标,gcj02 返回国测局坐标,可用于 uni.openLocation 和 map 组件坐标,App 和 H5 需配置定位 SDK 信息才可支持 gcj02。 | |
altitude | Boolean | 否 | 传入 true 会返回高度信息,由于获取高度需要较高精确度,会减慢接口返回速度 | 抖音小程序、飞书小程序、支付宝小程序不支持 |
geocode | Boolean | No | The default is false, whether to resolve the address information | Supported only by the App platform (Android needs to specify the type as gcj02 and configure the three-party positioning SDK) |
highAccuracyExpireTime | Number | No | High Accuracy Positioning Timeout Time (ms), returns the highest accuracy within the specified time, this value is more than 3000ms for high accuracy positioning to be effective | App (3.2.11+), H5 (3.2.11+), WeChat MiniApp(basic library 2.9.0+) |
timeout | String | No | The default is 5, the positioning timeout, in seconds | Only supported by MiniApp |
cacheTimeout | Number | No | Location cache timeout, in seconds; the current location data is cached every time, and the timestamp is recorded. When the next call is within cacheTimeout, the cached data will be returned | Only Feishu MiniApp, Alipay MiniApp Support |
accuracy | String | No | The default is high, specify the expected accuracy, support high, best. When specifying high, the expected precision value is 100m, and when specifying best, the expected precision value is 20m. When the accuracy obtained from the positioning does not meet the conditions, the positioning will continue before the timeout, and try to obtain the positioning results that meet the requirements | Only supported by MiniApp |
isHighAccuracy | Boolean | No | Enable high-precision positioning | App (3.4.0+), H5 (3.4.0+), WeChat MiniApp(basic library 2.9.0+) |
success | Function | is the callback function for the successful call of the interface. For details, please refer to the return parameter description. | ||
fail | Function | No | Callback function for interface call failure | |
complete | Function | No | The callback function of the end of the interface call (the call will be executed if the call succeeds or fails) |
success return parameter description
Parameters | Description |
---|---|
latitude | Latitude, a floating-point number, the range is -90~90, a negative number means south latitude |
longitude | Longitude, a floating point number, the range is -180~180, a negative number means west longitude |
speed | speed, float, unit m/s |
accuracy | Accuracy of location |
altitude | Altitude in m |
verticalAccuracy | Vertical Accuracy, in m (unavailable for Android, returns 0) |
horizontalAccuracy | Horizontal Accuracy, in m |
address | Address information (only supported by the App, you need to configure geocode to true) |
address address information description
property | type | description | description |
---|---|---|---|
country | String | Country | For example, "China", if this information cannot be obtained, return undefined |
province | String | province name | such as "Beijing", if this information cannot be obtained, return undefined |
city | String | City name | For example, "Beijing", if this information cannot be obtained, return undefined |
district | String | District (county) name | For example, "Chaoyang District", if this information cannot be obtained, return undefined |
street | String | Street information | For example, "Jiuxianqiao Road", if this information cannot be obtained, return undefined |
streetNum | String | Get the street number information | For example, "No. 3", if this information cannot be obtained, return undefined |
poiName | String | POI information | For example, "Electronic City. International Electronic Headquarters", if this information cannot be obtained, return undefined |
postalCode | String | Postal code | For example "100016", if this information cannot be obtained, return undefined |
cityCode | String | City Code | For example, "010", if this information cannot be obtained, return undefined |
Example
uni.getLocation({
type: 'wgs84',
success: function (res) {
console.log('当前位置的经度:' + res.longitude);
console.log('当前位置的纬度:' + res.latitude);
}
});
注意事项
H5 Platform
Android phone
cannot locate when the native app is embedded with H5, and the native app needs to process the Webview.移动端浏览器
普遍仅支持GPS定位,在GPS信号弱的地方可能定位失败。PC 设备
使用 Chrome 浏览器的时候,位置信息是连接谷歌服务器获取的,国内用户可能获取位置信息失败,推荐使用Edge进行获取位置信息Version 2.9.9 and above
, optimize uni.getLocation to support location by IP. By default, it is obtained through GPS. If the acquisition fails, the alternative is to obtain it through IP positioning, and the secret key of the third-party map service platform needs to be filled in. key configuration: manifest.json ---> H5 configuration ---> positioning and map ---> key.App Platform
<map>
component defaults to the coordinate gcj02 of the National Survey Bureau. When calling uni.getLocation
to return the result to the <map>
component, you need to specify the type as gcj02.getLocation
, it can be displayed on any map map, such as positioning using Gaode, and the map using google's webview version of the map. If the coordinate system is different, pay attention to converting the coordinate system.web-view
to load the map, there is no need to configure the sdk configuration of the map in the manifest.3.3.0 版本以上
优化系统定位模块,可不使用三方定位SDK的进行高精度定位,具体参考:系统定位。type: 'gcj02'
MiniApp Platform
plus.geolocation
to get the Chinese address. The App SDK configuration in the manifest isonly used for the app, and the MiniApp not need to be configured here.WeChat MiniApp Program
, when the user leaves the app, this interface cannot be called, and you need to apply for [Background continuous positioning permission](https://developers.weixin.qq.com/miniprogram/dev/framework/open-ability /authorize.html), and in the new version, you need to use [wx.onLocationChange]( https://developers.weixin.qq.com/miniprogram/dev/api/location/wx.onLocationChange.html ) to monitor location information changes; when This interface can continue to be called when the user clicks "Show on top of chat".Open the map to select a location.
Platform Difference Description
App | H5 | 微信小程序 | 支付宝小程序 | 百度小程序 | 抖音小程序、飞书小程序 | QQ小程序 | 快手小程序 | 京东小程序 |
---|---|---|---|---|---|---|---|---|
√ | √ | √ | √ | √ | √ | √ | x | x |
OBJECT parameter description
Parameter Name | Type | Required | Description | Platform Difference Description |
---|---|---|---|---|
latitude | Number | No | Destination latitude | WeChat MiniApp(2.9.0+), H5-Vue3 (3.2.10+) |
longitude | Number | No | Destination longitude | WeChat MiniApp(2.9.0+), H5-Vue3 (3.2.10+) |
keyword | String | No | Search keywords, only supported by App platform | |
success | Function | is the callback function for the successful call of the interface. For details, please refer to the return parameter description. | ||
fail | Function | No | The callback function of the failed API call (triggered when the location fails to be acquired, the user cancels, etc.) | |
complete | Function | No | The callback function of the end of the interface call (the call will be executed if the call succeeds or fails) |
Notice
success return parameter description
Parameters | Description |
---|---|
name | Location Name |
address | Detailed address |
latitude | Latitude, a floating-point number, the range is -90~90, a negative number represents the southern latitude, using the gcj02 National Survey Bureau coordinate system. |
longitude | Longitude, a floating-point number, the range is -180~180, the negative number represents the west longitude, using the gcj02 National Survey Bureau coordinate system. |
Example
uni.chooseLocation({
success: function (res) {
console.log('位置名称:' + res.name);
console.log('详细地址:' + res.address);
console.log('纬度:' + res.latitude);
console.log('经度:' + res.longitude);
}
});
Notice
app
, configured in the manifest, and it will take effect after packaging.app-nvue
, but Baidu Maps cannot be used. In addition, the API for selecting a map and viewing the map location only supports AutoNavi and Google Maps (3.4+). Therefore, if there is no special need on the App side, it is recommended to use the Gaode map.H5 terminal
uses maps and positioning related, you need to configure the secret key (key) applied by third-party map service providers such as Tencent or Google in manifest.json.WeChat built-in browser
, see detailsAndroid App端
位置不准,见上文 uni.getLocation 的注意事项使用三方定位或者地图服务,需向服务提供商(如:高德地图、百度地图、腾讯地图、谷歌地图)申请商业授权和缴纳费用(5万/年)。
DCloud为开发者争取了福利,可优惠获取高德、腾讯的商业授权。如有需求请发邮件到bd@dcloud.io
(注明你的公司名称、应用介绍、HBuilder账户);你也可以直接通过uni-im
发起在线咨询,在线咨询地址:DCloud地图服务专员。
详见:https://uniapp.dcloud.net.cn/tutorial/app-geolocation.html#lic
若想要实现城市选择功能,可以使用 unicloud-city-select
城市选择组件。
运行效果图
下载地址:https://ext.dcloud.net.cn/plugin?name=unicloud-city-select
文档地址:https://doc.dcloud.net.cn/uniCloud/unicloud-city-select.html